Unexpected namespace behavior with closures
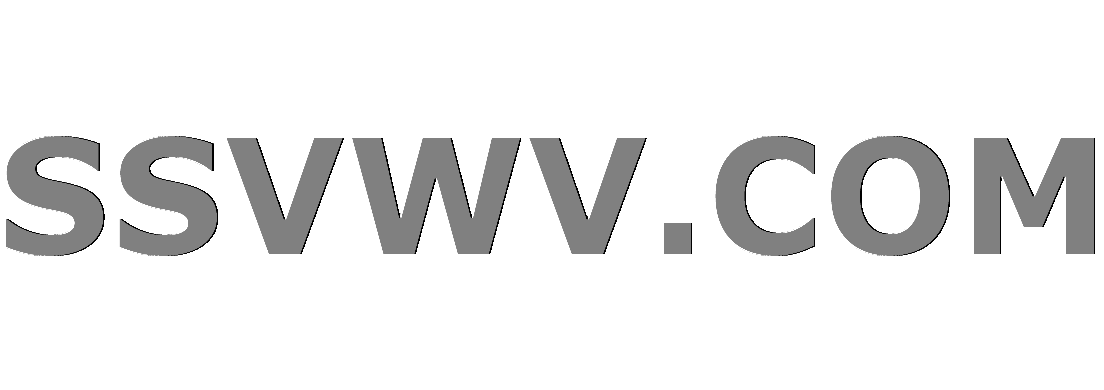
Multi tool use
up vote
1
down vote
favorite
Using PHP 7.0, consider the code below:
<?php
namespace A {
$closure = function() {
echo __NAMESPACE__;
};
}
namespace B {
$closure = function () {
echo __NAMESPACE__;
};
}
namespace C {
$closure();
}
To me, the expected output would be:
PHP Notice: Undefined variable: closure
But somehow the result is
B
Then consider this code below:
<?php
namespace A {
$closure = function() {
echo __NAMESPACE__;
};
}
namespace B {
$closure = function () {
echo __NAMESPACE__;
};
}
namespace C {
A$closure();
}
Now knowing (but not yet understanding) the behavior of the first example the expected output to me would be:
A
But instead I get
PHP Parse error: syntax error, unexpected '$closure' (T_VARIABLE), expecting identifier (T_STRING)
This behavior completely confuses me.
Question part 1: can someone explain me what is wrong with my expectation in the first example?
Question part 2: how is the behavior consistent with the first example?
php namespaces closures
New contributor
Hakariki is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
1
down vote
favorite
Using PHP 7.0, consider the code below:
<?php
namespace A {
$closure = function() {
echo __NAMESPACE__;
};
}
namespace B {
$closure = function () {
echo __NAMESPACE__;
};
}
namespace C {
$closure();
}
To me, the expected output would be:
PHP Notice: Undefined variable: closure
But somehow the result is
B
Then consider this code below:
<?php
namespace A {
$closure = function() {
echo __NAMESPACE__;
};
}
namespace B {
$closure = function () {
echo __NAMESPACE__;
};
}
namespace C {
A$closure();
}
Now knowing (but not yet understanding) the behavior of the first example the expected output to me would be:
A
But instead I get
PHP Parse error: syntax error, unexpected '$closure' (T_VARIABLE), expecting identifier (T_STRING)
This behavior completely confuses me.
Question part 1: can someone explain me what is wrong with my expectation in the first example?
Question part 2: how is the behavior consistent with the first example?
php namespaces closures
New contributor
Hakariki is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
2
In a namespace you can define classes, constants and functions. Variable is not class, not function, not constant.
– u_mulder
18 hours ago
For your last example, I guess (but I'm not sure) that the$closure
variable goes out of scope at the end of its namespace. But I don't know how you can get 'B' in your first example.
– AnthonyB
17 hours ago
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
Using PHP 7.0, consider the code below:
<?php
namespace A {
$closure = function() {
echo __NAMESPACE__;
};
}
namespace B {
$closure = function () {
echo __NAMESPACE__;
};
}
namespace C {
$closure();
}
To me, the expected output would be:
PHP Notice: Undefined variable: closure
But somehow the result is
B
Then consider this code below:
<?php
namespace A {
$closure = function() {
echo __NAMESPACE__;
};
}
namespace B {
$closure = function () {
echo __NAMESPACE__;
};
}
namespace C {
A$closure();
}
Now knowing (but not yet understanding) the behavior of the first example the expected output to me would be:
A
But instead I get
PHP Parse error: syntax error, unexpected '$closure' (T_VARIABLE), expecting identifier (T_STRING)
This behavior completely confuses me.
Question part 1: can someone explain me what is wrong with my expectation in the first example?
Question part 2: how is the behavior consistent with the first example?
php namespaces closures
New contributor
Hakariki is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Using PHP 7.0, consider the code below:
<?php
namespace A {
$closure = function() {
echo __NAMESPACE__;
};
}
namespace B {
$closure = function () {
echo __NAMESPACE__;
};
}
namespace C {
$closure();
}
To me, the expected output would be:
PHP Notice: Undefined variable: closure
But somehow the result is
B
Then consider this code below:
<?php
namespace A {
$closure = function() {
echo __NAMESPACE__;
};
}
namespace B {
$closure = function () {
echo __NAMESPACE__;
};
}
namespace C {
A$closure();
}
Now knowing (but not yet understanding) the behavior of the first example the expected output to me would be:
A
But instead I get
PHP Parse error: syntax error, unexpected '$closure' (T_VARIABLE), expecting identifier (T_STRING)
This behavior completely confuses me.
Question part 1: can someone explain me what is wrong with my expectation in the first example?
Question part 2: how is the behavior consistent with the first example?
php namespaces closures
php namespaces closures
New contributor
Hakariki is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Hakariki is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Hakariki is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked 18 hours ago
Hakariki
83
83
New contributor
Hakariki is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Hakariki is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Hakariki is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
2
In a namespace you can define classes, constants and functions. Variable is not class, not function, not constant.
– u_mulder
18 hours ago
For your last example, I guess (but I'm not sure) that the$closure
variable goes out of scope at the end of its namespace. But I don't know how you can get 'B' in your first example.
– AnthonyB
17 hours ago
add a comment |
2
In a namespace you can define classes, constants and functions. Variable is not class, not function, not constant.
– u_mulder
18 hours ago
For your last example, I guess (but I'm not sure) that the$closure
variable goes out of scope at the end of its namespace. But I don't know how you can get 'B' in your first example.
– AnthonyB
17 hours ago
2
2
In a namespace you can define classes, constants and functions. Variable is not class, not function, not constant.
– u_mulder
18 hours ago
In a namespace you can define classes, constants and functions. Variable is not class, not function, not constant.
– u_mulder
18 hours ago
For your last example, I guess (but I'm not sure) that the
$closure
variable goes out of scope at the end of its namespace. But I don't know how you can get 'B' in your first example.– AnthonyB
17 hours ago
For your last example, I guess (but I'm not sure) that the
$closure
variable goes out of scope at the end of its namespace. But I don't know how you can get 'B' in your first example.– AnthonyB
17 hours ago
add a comment |
1 Answer
1
active
oldest
votes
up vote
2
down vote
accepted
The behavior you observed should not confuse you.
It is what supposed to happen.
It is exactly how PHP namespace works.
PHP manual explains:
PHP Namespaces provide a way in which to group related classes, interfaces, functions and constants.
Not variables.
This means that $closure
in your code is the exact same variable in all the namespaces you defined (A, B and C).
It is first defined in namespace A.
Then the value is replaced in namespace B.
Then you call the closure it contains in namespace C.
The second example is the same.
Because namespaces are not for grouping variables, it should be obvious that A$closure()
is an invalid syntax.
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
The behavior you observed should not confuse you.
It is what supposed to happen.
It is exactly how PHP namespace works.
PHP manual explains:
PHP Namespaces provide a way in which to group related classes, interfaces, functions and constants.
Not variables.
This means that $closure
in your code is the exact same variable in all the namespaces you defined (A, B and C).
It is first defined in namespace A.
Then the value is replaced in namespace B.
Then you call the closure it contains in namespace C.
The second example is the same.
Because namespaces are not for grouping variables, it should be obvious that A$closure()
is an invalid syntax.
add a comment |
up vote
2
down vote
accepted
The behavior you observed should not confuse you.
It is what supposed to happen.
It is exactly how PHP namespace works.
PHP manual explains:
PHP Namespaces provide a way in which to group related classes, interfaces, functions and constants.
Not variables.
This means that $closure
in your code is the exact same variable in all the namespaces you defined (A, B and C).
It is first defined in namespace A.
Then the value is replaced in namespace B.
Then you call the closure it contains in namespace C.
The second example is the same.
Because namespaces are not for grouping variables, it should be obvious that A$closure()
is an invalid syntax.
add a comment |
up vote
2
down vote
accepted
up vote
2
down vote
accepted
The behavior you observed should not confuse you.
It is what supposed to happen.
It is exactly how PHP namespace works.
PHP manual explains:
PHP Namespaces provide a way in which to group related classes, interfaces, functions and constants.
Not variables.
This means that $closure
in your code is the exact same variable in all the namespaces you defined (A, B and C).
It is first defined in namespace A.
Then the value is replaced in namespace B.
Then you call the closure it contains in namespace C.
The second example is the same.
Because namespaces are not for grouping variables, it should be obvious that A$closure()
is an invalid syntax.
The behavior you observed should not confuse you.
It is what supposed to happen.
It is exactly how PHP namespace works.
PHP manual explains:
PHP Namespaces provide a way in which to group related classes, interfaces, functions and constants.
Not variables.
This means that $closure
in your code is the exact same variable in all the namespaces you defined (A, B and C).
It is first defined in namespace A.
Then the value is replaced in namespace B.
Then you call the closure it contains in namespace C.
The second example is the same.
Because namespaces are not for grouping variables, it should be obvious that A$closure()
is an invalid syntax.
answered 15 hours ago


Rei
5,411623
5,411623
add a comment |
add a comment |
Hakariki is a new contributor. Be nice, and check out our Code of Conduct.
Hakariki is a new contributor. Be nice, and check out our Code of Conduct.
Hakariki is a new contributor. Be nice, and check out our Code of Conduct.
Hakariki is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53237533%2funexpected-namespace-behavior-with-closures%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
q5aDe9G k6x9 cFVFBr2nrtD Jfrq,2o6e9bbQWlk3i8c,6ema,q1iqiX,AKIAbsxfY g7nEGzsa
2
In a namespace you can define classes, constants and functions. Variable is not class, not function, not constant.
– u_mulder
18 hours ago
For your last example, I guess (but I'm not sure) that the
$closure
variable goes out of scope at the end of its namespace. But I don't know how you can get 'B' in your first example.– AnthonyB
17 hours ago