javascript: how to convert a variable to a function with the same name that returns the original value
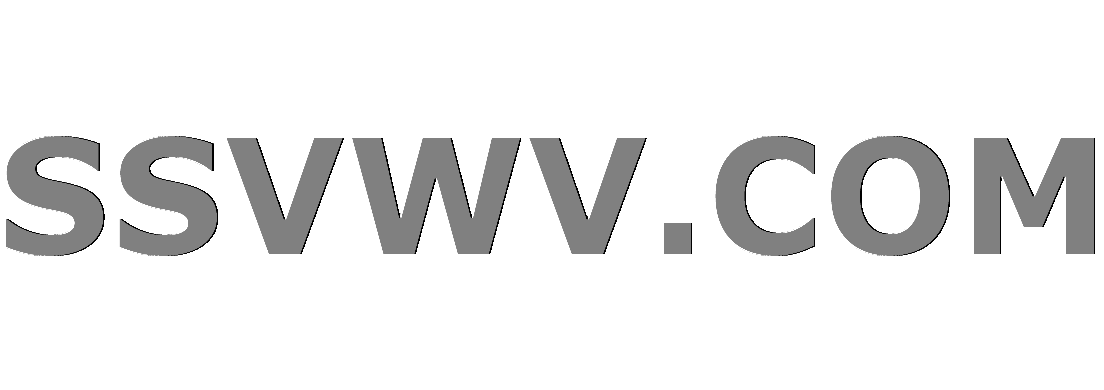
Multi tool use
up vote
1
down vote
favorite
let's say I have a numeric variable (or any other type),
I want to convert this variable to a function which has the same variable name and returns the original value (without using additional variables)
var x=1
x=()=>x
this will copy x by reference, not by value, so the new x will always returns itself (i.e a function) not the original value
I reached the result using an additional variable (temp)
var x=1
var temp=x
var x=()=>temp
what I want is to copy this variable by value, not by reference
I know how to copy an object to another object, but it is not the same case
note: using the same name is important for the flow of the next code (i.e: I have to use a function, if another type provided, I have to convert it to a function of the same name)
javascript node.js reference clone
|
show 2 more comments
up vote
1
down vote
favorite
let's say I have a numeric variable (or any other type),
I want to convert this variable to a function which has the same variable name and returns the original value (without using additional variables)
var x=1
x=()=>x
this will copy x by reference, not by value, so the new x will always returns itself (i.e a function) not the original value
I reached the result using an additional variable (temp)
var x=1
var temp=x
var x=()=>temp
what I want is to copy this variable by value, not by reference
I know how to copy an object to another object, but it is not the same case
note: using the same name is important for the flow of the next code (i.e: I have to use a function, if another type provided, I have to convert it to a function of the same name)
javascript node.js reference clone
Sounds very much like an X/Y problem, but doesarguments
orthis
count as a variable in your situation? :P (I'm assuming that defining a parameter would count as a variable) Or, can you explain what the problem is with using another variable?
– CertainPerformance
23 hours ago
4
JavaScript only has call/pass by value, never call/pass by reference. Either way, I don't see how these concepts are related to what you want to do. You cannot have the same variable refer to two different values at the same. You have to introduce another variable one way or the other. If you want to have it more "self-contained", use an IIFE:x = (x => () => x)(x);
(I guess technically this is an "IIAF" (immediately invoked arrow function)).
– Felix Kling
23 hours ago
You're trying to do some thing that isn't natural for JS. It's unclear what the purpose is but it's very likely that this is XY problem, as it was already mentioned.
– estus
21 hours ago
What's the use case for this, kindly?
– Sello Mkantjwa
20 hours ago
all values passed to the next function must be a function so I have to convert any non-function value to a function that returns the original value @estus
– xx yy
20 hours ago
|
show 2 more comments
up vote
1
down vote
favorite
up vote
1
down vote
favorite
let's say I have a numeric variable (or any other type),
I want to convert this variable to a function which has the same variable name and returns the original value (without using additional variables)
var x=1
x=()=>x
this will copy x by reference, not by value, so the new x will always returns itself (i.e a function) not the original value
I reached the result using an additional variable (temp)
var x=1
var temp=x
var x=()=>temp
what I want is to copy this variable by value, not by reference
I know how to copy an object to another object, but it is not the same case
note: using the same name is important for the flow of the next code (i.e: I have to use a function, if another type provided, I have to convert it to a function of the same name)
javascript node.js reference clone
let's say I have a numeric variable (or any other type),
I want to convert this variable to a function which has the same variable name and returns the original value (without using additional variables)
var x=1
x=()=>x
this will copy x by reference, not by value, so the new x will always returns itself (i.e a function) not the original value
I reached the result using an additional variable (temp)
var x=1
var temp=x
var x=()=>temp
what I want is to copy this variable by value, not by reference
I know how to copy an object to another object, but it is not the same case
note: using the same name is important for the flow of the next code (i.e: I have to use a function, if another type provided, I have to convert it to a function of the same name)
javascript node.js reference clone
javascript node.js reference clone
edited 21 hours ago
Sridhar
4,90732636
4,90732636
asked 23 hours ago
xx yy
205
205
Sounds very much like an X/Y problem, but doesarguments
orthis
count as a variable in your situation? :P (I'm assuming that defining a parameter would count as a variable) Or, can you explain what the problem is with using another variable?
– CertainPerformance
23 hours ago
4
JavaScript only has call/pass by value, never call/pass by reference. Either way, I don't see how these concepts are related to what you want to do. You cannot have the same variable refer to two different values at the same. You have to introduce another variable one way or the other. If you want to have it more "self-contained", use an IIFE:x = (x => () => x)(x);
(I guess technically this is an "IIAF" (immediately invoked arrow function)).
– Felix Kling
23 hours ago
You're trying to do some thing that isn't natural for JS. It's unclear what the purpose is but it's very likely that this is XY problem, as it was already mentioned.
– estus
21 hours ago
What's the use case for this, kindly?
– Sello Mkantjwa
20 hours ago
all values passed to the next function must be a function so I have to convert any non-function value to a function that returns the original value @estus
– xx yy
20 hours ago
|
show 2 more comments
Sounds very much like an X/Y problem, but doesarguments
orthis
count as a variable in your situation? :P (I'm assuming that defining a parameter would count as a variable) Or, can you explain what the problem is with using another variable?
– CertainPerformance
23 hours ago
4
JavaScript only has call/pass by value, never call/pass by reference. Either way, I don't see how these concepts are related to what you want to do. You cannot have the same variable refer to two different values at the same. You have to introduce another variable one way or the other. If you want to have it more "self-contained", use an IIFE:x = (x => () => x)(x);
(I guess technically this is an "IIAF" (immediately invoked arrow function)).
– Felix Kling
23 hours ago
You're trying to do some thing that isn't natural for JS. It's unclear what the purpose is but it's very likely that this is XY problem, as it was already mentioned.
– estus
21 hours ago
What's the use case for this, kindly?
– Sello Mkantjwa
20 hours ago
all values passed to the next function must be a function so I have to convert any non-function value to a function that returns the original value @estus
– xx yy
20 hours ago
Sounds very much like an X/Y problem, but does
arguments
or this
count as a variable in your situation? :P (I'm assuming that defining a parameter would count as a variable) Or, can you explain what the problem is with using another variable?– CertainPerformance
23 hours ago
Sounds very much like an X/Y problem, but does
arguments
or this
count as a variable in your situation? :P (I'm assuming that defining a parameter would count as a variable) Or, can you explain what the problem is with using another variable?– CertainPerformance
23 hours ago
4
4
JavaScript only has call/pass by value, never call/pass by reference. Either way, I don't see how these concepts are related to what you want to do. You cannot have the same variable refer to two different values at the same. You have to introduce another variable one way or the other. If you want to have it more "self-contained", use an IIFE:
x = (x => () => x)(x);
(I guess technically this is an "IIAF" (immediately invoked arrow function)).– Felix Kling
23 hours ago
JavaScript only has call/pass by value, never call/pass by reference. Either way, I don't see how these concepts are related to what you want to do. You cannot have the same variable refer to two different values at the same. You have to introduce another variable one way or the other. If you want to have it more "self-contained", use an IIFE:
x = (x => () => x)(x);
(I guess technically this is an "IIAF" (immediately invoked arrow function)).– Felix Kling
23 hours ago
You're trying to do some thing that isn't natural for JS. It's unclear what the purpose is but it's very likely that this is XY problem, as it was already mentioned.
– estus
21 hours ago
You're trying to do some thing that isn't natural for JS. It's unclear what the purpose is but it's very likely that this is XY problem, as it was already mentioned.
– estus
21 hours ago
What's the use case for this, kindly?
– Sello Mkantjwa
20 hours ago
What's the use case for this, kindly?
– Sello Mkantjwa
20 hours ago
all values passed to the next function must be a function so I have to convert any non-function value to a function that returns the original value @estus
– xx yy
20 hours ago
all values passed to the next function must be a function so I have to convert any non-function value to a function that returns the original value @estus
– xx yy
20 hours ago
|
show 2 more comments
1 Answer
1
active
oldest
votes
up vote
1
down vote
Just write a function:
function constFunction(temp) {
return () => temp;
}
so that you can call it as x = constFunction(x)
. Like every function call or assignment, this copies the value of the variable, not a reference. And no, you cannot really avoid a second variable (temp
in this case), as you can't have x
refer to both the function and the original value.
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
Just write a function:
function constFunction(temp) {
return () => temp;
}
so that you can call it as x = constFunction(x)
. Like every function call or assignment, this copies the value of the variable, not a reference. And no, you cannot really avoid a second variable (temp
in this case), as you can't have x
refer to both the function and the original value.
add a comment |
up vote
1
down vote
Just write a function:
function constFunction(temp) {
return () => temp;
}
so that you can call it as x = constFunction(x)
. Like every function call or assignment, this copies the value of the variable, not a reference. And no, you cannot really avoid a second variable (temp
in this case), as you can't have x
refer to both the function and the original value.
add a comment |
up vote
1
down vote
up vote
1
down vote
Just write a function:
function constFunction(temp) {
return () => temp;
}
so that you can call it as x = constFunction(x)
. Like every function call or assignment, this copies the value of the variable, not a reference. And no, you cannot really avoid a second variable (temp
in this case), as you can't have x
refer to both the function and the original value.
Just write a function:
function constFunction(temp) {
return () => temp;
}
so that you can call it as x = constFunction(x)
. Like every function call or assignment, this copies the value of the variable, not a reference. And no, you cannot really avoid a second variable (temp
in this case), as you can't have x
refer to both the function and the original value.
answered 20 hours ago
Bergi
355k55527845
355k55527845
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53236690%2fjavascript-how-to-convert-a-variable-to-a-function-with-the-same-name-that-retu%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
druTG6 g6Aq3um6k,Ce5onkrQ8oR4G,1WKcKqOnWkTrNV2uuE,z CcrdjEg03,a9IDKf0PK,iD82TJ,mYU
Sounds very much like an X/Y problem, but does
arguments
orthis
count as a variable in your situation? :P (I'm assuming that defining a parameter would count as a variable) Or, can you explain what the problem is with using another variable?– CertainPerformance
23 hours ago
4
JavaScript only has call/pass by value, never call/pass by reference. Either way, I don't see how these concepts are related to what you want to do. You cannot have the same variable refer to two different values at the same. You have to introduce another variable one way or the other. If you want to have it more "self-contained", use an IIFE:
x = (x => () => x)(x);
(I guess technically this is an "IIAF" (immediately invoked arrow function)).– Felix Kling
23 hours ago
You're trying to do some thing that isn't natural for JS. It's unclear what the purpose is but it's very likely that this is XY problem, as it was already mentioned.
– estus
21 hours ago
What's the use case for this, kindly?
– Sello Mkantjwa
20 hours ago
all values passed to the next function must be a function so I have to convert any non-function value to a function that returns the original value @estus
– xx yy
20 hours ago