Count specific digits and replace even digits with 0 using recursion
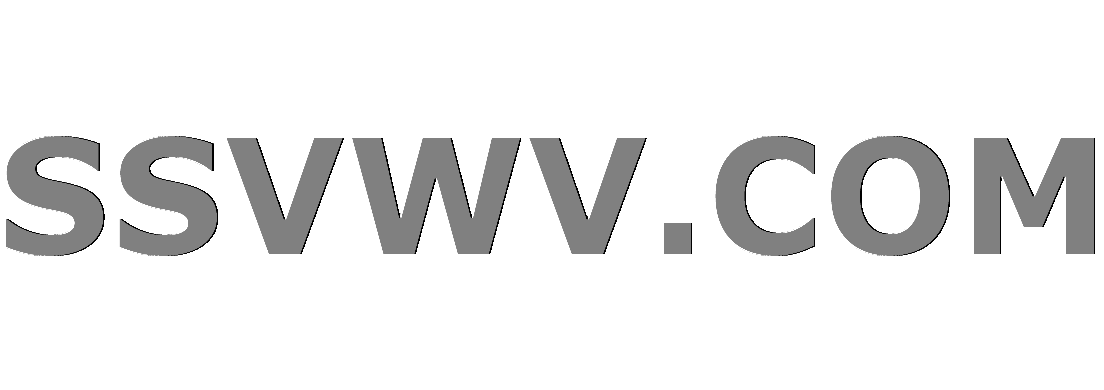
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I am new to C++, this is my first hard assignment and I am having trouble understanding recursion. I need to program a recursive function to count how many times a user enters 5 and 8. As well as replace even digits with 0. Example:
User enter: 4585
Count of 5 & 8: 3
Replace even with 0: 0505
The code below is completely wrong but this is the language I need to use. And take note how it must include recursion. And I can call upon the function later on.
#include <iostream>
using namespace std;
int countThreeFives(int num) {
static int count=0;
int digit = num % 10;
if (n == 5 || n == 8)
{
count++;
countThreeFives(num/10);
}
else
{
return count;
}
}
int replaceEven(int num){
int digit = num % 10;
if (digit % 2 != 0) {
// Confused here (incomplete)
}
int main() {
int x;
cout << "Enter a positive number: ";
cin >> x;
cout << "The count of 8 & 5 is: " << countThreeFives(x);
cout << "Replacing even with 0: " << replaceEven(x);
}
c++ recursion
|
show 2 more comments
I am new to C++, this is my first hard assignment and I am having trouble understanding recursion. I need to program a recursive function to count how many times a user enters 5 and 8. As well as replace even digits with 0. Example:
User enter: 4585
Count of 5 & 8: 3
Replace even with 0: 0505
The code below is completely wrong but this is the language I need to use. And take note how it must include recursion. And I can call upon the function later on.
#include <iostream>
using namespace std;
int countThreeFives(int num) {
static int count=0;
int digit = num % 10;
if (n == 5 || n == 8)
{
count++;
countThreeFives(num/10);
}
else
{
return count;
}
}
int replaceEven(int num){
int digit = num % 10;
if (digit % 2 != 0) {
// Confused here (incomplete)
}
int main() {
int x;
cout << "Enter a positive number: ";
cin >> x;
cout << "The count of 8 & 5 is: " << countThreeFives(x);
cout << "Replacing even with 0: " << replaceEven(x);
}
c++ recursion
Please give a Minimal, complete, and verifiable piece of code: stackoverflow.com/help/mcve
– Little Boy Blue
Nov 16 '18 at 19:05
@LittleBoyBlue there are a bunch of shortcuts you can use for commonly used messages. For example,[mcve]
expands to Minimal, Complete, and Verifiable example.
– user4581301
Nov 16 '18 at 19:43
Sharan, you will find your questions will almost always get a better reception when accompanied by a credible attempt at solving the problem. Often these attempts are close enough to correct that they can be fixed with a few simple alterations and if not the code provides a baseline of your understanding of the problem so that answers can be better tailored. On the social front, they show that you aren't simply expecting people to do your homework for you.
– user4581301
Nov 16 '18 at 19:47
I wasn't expecting that no one to do my homework, but I was totally confused trying to understand recursive.
– Sharan Banik
Nov 16 '18 at 19:53
The Master once said that to understand recursion first you must understand recursion. Since this really isn't that helpful, the Master was a bit of a dick, the best thing to do is to start with really simple stuff like "print out a string one character at a time with recursion." Understand complicated recursion by first understanding simple recursion.
– user4581301
Nov 17 '18 at 1:34
|
show 2 more comments
I am new to C++, this is my first hard assignment and I am having trouble understanding recursion. I need to program a recursive function to count how many times a user enters 5 and 8. As well as replace even digits with 0. Example:
User enter: 4585
Count of 5 & 8: 3
Replace even with 0: 0505
The code below is completely wrong but this is the language I need to use. And take note how it must include recursion. And I can call upon the function later on.
#include <iostream>
using namespace std;
int countThreeFives(int num) {
static int count=0;
int digit = num % 10;
if (n == 5 || n == 8)
{
count++;
countThreeFives(num/10);
}
else
{
return count;
}
}
int replaceEven(int num){
int digit = num % 10;
if (digit % 2 != 0) {
// Confused here (incomplete)
}
int main() {
int x;
cout << "Enter a positive number: ";
cin >> x;
cout << "The count of 8 & 5 is: " << countThreeFives(x);
cout << "Replacing even with 0: " << replaceEven(x);
}
c++ recursion
I am new to C++, this is my first hard assignment and I am having trouble understanding recursion. I need to program a recursive function to count how many times a user enters 5 and 8. As well as replace even digits with 0. Example:
User enter: 4585
Count of 5 & 8: 3
Replace even with 0: 0505
The code below is completely wrong but this is the language I need to use. And take note how it must include recursion. And I can call upon the function later on.
#include <iostream>
using namespace std;
int countThreeFives(int num) {
static int count=0;
int digit = num % 10;
if (n == 5 || n == 8)
{
count++;
countThreeFives(num/10);
}
else
{
return count;
}
}
int replaceEven(int num){
int digit = num % 10;
if (digit % 2 != 0) {
// Confused here (incomplete)
}
int main() {
int x;
cout << "Enter a positive number: ";
cin >> x;
cout << "The count of 8 & 5 is: " << countThreeFives(x);
cout << "Replacing even with 0: " << replaceEven(x);
}
c++ recursion
c++ recursion
edited Nov 17 '18 at 1:37
cdlane
20k21245
20k21245
asked Nov 16 '18 at 19:02


Sharan BanikSharan Banik
111
111
Please give a Minimal, complete, and verifiable piece of code: stackoverflow.com/help/mcve
– Little Boy Blue
Nov 16 '18 at 19:05
@LittleBoyBlue there are a bunch of shortcuts you can use for commonly used messages. For example,[mcve]
expands to Minimal, Complete, and Verifiable example.
– user4581301
Nov 16 '18 at 19:43
Sharan, you will find your questions will almost always get a better reception when accompanied by a credible attempt at solving the problem. Often these attempts are close enough to correct that they can be fixed with a few simple alterations and if not the code provides a baseline of your understanding of the problem so that answers can be better tailored. On the social front, they show that you aren't simply expecting people to do your homework for you.
– user4581301
Nov 16 '18 at 19:47
I wasn't expecting that no one to do my homework, but I was totally confused trying to understand recursive.
– Sharan Banik
Nov 16 '18 at 19:53
The Master once said that to understand recursion first you must understand recursion. Since this really isn't that helpful, the Master was a bit of a dick, the best thing to do is to start with really simple stuff like "print out a string one character at a time with recursion." Understand complicated recursion by first understanding simple recursion.
– user4581301
Nov 17 '18 at 1:34
|
show 2 more comments
Please give a Minimal, complete, and verifiable piece of code: stackoverflow.com/help/mcve
– Little Boy Blue
Nov 16 '18 at 19:05
@LittleBoyBlue there are a bunch of shortcuts you can use for commonly used messages. For example,[mcve]
expands to Minimal, Complete, and Verifiable example.
– user4581301
Nov 16 '18 at 19:43
Sharan, you will find your questions will almost always get a better reception when accompanied by a credible attempt at solving the problem. Often these attempts are close enough to correct that they can be fixed with a few simple alterations and if not the code provides a baseline of your understanding of the problem so that answers can be better tailored. On the social front, they show that you aren't simply expecting people to do your homework for you.
– user4581301
Nov 16 '18 at 19:47
I wasn't expecting that no one to do my homework, but I was totally confused trying to understand recursive.
– Sharan Banik
Nov 16 '18 at 19:53
The Master once said that to understand recursion first you must understand recursion. Since this really isn't that helpful, the Master was a bit of a dick, the best thing to do is to start with really simple stuff like "print out a string one character at a time with recursion." Understand complicated recursion by first understanding simple recursion.
– user4581301
Nov 17 '18 at 1:34
Please give a Minimal, complete, and verifiable piece of code: stackoverflow.com/help/mcve
– Little Boy Blue
Nov 16 '18 at 19:05
Please give a Minimal, complete, and verifiable piece of code: stackoverflow.com/help/mcve
– Little Boy Blue
Nov 16 '18 at 19:05
@LittleBoyBlue there are a bunch of shortcuts you can use for commonly used messages. For example,
[mcve]
expands to Minimal, Complete, and Verifiable example.– user4581301
Nov 16 '18 at 19:43
@LittleBoyBlue there are a bunch of shortcuts you can use for commonly used messages. For example,
[mcve]
expands to Minimal, Complete, and Verifiable example.– user4581301
Nov 16 '18 at 19:43
Sharan, you will find your questions will almost always get a better reception when accompanied by a credible attempt at solving the problem. Often these attempts are close enough to correct that they can be fixed with a few simple alterations and if not the code provides a baseline of your understanding of the problem so that answers can be better tailored. On the social front, they show that you aren't simply expecting people to do your homework for you.
– user4581301
Nov 16 '18 at 19:47
Sharan, you will find your questions will almost always get a better reception when accompanied by a credible attempt at solving the problem. Often these attempts are close enough to correct that they can be fixed with a few simple alterations and if not the code provides a baseline of your understanding of the problem so that answers can be better tailored. On the social front, they show that you aren't simply expecting people to do your homework for you.
– user4581301
Nov 16 '18 at 19:47
I wasn't expecting that no one to do my homework, but I was totally confused trying to understand recursive.
– Sharan Banik
Nov 16 '18 at 19:53
I wasn't expecting that no one to do my homework, but I was totally confused trying to understand recursive.
– Sharan Banik
Nov 16 '18 at 19:53
The Master once said that to understand recursion first you must understand recursion. Since this really isn't that helpful, the Master was a bit of a dick, the best thing to do is to start with really simple stuff like "print out a string one character at a time with recursion." Understand complicated recursion by first understanding simple recursion.
– user4581301
Nov 17 '18 at 1:34
The Master once said that to understand recursion first you must understand recursion. Since this really isn't that helpful, the Master was a bit of a dick, the best thing to do is to start with really simple stuff like "print out a string one character at a time with recursion." Understand complicated recursion by first understanding simple recursion.
– user4581301
Nov 17 '18 at 1:34
|
show 2 more comments
5 Answers
5
active
oldest
votes
I'm guessing this is a school assignment hence the requirement to use recursion here. Anyways, here is a recursive solution:
Let us denote by f(d, n) the number of 5s and 8s in the first n digits of a string of digits d. Then we can form the following recursive relation:
f(d, n) = 1 + f(d, n - 1) if the nth digit is either 5 or 8
f(d, n) = f(d, n - 1) if the nth digit is neither a 5 nor an 8
and our base case is f(d, 0) = 0, since a string of size 0 will have no 5s and no 8s
#include <iostream>
#include <string>
int countAndReplace(std::string& digits, int n)
{
if(n == 0)
{
return 0;
}
bool addOne = (digits[n - 1] == '5' || digits[n - 1] == '8');
if(digits[n - 1] % 2 == 0)
{
digits[n - 1] = '0';
}
return addOne + countAndReplace(digits, n - 1);
}
int main()
{
std::string digits;
std::cin >> digits;
std::cout << countAndReplace(digits, digits.size()) << 'n';
std::cout << digits << 'n';
return 0;
}
First we need to read the digits from standard input for which it is best to use a std::string since we don't know the number of digits in advance. Then we call our recursive function that takes two arguments - a reference to the string of digits (used for changing the digits in place, for the second part of the task), and the length of said string. In the function, for each digit we also check if it needs to be replaced according to the rules you posted, and if it does we do the replacement in place, hence why a reference to the string is used as an argument.
This is a very easy problem. Please, next time, try solving your assignments by yourself before asking for help. Otherwise you will have trouble progressing.
add a comment |
You may simply use std::unordered_map to keep track of the number of unique integers. Here's an example implementation:
#include <iostream>
#include <unordered_map>
int main() {
std::unordered_map<int, int> numbs;
int numb;
while (std::cin >> numb) {
if (numb % 2 == 0) {
++numbs[0];
}
else {
++numbs[numb];
}
}
for (const auto p : numbs) {
std::cout << p.first << " was seen " << p.second << " time(s) " << std::endl;
}
return 0;
}
Input:
1 2 3 4 6 5 7 4 2 5 7 5
Output:
5 was seen 3 time(s)
3 was seen 1 time(s)
1 was seen 1 time(s)
7 was seen 2 time(s)
0 was seen 5 time(s)
Note the ++numbs[0]
part. As you can see, int this example we don't check if a key exists in the numbs
, we simply increase it by one. That is because when we use [numb]
, an object with that key is created if it does't already exist, and we may simply increment it by one, since int
is default initialized to 0
.
Sorry, but I haven't learned most of the terms which you have used.
– Sharan Banik
Nov 16 '18 at 19:21
@SharanBanik Which parts do you need being explained more thoroughly?
– Ayxan
Nov 16 '18 at 19:22
If possible can you code with beginner C++ code and include a recursive, I will provide what I completed so far.
– Sharan Banik
Nov 16 '18 at 19:25
Do you have to make it recursive? It is much cleaner this way?
– Ayxan
Nov 16 '18 at 19:27
I have added a code, maybe you could guide me from there, very beginner language
– Sharan Banik
Nov 16 '18 at 19:54
add a comment |
This program will do what you need, but you really don't need any recursivness. Recursive functions, in case you don't know, is a function that calls itself. Computing factorials, for example, would use a recursive function:
#include <iostream>
#include <string>
int main()
{
std::string input;
std::cout << "User Enter: ";
std::cin >> input;
int num8 = 0;
int num5 = 0;
for (std::string::size_type i = 0; i < input.size(); ++i)
{
if (input[i] == '8')
{
num8++;
}
if (input[i] == '5')
{
num5++;
}
if (input[i] == '2' || input[i] == '4' || input[i] == '6' || input[i] == '8')
{
input[i] = '0';
}
}
std::cout << "Count of 5 & 8: " << num5 + num8 << std::endl;
std::cout << "Replace Even : " << input << std::endl;
}
What this does and is grab input from the user, then loops through each character in the string. If it comes to an 8
or 5
, it increments a counter. At the same time, it checks the character to see if it's an even number (2
, 4
, 6
, or 8
) and if so, it replaces it with a 0
. Finally, it prints the output.
add a comment |
Here I suggest using std::string
class with its many functions for operating on character sequences, much easier than writing your own algorithms.
The key to recursion is 3 part:
- Define a base case that handles no matching characters
- Define a recursive case that handles the first occurrence of a matching character
- Define a recursive case that continues to search the remainder of the string after part 2 is met.
Works for any length input.
#include <iostream>
#include <string>
int numChars(char, std::string, int);
std::string replaceEvenWithZero(std::string);
int main()
{
std::string str;
std::cout << "User Enter: ";
std::cin >> str;
// Display the number of times the '5' or '8' appear in the string.
std::cout << "Count of 5 & 8: " << numChars('5', str, 0) + numChars('8', str, 0) << " times.n";
std::cout << "Replace even with 0: " << replaceEvenWithZero(str) << std::endl;
return 0;
}
int numChars(char search, std::string str, int subscript)
{
if (subscript >= str.length())
{
// Base case: end of the string was reached.
return 0;
}
else if (str[subscript] == search)
{
// Recursive case: A matching character was found. Return
// 1 plus the number of times the search character appears
// in the string.
return 1 + numChars(search, str, subscript + 1);
}
else
{
// Recursive case: A character that does not match the search
// character was found. Return the number of times the search
// character appears in the rest of the string.
return numChars(search, str, subscript + 1);
}
}
std::string replaceEvenWithZero(std::string str)
{
// check the index (position) of each character in the string
for (int i=0; i < str.length(); i++){
// if the index even number position
// (divides by 2 with no remainder, then true)
// replace character with `0` at this index
if (i % 2 != 0){
str = str.substr(0,i-1) + "0" + str.substr(i, str.length());
}
}
return str; // return our newly created string with `0`s
}
Demo:
User Enter: 4585
Count of 5 & 8: 3 times.
Replace even with 0: 0505
User Enter: 6856756453
Count of 5 & 8: 4 times.
Replace even with 0: 0806050403
add a comment |
Other folks responded to your question with solutions that use different signatures than your code:
int countThreeFives(int num);
int replaceEven(int num);
and/or simply tossed the recursion requirement. Also, you specified int
yet asked your user for a positive number -- if your code really only works on positive numbers, you should consider unsigned int
instead of int
. Or just deal with negative numbers. Addressing all this, and keeping the spirit of your original code:
#include <iostream>
using namespace std;
int countThreeFives(int number) {
if (number != 0) {
int count = 0, digit = abs(number % 10);
if (digit == 5 || digit == 8) {
count++;
}
return count + countThreeFives(number / 10);
}
return 0;
}
int replaceEven(int number) {
if (number != 0) {
int digit = (number % 10) * (number % 2);
return ((number > 0) ? 1 : -1) * (replaceEven(abs(number) / 10) * 10 + digit);
}
return number;
}
int main() {
int number;
cout << "Enter a number: ";
cin >> number;
cout << "The count of 8 & 5 is: " << countThreeFives(number) << endl;
cout << "Replacing even with 0: " << replaceEven(number) << endl;
}
Example usage showing negative number support:
> ./a.out
Enter a number: -382135
The count of 8 & 5 is: 2
Replacing even with 0: -300135
>
However, there are a couple of other issues with your two function taking int
as arguments and returning an int
. E.g., your example:
User enter: 4585
Count of 5 & 8: 3
Replace even with 0: 0505
is wrong as using integers the replaced number would be:
> ./a.out
Enter a number: 4585
The count of 8 & 5 is: 3
Replacing even with 0: 505
Mac-mini>
I.e. no leading zeros. You only get a leading zero with a non-numeric approach. The other issue with using integers is that the length of the number input is limited. A typically maximum length input would be:
> ./a.out
Enter a number: 356756453
The count of 8 & 5 is: 3
Replacing even with 0: 350750053
>
Any longer, and you'd need to use a larger integer type (e.g. unsigned long long
) or again take a non-numeric approach.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53343912%2fcount-specific-digits-and-replace-even-digits-with-0-using-recursion%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
5 Answers
5
active
oldest
votes
5 Answers
5
active
oldest
votes
active
oldest
votes
active
oldest
votes
I'm guessing this is a school assignment hence the requirement to use recursion here. Anyways, here is a recursive solution:
Let us denote by f(d, n) the number of 5s and 8s in the first n digits of a string of digits d. Then we can form the following recursive relation:
f(d, n) = 1 + f(d, n - 1) if the nth digit is either 5 or 8
f(d, n) = f(d, n - 1) if the nth digit is neither a 5 nor an 8
and our base case is f(d, 0) = 0, since a string of size 0 will have no 5s and no 8s
#include <iostream>
#include <string>
int countAndReplace(std::string& digits, int n)
{
if(n == 0)
{
return 0;
}
bool addOne = (digits[n - 1] == '5' || digits[n - 1] == '8');
if(digits[n - 1] % 2 == 0)
{
digits[n - 1] = '0';
}
return addOne + countAndReplace(digits, n - 1);
}
int main()
{
std::string digits;
std::cin >> digits;
std::cout << countAndReplace(digits, digits.size()) << 'n';
std::cout << digits << 'n';
return 0;
}
First we need to read the digits from standard input for which it is best to use a std::string since we don't know the number of digits in advance. Then we call our recursive function that takes two arguments - a reference to the string of digits (used for changing the digits in place, for the second part of the task), and the length of said string. In the function, for each digit we also check if it needs to be replaced according to the rules you posted, and if it does we do the replacement in place, hence why a reference to the string is used as an argument.
This is a very easy problem. Please, next time, try solving your assignments by yourself before asking for help. Otherwise you will have trouble progressing.
add a comment |
I'm guessing this is a school assignment hence the requirement to use recursion here. Anyways, here is a recursive solution:
Let us denote by f(d, n) the number of 5s and 8s in the first n digits of a string of digits d. Then we can form the following recursive relation:
f(d, n) = 1 + f(d, n - 1) if the nth digit is either 5 or 8
f(d, n) = f(d, n - 1) if the nth digit is neither a 5 nor an 8
and our base case is f(d, 0) = 0, since a string of size 0 will have no 5s and no 8s
#include <iostream>
#include <string>
int countAndReplace(std::string& digits, int n)
{
if(n == 0)
{
return 0;
}
bool addOne = (digits[n - 1] == '5' || digits[n - 1] == '8');
if(digits[n - 1] % 2 == 0)
{
digits[n - 1] = '0';
}
return addOne + countAndReplace(digits, n - 1);
}
int main()
{
std::string digits;
std::cin >> digits;
std::cout << countAndReplace(digits, digits.size()) << 'n';
std::cout << digits << 'n';
return 0;
}
First we need to read the digits from standard input for which it is best to use a std::string since we don't know the number of digits in advance. Then we call our recursive function that takes two arguments - a reference to the string of digits (used for changing the digits in place, for the second part of the task), and the length of said string. In the function, for each digit we also check if it needs to be replaced according to the rules you posted, and if it does we do the replacement in place, hence why a reference to the string is used as an argument.
This is a very easy problem. Please, next time, try solving your assignments by yourself before asking for help. Otherwise you will have trouble progressing.
add a comment |
I'm guessing this is a school assignment hence the requirement to use recursion here. Anyways, here is a recursive solution:
Let us denote by f(d, n) the number of 5s and 8s in the first n digits of a string of digits d. Then we can form the following recursive relation:
f(d, n) = 1 + f(d, n - 1) if the nth digit is either 5 or 8
f(d, n) = f(d, n - 1) if the nth digit is neither a 5 nor an 8
and our base case is f(d, 0) = 0, since a string of size 0 will have no 5s and no 8s
#include <iostream>
#include <string>
int countAndReplace(std::string& digits, int n)
{
if(n == 0)
{
return 0;
}
bool addOne = (digits[n - 1] == '5' || digits[n - 1] == '8');
if(digits[n - 1] % 2 == 0)
{
digits[n - 1] = '0';
}
return addOne + countAndReplace(digits, n - 1);
}
int main()
{
std::string digits;
std::cin >> digits;
std::cout << countAndReplace(digits, digits.size()) << 'n';
std::cout << digits << 'n';
return 0;
}
First we need to read the digits from standard input for which it is best to use a std::string since we don't know the number of digits in advance. Then we call our recursive function that takes two arguments - a reference to the string of digits (used for changing the digits in place, for the second part of the task), and the length of said string. In the function, for each digit we also check if it needs to be replaced according to the rules you posted, and if it does we do the replacement in place, hence why a reference to the string is used as an argument.
This is a very easy problem. Please, next time, try solving your assignments by yourself before asking for help. Otherwise you will have trouble progressing.
I'm guessing this is a school assignment hence the requirement to use recursion here. Anyways, here is a recursive solution:
Let us denote by f(d, n) the number of 5s and 8s in the first n digits of a string of digits d. Then we can form the following recursive relation:
f(d, n) = 1 + f(d, n - 1) if the nth digit is either 5 or 8
f(d, n) = f(d, n - 1) if the nth digit is neither a 5 nor an 8
and our base case is f(d, 0) = 0, since a string of size 0 will have no 5s and no 8s
#include <iostream>
#include <string>
int countAndReplace(std::string& digits, int n)
{
if(n == 0)
{
return 0;
}
bool addOne = (digits[n - 1] == '5' || digits[n - 1] == '8');
if(digits[n - 1] % 2 == 0)
{
digits[n - 1] = '0';
}
return addOne + countAndReplace(digits, n - 1);
}
int main()
{
std::string digits;
std::cin >> digits;
std::cout << countAndReplace(digits, digits.size()) << 'n';
std::cout << digits << 'n';
return 0;
}
First we need to read the digits from standard input for which it is best to use a std::string since we don't know the number of digits in advance. Then we call our recursive function that takes two arguments - a reference to the string of digits (used for changing the digits in place, for the second part of the task), and the length of said string. In the function, for each digit we also check if it needs to be replaced according to the rules you posted, and if it does we do the replacement in place, hence why a reference to the string is used as an argument.
This is a very easy problem. Please, next time, try solving your assignments by yourself before asking for help. Otherwise you will have trouble progressing.
edited Nov 16 '18 at 20:19
answered Nov 16 '18 at 19:31
Hristijan GjorshevskiHristijan Gjorshevski
115117
115117
add a comment |
add a comment |
You may simply use std::unordered_map to keep track of the number of unique integers. Here's an example implementation:
#include <iostream>
#include <unordered_map>
int main() {
std::unordered_map<int, int> numbs;
int numb;
while (std::cin >> numb) {
if (numb % 2 == 0) {
++numbs[0];
}
else {
++numbs[numb];
}
}
for (const auto p : numbs) {
std::cout << p.first << " was seen " << p.second << " time(s) " << std::endl;
}
return 0;
}
Input:
1 2 3 4 6 5 7 4 2 5 7 5
Output:
5 was seen 3 time(s)
3 was seen 1 time(s)
1 was seen 1 time(s)
7 was seen 2 time(s)
0 was seen 5 time(s)
Note the ++numbs[0]
part. As you can see, int this example we don't check if a key exists in the numbs
, we simply increase it by one. That is because when we use [numb]
, an object with that key is created if it does't already exist, and we may simply increment it by one, since int
is default initialized to 0
.
Sorry, but I haven't learned most of the terms which you have used.
– Sharan Banik
Nov 16 '18 at 19:21
@SharanBanik Which parts do you need being explained more thoroughly?
– Ayxan
Nov 16 '18 at 19:22
If possible can you code with beginner C++ code and include a recursive, I will provide what I completed so far.
– Sharan Banik
Nov 16 '18 at 19:25
Do you have to make it recursive? It is much cleaner this way?
– Ayxan
Nov 16 '18 at 19:27
I have added a code, maybe you could guide me from there, very beginner language
– Sharan Banik
Nov 16 '18 at 19:54
add a comment |
You may simply use std::unordered_map to keep track of the number of unique integers. Here's an example implementation:
#include <iostream>
#include <unordered_map>
int main() {
std::unordered_map<int, int> numbs;
int numb;
while (std::cin >> numb) {
if (numb % 2 == 0) {
++numbs[0];
}
else {
++numbs[numb];
}
}
for (const auto p : numbs) {
std::cout << p.first << " was seen " << p.second << " time(s) " << std::endl;
}
return 0;
}
Input:
1 2 3 4 6 5 7 4 2 5 7 5
Output:
5 was seen 3 time(s)
3 was seen 1 time(s)
1 was seen 1 time(s)
7 was seen 2 time(s)
0 was seen 5 time(s)
Note the ++numbs[0]
part. As you can see, int this example we don't check if a key exists in the numbs
, we simply increase it by one. That is because when we use [numb]
, an object with that key is created if it does't already exist, and we may simply increment it by one, since int
is default initialized to 0
.
Sorry, but I haven't learned most of the terms which you have used.
– Sharan Banik
Nov 16 '18 at 19:21
@SharanBanik Which parts do you need being explained more thoroughly?
– Ayxan
Nov 16 '18 at 19:22
If possible can you code with beginner C++ code and include a recursive, I will provide what I completed so far.
– Sharan Banik
Nov 16 '18 at 19:25
Do you have to make it recursive? It is much cleaner this way?
– Ayxan
Nov 16 '18 at 19:27
I have added a code, maybe you could guide me from there, very beginner language
– Sharan Banik
Nov 16 '18 at 19:54
add a comment |
You may simply use std::unordered_map to keep track of the number of unique integers. Here's an example implementation:
#include <iostream>
#include <unordered_map>
int main() {
std::unordered_map<int, int> numbs;
int numb;
while (std::cin >> numb) {
if (numb % 2 == 0) {
++numbs[0];
}
else {
++numbs[numb];
}
}
for (const auto p : numbs) {
std::cout << p.first << " was seen " << p.second << " time(s) " << std::endl;
}
return 0;
}
Input:
1 2 3 4 6 5 7 4 2 5 7 5
Output:
5 was seen 3 time(s)
3 was seen 1 time(s)
1 was seen 1 time(s)
7 was seen 2 time(s)
0 was seen 5 time(s)
Note the ++numbs[0]
part. As you can see, int this example we don't check if a key exists in the numbs
, we simply increase it by one. That is because when we use [numb]
, an object with that key is created if it does't already exist, and we may simply increment it by one, since int
is default initialized to 0
.
You may simply use std::unordered_map to keep track of the number of unique integers. Here's an example implementation:
#include <iostream>
#include <unordered_map>
int main() {
std::unordered_map<int, int> numbs;
int numb;
while (std::cin >> numb) {
if (numb % 2 == 0) {
++numbs[0];
}
else {
++numbs[numb];
}
}
for (const auto p : numbs) {
std::cout << p.first << " was seen " << p.second << " time(s) " << std::endl;
}
return 0;
}
Input:
1 2 3 4 6 5 7 4 2 5 7 5
Output:
5 was seen 3 time(s)
3 was seen 1 time(s)
1 was seen 1 time(s)
7 was seen 2 time(s)
0 was seen 5 time(s)
Note the ++numbs[0]
part. As you can see, int this example we don't check if a key exists in the numbs
, we simply increase it by one. That is because when we use [numb]
, an object with that key is created if it does't already exist, and we may simply increment it by one, since int
is default initialized to 0
.
edited Nov 16 '18 at 20:42
answered Nov 16 '18 at 19:11


AyxanAyxan
2,347622
2,347622
Sorry, but I haven't learned most of the terms which you have used.
– Sharan Banik
Nov 16 '18 at 19:21
@SharanBanik Which parts do you need being explained more thoroughly?
– Ayxan
Nov 16 '18 at 19:22
If possible can you code with beginner C++ code and include a recursive, I will provide what I completed so far.
– Sharan Banik
Nov 16 '18 at 19:25
Do you have to make it recursive? It is much cleaner this way?
– Ayxan
Nov 16 '18 at 19:27
I have added a code, maybe you could guide me from there, very beginner language
– Sharan Banik
Nov 16 '18 at 19:54
add a comment |
Sorry, but I haven't learned most of the terms which you have used.
– Sharan Banik
Nov 16 '18 at 19:21
@SharanBanik Which parts do you need being explained more thoroughly?
– Ayxan
Nov 16 '18 at 19:22
If possible can you code with beginner C++ code and include a recursive, I will provide what I completed so far.
– Sharan Banik
Nov 16 '18 at 19:25
Do you have to make it recursive? It is much cleaner this way?
– Ayxan
Nov 16 '18 at 19:27
I have added a code, maybe you could guide me from there, very beginner language
– Sharan Banik
Nov 16 '18 at 19:54
Sorry, but I haven't learned most of the terms which you have used.
– Sharan Banik
Nov 16 '18 at 19:21
Sorry, but I haven't learned most of the terms which you have used.
– Sharan Banik
Nov 16 '18 at 19:21
@SharanBanik Which parts do you need being explained more thoroughly?
– Ayxan
Nov 16 '18 at 19:22
@SharanBanik Which parts do you need being explained more thoroughly?
– Ayxan
Nov 16 '18 at 19:22
If possible can you code with beginner C++ code and include a recursive, I will provide what I completed so far.
– Sharan Banik
Nov 16 '18 at 19:25
If possible can you code with beginner C++ code and include a recursive, I will provide what I completed so far.
– Sharan Banik
Nov 16 '18 at 19:25
Do you have to make it recursive? It is much cleaner this way?
– Ayxan
Nov 16 '18 at 19:27
Do you have to make it recursive? It is much cleaner this way?
– Ayxan
Nov 16 '18 at 19:27
I have added a code, maybe you could guide me from there, very beginner language
– Sharan Banik
Nov 16 '18 at 19:54
I have added a code, maybe you could guide me from there, very beginner language
– Sharan Banik
Nov 16 '18 at 19:54
add a comment |
This program will do what you need, but you really don't need any recursivness. Recursive functions, in case you don't know, is a function that calls itself. Computing factorials, for example, would use a recursive function:
#include <iostream>
#include <string>
int main()
{
std::string input;
std::cout << "User Enter: ";
std::cin >> input;
int num8 = 0;
int num5 = 0;
for (std::string::size_type i = 0; i < input.size(); ++i)
{
if (input[i] == '8')
{
num8++;
}
if (input[i] == '5')
{
num5++;
}
if (input[i] == '2' || input[i] == '4' || input[i] == '6' || input[i] == '8')
{
input[i] = '0';
}
}
std::cout << "Count of 5 & 8: " << num5 + num8 << std::endl;
std::cout << "Replace Even : " << input << std::endl;
}
What this does and is grab input from the user, then loops through each character in the string. If it comes to an 8
or 5
, it increments a counter. At the same time, it checks the character to see if it's an even number (2
, 4
, 6
, or 8
) and if so, it replaces it with a 0
. Finally, it prints the output.
add a comment |
This program will do what you need, but you really don't need any recursivness. Recursive functions, in case you don't know, is a function that calls itself. Computing factorials, for example, would use a recursive function:
#include <iostream>
#include <string>
int main()
{
std::string input;
std::cout << "User Enter: ";
std::cin >> input;
int num8 = 0;
int num5 = 0;
for (std::string::size_type i = 0; i < input.size(); ++i)
{
if (input[i] == '8')
{
num8++;
}
if (input[i] == '5')
{
num5++;
}
if (input[i] == '2' || input[i] == '4' || input[i] == '6' || input[i] == '8')
{
input[i] = '0';
}
}
std::cout << "Count of 5 & 8: " << num5 + num8 << std::endl;
std::cout << "Replace Even : " << input << std::endl;
}
What this does and is grab input from the user, then loops through each character in the string. If it comes to an 8
or 5
, it increments a counter. At the same time, it checks the character to see if it's an even number (2
, 4
, 6
, or 8
) and if so, it replaces it with a 0
. Finally, it prints the output.
add a comment |
This program will do what you need, but you really don't need any recursivness. Recursive functions, in case you don't know, is a function that calls itself. Computing factorials, for example, would use a recursive function:
#include <iostream>
#include <string>
int main()
{
std::string input;
std::cout << "User Enter: ";
std::cin >> input;
int num8 = 0;
int num5 = 0;
for (std::string::size_type i = 0; i < input.size(); ++i)
{
if (input[i] == '8')
{
num8++;
}
if (input[i] == '5')
{
num5++;
}
if (input[i] == '2' || input[i] == '4' || input[i] == '6' || input[i] == '8')
{
input[i] = '0';
}
}
std::cout << "Count of 5 & 8: " << num5 + num8 << std::endl;
std::cout << "Replace Even : " << input << std::endl;
}
What this does and is grab input from the user, then loops through each character in the string. If it comes to an 8
or 5
, it increments a counter. At the same time, it checks the character to see if it's an even number (2
, 4
, 6
, or 8
) and if so, it replaces it with a 0
. Finally, it prints the output.
This program will do what you need, but you really don't need any recursivness. Recursive functions, in case you don't know, is a function that calls itself. Computing factorials, for example, would use a recursive function:
#include <iostream>
#include <string>
int main()
{
std::string input;
std::cout << "User Enter: ";
std::cin >> input;
int num8 = 0;
int num5 = 0;
for (std::string::size_type i = 0; i < input.size(); ++i)
{
if (input[i] == '8')
{
num8++;
}
if (input[i] == '5')
{
num5++;
}
if (input[i] == '2' || input[i] == '4' || input[i] == '6' || input[i] == '8')
{
input[i] = '0';
}
}
std::cout << "Count of 5 & 8: " << num5 + num8 << std::endl;
std::cout << "Replace Even : " << input << std::endl;
}
What this does and is grab input from the user, then loops through each character in the string. If it comes to an 8
or 5
, it increments a counter. At the same time, it checks the character to see if it's an even number (2
, 4
, 6
, or 8
) and if so, it replaces it with a 0
. Finally, it prints the output.
answered Nov 16 '18 at 19:26


IcemanindIcemanind
32k39139251
32k39139251
add a comment |
add a comment |
Here I suggest using std::string
class with its many functions for operating on character sequences, much easier than writing your own algorithms.
The key to recursion is 3 part:
- Define a base case that handles no matching characters
- Define a recursive case that handles the first occurrence of a matching character
- Define a recursive case that continues to search the remainder of the string after part 2 is met.
Works for any length input.
#include <iostream>
#include <string>
int numChars(char, std::string, int);
std::string replaceEvenWithZero(std::string);
int main()
{
std::string str;
std::cout << "User Enter: ";
std::cin >> str;
// Display the number of times the '5' or '8' appear in the string.
std::cout << "Count of 5 & 8: " << numChars('5', str, 0) + numChars('8', str, 0) << " times.n";
std::cout << "Replace even with 0: " << replaceEvenWithZero(str) << std::endl;
return 0;
}
int numChars(char search, std::string str, int subscript)
{
if (subscript >= str.length())
{
// Base case: end of the string was reached.
return 0;
}
else if (str[subscript] == search)
{
// Recursive case: A matching character was found. Return
// 1 plus the number of times the search character appears
// in the string.
return 1 + numChars(search, str, subscript + 1);
}
else
{
// Recursive case: A character that does not match the search
// character was found. Return the number of times the search
// character appears in the rest of the string.
return numChars(search, str, subscript + 1);
}
}
std::string replaceEvenWithZero(std::string str)
{
// check the index (position) of each character in the string
for (int i=0; i < str.length(); i++){
// if the index even number position
// (divides by 2 with no remainder, then true)
// replace character with `0` at this index
if (i % 2 != 0){
str = str.substr(0,i-1) + "0" + str.substr(i, str.length());
}
}
return str; // return our newly created string with `0`s
}
Demo:
User Enter: 4585
Count of 5 & 8: 3 times.
Replace even with 0: 0505
User Enter: 6856756453
Count of 5 & 8: 4 times.
Replace even with 0: 0806050403
add a comment |
Here I suggest using std::string
class with its many functions for operating on character sequences, much easier than writing your own algorithms.
The key to recursion is 3 part:
- Define a base case that handles no matching characters
- Define a recursive case that handles the first occurrence of a matching character
- Define a recursive case that continues to search the remainder of the string after part 2 is met.
Works for any length input.
#include <iostream>
#include <string>
int numChars(char, std::string, int);
std::string replaceEvenWithZero(std::string);
int main()
{
std::string str;
std::cout << "User Enter: ";
std::cin >> str;
// Display the number of times the '5' or '8' appear in the string.
std::cout << "Count of 5 & 8: " << numChars('5', str, 0) + numChars('8', str, 0) << " times.n";
std::cout << "Replace even with 0: " << replaceEvenWithZero(str) << std::endl;
return 0;
}
int numChars(char search, std::string str, int subscript)
{
if (subscript >= str.length())
{
// Base case: end of the string was reached.
return 0;
}
else if (str[subscript] == search)
{
// Recursive case: A matching character was found. Return
// 1 plus the number of times the search character appears
// in the string.
return 1 + numChars(search, str, subscript + 1);
}
else
{
// Recursive case: A character that does not match the search
// character was found. Return the number of times the search
// character appears in the rest of the string.
return numChars(search, str, subscript + 1);
}
}
std::string replaceEvenWithZero(std::string str)
{
// check the index (position) of each character in the string
for (int i=0; i < str.length(); i++){
// if the index even number position
// (divides by 2 with no remainder, then true)
// replace character with `0` at this index
if (i % 2 != 0){
str = str.substr(0,i-1) + "0" + str.substr(i, str.length());
}
}
return str; // return our newly created string with `0`s
}
Demo:
User Enter: 4585
Count of 5 & 8: 3 times.
Replace even with 0: 0505
User Enter: 6856756453
Count of 5 & 8: 4 times.
Replace even with 0: 0806050403
add a comment |
Here I suggest using std::string
class with its many functions for operating on character sequences, much easier than writing your own algorithms.
The key to recursion is 3 part:
- Define a base case that handles no matching characters
- Define a recursive case that handles the first occurrence of a matching character
- Define a recursive case that continues to search the remainder of the string after part 2 is met.
Works for any length input.
#include <iostream>
#include <string>
int numChars(char, std::string, int);
std::string replaceEvenWithZero(std::string);
int main()
{
std::string str;
std::cout << "User Enter: ";
std::cin >> str;
// Display the number of times the '5' or '8' appear in the string.
std::cout << "Count of 5 & 8: " << numChars('5', str, 0) + numChars('8', str, 0) << " times.n";
std::cout << "Replace even with 0: " << replaceEvenWithZero(str) << std::endl;
return 0;
}
int numChars(char search, std::string str, int subscript)
{
if (subscript >= str.length())
{
// Base case: end of the string was reached.
return 0;
}
else if (str[subscript] == search)
{
// Recursive case: A matching character was found. Return
// 1 plus the number of times the search character appears
// in the string.
return 1 + numChars(search, str, subscript + 1);
}
else
{
// Recursive case: A character that does not match the search
// character was found. Return the number of times the search
// character appears in the rest of the string.
return numChars(search, str, subscript + 1);
}
}
std::string replaceEvenWithZero(std::string str)
{
// check the index (position) of each character in the string
for (int i=0; i < str.length(); i++){
// if the index even number position
// (divides by 2 with no remainder, then true)
// replace character with `0` at this index
if (i % 2 != 0){
str = str.substr(0,i-1) + "0" + str.substr(i, str.length());
}
}
return str; // return our newly created string with `0`s
}
Demo:
User Enter: 4585
Count of 5 & 8: 3 times.
Replace even with 0: 0505
User Enter: 6856756453
Count of 5 & 8: 4 times.
Replace even with 0: 0806050403
Here I suggest using std::string
class with its many functions for operating on character sequences, much easier than writing your own algorithms.
The key to recursion is 3 part:
- Define a base case that handles no matching characters
- Define a recursive case that handles the first occurrence of a matching character
- Define a recursive case that continues to search the remainder of the string after part 2 is met.
Works for any length input.
#include <iostream>
#include <string>
int numChars(char, std::string, int);
std::string replaceEvenWithZero(std::string);
int main()
{
std::string str;
std::cout << "User Enter: ";
std::cin >> str;
// Display the number of times the '5' or '8' appear in the string.
std::cout << "Count of 5 & 8: " << numChars('5', str, 0) + numChars('8', str, 0) << " times.n";
std::cout << "Replace even with 0: " << replaceEvenWithZero(str) << std::endl;
return 0;
}
int numChars(char search, std::string str, int subscript)
{
if (subscript >= str.length())
{
// Base case: end of the string was reached.
return 0;
}
else if (str[subscript] == search)
{
// Recursive case: A matching character was found. Return
// 1 plus the number of times the search character appears
// in the string.
return 1 + numChars(search, str, subscript + 1);
}
else
{
// Recursive case: A character that does not match the search
// character was found. Return the number of times the search
// character appears in the rest of the string.
return numChars(search, str, subscript + 1);
}
}
std::string replaceEvenWithZero(std::string str)
{
// check the index (position) of each character in the string
for (int i=0; i < str.length(); i++){
// if the index even number position
// (divides by 2 with no remainder, then true)
// replace character with `0` at this index
if (i % 2 != 0){
str = str.substr(0,i-1) + "0" + str.substr(i, str.length());
}
}
return str; // return our newly created string with `0`s
}
Demo:
User Enter: 4585
Count of 5 & 8: 3 times.
Replace even with 0: 0505
User Enter: 6856756453
Count of 5 & 8: 4 times.
Replace even with 0: 0806050403
edited Nov 16 '18 at 23:59
answered Nov 16 '18 at 23:44


davedwardsdavedwards
6,04321235
6,04321235
add a comment |
add a comment |
Other folks responded to your question with solutions that use different signatures than your code:
int countThreeFives(int num);
int replaceEven(int num);
and/or simply tossed the recursion requirement. Also, you specified int
yet asked your user for a positive number -- if your code really only works on positive numbers, you should consider unsigned int
instead of int
. Or just deal with negative numbers. Addressing all this, and keeping the spirit of your original code:
#include <iostream>
using namespace std;
int countThreeFives(int number) {
if (number != 0) {
int count = 0, digit = abs(number % 10);
if (digit == 5 || digit == 8) {
count++;
}
return count + countThreeFives(number / 10);
}
return 0;
}
int replaceEven(int number) {
if (number != 0) {
int digit = (number % 10) * (number % 2);
return ((number > 0) ? 1 : -1) * (replaceEven(abs(number) / 10) * 10 + digit);
}
return number;
}
int main() {
int number;
cout << "Enter a number: ";
cin >> number;
cout << "The count of 8 & 5 is: " << countThreeFives(number) << endl;
cout << "Replacing even with 0: " << replaceEven(number) << endl;
}
Example usage showing negative number support:
> ./a.out
Enter a number: -382135
The count of 8 & 5 is: 2
Replacing even with 0: -300135
>
However, there are a couple of other issues with your two function taking int
as arguments and returning an int
. E.g., your example:
User enter: 4585
Count of 5 & 8: 3
Replace even with 0: 0505
is wrong as using integers the replaced number would be:
> ./a.out
Enter a number: 4585
The count of 8 & 5 is: 3
Replacing even with 0: 505
Mac-mini>
I.e. no leading zeros. You only get a leading zero with a non-numeric approach. The other issue with using integers is that the length of the number input is limited. A typically maximum length input would be:
> ./a.out
Enter a number: 356756453
The count of 8 & 5 is: 3
Replacing even with 0: 350750053
>
Any longer, and you'd need to use a larger integer type (e.g. unsigned long long
) or again take a non-numeric approach.
add a comment |
Other folks responded to your question with solutions that use different signatures than your code:
int countThreeFives(int num);
int replaceEven(int num);
and/or simply tossed the recursion requirement. Also, you specified int
yet asked your user for a positive number -- if your code really only works on positive numbers, you should consider unsigned int
instead of int
. Or just deal with negative numbers. Addressing all this, and keeping the spirit of your original code:
#include <iostream>
using namespace std;
int countThreeFives(int number) {
if (number != 0) {
int count = 0, digit = abs(number % 10);
if (digit == 5 || digit == 8) {
count++;
}
return count + countThreeFives(number / 10);
}
return 0;
}
int replaceEven(int number) {
if (number != 0) {
int digit = (number % 10) * (number % 2);
return ((number > 0) ? 1 : -1) * (replaceEven(abs(number) / 10) * 10 + digit);
}
return number;
}
int main() {
int number;
cout << "Enter a number: ";
cin >> number;
cout << "The count of 8 & 5 is: " << countThreeFives(number) << endl;
cout << "Replacing even with 0: " << replaceEven(number) << endl;
}
Example usage showing negative number support:
> ./a.out
Enter a number: -382135
The count of 8 & 5 is: 2
Replacing even with 0: -300135
>
However, there are a couple of other issues with your two function taking int
as arguments and returning an int
. E.g., your example:
User enter: 4585
Count of 5 & 8: 3
Replace even with 0: 0505
is wrong as using integers the replaced number would be:
> ./a.out
Enter a number: 4585
The count of 8 & 5 is: 3
Replacing even with 0: 505
Mac-mini>
I.e. no leading zeros. You only get a leading zero with a non-numeric approach. The other issue with using integers is that the length of the number input is limited. A typically maximum length input would be:
> ./a.out
Enter a number: 356756453
The count of 8 & 5 is: 3
Replacing even with 0: 350750053
>
Any longer, and you'd need to use a larger integer type (e.g. unsigned long long
) or again take a non-numeric approach.
add a comment |
Other folks responded to your question with solutions that use different signatures than your code:
int countThreeFives(int num);
int replaceEven(int num);
and/or simply tossed the recursion requirement. Also, you specified int
yet asked your user for a positive number -- if your code really only works on positive numbers, you should consider unsigned int
instead of int
. Or just deal with negative numbers. Addressing all this, and keeping the spirit of your original code:
#include <iostream>
using namespace std;
int countThreeFives(int number) {
if (number != 0) {
int count = 0, digit = abs(number % 10);
if (digit == 5 || digit == 8) {
count++;
}
return count + countThreeFives(number / 10);
}
return 0;
}
int replaceEven(int number) {
if (number != 0) {
int digit = (number % 10) * (number % 2);
return ((number > 0) ? 1 : -1) * (replaceEven(abs(number) / 10) * 10 + digit);
}
return number;
}
int main() {
int number;
cout << "Enter a number: ";
cin >> number;
cout << "The count of 8 & 5 is: " << countThreeFives(number) << endl;
cout << "Replacing even with 0: " << replaceEven(number) << endl;
}
Example usage showing negative number support:
> ./a.out
Enter a number: -382135
The count of 8 & 5 is: 2
Replacing even with 0: -300135
>
However, there are a couple of other issues with your two function taking int
as arguments and returning an int
. E.g., your example:
User enter: 4585
Count of 5 & 8: 3
Replace even with 0: 0505
is wrong as using integers the replaced number would be:
> ./a.out
Enter a number: 4585
The count of 8 & 5 is: 3
Replacing even with 0: 505
Mac-mini>
I.e. no leading zeros. You only get a leading zero with a non-numeric approach. The other issue with using integers is that the length of the number input is limited. A typically maximum length input would be:
> ./a.out
Enter a number: 356756453
The count of 8 & 5 is: 3
Replacing even with 0: 350750053
>
Any longer, and you'd need to use a larger integer type (e.g. unsigned long long
) or again take a non-numeric approach.
Other folks responded to your question with solutions that use different signatures than your code:
int countThreeFives(int num);
int replaceEven(int num);
and/or simply tossed the recursion requirement. Also, you specified int
yet asked your user for a positive number -- if your code really only works on positive numbers, you should consider unsigned int
instead of int
. Or just deal with negative numbers. Addressing all this, and keeping the spirit of your original code:
#include <iostream>
using namespace std;
int countThreeFives(int number) {
if (number != 0) {
int count = 0, digit = abs(number % 10);
if (digit == 5 || digit == 8) {
count++;
}
return count + countThreeFives(number / 10);
}
return 0;
}
int replaceEven(int number) {
if (number != 0) {
int digit = (number % 10) * (number % 2);
return ((number > 0) ? 1 : -1) * (replaceEven(abs(number) / 10) * 10 + digit);
}
return number;
}
int main() {
int number;
cout << "Enter a number: ";
cin >> number;
cout << "The count of 8 & 5 is: " << countThreeFives(number) << endl;
cout << "Replacing even with 0: " << replaceEven(number) << endl;
}
Example usage showing negative number support:
> ./a.out
Enter a number: -382135
The count of 8 & 5 is: 2
Replacing even with 0: -300135
>
However, there are a couple of other issues with your two function taking int
as arguments and returning an int
. E.g., your example:
User enter: 4585
Count of 5 & 8: 3
Replace even with 0: 0505
is wrong as using integers the replaced number would be:
> ./a.out
Enter a number: 4585
The count of 8 & 5 is: 3
Replacing even with 0: 505
Mac-mini>
I.e. no leading zeros. You only get a leading zero with a non-numeric approach. The other issue with using integers is that the length of the number input is limited. A typically maximum length input would be:
> ./a.out
Enter a number: 356756453
The count of 8 & 5 is: 3
Replacing even with 0: 350750053
>
Any longer, and you'd need to use a larger integer type (e.g. unsigned long long
) or again take a non-numeric approach.
answered Nov 17 '18 at 1:17
cdlanecdlane
20k21245
20k21245
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53343912%2fcount-specific-digits-and-replace-even-digits-with-0-using-recursion%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
S mHoqHH1gfwH6TBS74dSP G0sBiyfrdopKL5Yu ofX5F2A2pI0mcSUVRgharXqTpsqFxFaSzRRhCmGRk1k7w
Please give a Minimal, complete, and verifiable piece of code: stackoverflow.com/help/mcve
– Little Boy Blue
Nov 16 '18 at 19:05
@LittleBoyBlue there are a bunch of shortcuts you can use for commonly used messages. For example,
[mcve]
expands to Minimal, Complete, and Verifiable example.– user4581301
Nov 16 '18 at 19:43
Sharan, you will find your questions will almost always get a better reception when accompanied by a credible attempt at solving the problem. Often these attempts are close enough to correct that they can be fixed with a few simple alterations and if not the code provides a baseline of your understanding of the problem so that answers can be better tailored. On the social front, they show that you aren't simply expecting people to do your homework for you.
– user4581301
Nov 16 '18 at 19:47
I wasn't expecting that no one to do my homework, but I was totally confused trying to understand recursive.
– Sharan Banik
Nov 16 '18 at 19:53
The Master once said that to understand recursion first you must understand recursion. Since this really isn't that helpful, the Master was a bit of a dick, the best thing to do is to start with really simple stuff like "print out a string one character at a time with recursion." Understand complicated recursion by first understanding simple recursion.
– user4581301
Nov 17 '18 at 1:34