Linq on nested Dictionary
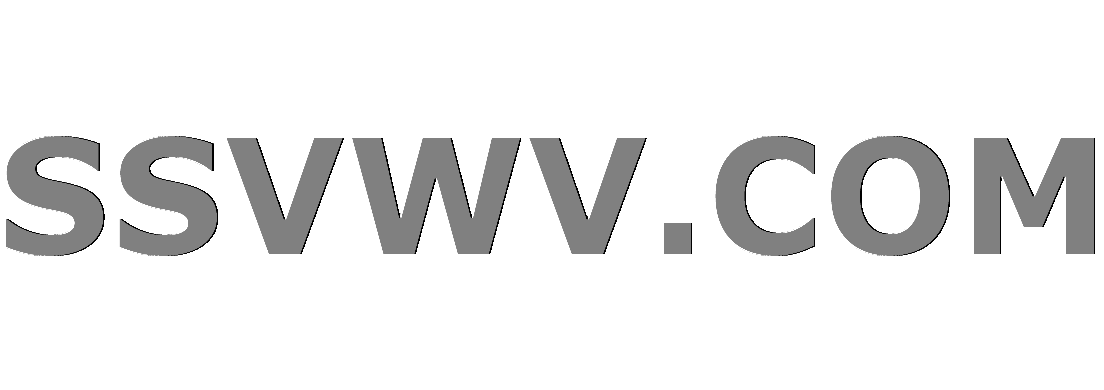
Multi tool use
I have a dictionary of dictionary and I want to find a value from inner dictionary by Linq .
My code is:
private Dictionary<string, Dictionary<int, string>> SubCategoryDictionary = new Dictionary<string, Dictionary<int, string>>();
private Dictionary<int, string> BGA_Dictionary = new Dictionary<int, string>();
private Dictionary<int, string> Lead3D_Dictionary = new Dictionary<int, string>();
private Dictionary<int, string> Lead2D_Dictionary = new Dictionary<int, string>();
private Dictionary<int, string> Leadless_Dictionary = new Dictionary<int, string>();
private Dictionary<int, string> PIC_Dictionary = new Dictionary<int, string>();
In my constructor I have all values like this:--
BGA_Dictionary.Add(1, "Body_Measurement");
BGA_Dictionary.Add(2, "Ball_Measurement");
SubCategoryDictionary.Add("BGA", BGA_Dictionary);
Lead3D_Dictionary.Add(1, "Component_Height");
Lead3D_Dictionary.Add(2, "Rib_Measurement");
SubCategoryDictionary.Add("Package", Lead3D_Dictionary);
Lead2D_Dictionary.Add(1, "Dirt_Inspection");
Lead2D_Dictionary.Add(2, "Half_Cut_Inspection");
SubCategoryDictionary.Add("Mark", Lead2D_Dictionary);
Now I need a Lambda expression which will give me something like :
when key of SubCategoryDictionary ="Mark" and key of Lead3D_Dictionary =2 then I should get "Rib_Measurement".
I tried with following code :
string q = (from cls in SubCategoryDictionary
from s in cls.Value
where cls.Key == "Mark" && s.Key == 3
select s.Value).FirstOrDefault();
foreach (var a in q)
{
}
This above code works but I need in lambda expression. So if someone help me in formation of Lambda formation. It will be of great help.
Thanks.
c# .net linq
add a comment |
I have a dictionary of dictionary and I want to find a value from inner dictionary by Linq .
My code is:
private Dictionary<string, Dictionary<int, string>> SubCategoryDictionary = new Dictionary<string, Dictionary<int, string>>();
private Dictionary<int, string> BGA_Dictionary = new Dictionary<int, string>();
private Dictionary<int, string> Lead3D_Dictionary = new Dictionary<int, string>();
private Dictionary<int, string> Lead2D_Dictionary = new Dictionary<int, string>();
private Dictionary<int, string> Leadless_Dictionary = new Dictionary<int, string>();
private Dictionary<int, string> PIC_Dictionary = new Dictionary<int, string>();
In my constructor I have all values like this:--
BGA_Dictionary.Add(1, "Body_Measurement");
BGA_Dictionary.Add(2, "Ball_Measurement");
SubCategoryDictionary.Add("BGA", BGA_Dictionary);
Lead3D_Dictionary.Add(1, "Component_Height");
Lead3D_Dictionary.Add(2, "Rib_Measurement");
SubCategoryDictionary.Add("Package", Lead3D_Dictionary);
Lead2D_Dictionary.Add(1, "Dirt_Inspection");
Lead2D_Dictionary.Add(2, "Half_Cut_Inspection");
SubCategoryDictionary.Add("Mark", Lead2D_Dictionary);
Now I need a Lambda expression which will give me something like :
when key of SubCategoryDictionary ="Mark" and key of Lead3D_Dictionary =2 then I should get "Rib_Measurement".
I tried with following code :
string q = (from cls in SubCategoryDictionary
from s in cls.Value
where cls.Key == "Mark" && s.Key == 3
select s.Value).FirstOrDefault();
foreach (var a in q)
{
}
This above code works but I need in lambda expression. So if someone help me in formation of Lambda formation. It will be of great help.
Thanks.
c# .net linq
3
are you talking aboutSubCategoryDictionary["Mark"][2]
?
– vasily.sib
Nov 15 '18 at 3:54
@vasily.sib I was trying like string q = (from cls in SubCategoryDictionary from s in cls.Value where cls.Key == "Mark" && s.Key == 3 select s.Value).FirstOrDefault(); and this gives me result. Just can not form a Lambda expression out of this. Could you please help me on Lambda formation.
– New Programmer
Nov 15 '18 at 4:45
your linq is just accessing single element by keys. Why do you ever think you need a lambda for this? Anyway, here it is:Func<string,int,string> lambda = (k1, k2) => SubCategoryDictionary[k1][k2];
– vasily.sib
Nov 15 '18 at 11:20
@vasily.sib how to mark your answer as the answer . Thanks for this . please post it in answer section. thanks a lot.
– New Programmer
Nov 16 '18 at 4:27
add a comment |
I have a dictionary of dictionary and I want to find a value from inner dictionary by Linq .
My code is:
private Dictionary<string, Dictionary<int, string>> SubCategoryDictionary = new Dictionary<string, Dictionary<int, string>>();
private Dictionary<int, string> BGA_Dictionary = new Dictionary<int, string>();
private Dictionary<int, string> Lead3D_Dictionary = new Dictionary<int, string>();
private Dictionary<int, string> Lead2D_Dictionary = new Dictionary<int, string>();
private Dictionary<int, string> Leadless_Dictionary = new Dictionary<int, string>();
private Dictionary<int, string> PIC_Dictionary = new Dictionary<int, string>();
In my constructor I have all values like this:--
BGA_Dictionary.Add(1, "Body_Measurement");
BGA_Dictionary.Add(2, "Ball_Measurement");
SubCategoryDictionary.Add("BGA", BGA_Dictionary);
Lead3D_Dictionary.Add(1, "Component_Height");
Lead3D_Dictionary.Add(2, "Rib_Measurement");
SubCategoryDictionary.Add("Package", Lead3D_Dictionary);
Lead2D_Dictionary.Add(1, "Dirt_Inspection");
Lead2D_Dictionary.Add(2, "Half_Cut_Inspection");
SubCategoryDictionary.Add("Mark", Lead2D_Dictionary);
Now I need a Lambda expression which will give me something like :
when key of SubCategoryDictionary ="Mark" and key of Lead3D_Dictionary =2 then I should get "Rib_Measurement".
I tried with following code :
string q = (from cls in SubCategoryDictionary
from s in cls.Value
where cls.Key == "Mark" && s.Key == 3
select s.Value).FirstOrDefault();
foreach (var a in q)
{
}
This above code works but I need in lambda expression. So if someone help me in formation of Lambda formation. It will be of great help.
Thanks.
c# .net linq
I have a dictionary of dictionary and I want to find a value from inner dictionary by Linq .
My code is:
private Dictionary<string, Dictionary<int, string>> SubCategoryDictionary = new Dictionary<string, Dictionary<int, string>>();
private Dictionary<int, string> BGA_Dictionary = new Dictionary<int, string>();
private Dictionary<int, string> Lead3D_Dictionary = new Dictionary<int, string>();
private Dictionary<int, string> Lead2D_Dictionary = new Dictionary<int, string>();
private Dictionary<int, string> Leadless_Dictionary = new Dictionary<int, string>();
private Dictionary<int, string> PIC_Dictionary = new Dictionary<int, string>();
In my constructor I have all values like this:--
BGA_Dictionary.Add(1, "Body_Measurement");
BGA_Dictionary.Add(2, "Ball_Measurement");
SubCategoryDictionary.Add("BGA", BGA_Dictionary);
Lead3D_Dictionary.Add(1, "Component_Height");
Lead3D_Dictionary.Add(2, "Rib_Measurement");
SubCategoryDictionary.Add("Package", Lead3D_Dictionary);
Lead2D_Dictionary.Add(1, "Dirt_Inspection");
Lead2D_Dictionary.Add(2, "Half_Cut_Inspection");
SubCategoryDictionary.Add("Mark", Lead2D_Dictionary);
Now I need a Lambda expression which will give me something like :
when key of SubCategoryDictionary ="Mark" and key of Lead3D_Dictionary =2 then I should get "Rib_Measurement".
I tried with following code :
string q = (from cls in SubCategoryDictionary
from s in cls.Value
where cls.Key == "Mark" && s.Key == 3
select s.Value).FirstOrDefault();
foreach (var a in q)
{
}
This above code works but I need in lambda expression. So if someone help me in formation of Lambda formation. It will be of great help.
Thanks.
c# .net linq
c# .net linq
edited Nov 15 '18 at 4:50
New Programmer
asked Nov 15 '18 at 3:45
New ProgrammerNew Programmer
102
102
3
are you talking aboutSubCategoryDictionary["Mark"][2]
?
– vasily.sib
Nov 15 '18 at 3:54
@vasily.sib I was trying like string q = (from cls in SubCategoryDictionary from s in cls.Value where cls.Key == "Mark" && s.Key == 3 select s.Value).FirstOrDefault(); and this gives me result. Just can not form a Lambda expression out of this. Could you please help me on Lambda formation.
– New Programmer
Nov 15 '18 at 4:45
your linq is just accessing single element by keys. Why do you ever think you need a lambda for this? Anyway, here it is:Func<string,int,string> lambda = (k1, k2) => SubCategoryDictionary[k1][k2];
– vasily.sib
Nov 15 '18 at 11:20
@vasily.sib how to mark your answer as the answer . Thanks for this . please post it in answer section. thanks a lot.
– New Programmer
Nov 16 '18 at 4:27
add a comment |
3
are you talking aboutSubCategoryDictionary["Mark"][2]
?
– vasily.sib
Nov 15 '18 at 3:54
@vasily.sib I was trying like string q = (from cls in SubCategoryDictionary from s in cls.Value where cls.Key == "Mark" && s.Key == 3 select s.Value).FirstOrDefault(); and this gives me result. Just can not form a Lambda expression out of this. Could you please help me on Lambda formation.
– New Programmer
Nov 15 '18 at 4:45
your linq is just accessing single element by keys. Why do you ever think you need a lambda for this? Anyway, here it is:Func<string,int,string> lambda = (k1, k2) => SubCategoryDictionary[k1][k2];
– vasily.sib
Nov 15 '18 at 11:20
@vasily.sib how to mark your answer as the answer . Thanks for this . please post it in answer section. thanks a lot.
– New Programmer
Nov 16 '18 at 4:27
3
3
are you talking about
SubCategoryDictionary["Mark"][2]
?– vasily.sib
Nov 15 '18 at 3:54
are you talking about
SubCategoryDictionary["Mark"][2]
?– vasily.sib
Nov 15 '18 at 3:54
@vasily.sib I was trying like string q = (from cls in SubCategoryDictionary from s in cls.Value where cls.Key == "Mark" && s.Key == 3 select s.Value).FirstOrDefault(); and this gives me result. Just can not form a Lambda expression out of this. Could you please help me on Lambda formation.
– New Programmer
Nov 15 '18 at 4:45
@vasily.sib I was trying like string q = (from cls in SubCategoryDictionary from s in cls.Value where cls.Key == "Mark" && s.Key == 3 select s.Value).FirstOrDefault(); and this gives me result. Just can not form a Lambda expression out of this. Could you please help me on Lambda formation.
– New Programmer
Nov 15 '18 at 4:45
your linq is just accessing single element by keys. Why do you ever think you need a lambda for this? Anyway, here it is:
Func<string,int,string> lambda = (k1, k2) => SubCategoryDictionary[k1][k2];
– vasily.sib
Nov 15 '18 at 11:20
your linq is just accessing single element by keys. Why do you ever think you need a lambda for this? Anyway, here it is:
Func<string,int,string> lambda = (k1, k2) => SubCategoryDictionary[k1][k2];
– vasily.sib
Nov 15 '18 at 11:20
@vasily.sib how to mark your answer as the answer . Thanks for this . please post it in answer section. thanks a lot.
– New Programmer
Nov 16 '18 at 4:27
@vasily.sib how to mark your answer as the answer . Thanks for this . please post it in answer section. thanks a lot.
– New Programmer
Nov 16 '18 at 4:27
add a comment |
2 Answers
2
active
oldest
votes
I'm not sure why you ever need a lambda for accessing a dictionary by keys, but here it is:
Func<string,int,string> lambda = (k1, k2) => SubCategoryDictionary[k1][k2];
Now you can invoke it with var subCategory = lambda("Mark", 2);
add a comment |
I guess below code should get you the value you are trying to get.
var lead3 = SubCategoryDictionary["Mark"].SingleOrDefault(x => x.Key == 2).Value;
The idea is to use the Key of the first dictionary and get the value of it than filter it with SingleOrDefault method by providing a key to the inner dictionary value you are interested in.
Hope this helps
Why useSingleOrDefault
?
– mjwills
Nov 15 '18 at 4:25
@Farrukh Manzoor you have mentioned SubCategoryDictionary["Mark"] which is not linq . I was trying something like string q = (from cls in SubCategoryDictionary from s in cls.Value where cls.Key == "Mark" && s.Key == 3 select s.Value).FirstOrDefault(); it is giving me output when I iterate over foreach but I need in a Lambda format way
– New Programmer
Nov 15 '18 at 4:43
@NewProgrammer Using LINQ to do a lookup on aDictionary
is foolhardy. ADictionary
is incredibly good at key lookups. Let it do its job. When I want to drive somewhere, I don't get the kids in the card and then push the car. No, I drive it - because that is what it is good at.
– mjwills
Nov 15 '18 at 5:16
@mjwills nice comment . will follow.
– New Programmer
Nov 15 '18 at 5:34
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53312132%2flinq-on-nested-dictionary%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
I'm not sure why you ever need a lambda for accessing a dictionary by keys, but here it is:
Func<string,int,string> lambda = (k1, k2) => SubCategoryDictionary[k1][k2];
Now you can invoke it with var subCategory = lambda("Mark", 2);
add a comment |
I'm not sure why you ever need a lambda for accessing a dictionary by keys, but here it is:
Func<string,int,string> lambda = (k1, k2) => SubCategoryDictionary[k1][k2];
Now you can invoke it with var subCategory = lambda("Mark", 2);
add a comment |
I'm not sure why you ever need a lambda for accessing a dictionary by keys, but here it is:
Func<string,int,string> lambda = (k1, k2) => SubCategoryDictionary[k1][k2];
Now you can invoke it with var subCategory = lambda("Mark", 2);
I'm not sure why you ever need a lambda for accessing a dictionary by keys, but here it is:
Func<string,int,string> lambda = (k1, k2) => SubCategoryDictionary[k1][k2];
Now you can invoke it with var subCategory = lambda("Mark", 2);
answered Nov 16 '18 at 8:11
vasily.sibvasily.sib
2,21821021
2,21821021
add a comment |
add a comment |
I guess below code should get you the value you are trying to get.
var lead3 = SubCategoryDictionary["Mark"].SingleOrDefault(x => x.Key == 2).Value;
The idea is to use the Key of the first dictionary and get the value of it than filter it with SingleOrDefault method by providing a key to the inner dictionary value you are interested in.
Hope this helps
Why useSingleOrDefault
?
– mjwills
Nov 15 '18 at 4:25
@Farrukh Manzoor you have mentioned SubCategoryDictionary["Mark"] which is not linq . I was trying something like string q = (from cls in SubCategoryDictionary from s in cls.Value where cls.Key == "Mark" && s.Key == 3 select s.Value).FirstOrDefault(); it is giving me output when I iterate over foreach but I need in a Lambda format way
– New Programmer
Nov 15 '18 at 4:43
@NewProgrammer Using LINQ to do a lookup on aDictionary
is foolhardy. ADictionary
is incredibly good at key lookups. Let it do its job. When I want to drive somewhere, I don't get the kids in the card and then push the car. No, I drive it - because that is what it is good at.
– mjwills
Nov 15 '18 at 5:16
@mjwills nice comment . will follow.
– New Programmer
Nov 15 '18 at 5:34
add a comment |
I guess below code should get you the value you are trying to get.
var lead3 = SubCategoryDictionary["Mark"].SingleOrDefault(x => x.Key == 2).Value;
The idea is to use the Key of the first dictionary and get the value of it than filter it with SingleOrDefault method by providing a key to the inner dictionary value you are interested in.
Hope this helps
Why useSingleOrDefault
?
– mjwills
Nov 15 '18 at 4:25
@Farrukh Manzoor you have mentioned SubCategoryDictionary["Mark"] which is not linq . I was trying something like string q = (from cls in SubCategoryDictionary from s in cls.Value where cls.Key == "Mark" && s.Key == 3 select s.Value).FirstOrDefault(); it is giving me output when I iterate over foreach but I need in a Lambda format way
– New Programmer
Nov 15 '18 at 4:43
@NewProgrammer Using LINQ to do a lookup on aDictionary
is foolhardy. ADictionary
is incredibly good at key lookups. Let it do its job. When I want to drive somewhere, I don't get the kids in the card and then push the car. No, I drive it - because that is what it is good at.
– mjwills
Nov 15 '18 at 5:16
@mjwills nice comment . will follow.
– New Programmer
Nov 15 '18 at 5:34
add a comment |
I guess below code should get you the value you are trying to get.
var lead3 = SubCategoryDictionary["Mark"].SingleOrDefault(x => x.Key == 2).Value;
The idea is to use the Key of the first dictionary and get the value of it than filter it with SingleOrDefault method by providing a key to the inner dictionary value you are interested in.
Hope this helps
I guess below code should get you the value you are trying to get.
var lead3 = SubCategoryDictionary["Mark"].SingleOrDefault(x => x.Key == 2).Value;
The idea is to use the Key of the first dictionary and get the value of it than filter it with SingleOrDefault method by providing a key to the inner dictionary value you are interested in.
Hope this helps
answered Nov 15 '18 at 4:19
Farrukh ManzoorFarrukh Manzoor
493
493
Why useSingleOrDefault
?
– mjwills
Nov 15 '18 at 4:25
@Farrukh Manzoor you have mentioned SubCategoryDictionary["Mark"] which is not linq . I was trying something like string q = (from cls in SubCategoryDictionary from s in cls.Value where cls.Key == "Mark" && s.Key == 3 select s.Value).FirstOrDefault(); it is giving me output when I iterate over foreach but I need in a Lambda format way
– New Programmer
Nov 15 '18 at 4:43
@NewProgrammer Using LINQ to do a lookup on aDictionary
is foolhardy. ADictionary
is incredibly good at key lookups. Let it do its job. When I want to drive somewhere, I don't get the kids in the card and then push the car. No, I drive it - because that is what it is good at.
– mjwills
Nov 15 '18 at 5:16
@mjwills nice comment . will follow.
– New Programmer
Nov 15 '18 at 5:34
add a comment |
Why useSingleOrDefault
?
– mjwills
Nov 15 '18 at 4:25
@Farrukh Manzoor you have mentioned SubCategoryDictionary["Mark"] which is not linq . I was trying something like string q = (from cls in SubCategoryDictionary from s in cls.Value where cls.Key == "Mark" && s.Key == 3 select s.Value).FirstOrDefault(); it is giving me output when I iterate over foreach but I need in a Lambda format way
– New Programmer
Nov 15 '18 at 4:43
@NewProgrammer Using LINQ to do a lookup on aDictionary
is foolhardy. ADictionary
is incredibly good at key lookups. Let it do its job. When I want to drive somewhere, I don't get the kids in the card and then push the car. No, I drive it - because that is what it is good at.
– mjwills
Nov 15 '18 at 5:16
@mjwills nice comment . will follow.
– New Programmer
Nov 15 '18 at 5:34
Why use
SingleOrDefault
?– mjwills
Nov 15 '18 at 4:25
Why use
SingleOrDefault
?– mjwills
Nov 15 '18 at 4:25
@Farrukh Manzoor you have mentioned SubCategoryDictionary["Mark"] which is not linq . I was trying something like string q = (from cls in SubCategoryDictionary from s in cls.Value where cls.Key == "Mark" && s.Key == 3 select s.Value).FirstOrDefault(); it is giving me output when I iterate over foreach but I need in a Lambda format way
– New Programmer
Nov 15 '18 at 4:43
@Farrukh Manzoor you have mentioned SubCategoryDictionary["Mark"] which is not linq . I was trying something like string q = (from cls in SubCategoryDictionary from s in cls.Value where cls.Key == "Mark" && s.Key == 3 select s.Value).FirstOrDefault(); it is giving me output when I iterate over foreach but I need in a Lambda format way
– New Programmer
Nov 15 '18 at 4:43
@NewProgrammer Using LINQ to do a lookup on a
Dictionary
is foolhardy. A Dictionary
is incredibly good at key lookups. Let it do its job. When I want to drive somewhere, I don't get the kids in the card and then push the car. No, I drive it - because that is what it is good at.– mjwills
Nov 15 '18 at 5:16
@NewProgrammer Using LINQ to do a lookup on a
Dictionary
is foolhardy. A Dictionary
is incredibly good at key lookups. Let it do its job. When I want to drive somewhere, I don't get the kids in the card and then push the car. No, I drive it - because that is what it is good at.– mjwills
Nov 15 '18 at 5:16
@mjwills nice comment . will follow.
– New Programmer
Nov 15 '18 at 5:34
@mjwills nice comment . will follow.
– New Programmer
Nov 15 '18 at 5:34
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53312132%2flinq-on-nested-dictionary%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
w28wf6sw,1,z6TJ2 LVEjJ,9V,Z,Yp47O0Q3qdU,z
3
are you talking about
SubCategoryDictionary["Mark"][2]
?– vasily.sib
Nov 15 '18 at 3:54
@vasily.sib I was trying like string q = (from cls in SubCategoryDictionary from s in cls.Value where cls.Key == "Mark" && s.Key == 3 select s.Value).FirstOrDefault(); and this gives me result. Just can not form a Lambda expression out of this. Could you please help me on Lambda formation.
– New Programmer
Nov 15 '18 at 4:45
your linq is just accessing single element by keys. Why do you ever think you need a lambda for this? Anyway, here it is:
Func<string,int,string> lambda = (k1, k2) => SubCategoryDictionary[k1][k2];
– vasily.sib
Nov 15 '18 at 11:20
@vasily.sib how to mark your answer as the answer . Thanks for this . please post it in answer section. thanks a lot.
– New Programmer
Nov 16 '18 at 4:27