What data type do I store 16 digit number into database?
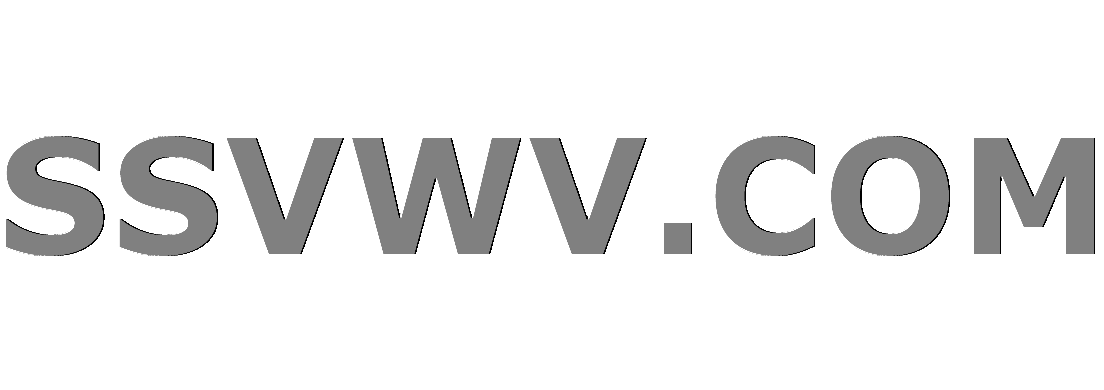
Multi tool use
up vote
0
down vote
favorite
I am new to C#, so sorry if this is an easy question.
I have a database connected to my datagrid via MS-Access. I have the user input the information and save it to the database. I am having a problem where the "CARD NUMBER" cannot be 16 digits, as it throws an error for data type. thank you in advance!
OleDbCommand updateQuery = new OleDbCommand("INSERT INTO Profiles ([PROFILE NAME],[LOGIN EMAIL],[PASSWORD],[FULL NAME],[CARD NUMBER],[EXP MONTH],[EXP YEAR],CVV) VALUES(@name1,@email,@pass,@name2,@card,@expm,@expy,@cvv)", connection);
updateQuery.Parameters.Add("@name1", OleDbType.Variant).Value = textBox4.Text; //Profile Name
updateQuery.Parameters.Add("@email", OleDbType.Variant).Value = textBox2.Text; //Email
updateQuery.Parameters.Add("@pass", OleDbType.Variant).Value = textBox3.Text; //Pass
updateQuery.Parameters.Add("@name2", OleDbType.Variant).Value = textBox1.Text; //Full Name
updateQuery.Parameters.Add("@card", OleDbType.VarNumeric).Value = textBox5.Text; //CardNumber
updateQuery.Parameters.Add("@expm", OleDbType.Numeric).Value = comboBox1.Text; //EXPMonth
updateQuery.Parameters.Add("@expy", OleDbType.Numeric).Value = comboBox2.Text; //EXPYear
updateQuery.Parameters.Add("@cvv", OleDbType.Numeric).Value = textBox7.Text; //CVV
updateQuery.ExecuteNonQuery();
connection.Close();
MessageBox.Show("Profile Saved");
this.Close();
RefreshDBConnection();
Note: I changed my code to:
updateQuery.Parameters.Add("@card", OleDbType.VarNumeric).Value = textBox5.Text; //CardNumber
and now it throws an overflow error. Not sure what's happening.
c# ms-access types
New contributor
Caden Buckelew is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
favorite
I am new to C#, so sorry if this is an easy question.
I have a database connected to my datagrid via MS-Access. I have the user input the information and save it to the database. I am having a problem where the "CARD NUMBER" cannot be 16 digits, as it throws an error for data type. thank you in advance!
OleDbCommand updateQuery = new OleDbCommand("INSERT INTO Profiles ([PROFILE NAME],[LOGIN EMAIL],[PASSWORD],[FULL NAME],[CARD NUMBER],[EXP MONTH],[EXP YEAR],CVV) VALUES(@name1,@email,@pass,@name2,@card,@expm,@expy,@cvv)", connection);
updateQuery.Parameters.Add("@name1", OleDbType.Variant).Value = textBox4.Text; //Profile Name
updateQuery.Parameters.Add("@email", OleDbType.Variant).Value = textBox2.Text; //Email
updateQuery.Parameters.Add("@pass", OleDbType.Variant).Value = textBox3.Text; //Pass
updateQuery.Parameters.Add("@name2", OleDbType.Variant).Value = textBox1.Text; //Full Name
updateQuery.Parameters.Add("@card", OleDbType.VarNumeric).Value = textBox5.Text; //CardNumber
updateQuery.Parameters.Add("@expm", OleDbType.Numeric).Value = comboBox1.Text; //EXPMonth
updateQuery.Parameters.Add("@expy", OleDbType.Numeric).Value = comboBox2.Text; //EXPYear
updateQuery.Parameters.Add("@cvv", OleDbType.Numeric).Value = textBox7.Text; //CVV
updateQuery.ExecuteNonQuery();
connection.Close();
MessageBox.Show("Profile Saved");
this.Close();
RefreshDBConnection();
Note: I changed my code to:
updateQuery.Parameters.Add("@card", OleDbType.VarNumeric).Value = textBox5.Text; //CardNumber
and now it throws an overflow error. Not sure what's happening.
c# ms-access types
New contributor
Caden Buckelew is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
1
Are you going to do arithmetic with that number? If not, just store it as a string.
– Sweeper
18 hours ago
How do I store as a string though?
– Caden Buckelew
18 hours ago
The same way you storename1
.
– Stijn
18 hours ago
1
FYI it's possible to change the name of a text box, so you can give it a name that is more clear thantextBox4
etc.
– Stijn
18 hours ago
I then get the error 'Overflow' @Stijn
– Caden Buckelew
18 hours ago
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I am new to C#, so sorry if this is an easy question.
I have a database connected to my datagrid via MS-Access. I have the user input the information and save it to the database. I am having a problem where the "CARD NUMBER" cannot be 16 digits, as it throws an error for data type. thank you in advance!
OleDbCommand updateQuery = new OleDbCommand("INSERT INTO Profiles ([PROFILE NAME],[LOGIN EMAIL],[PASSWORD],[FULL NAME],[CARD NUMBER],[EXP MONTH],[EXP YEAR],CVV) VALUES(@name1,@email,@pass,@name2,@card,@expm,@expy,@cvv)", connection);
updateQuery.Parameters.Add("@name1", OleDbType.Variant).Value = textBox4.Text; //Profile Name
updateQuery.Parameters.Add("@email", OleDbType.Variant).Value = textBox2.Text; //Email
updateQuery.Parameters.Add("@pass", OleDbType.Variant).Value = textBox3.Text; //Pass
updateQuery.Parameters.Add("@name2", OleDbType.Variant).Value = textBox1.Text; //Full Name
updateQuery.Parameters.Add("@card", OleDbType.VarNumeric).Value = textBox5.Text; //CardNumber
updateQuery.Parameters.Add("@expm", OleDbType.Numeric).Value = comboBox1.Text; //EXPMonth
updateQuery.Parameters.Add("@expy", OleDbType.Numeric).Value = comboBox2.Text; //EXPYear
updateQuery.Parameters.Add("@cvv", OleDbType.Numeric).Value = textBox7.Text; //CVV
updateQuery.ExecuteNonQuery();
connection.Close();
MessageBox.Show("Profile Saved");
this.Close();
RefreshDBConnection();
Note: I changed my code to:
updateQuery.Parameters.Add("@card", OleDbType.VarNumeric).Value = textBox5.Text; //CardNumber
and now it throws an overflow error. Not sure what's happening.
c# ms-access types
New contributor
Caden Buckelew is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
I am new to C#, so sorry if this is an easy question.
I have a database connected to my datagrid via MS-Access. I have the user input the information and save it to the database. I am having a problem where the "CARD NUMBER" cannot be 16 digits, as it throws an error for data type. thank you in advance!
OleDbCommand updateQuery = new OleDbCommand("INSERT INTO Profiles ([PROFILE NAME],[LOGIN EMAIL],[PASSWORD],[FULL NAME],[CARD NUMBER],[EXP MONTH],[EXP YEAR],CVV) VALUES(@name1,@email,@pass,@name2,@card,@expm,@expy,@cvv)", connection);
updateQuery.Parameters.Add("@name1", OleDbType.Variant).Value = textBox4.Text; //Profile Name
updateQuery.Parameters.Add("@email", OleDbType.Variant).Value = textBox2.Text; //Email
updateQuery.Parameters.Add("@pass", OleDbType.Variant).Value = textBox3.Text; //Pass
updateQuery.Parameters.Add("@name2", OleDbType.Variant).Value = textBox1.Text; //Full Name
updateQuery.Parameters.Add("@card", OleDbType.VarNumeric).Value = textBox5.Text; //CardNumber
updateQuery.Parameters.Add("@expm", OleDbType.Numeric).Value = comboBox1.Text; //EXPMonth
updateQuery.Parameters.Add("@expy", OleDbType.Numeric).Value = comboBox2.Text; //EXPYear
updateQuery.Parameters.Add("@cvv", OleDbType.Numeric).Value = textBox7.Text; //CVV
updateQuery.ExecuteNonQuery();
connection.Close();
MessageBox.Show("Profile Saved");
this.Close();
RefreshDBConnection();
Note: I changed my code to:
updateQuery.Parameters.Add("@card", OleDbType.VarNumeric).Value = textBox5.Text; //CardNumber
and now it throws an overflow error. Not sure what's happening.
c# ms-access types
c# ms-access types
New contributor
Caden Buckelew is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Caden Buckelew is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
edited 18 hours ago
marc_s
564k12510871240
564k12510871240
New contributor
Caden Buckelew is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked 18 hours ago


Caden Buckelew
155
155
New contributor
Caden Buckelew is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Caden Buckelew is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Caden Buckelew is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
1
Are you going to do arithmetic with that number? If not, just store it as a string.
– Sweeper
18 hours ago
How do I store as a string though?
– Caden Buckelew
18 hours ago
The same way you storename1
.
– Stijn
18 hours ago
1
FYI it's possible to change the name of a text box, so you can give it a name that is more clear thantextBox4
etc.
– Stijn
18 hours ago
I then get the error 'Overflow' @Stijn
– Caden Buckelew
18 hours ago
add a comment |
1
Are you going to do arithmetic with that number? If not, just store it as a string.
– Sweeper
18 hours ago
How do I store as a string though?
– Caden Buckelew
18 hours ago
The same way you storename1
.
– Stijn
18 hours ago
1
FYI it's possible to change the name of a text box, so you can give it a name that is more clear thantextBox4
etc.
– Stijn
18 hours ago
I then get the error 'Overflow' @Stijn
– Caden Buckelew
18 hours ago
1
1
Are you going to do arithmetic with that number? If not, just store it as a string.
– Sweeper
18 hours ago
Are you going to do arithmetic with that number? If not, just store it as a string.
– Sweeper
18 hours ago
How do I store as a string though?
– Caden Buckelew
18 hours ago
How do I store as a string though?
– Caden Buckelew
18 hours ago
The same way you store
name1
.– Stijn
18 hours ago
The same way you store
name1
.– Stijn
18 hours ago
1
1
FYI it's possible to change the name of a text box, so you can give it a name that is more clear than
textBox4
etc.– Stijn
18 hours ago
FYI it's possible to change the name of a text box, so you can give it a name that is more clear than
textBox4
etc.– Stijn
18 hours ago
I then get the error 'Overflow' @Stijn
– Caden Buckelew
18 hours ago
I then get the error 'Overflow' @Stijn
– Caden Buckelew
18 hours ago
add a comment |
2 Answers
2
active
oldest
votes
up vote
0
down vote
accepted
what is the datatype in db, if it is int!!! change it to BigInt and it will work :)
New contributor
vijay is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Note that BigInt support is only available in Access 2016 and later.
– Stijn
18 hours ago
BigInt isn't an option, only Number. Maybe I can save it as a later version? instead of a .mbd file
– Caden Buckelew
18 hours ago
1
I strongly recommend not using BigInt for external applications. Like Stijn said, it's Access 2016 only, but also only later builds of Access 2016, and the Access Database Engine Redistributable is behind in versioning. Obviously, it's accdb only.
– Erik von Asmuth
18 hours ago
I changed it from number to long text and it works now :)
– Caden Buckelew
18 hours ago
add a comment |
up vote
2
down vote
Using BigInt will cause severe limitations as already noted, and using Long Text (Note field) is the worst thing you could do.
So, either use Short Text or Decimal set to hold at least 16 digits:
What's bad about using a long text?, and I only have short text, long text, and number options on my ms access file.
– Caden Buckelew
8 hours ago
Here is one reason, @caden-buckelew. Others could be found in that link.
– marlan
6 hours ago
No index is another. You can put it: There is not a single good reason to use Long Text to hold 16 characters.
– Gustav
6 hours ago
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
what is the datatype in db, if it is int!!! change it to BigInt and it will work :)
New contributor
vijay is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Note that BigInt support is only available in Access 2016 and later.
– Stijn
18 hours ago
BigInt isn't an option, only Number. Maybe I can save it as a later version? instead of a .mbd file
– Caden Buckelew
18 hours ago
1
I strongly recommend not using BigInt for external applications. Like Stijn said, it's Access 2016 only, but also only later builds of Access 2016, and the Access Database Engine Redistributable is behind in versioning. Obviously, it's accdb only.
– Erik von Asmuth
18 hours ago
I changed it from number to long text and it works now :)
– Caden Buckelew
18 hours ago
add a comment |
up vote
0
down vote
accepted
what is the datatype in db, if it is int!!! change it to BigInt and it will work :)
New contributor
vijay is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Note that BigInt support is only available in Access 2016 and later.
– Stijn
18 hours ago
BigInt isn't an option, only Number. Maybe I can save it as a later version? instead of a .mbd file
– Caden Buckelew
18 hours ago
1
I strongly recommend not using BigInt for external applications. Like Stijn said, it's Access 2016 only, but also only later builds of Access 2016, and the Access Database Engine Redistributable is behind in versioning. Obviously, it's accdb only.
– Erik von Asmuth
18 hours ago
I changed it from number to long text and it works now :)
– Caden Buckelew
18 hours ago
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
what is the datatype in db, if it is int!!! change it to BigInt and it will work :)
New contributor
vijay is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
what is the datatype in db, if it is int!!! change it to BigInt and it will work :)
New contributor
vijay is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
vijay is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
answered 18 hours ago


vijay
143
143
New contributor
vijay is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
vijay is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
vijay is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Note that BigInt support is only available in Access 2016 and later.
– Stijn
18 hours ago
BigInt isn't an option, only Number. Maybe I can save it as a later version? instead of a .mbd file
– Caden Buckelew
18 hours ago
1
I strongly recommend not using BigInt for external applications. Like Stijn said, it's Access 2016 only, but also only later builds of Access 2016, and the Access Database Engine Redistributable is behind in versioning. Obviously, it's accdb only.
– Erik von Asmuth
18 hours ago
I changed it from number to long text and it works now :)
– Caden Buckelew
18 hours ago
add a comment |
Note that BigInt support is only available in Access 2016 and later.
– Stijn
18 hours ago
BigInt isn't an option, only Number. Maybe I can save it as a later version? instead of a .mbd file
– Caden Buckelew
18 hours ago
1
I strongly recommend not using BigInt for external applications. Like Stijn said, it's Access 2016 only, but also only later builds of Access 2016, and the Access Database Engine Redistributable is behind in versioning. Obviously, it's accdb only.
– Erik von Asmuth
18 hours ago
I changed it from number to long text and it works now :)
– Caden Buckelew
18 hours ago
Note that BigInt support is only available in Access 2016 and later.
– Stijn
18 hours ago
Note that BigInt support is only available in Access 2016 and later.
– Stijn
18 hours ago
BigInt isn't an option, only Number. Maybe I can save it as a later version? instead of a .mbd file
– Caden Buckelew
18 hours ago
BigInt isn't an option, only Number. Maybe I can save it as a later version? instead of a .mbd file
– Caden Buckelew
18 hours ago
1
1
I strongly recommend not using BigInt for external applications. Like Stijn said, it's Access 2016 only, but also only later builds of Access 2016, and the Access Database Engine Redistributable is behind in versioning. Obviously, it's accdb only.
– Erik von Asmuth
18 hours ago
I strongly recommend not using BigInt for external applications. Like Stijn said, it's Access 2016 only, but also only later builds of Access 2016, and the Access Database Engine Redistributable is behind in versioning. Obviously, it's accdb only.
– Erik von Asmuth
18 hours ago
I changed it from number to long text and it works now :)
– Caden Buckelew
18 hours ago
I changed it from number to long text and it works now :)
– Caden Buckelew
18 hours ago
add a comment |
up vote
2
down vote
Using BigInt will cause severe limitations as already noted, and using Long Text (Note field) is the worst thing you could do.
So, either use Short Text or Decimal set to hold at least 16 digits:
What's bad about using a long text?, and I only have short text, long text, and number options on my ms access file.
– Caden Buckelew
8 hours ago
Here is one reason, @caden-buckelew. Others could be found in that link.
– marlan
6 hours ago
No index is another. You can put it: There is not a single good reason to use Long Text to hold 16 characters.
– Gustav
6 hours ago
add a comment |
up vote
2
down vote
Using BigInt will cause severe limitations as already noted, and using Long Text (Note field) is the worst thing you could do.
So, either use Short Text or Decimal set to hold at least 16 digits:
What's bad about using a long text?, and I only have short text, long text, and number options on my ms access file.
– Caden Buckelew
8 hours ago
Here is one reason, @caden-buckelew. Others could be found in that link.
– marlan
6 hours ago
No index is another. You can put it: There is not a single good reason to use Long Text to hold 16 characters.
– Gustav
6 hours ago
add a comment |
up vote
2
down vote
up vote
2
down vote
Using BigInt will cause severe limitations as already noted, and using Long Text (Note field) is the worst thing you could do.
So, either use Short Text or Decimal set to hold at least 16 digits:
Using BigInt will cause severe limitations as already noted, and using Long Text (Note field) is the worst thing you could do.
So, either use Short Text or Decimal set to hold at least 16 digits:
answered 13 hours ago


Gustav
28.5k51734
28.5k51734
What's bad about using a long text?, and I only have short text, long text, and number options on my ms access file.
– Caden Buckelew
8 hours ago
Here is one reason, @caden-buckelew. Others could be found in that link.
– marlan
6 hours ago
No index is another. You can put it: There is not a single good reason to use Long Text to hold 16 characters.
– Gustav
6 hours ago
add a comment |
What's bad about using a long text?, and I only have short text, long text, and number options on my ms access file.
– Caden Buckelew
8 hours ago
Here is one reason, @caden-buckelew. Others could be found in that link.
– marlan
6 hours ago
No index is another. You can put it: There is not a single good reason to use Long Text to hold 16 characters.
– Gustav
6 hours ago
What's bad about using a long text?, and I only have short text, long text, and number options on my ms access file.
– Caden Buckelew
8 hours ago
What's bad about using a long text?, and I only have short text, long text, and number options on my ms access file.
– Caden Buckelew
8 hours ago
Here is one reason, @caden-buckelew. Others could be found in that link.
– marlan
6 hours ago
Here is one reason, @caden-buckelew. Others could be found in that link.
– marlan
6 hours ago
No index is another. You can put it: There is not a single good reason to use Long Text to hold 16 characters.
– Gustav
6 hours ago
No index is another. You can put it: There is not a single good reason to use Long Text to hold 16 characters.
– Gustav
6 hours ago
add a comment |
Caden Buckelew is a new contributor. Be nice, and check out our Code of Conduct.
Caden Buckelew is a new contributor. Be nice, and check out our Code of Conduct.
Caden Buckelew is a new contributor. Be nice, and check out our Code of Conduct.
Caden Buckelew is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53237531%2fwhat-data-type-do-i-store-16-digit-number-into-database%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
DmwfAYwK5Rb4BU39s PyQZqm,D9UzgawfvE6vr,E5TLRn Q0Wr
1
Are you going to do arithmetic with that number? If not, just store it as a string.
– Sweeper
18 hours ago
How do I store as a string though?
– Caden Buckelew
18 hours ago
The same way you store
name1
.– Stijn
18 hours ago
1
FYI it's possible to change the name of a text box, so you can give it a name that is more clear than
textBox4
etc.– Stijn
18 hours ago
I then get the error 'Overflow' @Stijn
– Caden Buckelew
18 hours ago