Deleting records from Core Data
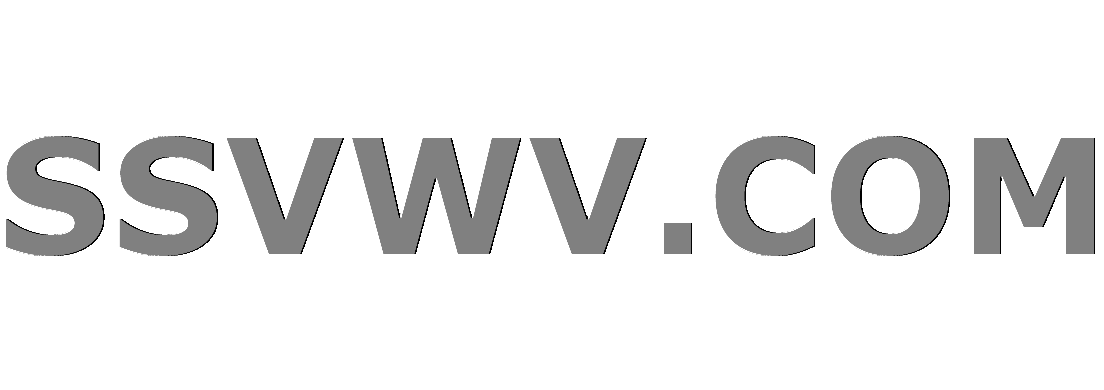
Multi tool use
up vote
0
down vote
favorite
I have 2 tables in my database: Car
and Garage
where Car
and Garage
are connected via foreign keys with each other:
In Car
, the foreign key is carHasGarages
. The whole schema looks like:
Destination: Garage
Inverse: `garageHasCar`
Delete rule: `Cascade`
Type: `To Many`
In Garage
the foreign key is garageHasCar
and the schema is:
Destination: Car
Inverse: `carHasGarage`
Delete rule: `Nullify`
Type: `To Many`
When I try to batch delete my garages
by using the predicate:
let predicate = NSPredicate(format: "carNbr IN %@", inactiveCars)
let request = NSFetchRequest<NSFetchRequestResult>(entityName: "Car")
request.predicate = predicate
let batchDelete = NSBatchDeleteRequest(fetchRequest: request)
do {
try context.execute(batchDelete)
}...
where inactiveCars
is the array of [Int32]
I get the next error:
error: Unhandled error from executeBatchDeleteRequest Constraint
violation: Batch delete failed due to mandatory MTM nullify inverse on Garage/garageHasCar and userInfo {
"_NSCoreDataOptimisticLockingFailureConflictsKey" = (
"<null>"
);
}
Can someone point to my mistake? I'm trying to solve this problem 2 days already and cannot manage it. So I thought maybe I do not see anything or miss something.
Thanks in advance for your time!
ios swift core-data
add a comment |
up vote
0
down vote
favorite
I have 2 tables in my database: Car
and Garage
where Car
and Garage
are connected via foreign keys with each other:
In Car
, the foreign key is carHasGarages
. The whole schema looks like:
Destination: Garage
Inverse: `garageHasCar`
Delete rule: `Cascade`
Type: `To Many`
In Garage
the foreign key is garageHasCar
and the schema is:
Destination: Car
Inverse: `carHasGarage`
Delete rule: `Nullify`
Type: `To Many`
When I try to batch delete my garages
by using the predicate:
let predicate = NSPredicate(format: "carNbr IN %@", inactiveCars)
let request = NSFetchRequest<NSFetchRequestResult>(entityName: "Car")
request.predicate = predicate
let batchDelete = NSBatchDeleteRequest(fetchRequest: request)
do {
try context.execute(batchDelete)
}...
where inactiveCars
is the array of [Int32]
I get the next error:
error: Unhandled error from executeBatchDeleteRequest Constraint
violation: Batch delete failed due to mandatory MTM nullify inverse on Garage/garageHasCar and userInfo {
"_NSCoreDataOptimisticLockingFailureConflictsKey" = (
"<null>"
);
}
Can someone point to my mistake? I'm trying to solve this problem 2 days already and cannot manage it. So I thought maybe I do not see anything or miss something.
Thanks in advance for your time!
ios swift core-data
2
Why is your delete ruleCascade
? Deleting a single car shouldn't delete the garage. Also, why is it "to many"? Surely a car can only be in at most one garage at a time? Your garage->car should be to-many as a garage can have more than one car. In both cases the delete rule should probably be "nullify"
– Paulw11
Nov 10 at 20:30
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have 2 tables in my database: Car
and Garage
where Car
and Garage
are connected via foreign keys with each other:
In Car
, the foreign key is carHasGarages
. The whole schema looks like:
Destination: Garage
Inverse: `garageHasCar`
Delete rule: `Cascade`
Type: `To Many`
In Garage
the foreign key is garageHasCar
and the schema is:
Destination: Car
Inverse: `carHasGarage`
Delete rule: `Nullify`
Type: `To Many`
When I try to batch delete my garages
by using the predicate:
let predicate = NSPredicate(format: "carNbr IN %@", inactiveCars)
let request = NSFetchRequest<NSFetchRequestResult>(entityName: "Car")
request.predicate = predicate
let batchDelete = NSBatchDeleteRequest(fetchRequest: request)
do {
try context.execute(batchDelete)
}...
where inactiveCars
is the array of [Int32]
I get the next error:
error: Unhandled error from executeBatchDeleteRequest Constraint
violation: Batch delete failed due to mandatory MTM nullify inverse on Garage/garageHasCar and userInfo {
"_NSCoreDataOptimisticLockingFailureConflictsKey" = (
"<null>"
);
}
Can someone point to my mistake? I'm trying to solve this problem 2 days already and cannot manage it. So I thought maybe I do not see anything or miss something.
Thanks in advance for your time!
ios swift core-data
I have 2 tables in my database: Car
and Garage
where Car
and Garage
are connected via foreign keys with each other:
In Car
, the foreign key is carHasGarages
. The whole schema looks like:
Destination: Garage
Inverse: `garageHasCar`
Delete rule: `Cascade`
Type: `To Many`
In Garage
the foreign key is garageHasCar
and the schema is:
Destination: Car
Inverse: `carHasGarage`
Delete rule: `Nullify`
Type: `To Many`
When I try to batch delete my garages
by using the predicate:
let predicate = NSPredicate(format: "carNbr IN %@", inactiveCars)
let request = NSFetchRequest<NSFetchRequestResult>(entityName: "Car")
request.predicate = predicate
let batchDelete = NSBatchDeleteRequest(fetchRequest: request)
do {
try context.execute(batchDelete)
}...
where inactiveCars
is the array of [Int32]
I get the next error:
error: Unhandled error from executeBatchDeleteRequest Constraint
violation: Batch delete failed due to mandatory MTM nullify inverse on Garage/garageHasCar and userInfo {
"_NSCoreDataOptimisticLockingFailureConflictsKey" = (
"<null>"
);
}
Can someone point to my mistake? I'm trying to solve this problem 2 days already and cannot manage it. So I thought maybe I do not see anything or miss something.
Thanks in advance for your time!
ios swift core-data
ios swift core-data
asked Nov 10 at 19:28
John Doe
195
195
2
Why is your delete ruleCascade
? Deleting a single car shouldn't delete the garage. Also, why is it "to many"? Surely a car can only be in at most one garage at a time? Your garage->car should be to-many as a garage can have more than one car. In both cases the delete rule should probably be "nullify"
– Paulw11
Nov 10 at 20:30
add a comment |
2
Why is your delete ruleCascade
? Deleting a single car shouldn't delete the garage. Also, why is it "to many"? Surely a car can only be in at most one garage at a time? Your garage->car should be to-many as a garage can have more than one car. In both cases the delete rule should probably be "nullify"
– Paulw11
Nov 10 at 20:30
2
2
Why is your delete rule
Cascade
? Deleting a single car shouldn't delete the garage. Also, why is it "to many"? Surely a car can only be in at most one garage at a time? Your garage->car should be to-many as a garage can have more than one car. In both cases the delete rule should probably be "nullify"– Paulw11
Nov 10 at 20:30
Why is your delete rule
Cascade
? Deleting a single car shouldn't delete the garage. Also, why is it "to many"? Surely a car can only be in at most one garage at a time? Your garage->car should be to-many as a garage can have more than one car. In both cases the delete rule should probably be "nullify"– Paulw11
Nov 10 at 20:30
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
From the documentation, this request is directly called on the backing store (most probably you are using sqlite here). Since the core data framework layer isn't much involved here, it looks like your deletion is leaving your sqlite in a bad state.
My guess on bad state: all inactive cars are deleted, but the garages still hold a reference to them since their relationship wasn't handled.
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
From the documentation, this request is directly called on the backing store (most probably you are using sqlite here). Since the core data framework layer isn't much involved here, it looks like your deletion is leaving your sqlite in a bad state.
My guess on bad state: all inactive cars are deleted, but the garages still hold a reference to them since their relationship wasn't handled.
add a comment |
up vote
1
down vote
From the documentation, this request is directly called on the backing store (most probably you are using sqlite here). Since the core data framework layer isn't much involved here, it looks like your deletion is leaving your sqlite in a bad state.
My guess on bad state: all inactive cars are deleted, but the garages still hold a reference to them since their relationship wasn't handled.
add a comment |
up vote
1
down vote
up vote
1
down vote
From the documentation, this request is directly called on the backing store (most probably you are using sqlite here). Since the core data framework layer isn't much involved here, it looks like your deletion is leaving your sqlite in a bad state.
My guess on bad state: all inactive cars are deleted, but the garages still hold a reference to them since their relationship wasn't handled.
From the documentation, this request is directly called on the backing store (most probably you are using sqlite here). Since the core data framework layer isn't much involved here, it looks like your deletion is leaving your sqlite in a bad state.
My guess on bad state: all inactive cars are deleted, but the garages still hold a reference to them since their relationship wasn't handled.
answered Nov 10 at 20:18
Ayush Goel
2,5502334
2,5502334
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53242648%2fdeleting-records-from-core-data%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
WuEFEmof PKtzTc4oIxb6Gon5tJETOWDc9M4OG8GoaY tWIlB27AYCi3B6x3nRu,C1EW6BbAwQsyrcnX TEX
2
Why is your delete rule
Cascade
? Deleting a single car shouldn't delete the garage. Also, why is it "to many"? Surely a car can only be in at most one garage at a time? Your garage->car should be to-many as a garage can have more than one car. In both cases the delete rule should probably be "nullify"– Paulw11
Nov 10 at 20:30