IF and assignment statement are Thread safe
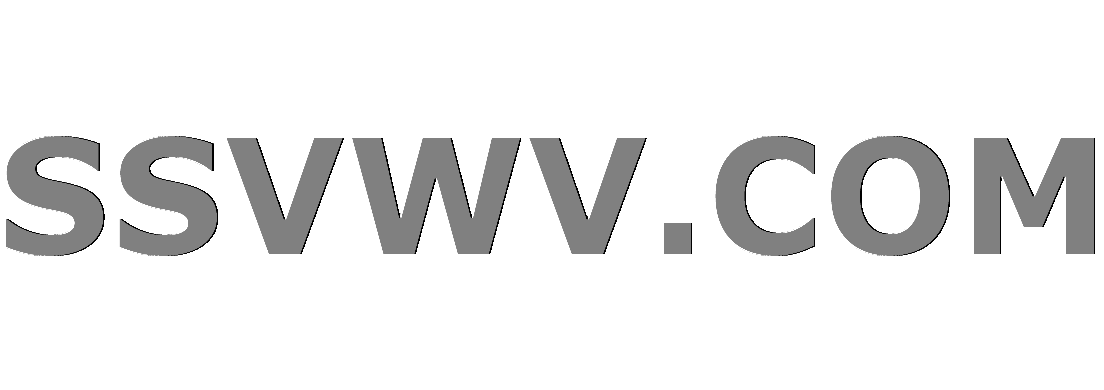
Multi tool use
private LinkedList<Integer> list = new LinkedList<Integer>();
private synchronized int foo(int x){
if(x>=0){ //do something
list.add(x);
return x }
else {
int tmp = list.removeFirst();
return tmp;
}
return -1;
}
public void test(int id)
{
if(foo(id)==-1)
//do something
}
public void test2(int id){
int y=foo(id);
System.out.println(id+" "+ y);
}
if the test and test2 method has been accessed by multiple threads is thread-safe meanwhile test and test2 are not declared synchronized?
Ps sorry i correct the code
java multithreading thread-safety
add a comment |
private LinkedList<Integer> list = new LinkedList<Integer>();
private synchronized int foo(int x){
if(x>=0){ //do something
list.add(x);
return x }
else {
int tmp = list.removeFirst();
return tmp;
}
return -1;
}
public void test(int id)
{
if(foo(id)==-1)
//do something
}
public void test2(int id){
int y=foo(id);
System.out.println(id+" "+ y);
}
if the test and test2 method has been accessed by multiple threads is thread-safe meanwhile test and test2 are not declared synchronized?
Ps sorry i correct the code
java multithreading thread-safety
1
This code isn't legal. But it is thread safe. No shared variables are being modified. All I see is local variables.
– Elliott Frisch
Nov 15 '18 at 18:18
// do something
needs elaboration, but the two comments above are correct.id
andx
are local variables, are primitives and therefore copies. There's nothing that can go wrong even if you remove thesynchronized
.
– markspace
Nov 15 '18 at 18:26
1
@markspace you need to synchronize the access to list because class LinkedList is not thread safe.
– Donat
Nov 15 '18 at 18:39
Ah, didn't see that, or it was added later. You're right, the linked list needs to be protected. @Donat
– markspace
Nov 15 '18 at 22:43
add a comment |
private LinkedList<Integer> list = new LinkedList<Integer>();
private synchronized int foo(int x){
if(x>=0){ //do something
list.add(x);
return x }
else {
int tmp = list.removeFirst();
return tmp;
}
return -1;
}
public void test(int id)
{
if(foo(id)==-1)
//do something
}
public void test2(int id){
int y=foo(id);
System.out.println(id+" "+ y);
}
if the test and test2 method has been accessed by multiple threads is thread-safe meanwhile test and test2 are not declared synchronized?
Ps sorry i correct the code
java multithreading thread-safety
private LinkedList<Integer> list = new LinkedList<Integer>();
private synchronized int foo(int x){
if(x>=0){ //do something
list.add(x);
return x }
else {
int tmp = list.removeFirst();
return tmp;
}
return -1;
}
public void test(int id)
{
if(foo(id)==-1)
//do something
}
public void test2(int id){
int y=foo(id);
System.out.println(id+" "+ y);
}
if the test and test2 method has been accessed by multiple threads is thread-safe meanwhile test and test2 are not declared synchronized?
Ps sorry i correct the code
java multithreading thread-safety
java multithreading thread-safety
edited Nov 15 '18 at 18:57
screatives
asked Nov 15 '18 at 18:16


screativesscreatives
32
32
1
This code isn't legal. But it is thread safe. No shared variables are being modified. All I see is local variables.
– Elliott Frisch
Nov 15 '18 at 18:18
// do something
needs elaboration, but the two comments above are correct.id
andx
are local variables, are primitives and therefore copies. There's nothing that can go wrong even if you remove thesynchronized
.
– markspace
Nov 15 '18 at 18:26
1
@markspace you need to synchronize the access to list because class LinkedList is not thread safe.
– Donat
Nov 15 '18 at 18:39
Ah, didn't see that, or it was added later. You're right, the linked list needs to be protected. @Donat
– markspace
Nov 15 '18 at 22:43
add a comment |
1
This code isn't legal. But it is thread safe. No shared variables are being modified. All I see is local variables.
– Elliott Frisch
Nov 15 '18 at 18:18
// do something
needs elaboration, but the two comments above are correct.id
andx
are local variables, are primitives and therefore copies. There's nothing that can go wrong even if you remove thesynchronized
.
– markspace
Nov 15 '18 at 18:26
1
@markspace you need to synchronize the access to list because class LinkedList is not thread safe.
– Donat
Nov 15 '18 at 18:39
Ah, didn't see that, or it was added later. You're right, the linked list needs to be protected. @Donat
– markspace
Nov 15 '18 at 22:43
1
1
This code isn't legal. But it is thread safe. No shared variables are being modified. All I see is local variables.
– Elliott Frisch
Nov 15 '18 at 18:18
This code isn't legal. But it is thread safe. No shared variables are being modified. All I see is local variables.
– Elliott Frisch
Nov 15 '18 at 18:18
// do something
needs elaboration, but the two comments above are correct. id
and x
are local variables, are primitives and therefore copies. There's nothing that can go wrong even if you remove the synchronized
.– markspace
Nov 15 '18 at 18:26
// do something
needs elaboration, but the two comments above are correct. id
and x
are local variables, are primitives and therefore copies. There's nothing that can go wrong even if you remove the synchronized
.– markspace
Nov 15 '18 at 18:26
1
1
@markspace you need to synchronize the access to list because class LinkedList is not thread safe.
– Donat
Nov 15 '18 at 18:39
@markspace you need to synchronize the access to list because class LinkedList is not thread safe.
– Donat
Nov 15 '18 at 18:39
Ah, didn't see that, or it was added later. You're right, the linked list needs to be protected. @Donat
– markspace
Nov 15 '18 at 22:43
Ah, didn't see that, or it was added later. You're right, the linked list needs to be protected. @Donat
– markspace
Nov 15 '18 at 22:43
add a comment |
1 Answer
1
active
oldest
votes
It is threadsafe if you don't touch the list anywhere but in the code you provided. If you doubt yet - provide all the code.
Short explanation:
Method foo
uses a List
object that can be shared between threads, but the list created during object instantiation so there is HB between write and read it. The method itself is synchronized so there is HB relationship between every reads and writes into the list.
In your test
and test2
methods you don't use any objects that can be shared between different threads, and you use only foo method that is threadsafe so the methods are threadsag.
PS: But if you modify the list field, or work with the list inside other methods it depends on how exectly you do it.
Is not possibile that a thread A undergoes preemption after go out of foo metod so a second thread B enter in foo Go out too and undergoes preemption before the assignment and result of thread A swap on thread B? Sorry again for my English
– screatives
Nov 15 '18 at 21:28
@screatives, I am not sure that I understood you right, but Java memory model guaranties that all changes made by thread A inside methodfoo
will be visible by thread B when B enters into methodfoo
. And only one thread can be inside methodfoo
at moment.
– Aleksandr Semyannikov
Nov 15 '18 at 21:39
Yep I know it but I talk about the method test and test2 the statement assignment is atomic? I mean could be happened the thread A after finish the method foo and before the assignment undergoes preemption and another thread enter in the foo finish too and preemption again so now the system.out print y of thread A and Id of thread B?
– screatives
Nov 15 '18 at 22:34
@screatives no, each thread will get it's own y, and that y will be used to constructid+" "+ y
– Aleksandr Semyannikov
Nov 16 '18 at 5:26
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53325605%2fif-and-assignment-statement-are-thread-safe%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
It is threadsafe if you don't touch the list anywhere but in the code you provided. If you doubt yet - provide all the code.
Short explanation:
Method foo
uses a List
object that can be shared between threads, but the list created during object instantiation so there is HB between write and read it. The method itself is synchronized so there is HB relationship between every reads and writes into the list.
In your test
and test2
methods you don't use any objects that can be shared between different threads, and you use only foo method that is threadsafe so the methods are threadsag.
PS: But if you modify the list field, or work with the list inside other methods it depends on how exectly you do it.
Is not possibile that a thread A undergoes preemption after go out of foo metod so a second thread B enter in foo Go out too and undergoes preemption before the assignment and result of thread A swap on thread B? Sorry again for my English
– screatives
Nov 15 '18 at 21:28
@screatives, I am not sure that I understood you right, but Java memory model guaranties that all changes made by thread A inside methodfoo
will be visible by thread B when B enters into methodfoo
. And only one thread can be inside methodfoo
at moment.
– Aleksandr Semyannikov
Nov 15 '18 at 21:39
Yep I know it but I talk about the method test and test2 the statement assignment is atomic? I mean could be happened the thread A after finish the method foo and before the assignment undergoes preemption and another thread enter in the foo finish too and preemption again so now the system.out print y of thread A and Id of thread B?
– screatives
Nov 15 '18 at 22:34
@screatives no, each thread will get it's own y, and that y will be used to constructid+" "+ y
– Aleksandr Semyannikov
Nov 16 '18 at 5:26
add a comment |
It is threadsafe if you don't touch the list anywhere but in the code you provided. If you doubt yet - provide all the code.
Short explanation:
Method foo
uses a List
object that can be shared between threads, but the list created during object instantiation so there is HB between write and read it. The method itself is synchronized so there is HB relationship between every reads and writes into the list.
In your test
and test2
methods you don't use any objects that can be shared between different threads, and you use only foo method that is threadsafe so the methods are threadsag.
PS: But if you modify the list field, or work with the list inside other methods it depends on how exectly you do it.
Is not possibile that a thread A undergoes preemption after go out of foo metod so a second thread B enter in foo Go out too and undergoes preemption before the assignment and result of thread A swap on thread B? Sorry again for my English
– screatives
Nov 15 '18 at 21:28
@screatives, I am not sure that I understood you right, but Java memory model guaranties that all changes made by thread A inside methodfoo
will be visible by thread B when B enters into methodfoo
. And only one thread can be inside methodfoo
at moment.
– Aleksandr Semyannikov
Nov 15 '18 at 21:39
Yep I know it but I talk about the method test and test2 the statement assignment is atomic? I mean could be happened the thread A after finish the method foo and before the assignment undergoes preemption and another thread enter in the foo finish too and preemption again so now the system.out print y of thread A and Id of thread B?
– screatives
Nov 15 '18 at 22:34
@screatives no, each thread will get it's own y, and that y will be used to constructid+" "+ y
– Aleksandr Semyannikov
Nov 16 '18 at 5:26
add a comment |
It is threadsafe if you don't touch the list anywhere but in the code you provided. If you doubt yet - provide all the code.
Short explanation:
Method foo
uses a List
object that can be shared between threads, but the list created during object instantiation so there is HB between write and read it. The method itself is synchronized so there is HB relationship between every reads and writes into the list.
In your test
and test2
methods you don't use any objects that can be shared between different threads, and you use only foo method that is threadsafe so the methods are threadsag.
PS: But if you modify the list field, or work with the list inside other methods it depends on how exectly you do it.
It is threadsafe if you don't touch the list anywhere but in the code you provided. If you doubt yet - provide all the code.
Short explanation:
Method foo
uses a List
object that can be shared between threads, but the list created during object instantiation so there is HB between write and read it. The method itself is synchronized so there is HB relationship between every reads and writes into the list.
In your test
and test2
methods you don't use any objects that can be shared between different threads, and you use only foo method that is threadsafe so the methods are threadsag.
PS: But if you modify the list field, or work with the list inside other methods it depends on how exectly you do it.
edited Nov 18 '18 at 18:25
answered Nov 15 '18 at 19:48
Aleksandr SemyannikovAleksandr Semyannikov
591217
591217
Is not possibile that a thread A undergoes preemption after go out of foo metod so a second thread B enter in foo Go out too and undergoes preemption before the assignment and result of thread A swap on thread B? Sorry again for my English
– screatives
Nov 15 '18 at 21:28
@screatives, I am not sure that I understood you right, but Java memory model guaranties that all changes made by thread A inside methodfoo
will be visible by thread B when B enters into methodfoo
. And only one thread can be inside methodfoo
at moment.
– Aleksandr Semyannikov
Nov 15 '18 at 21:39
Yep I know it but I talk about the method test and test2 the statement assignment is atomic? I mean could be happened the thread A after finish the method foo and before the assignment undergoes preemption and another thread enter in the foo finish too and preemption again so now the system.out print y of thread A and Id of thread B?
– screatives
Nov 15 '18 at 22:34
@screatives no, each thread will get it's own y, and that y will be used to constructid+" "+ y
– Aleksandr Semyannikov
Nov 16 '18 at 5:26
add a comment |
Is not possibile that a thread A undergoes preemption after go out of foo metod so a second thread B enter in foo Go out too and undergoes preemption before the assignment and result of thread A swap on thread B? Sorry again for my English
– screatives
Nov 15 '18 at 21:28
@screatives, I am not sure that I understood you right, but Java memory model guaranties that all changes made by thread A inside methodfoo
will be visible by thread B when B enters into methodfoo
. And only one thread can be inside methodfoo
at moment.
– Aleksandr Semyannikov
Nov 15 '18 at 21:39
Yep I know it but I talk about the method test and test2 the statement assignment is atomic? I mean could be happened the thread A after finish the method foo and before the assignment undergoes preemption and another thread enter in the foo finish too and preemption again so now the system.out print y of thread A and Id of thread B?
– screatives
Nov 15 '18 at 22:34
@screatives no, each thread will get it's own y, and that y will be used to constructid+" "+ y
– Aleksandr Semyannikov
Nov 16 '18 at 5:26
Is not possibile that a thread A undergoes preemption after go out of foo metod so a second thread B enter in foo Go out too and undergoes preemption before the assignment and result of thread A swap on thread B? Sorry again for my English
– screatives
Nov 15 '18 at 21:28
Is not possibile that a thread A undergoes preemption after go out of foo metod so a second thread B enter in foo Go out too and undergoes preemption before the assignment and result of thread A swap on thread B? Sorry again for my English
– screatives
Nov 15 '18 at 21:28
@screatives, I am not sure that I understood you right, but Java memory model guaranties that all changes made by thread A inside method
foo
will be visible by thread B when B enters into method foo
. And only one thread can be inside method foo
at moment.– Aleksandr Semyannikov
Nov 15 '18 at 21:39
@screatives, I am not sure that I understood you right, but Java memory model guaranties that all changes made by thread A inside method
foo
will be visible by thread B when B enters into method foo
. And only one thread can be inside method foo
at moment.– Aleksandr Semyannikov
Nov 15 '18 at 21:39
Yep I know it but I talk about the method test and test2 the statement assignment is atomic? I mean could be happened the thread A after finish the method foo and before the assignment undergoes preemption and another thread enter in the foo finish too and preemption again so now the system.out print y of thread A and Id of thread B?
– screatives
Nov 15 '18 at 22:34
Yep I know it but I talk about the method test and test2 the statement assignment is atomic? I mean could be happened the thread A after finish the method foo and before the assignment undergoes preemption and another thread enter in the foo finish too and preemption again so now the system.out print y of thread A and Id of thread B?
– screatives
Nov 15 '18 at 22:34
@screatives no, each thread will get it's own y, and that y will be used to construct
id+" "+ y
– Aleksandr Semyannikov
Nov 16 '18 at 5:26
@screatives no, each thread will get it's own y, and that y will be used to construct
id+" "+ y
– Aleksandr Semyannikov
Nov 16 '18 at 5:26
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53325605%2fif-and-assignment-statement-are-thread-safe%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
4HR8hhbwJIa,kjm3XyBUiMb6,yIepS0xxXMazn bz Jj9wmActTMvWDXxWx,fG61s6i,fMllWfvDjnbqUbFQCPmcq,1FgXzDgUL VO,9
1
This code isn't legal. But it is thread safe. No shared variables are being modified. All I see is local variables.
– Elliott Frisch
Nov 15 '18 at 18:18
// do something
needs elaboration, but the two comments above are correct.id
andx
are local variables, are primitives and therefore copies. There's nothing that can go wrong even if you remove thesynchronized
.– markspace
Nov 15 '18 at 18:26
1
@markspace you need to synchronize the access to list because class LinkedList is not thread safe.
– Donat
Nov 15 '18 at 18:39
Ah, didn't see that, or it was added later. You're right, the linked list needs to be protected. @Donat
– markspace
Nov 15 '18 at 22:43