ASP.NET Testing sql query in c#
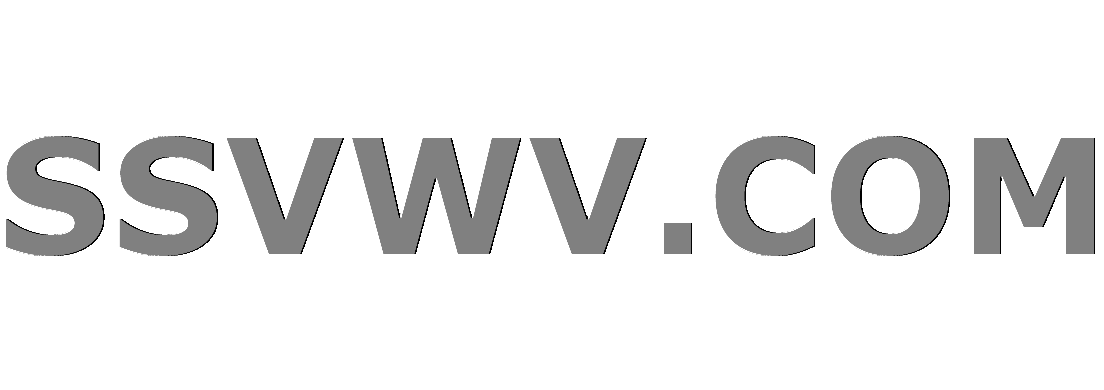
Multi tool use
up vote
0
down vote
favorite
As part of my Application Architecture Module at Uni I am testing the time taken to retrieve data from an MS SQL server local and remotely. I have a user interface using visual studio 2017 ASP.NET with c#. I instantiate and start a stopwatch control when a user clicks a button and stop the stopwatch when afer the line of code that bind the result to the gridview. I have two buttons, one runs the code to a local databaase created in visual studio and the other button runs identical code (except connectionstring) to remote SQL Server (GoDaddy). The results i am getting are very fast 30ms and 100ms remotely.
My question really is, are the times i am getting accurate for what i think its doing or it just giving me the time to create the page after postback.
Is there i way i can simulate simultaneous connections to each database to test performance under real world use.
protected void Btnlocal_Click(object sender, EventArgs e)
{
if (txthouseno.Text != "" && txtpostcode.Text != "")
{
Label4.Visible = true;
Stopwatch localStopWatch = new Stopwatch();
localStopWatch.Start();
string connectionString;
SqlConnection cnn;
connectionString = @"Data Source=(LocalDB)MSSQLLocalDB;AttachDbFilename=C:xxxxxxxxxxxxxxxx.mdf;Integrated Security=True;Connect Timeout=30";
cnn = new SqlConnection(connectionString);
cnn.Open();
lbllocal.Text = "Local Database Connected";
SqlCommand command;
SqlDataReader dataReader;
String sql = "select * from Customer where postcode = @custpcode AND houseno = @custhouseno";
command = new SqlCommand(sql, cnn);
var custpostParam = new SqlParameter("custpcode", SqlDbType.VarChar);
custpostParam.Value = txtpostcode.Text;
var custhouseParam = new SqlParameter("custhouseno", SqlDbType.VarChar);
custhouseParam.Value = txthouseno.Text;
command.Parameters.Add(custpostParam);
command.Parameters.Add(custhouseParam);
dataReader = command.ExecuteReader();
GridView1.DataSource = dataReader;
GridView1.DataBind();
localStopWatch.Stop();
command.Dispose();
cnn.Close();
connectionString = "";
lbllocal.Text = "Local PC Time Taken = " + localStopWatch.ElapsedMilliseconds.ToString() + " ms";
}
}
protected void Btnremote_Click(object sender, EventArgs e)
{
if (txthouseno.Text != "" && txtpostcode.Text != "")
{
Label5.Visible = true;
Stopwatch remoteStopWatch = new Stopwatch();
remoteStopWatch.Start();
ViewState["pageLoadTime"] = DateTime.Now;
string connectionString;
SqlConnection cnn;
connectionString = @"Data Source=xxx.xxx.xx.xxx;Integrated Security=False;User ID=xxxxxx; Password = xxxxxx; Connect Timeout=15;Encrypt=False;Packet Size=4096";
cnn = new SqlConnection(connectionString);
cnn.Open();
SqlCommand command;
SqlDataReader dataReader;
String sql = "select * from dbo.Customer where postcode = @custpcode AND houseno = @custhouseno";
command = new SqlCommand(sql, cnn);
var custpostParam = new SqlParameter("custpcode", SqlDbType.VarChar);
custpostParam.Value = txtpostcode.Text;
var custhouseParam = new SqlParameter("custhouseno", SqlDbType.VarChar);
custhouseParam.Value = txthouseno.Text;
command.Parameters.Add(custpostParam);
command.Parameters.Add(custhouseParam);
dataReader = command.ExecuteReader();
GridView2.DataSource = dataReader;
GridView2.DataBind();
remoteStopWatch.Stop();
command.Dispose();
cnn.Close();
connectionString = "";
lblremote.Text = "Remote Server Time Taken = " + remoteStopWatch.ElapsedMilliseconds.ToString() + " ms";
}
} enter code here
c# sql postback
add a comment |
up vote
0
down vote
favorite
As part of my Application Architecture Module at Uni I am testing the time taken to retrieve data from an MS SQL server local and remotely. I have a user interface using visual studio 2017 ASP.NET with c#. I instantiate and start a stopwatch control when a user clicks a button and stop the stopwatch when afer the line of code that bind the result to the gridview. I have two buttons, one runs the code to a local databaase created in visual studio and the other button runs identical code (except connectionstring) to remote SQL Server (GoDaddy). The results i am getting are very fast 30ms and 100ms remotely.
My question really is, are the times i am getting accurate for what i think its doing or it just giving me the time to create the page after postback.
Is there i way i can simulate simultaneous connections to each database to test performance under real world use.
protected void Btnlocal_Click(object sender, EventArgs e)
{
if (txthouseno.Text != "" && txtpostcode.Text != "")
{
Label4.Visible = true;
Stopwatch localStopWatch = new Stopwatch();
localStopWatch.Start();
string connectionString;
SqlConnection cnn;
connectionString = @"Data Source=(LocalDB)MSSQLLocalDB;AttachDbFilename=C:xxxxxxxxxxxxxxxx.mdf;Integrated Security=True;Connect Timeout=30";
cnn = new SqlConnection(connectionString);
cnn.Open();
lbllocal.Text = "Local Database Connected";
SqlCommand command;
SqlDataReader dataReader;
String sql = "select * from Customer where postcode = @custpcode AND houseno = @custhouseno";
command = new SqlCommand(sql, cnn);
var custpostParam = new SqlParameter("custpcode", SqlDbType.VarChar);
custpostParam.Value = txtpostcode.Text;
var custhouseParam = new SqlParameter("custhouseno", SqlDbType.VarChar);
custhouseParam.Value = txthouseno.Text;
command.Parameters.Add(custpostParam);
command.Parameters.Add(custhouseParam);
dataReader = command.ExecuteReader();
GridView1.DataSource = dataReader;
GridView1.DataBind();
localStopWatch.Stop();
command.Dispose();
cnn.Close();
connectionString = "";
lbllocal.Text = "Local PC Time Taken = " + localStopWatch.ElapsedMilliseconds.ToString() + " ms";
}
}
protected void Btnremote_Click(object sender, EventArgs e)
{
if (txthouseno.Text != "" && txtpostcode.Text != "")
{
Label5.Visible = true;
Stopwatch remoteStopWatch = new Stopwatch();
remoteStopWatch.Start();
ViewState["pageLoadTime"] = DateTime.Now;
string connectionString;
SqlConnection cnn;
connectionString = @"Data Source=xxx.xxx.xx.xxx;Integrated Security=False;User ID=xxxxxx; Password = xxxxxx; Connect Timeout=15;Encrypt=False;Packet Size=4096";
cnn = new SqlConnection(connectionString);
cnn.Open();
SqlCommand command;
SqlDataReader dataReader;
String sql = "select * from dbo.Customer where postcode = @custpcode AND houseno = @custhouseno";
command = new SqlCommand(sql, cnn);
var custpostParam = new SqlParameter("custpcode", SqlDbType.VarChar);
custpostParam.Value = txtpostcode.Text;
var custhouseParam = new SqlParameter("custhouseno", SqlDbType.VarChar);
custhouseParam.Value = txthouseno.Text;
command.Parameters.Add(custpostParam);
command.Parameters.Add(custhouseParam);
dataReader = command.ExecuteReader();
GridView2.DataSource = dataReader;
GridView2.DataBind();
remoteStopWatch.Stop();
command.Dispose();
cnn.Close();
connectionString = "";
lblremote.Text = "Remote Server Time Taken = " + remoteStopWatch.ElapsedMilliseconds.ToString() + " ms";
}
} enter code here
c# sql postback
A fairly quick and accurate way to get this data would be to add Application Insights to your app. You can either create a free instance on the Azure Portal, that your app will send data to, or just view the data locally in Visual Studio. The SQL calls will then appear as "Dependencies" complete with accurate timings.
– sellotape
Nov 10 at 16:33
Re your actually question, it seems reasonable that the SQL calls are complete when (or very close to when) you stop the stopwatch, as you're closing the connection and disposing the command then. Any deferred binding would not have access to these and would fail, so if he binding is indeed working, your timings are roughly correct.
– sellotape
Nov 10 at 16:39
Just remember that these are not SQL execution times, as the execution time would be less. What you're measuring is the time to go to SQL Server (through LAN/WAN), run the query and get the result back (LAN/WAN). If you need accurate SQL timing, you'll need SQL Server Profiler.
– Anderson Matos
Nov 10 at 16:58
Thanks for the comments.
– Allan McCaffery
Nov 11 at 14:45
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
As part of my Application Architecture Module at Uni I am testing the time taken to retrieve data from an MS SQL server local and remotely. I have a user interface using visual studio 2017 ASP.NET with c#. I instantiate and start a stopwatch control when a user clicks a button and stop the stopwatch when afer the line of code that bind the result to the gridview. I have two buttons, one runs the code to a local databaase created in visual studio and the other button runs identical code (except connectionstring) to remote SQL Server (GoDaddy). The results i am getting are very fast 30ms and 100ms remotely.
My question really is, are the times i am getting accurate for what i think its doing or it just giving me the time to create the page after postback.
Is there i way i can simulate simultaneous connections to each database to test performance under real world use.
protected void Btnlocal_Click(object sender, EventArgs e)
{
if (txthouseno.Text != "" && txtpostcode.Text != "")
{
Label4.Visible = true;
Stopwatch localStopWatch = new Stopwatch();
localStopWatch.Start();
string connectionString;
SqlConnection cnn;
connectionString = @"Data Source=(LocalDB)MSSQLLocalDB;AttachDbFilename=C:xxxxxxxxxxxxxxxx.mdf;Integrated Security=True;Connect Timeout=30";
cnn = new SqlConnection(connectionString);
cnn.Open();
lbllocal.Text = "Local Database Connected";
SqlCommand command;
SqlDataReader dataReader;
String sql = "select * from Customer where postcode = @custpcode AND houseno = @custhouseno";
command = new SqlCommand(sql, cnn);
var custpostParam = new SqlParameter("custpcode", SqlDbType.VarChar);
custpostParam.Value = txtpostcode.Text;
var custhouseParam = new SqlParameter("custhouseno", SqlDbType.VarChar);
custhouseParam.Value = txthouseno.Text;
command.Parameters.Add(custpostParam);
command.Parameters.Add(custhouseParam);
dataReader = command.ExecuteReader();
GridView1.DataSource = dataReader;
GridView1.DataBind();
localStopWatch.Stop();
command.Dispose();
cnn.Close();
connectionString = "";
lbllocal.Text = "Local PC Time Taken = " + localStopWatch.ElapsedMilliseconds.ToString() + " ms";
}
}
protected void Btnremote_Click(object sender, EventArgs e)
{
if (txthouseno.Text != "" && txtpostcode.Text != "")
{
Label5.Visible = true;
Stopwatch remoteStopWatch = new Stopwatch();
remoteStopWatch.Start();
ViewState["pageLoadTime"] = DateTime.Now;
string connectionString;
SqlConnection cnn;
connectionString = @"Data Source=xxx.xxx.xx.xxx;Integrated Security=False;User ID=xxxxxx; Password = xxxxxx; Connect Timeout=15;Encrypt=False;Packet Size=4096";
cnn = new SqlConnection(connectionString);
cnn.Open();
SqlCommand command;
SqlDataReader dataReader;
String sql = "select * from dbo.Customer where postcode = @custpcode AND houseno = @custhouseno";
command = new SqlCommand(sql, cnn);
var custpostParam = new SqlParameter("custpcode", SqlDbType.VarChar);
custpostParam.Value = txtpostcode.Text;
var custhouseParam = new SqlParameter("custhouseno", SqlDbType.VarChar);
custhouseParam.Value = txthouseno.Text;
command.Parameters.Add(custpostParam);
command.Parameters.Add(custhouseParam);
dataReader = command.ExecuteReader();
GridView2.DataSource = dataReader;
GridView2.DataBind();
remoteStopWatch.Stop();
command.Dispose();
cnn.Close();
connectionString = "";
lblremote.Text = "Remote Server Time Taken = " + remoteStopWatch.ElapsedMilliseconds.ToString() + " ms";
}
} enter code here
c# sql postback
As part of my Application Architecture Module at Uni I am testing the time taken to retrieve data from an MS SQL server local and remotely. I have a user interface using visual studio 2017 ASP.NET with c#. I instantiate and start a stopwatch control when a user clicks a button and stop the stopwatch when afer the line of code that bind the result to the gridview. I have two buttons, one runs the code to a local databaase created in visual studio and the other button runs identical code (except connectionstring) to remote SQL Server (GoDaddy). The results i am getting are very fast 30ms and 100ms remotely.
My question really is, are the times i am getting accurate for what i think its doing or it just giving me the time to create the page after postback.
Is there i way i can simulate simultaneous connections to each database to test performance under real world use.
protected void Btnlocal_Click(object sender, EventArgs e)
{
if (txthouseno.Text != "" && txtpostcode.Text != "")
{
Label4.Visible = true;
Stopwatch localStopWatch = new Stopwatch();
localStopWatch.Start();
string connectionString;
SqlConnection cnn;
connectionString = @"Data Source=(LocalDB)MSSQLLocalDB;AttachDbFilename=C:xxxxxxxxxxxxxxxx.mdf;Integrated Security=True;Connect Timeout=30";
cnn = new SqlConnection(connectionString);
cnn.Open();
lbllocal.Text = "Local Database Connected";
SqlCommand command;
SqlDataReader dataReader;
String sql = "select * from Customer where postcode = @custpcode AND houseno = @custhouseno";
command = new SqlCommand(sql, cnn);
var custpostParam = new SqlParameter("custpcode", SqlDbType.VarChar);
custpostParam.Value = txtpostcode.Text;
var custhouseParam = new SqlParameter("custhouseno", SqlDbType.VarChar);
custhouseParam.Value = txthouseno.Text;
command.Parameters.Add(custpostParam);
command.Parameters.Add(custhouseParam);
dataReader = command.ExecuteReader();
GridView1.DataSource = dataReader;
GridView1.DataBind();
localStopWatch.Stop();
command.Dispose();
cnn.Close();
connectionString = "";
lbllocal.Text = "Local PC Time Taken = " + localStopWatch.ElapsedMilliseconds.ToString() + " ms";
}
}
protected void Btnremote_Click(object sender, EventArgs e)
{
if (txthouseno.Text != "" && txtpostcode.Text != "")
{
Label5.Visible = true;
Stopwatch remoteStopWatch = new Stopwatch();
remoteStopWatch.Start();
ViewState["pageLoadTime"] = DateTime.Now;
string connectionString;
SqlConnection cnn;
connectionString = @"Data Source=xxx.xxx.xx.xxx;Integrated Security=False;User ID=xxxxxx; Password = xxxxxx; Connect Timeout=15;Encrypt=False;Packet Size=4096";
cnn = new SqlConnection(connectionString);
cnn.Open();
SqlCommand command;
SqlDataReader dataReader;
String sql = "select * from dbo.Customer where postcode = @custpcode AND houseno = @custhouseno";
command = new SqlCommand(sql, cnn);
var custpostParam = new SqlParameter("custpcode", SqlDbType.VarChar);
custpostParam.Value = txtpostcode.Text;
var custhouseParam = new SqlParameter("custhouseno", SqlDbType.VarChar);
custhouseParam.Value = txthouseno.Text;
command.Parameters.Add(custpostParam);
command.Parameters.Add(custhouseParam);
dataReader = command.ExecuteReader();
GridView2.DataSource = dataReader;
GridView2.DataBind();
remoteStopWatch.Stop();
command.Dispose();
cnn.Close();
connectionString = "";
lblremote.Text = "Remote Server Time Taken = " + remoteStopWatch.ElapsedMilliseconds.ToString() + " ms";
}
} enter code here
c# sql postback
c# sql postback
asked Nov 10 at 16:25
Allan McCaffery
11
11
A fairly quick and accurate way to get this data would be to add Application Insights to your app. You can either create a free instance on the Azure Portal, that your app will send data to, or just view the data locally in Visual Studio. The SQL calls will then appear as "Dependencies" complete with accurate timings.
– sellotape
Nov 10 at 16:33
Re your actually question, it seems reasonable that the SQL calls are complete when (or very close to when) you stop the stopwatch, as you're closing the connection and disposing the command then. Any deferred binding would not have access to these and would fail, so if he binding is indeed working, your timings are roughly correct.
– sellotape
Nov 10 at 16:39
Just remember that these are not SQL execution times, as the execution time would be less. What you're measuring is the time to go to SQL Server (through LAN/WAN), run the query and get the result back (LAN/WAN). If you need accurate SQL timing, you'll need SQL Server Profiler.
– Anderson Matos
Nov 10 at 16:58
Thanks for the comments.
– Allan McCaffery
Nov 11 at 14:45
add a comment |
A fairly quick and accurate way to get this data would be to add Application Insights to your app. You can either create a free instance on the Azure Portal, that your app will send data to, or just view the data locally in Visual Studio. The SQL calls will then appear as "Dependencies" complete with accurate timings.
– sellotape
Nov 10 at 16:33
Re your actually question, it seems reasonable that the SQL calls are complete when (or very close to when) you stop the stopwatch, as you're closing the connection and disposing the command then. Any deferred binding would not have access to these and would fail, so if he binding is indeed working, your timings are roughly correct.
– sellotape
Nov 10 at 16:39
Just remember that these are not SQL execution times, as the execution time would be less. What you're measuring is the time to go to SQL Server (through LAN/WAN), run the query and get the result back (LAN/WAN). If you need accurate SQL timing, you'll need SQL Server Profiler.
– Anderson Matos
Nov 10 at 16:58
Thanks for the comments.
– Allan McCaffery
Nov 11 at 14:45
A fairly quick and accurate way to get this data would be to add Application Insights to your app. You can either create a free instance on the Azure Portal, that your app will send data to, or just view the data locally in Visual Studio. The SQL calls will then appear as "Dependencies" complete with accurate timings.
– sellotape
Nov 10 at 16:33
A fairly quick and accurate way to get this data would be to add Application Insights to your app. You can either create a free instance on the Azure Portal, that your app will send data to, or just view the data locally in Visual Studio. The SQL calls will then appear as "Dependencies" complete with accurate timings.
– sellotape
Nov 10 at 16:33
Re your actually question, it seems reasonable that the SQL calls are complete when (or very close to when) you stop the stopwatch, as you're closing the connection and disposing the command then. Any deferred binding would not have access to these and would fail, so if he binding is indeed working, your timings are roughly correct.
– sellotape
Nov 10 at 16:39
Re your actually question, it seems reasonable that the SQL calls are complete when (or very close to when) you stop the stopwatch, as you're closing the connection and disposing the command then. Any deferred binding would not have access to these and would fail, so if he binding is indeed working, your timings are roughly correct.
– sellotape
Nov 10 at 16:39
Just remember that these are not SQL execution times, as the execution time would be less. What you're measuring is the time to go to SQL Server (through LAN/WAN), run the query and get the result back (LAN/WAN). If you need accurate SQL timing, you'll need SQL Server Profiler.
– Anderson Matos
Nov 10 at 16:58
Just remember that these are not SQL execution times, as the execution time would be less. What you're measuring is the time to go to SQL Server (through LAN/WAN), run the query and get the result back (LAN/WAN). If you need accurate SQL timing, you'll need SQL Server Profiler.
– Anderson Matos
Nov 10 at 16:58
Thanks for the comments.
– Allan McCaffery
Nov 11 at 14:45
Thanks for the comments.
– Allan McCaffery
Nov 11 at 14:45
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53240955%2fasp-net-testing-sql-query-in-c-sharp%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
VSKqKvp0 0 LmTjefB
A fairly quick and accurate way to get this data would be to add Application Insights to your app. You can either create a free instance on the Azure Portal, that your app will send data to, or just view the data locally in Visual Studio. The SQL calls will then appear as "Dependencies" complete with accurate timings.
– sellotape
Nov 10 at 16:33
Re your actually question, it seems reasonable that the SQL calls are complete when (or very close to when) you stop the stopwatch, as you're closing the connection and disposing the command then. Any deferred binding would not have access to these and would fail, so if he binding is indeed working, your timings are roughly correct.
– sellotape
Nov 10 at 16:39
Just remember that these are not SQL execution times, as the execution time would be less. What you're measuring is the time to go to SQL Server (through LAN/WAN), run the query and get the result back (LAN/WAN). If you need accurate SQL timing, you'll need SQL Server Profiler.
– Anderson Matos
Nov 10 at 16:58
Thanks for the comments.
– Allan McCaffery
Nov 11 at 14:45