I've got some troubles with return
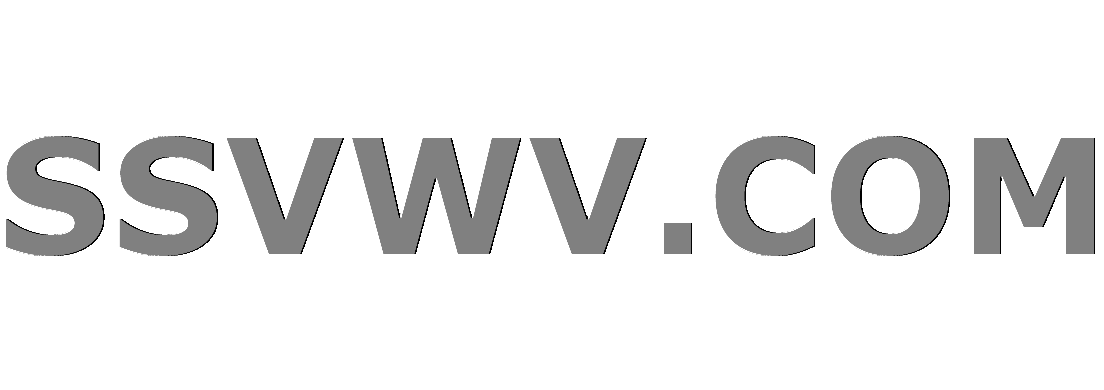
Multi tool use
I have a trouble: when button onclicked, I need to return position of a block and hold it till next onclick. On next click, I need to take position and change it again and hold its number. And make it for eternity xD. But I've done something wrong. Please help.
And one thing more: I need to make a reset position by clicking reset button. Reset must be to the 1.
window.onload = function core() {
// creating elements
let platform = document.createElement('div');
platform.setAttribute('id', 'platform');
document.body.appendChild(platform);
let up = document.createElement('button');
up.setAttribute('id', 'up');
up.setAttribute('class', 'actionButton');
document.body.appendChild(up);
document.getElementById('up').innerHTML = 'up';
let down = document.createElement('button');
down.setAttribute('id', 'down');
down.setAttribute('class', 'actionButton');
document.body.appendChild(down);
document.getElementById('down').innerHTML = 'down';
let left = document.createElement('button');
left.setAttribute('id', 'left');
left.setAttribute('class', 'actionButton');
document.body.appendChild(left);
document.getElementById('left').innerHTML = 'left';
let right = document.createElement('button');
right.setAttribute('id', 'right');
right.setAttribute('class', 'actionButton');
document.body.appendChild(right);
document.getElementById('right').innerHTML = 'right';
let reset = document.createElement('button');
reset.setAttribute('id', 'reset');
reset.setAttribute('class', 'actionButton');
document.body.appendChild(reset);
document.getElementById('reset').innerHTML = 'reset';
// binding platform
let count = 0;
let defaultPosition = 1;
for (let i = 1; i < 101; i++) {
let grid = document.createElement('button');
grid.setAttribute('id', i);
grid.setAttribute('class', 'eachGridBlock');
document.getElementById('platform').appendChild(grid);
count++;
if (count === 10) {
let newLine = document.createElement('br');
document.getElementById('platform').appendChild(newLine);
count = 0;
};
};
// waiting for action
document.getElementById(defaultPosition).setAttribute('class', 'focusedBlock');
console.log(defaultPosition);
document.getElementById('up').onclick = function() {
processUp(defaultPosition)
};
document.getElementById('down').onclick = function() {
processDown(defaultPosition)
};
document.getElementById('left').onclick = function() {
processLeft(defaultPosition)
};
document.getElementById('right').onclick = function() {
processRight(defaultPosition)
};
document.getElementById('reset').onclick = function() {
processReset(defaultPosition)
};
// action functions
function processUp(positionX) {
let nowPosition = positionX;
if (nowPosition <= 10) {
alert('you cant step here');
} else {
let nextPosition = nowPosition - 10;
document.getElementById(nowPosition).setAttribute('class', 'eachGridBlock');
document.getElementById(nextPosition).setAttribute('class', 'focusedBlock');
positionX = nextPosition;
};
console.log(positionX);
return positionX;
};
function processDown(positionX) {
let nowPosition = positionX;
if (nowPosition >= 91) {
alert('you cant step here');
} else {
let nextPosition = nowPosition + 10;
document.getElementById(nowPosition).setAttribute('class', 'eachGridBlock');
document.getElementById(nextPosition).setAttribute('class', 'focusedBlock');
positionX = nextPosition;
};
console.log(positionX);
return positionX;
};
function processLeft(positionX) {
let nowPosition = positionX;
if (nowPosition === 1 || nowPosition === 11 || nowPosition === 21 || nowPosition === 31 || nowPosition === 41 || nowPosition === 51 || nowPosition === 61 || nowPosition === 71 || nowPosition === 81 || nowPosition === 91) {
alert('you cant step here');
} else {
let nextPosition = nowPosition - 1;
document.getElementById(nowPosition).setAttribute('class', 'eachGridBlock');
document.getElementById(nextPosition).setAttribute('class', 'focusedBlock');
positionX = nextPosition;
};
console.log(positionX);
return positionX;
};
function processRight(positionX) {
let nowPosition = positionX;
if (nowPosition === 10 || nowPosition === 20 || nowPosition === 30 || nowPosition === 40 || nowPosition === 50 || nowPosition === 60 || nowPosition === 70 || nowPosition === 80 || nowPosition === 90 || nowPosition === 100) {
alert('you cant step here');
} else {
let nextPosition = nowPosition + 1;
document.getElementById(nowPosition).setAttribute('class', 'eachGridBlock');
document.getElementById(nextPosition).setAttribute('class', 'focusedBlock');
positionX = nextPosition;
};
console.log(positionX);
return positionX;
};
function processReset(positionX) {
let nowPosition = positionX
let nextPosition = 1;
document.getElementById(nowPosition).setAttribute('class', 'eachGridBlock');
document.getElementById(nextPosition).setAttribute('class', 'focusedBlock');
return positionX;
};
};
* {
margin: 0;
padding: 0;
}
#plarform {
width: 500px;
height: 500px;
background-color: #222222;
}
.eachGridBlock {
margin: 0;
padding: 0;
width: 50px;
height: 50px;
background-color: #000044;
border: 0;
}
.focusedBlock {
margin: 0;
padding: 0;
width: 50px;
height: 50px;
background-color: #9900ff;
border: 0;
}
.actionButton {
border: 0;
width: 100px;
height: 35px;
background-color: #999999;
color: #222222;
outline: none;
}
.actionButton:hover {
background-color: #dddddd;
}
<html>
<head>
</head>
<body>
</body>
</html>
javascript html
|
show 7 more comments
I have a trouble: when button onclicked, I need to return position of a block and hold it till next onclick. On next click, I need to take position and change it again and hold its number. And make it for eternity xD. But I've done something wrong. Please help.
And one thing more: I need to make a reset position by clicking reset button. Reset must be to the 1.
window.onload = function core() {
// creating elements
let platform = document.createElement('div');
platform.setAttribute('id', 'platform');
document.body.appendChild(platform);
let up = document.createElement('button');
up.setAttribute('id', 'up');
up.setAttribute('class', 'actionButton');
document.body.appendChild(up);
document.getElementById('up').innerHTML = 'up';
let down = document.createElement('button');
down.setAttribute('id', 'down');
down.setAttribute('class', 'actionButton');
document.body.appendChild(down);
document.getElementById('down').innerHTML = 'down';
let left = document.createElement('button');
left.setAttribute('id', 'left');
left.setAttribute('class', 'actionButton');
document.body.appendChild(left);
document.getElementById('left').innerHTML = 'left';
let right = document.createElement('button');
right.setAttribute('id', 'right');
right.setAttribute('class', 'actionButton');
document.body.appendChild(right);
document.getElementById('right').innerHTML = 'right';
let reset = document.createElement('button');
reset.setAttribute('id', 'reset');
reset.setAttribute('class', 'actionButton');
document.body.appendChild(reset);
document.getElementById('reset').innerHTML = 'reset';
// binding platform
let count = 0;
let defaultPosition = 1;
for (let i = 1; i < 101; i++) {
let grid = document.createElement('button');
grid.setAttribute('id', i);
grid.setAttribute('class', 'eachGridBlock');
document.getElementById('platform').appendChild(grid);
count++;
if (count === 10) {
let newLine = document.createElement('br');
document.getElementById('platform').appendChild(newLine);
count = 0;
};
};
// waiting for action
document.getElementById(defaultPosition).setAttribute('class', 'focusedBlock');
console.log(defaultPosition);
document.getElementById('up').onclick = function() {
processUp(defaultPosition)
};
document.getElementById('down').onclick = function() {
processDown(defaultPosition)
};
document.getElementById('left').onclick = function() {
processLeft(defaultPosition)
};
document.getElementById('right').onclick = function() {
processRight(defaultPosition)
};
document.getElementById('reset').onclick = function() {
processReset(defaultPosition)
};
// action functions
function processUp(positionX) {
let nowPosition = positionX;
if (nowPosition <= 10) {
alert('you cant step here');
} else {
let nextPosition = nowPosition - 10;
document.getElementById(nowPosition).setAttribute('class', 'eachGridBlock');
document.getElementById(nextPosition).setAttribute('class', 'focusedBlock');
positionX = nextPosition;
};
console.log(positionX);
return positionX;
};
function processDown(positionX) {
let nowPosition = positionX;
if (nowPosition >= 91) {
alert('you cant step here');
} else {
let nextPosition = nowPosition + 10;
document.getElementById(nowPosition).setAttribute('class', 'eachGridBlock');
document.getElementById(nextPosition).setAttribute('class', 'focusedBlock');
positionX = nextPosition;
};
console.log(positionX);
return positionX;
};
function processLeft(positionX) {
let nowPosition = positionX;
if (nowPosition === 1 || nowPosition === 11 || nowPosition === 21 || nowPosition === 31 || nowPosition === 41 || nowPosition === 51 || nowPosition === 61 || nowPosition === 71 || nowPosition === 81 || nowPosition === 91) {
alert('you cant step here');
} else {
let nextPosition = nowPosition - 1;
document.getElementById(nowPosition).setAttribute('class', 'eachGridBlock');
document.getElementById(nextPosition).setAttribute('class', 'focusedBlock');
positionX = nextPosition;
};
console.log(positionX);
return positionX;
};
function processRight(positionX) {
let nowPosition = positionX;
if (nowPosition === 10 || nowPosition === 20 || nowPosition === 30 || nowPosition === 40 || nowPosition === 50 || nowPosition === 60 || nowPosition === 70 || nowPosition === 80 || nowPosition === 90 || nowPosition === 100) {
alert('you cant step here');
} else {
let nextPosition = nowPosition + 1;
document.getElementById(nowPosition).setAttribute('class', 'eachGridBlock');
document.getElementById(nextPosition).setAttribute('class', 'focusedBlock');
positionX = nextPosition;
};
console.log(positionX);
return positionX;
};
function processReset(positionX) {
let nowPosition = positionX
let nextPosition = 1;
document.getElementById(nowPosition).setAttribute('class', 'eachGridBlock');
document.getElementById(nextPosition).setAttribute('class', 'focusedBlock');
return positionX;
};
};
* {
margin: 0;
padding: 0;
}
#plarform {
width: 500px;
height: 500px;
background-color: #222222;
}
.eachGridBlock {
margin: 0;
padding: 0;
width: 50px;
height: 50px;
background-color: #000044;
border: 0;
}
.focusedBlock {
margin: 0;
padding: 0;
width: 50px;
height: 50px;
background-color: #9900ff;
border: 0;
}
.actionButton {
border: 0;
width: 100px;
height: 35px;
background-color: #999999;
color: #222222;
outline: none;
}
.actionButton:hover {
background-color: #dddddd;
}
<html>
<head>
</head>
<body>
</body>
</html>
javascript html
1
I cannot think of more unreadable core. Perhaps the odd line break and comment?
– Ed Heal
Nov 15 '18 at 0:36
Please only add the bare essentials.
– Ed Heal
Nov 15 '18 at 0:37
Look at the code's comments. I think it's enough commenting. Just need to return position value and use it when some button clicked again.
– Eugene Zolotuhin
Nov 15 '18 at 0:38
Look at the //action functions
– Eugene Zolotuhin
Nov 15 '18 at 0:39
1. 4 comments stating the obvious. 2.A lot of code for us to decipher. 3. Where is the HTML. 4 Perhaps reproduce in jsfiddle et al.
– Ed Heal
Nov 15 '18 at 0:41
|
show 7 more comments
I have a trouble: when button onclicked, I need to return position of a block and hold it till next onclick. On next click, I need to take position and change it again and hold its number. And make it for eternity xD. But I've done something wrong. Please help.
And one thing more: I need to make a reset position by clicking reset button. Reset must be to the 1.
window.onload = function core() {
// creating elements
let platform = document.createElement('div');
platform.setAttribute('id', 'platform');
document.body.appendChild(platform);
let up = document.createElement('button');
up.setAttribute('id', 'up');
up.setAttribute('class', 'actionButton');
document.body.appendChild(up);
document.getElementById('up').innerHTML = 'up';
let down = document.createElement('button');
down.setAttribute('id', 'down');
down.setAttribute('class', 'actionButton');
document.body.appendChild(down);
document.getElementById('down').innerHTML = 'down';
let left = document.createElement('button');
left.setAttribute('id', 'left');
left.setAttribute('class', 'actionButton');
document.body.appendChild(left);
document.getElementById('left').innerHTML = 'left';
let right = document.createElement('button');
right.setAttribute('id', 'right');
right.setAttribute('class', 'actionButton');
document.body.appendChild(right);
document.getElementById('right').innerHTML = 'right';
let reset = document.createElement('button');
reset.setAttribute('id', 'reset');
reset.setAttribute('class', 'actionButton');
document.body.appendChild(reset);
document.getElementById('reset').innerHTML = 'reset';
// binding platform
let count = 0;
let defaultPosition = 1;
for (let i = 1; i < 101; i++) {
let grid = document.createElement('button');
grid.setAttribute('id', i);
grid.setAttribute('class', 'eachGridBlock');
document.getElementById('platform').appendChild(grid);
count++;
if (count === 10) {
let newLine = document.createElement('br');
document.getElementById('platform').appendChild(newLine);
count = 0;
};
};
// waiting for action
document.getElementById(defaultPosition).setAttribute('class', 'focusedBlock');
console.log(defaultPosition);
document.getElementById('up').onclick = function() {
processUp(defaultPosition)
};
document.getElementById('down').onclick = function() {
processDown(defaultPosition)
};
document.getElementById('left').onclick = function() {
processLeft(defaultPosition)
};
document.getElementById('right').onclick = function() {
processRight(defaultPosition)
};
document.getElementById('reset').onclick = function() {
processReset(defaultPosition)
};
// action functions
function processUp(positionX) {
let nowPosition = positionX;
if (nowPosition <= 10) {
alert('you cant step here');
} else {
let nextPosition = nowPosition - 10;
document.getElementById(nowPosition).setAttribute('class', 'eachGridBlock');
document.getElementById(nextPosition).setAttribute('class', 'focusedBlock');
positionX = nextPosition;
};
console.log(positionX);
return positionX;
};
function processDown(positionX) {
let nowPosition = positionX;
if (nowPosition >= 91) {
alert('you cant step here');
} else {
let nextPosition = nowPosition + 10;
document.getElementById(nowPosition).setAttribute('class', 'eachGridBlock');
document.getElementById(nextPosition).setAttribute('class', 'focusedBlock');
positionX = nextPosition;
};
console.log(positionX);
return positionX;
};
function processLeft(positionX) {
let nowPosition = positionX;
if (nowPosition === 1 || nowPosition === 11 || nowPosition === 21 || nowPosition === 31 || nowPosition === 41 || nowPosition === 51 || nowPosition === 61 || nowPosition === 71 || nowPosition === 81 || nowPosition === 91) {
alert('you cant step here');
} else {
let nextPosition = nowPosition - 1;
document.getElementById(nowPosition).setAttribute('class', 'eachGridBlock');
document.getElementById(nextPosition).setAttribute('class', 'focusedBlock');
positionX = nextPosition;
};
console.log(positionX);
return positionX;
};
function processRight(positionX) {
let nowPosition = positionX;
if (nowPosition === 10 || nowPosition === 20 || nowPosition === 30 || nowPosition === 40 || nowPosition === 50 || nowPosition === 60 || nowPosition === 70 || nowPosition === 80 || nowPosition === 90 || nowPosition === 100) {
alert('you cant step here');
} else {
let nextPosition = nowPosition + 1;
document.getElementById(nowPosition).setAttribute('class', 'eachGridBlock');
document.getElementById(nextPosition).setAttribute('class', 'focusedBlock');
positionX = nextPosition;
};
console.log(positionX);
return positionX;
};
function processReset(positionX) {
let nowPosition = positionX
let nextPosition = 1;
document.getElementById(nowPosition).setAttribute('class', 'eachGridBlock');
document.getElementById(nextPosition).setAttribute('class', 'focusedBlock');
return positionX;
};
};
* {
margin: 0;
padding: 0;
}
#plarform {
width: 500px;
height: 500px;
background-color: #222222;
}
.eachGridBlock {
margin: 0;
padding: 0;
width: 50px;
height: 50px;
background-color: #000044;
border: 0;
}
.focusedBlock {
margin: 0;
padding: 0;
width: 50px;
height: 50px;
background-color: #9900ff;
border: 0;
}
.actionButton {
border: 0;
width: 100px;
height: 35px;
background-color: #999999;
color: #222222;
outline: none;
}
.actionButton:hover {
background-color: #dddddd;
}
<html>
<head>
</head>
<body>
</body>
</html>
javascript html
I have a trouble: when button onclicked, I need to return position of a block and hold it till next onclick. On next click, I need to take position and change it again and hold its number. And make it for eternity xD. But I've done something wrong. Please help.
And one thing more: I need to make a reset position by clicking reset button. Reset must be to the 1.
window.onload = function core() {
// creating elements
let platform = document.createElement('div');
platform.setAttribute('id', 'platform');
document.body.appendChild(platform);
let up = document.createElement('button');
up.setAttribute('id', 'up');
up.setAttribute('class', 'actionButton');
document.body.appendChild(up);
document.getElementById('up').innerHTML = 'up';
let down = document.createElement('button');
down.setAttribute('id', 'down');
down.setAttribute('class', 'actionButton');
document.body.appendChild(down);
document.getElementById('down').innerHTML = 'down';
let left = document.createElement('button');
left.setAttribute('id', 'left');
left.setAttribute('class', 'actionButton');
document.body.appendChild(left);
document.getElementById('left').innerHTML = 'left';
let right = document.createElement('button');
right.setAttribute('id', 'right');
right.setAttribute('class', 'actionButton');
document.body.appendChild(right);
document.getElementById('right').innerHTML = 'right';
let reset = document.createElement('button');
reset.setAttribute('id', 'reset');
reset.setAttribute('class', 'actionButton');
document.body.appendChild(reset);
document.getElementById('reset').innerHTML = 'reset';
// binding platform
let count = 0;
let defaultPosition = 1;
for (let i = 1; i < 101; i++) {
let grid = document.createElement('button');
grid.setAttribute('id', i);
grid.setAttribute('class', 'eachGridBlock');
document.getElementById('platform').appendChild(grid);
count++;
if (count === 10) {
let newLine = document.createElement('br');
document.getElementById('platform').appendChild(newLine);
count = 0;
};
};
// waiting for action
document.getElementById(defaultPosition).setAttribute('class', 'focusedBlock');
console.log(defaultPosition);
document.getElementById('up').onclick = function() {
processUp(defaultPosition)
};
document.getElementById('down').onclick = function() {
processDown(defaultPosition)
};
document.getElementById('left').onclick = function() {
processLeft(defaultPosition)
};
document.getElementById('right').onclick = function() {
processRight(defaultPosition)
};
document.getElementById('reset').onclick = function() {
processReset(defaultPosition)
};
// action functions
function processUp(positionX) {
let nowPosition = positionX;
if (nowPosition <= 10) {
alert('you cant step here');
} else {
let nextPosition = nowPosition - 10;
document.getElementById(nowPosition).setAttribute('class', 'eachGridBlock');
document.getElementById(nextPosition).setAttribute('class', 'focusedBlock');
positionX = nextPosition;
};
console.log(positionX);
return positionX;
};
function processDown(positionX) {
let nowPosition = positionX;
if (nowPosition >= 91) {
alert('you cant step here');
} else {
let nextPosition = nowPosition + 10;
document.getElementById(nowPosition).setAttribute('class', 'eachGridBlock');
document.getElementById(nextPosition).setAttribute('class', 'focusedBlock');
positionX = nextPosition;
};
console.log(positionX);
return positionX;
};
function processLeft(positionX) {
let nowPosition = positionX;
if (nowPosition === 1 || nowPosition === 11 || nowPosition === 21 || nowPosition === 31 || nowPosition === 41 || nowPosition === 51 || nowPosition === 61 || nowPosition === 71 || nowPosition === 81 || nowPosition === 91) {
alert('you cant step here');
} else {
let nextPosition = nowPosition - 1;
document.getElementById(nowPosition).setAttribute('class', 'eachGridBlock');
document.getElementById(nextPosition).setAttribute('class', 'focusedBlock');
positionX = nextPosition;
};
console.log(positionX);
return positionX;
};
function processRight(positionX) {
let nowPosition = positionX;
if (nowPosition === 10 || nowPosition === 20 || nowPosition === 30 || nowPosition === 40 || nowPosition === 50 || nowPosition === 60 || nowPosition === 70 || nowPosition === 80 || nowPosition === 90 || nowPosition === 100) {
alert('you cant step here');
} else {
let nextPosition = nowPosition + 1;
document.getElementById(nowPosition).setAttribute('class', 'eachGridBlock');
document.getElementById(nextPosition).setAttribute('class', 'focusedBlock');
positionX = nextPosition;
};
console.log(positionX);
return positionX;
};
function processReset(positionX) {
let nowPosition = positionX
let nextPosition = 1;
document.getElementById(nowPosition).setAttribute('class', 'eachGridBlock');
document.getElementById(nextPosition).setAttribute('class', 'focusedBlock');
return positionX;
};
};
* {
margin: 0;
padding: 0;
}
#plarform {
width: 500px;
height: 500px;
background-color: #222222;
}
.eachGridBlock {
margin: 0;
padding: 0;
width: 50px;
height: 50px;
background-color: #000044;
border: 0;
}
.focusedBlock {
margin: 0;
padding: 0;
width: 50px;
height: 50px;
background-color: #9900ff;
border: 0;
}
.actionButton {
border: 0;
width: 100px;
height: 35px;
background-color: #999999;
color: #222222;
outline: none;
}
.actionButton:hover {
background-color: #dddddd;
}
<html>
<head>
</head>
<body>
</body>
</html>
window.onload = function core() {
// creating elements
let platform = document.createElement('div');
platform.setAttribute('id', 'platform');
document.body.appendChild(platform);
let up = document.createElement('button');
up.setAttribute('id', 'up');
up.setAttribute('class', 'actionButton');
document.body.appendChild(up);
document.getElementById('up').innerHTML = 'up';
let down = document.createElement('button');
down.setAttribute('id', 'down');
down.setAttribute('class', 'actionButton');
document.body.appendChild(down);
document.getElementById('down').innerHTML = 'down';
let left = document.createElement('button');
left.setAttribute('id', 'left');
left.setAttribute('class', 'actionButton');
document.body.appendChild(left);
document.getElementById('left').innerHTML = 'left';
let right = document.createElement('button');
right.setAttribute('id', 'right');
right.setAttribute('class', 'actionButton');
document.body.appendChild(right);
document.getElementById('right').innerHTML = 'right';
let reset = document.createElement('button');
reset.setAttribute('id', 'reset');
reset.setAttribute('class', 'actionButton');
document.body.appendChild(reset);
document.getElementById('reset').innerHTML = 'reset';
// binding platform
let count = 0;
let defaultPosition = 1;
for (let i = 1; i < 101; i++) {
let grid = document.createElement('button');
grid.setAttribute('id', i);
grid.setAttribute('class', 'eachGridBlock');
document.getElementById('platform').appendChild(grid);
count++;
if (count === 10) {
let newLine = document.createElement('br');
document.getElementById('platform').appendChild(newLine);
count = 0;
};
};
// waiting for action
document.getElementById(defaultPosition).setAttribute('class', 'focusedBlock');
console.log(defaultPosition);
document.getElementById('up').onclick = function() {
processUp(defaultPosition)
};
document.getElementById('down').onclick = function() {
processDown(defaultPosition)
};
document.getElementById('left').onclick = function() {
processLeft(defaultPosition)
};
document.getElementById('right').onclick = function() {
processRight(defaultPosition)
};
document.getElementById('reset').onclick = function() {
processReset(defaultPosition)
};
// action functions
function processUp(positionX) {
let nowPosition = positionX;
if (nowPosition <= 10) {
alert('you cant step here');
} else {
let nextPosition = nowPosition - 10;
document.getElementById(nowPosition).setAttribute('class', 'eachGridBlock');
document.getElementById(nextPosition).setAttribute('class', 'focusedBlock');
positionX = nextPosition;
};
console.log(positionX);
return positionX;
};
function processDown(positionX) {
let nowPosition = positionX;
if (nowPosition >= 91) {
alert('you cant step here');
} else {
let nextPosition = nowPosition + 10;
document.getElementById(nowPosition).setAttribute('class', 'eachGridBlock');
document.getElementById(nextPosition).setAttribute('class', 'focusedBlock');
positionX = nextPosition;
};
console.log(positionX);
return positionX;
};
function processLeft(positionX) {
let nowPosition = positionX;
if (nowPosition === 1 || nowPosition === 11 || nowPosition === 21 || nowPosition === 31 || nowPosition === 41 || nowPosition === 51 || nowPosition === 61 || nowPosition === 71 || nowPosition === 81 || nowPosition === 91) {
alert('you cant step here');
} else {
let nextPosition = nowPosition - 1;
document.getElementById(nowPosition).setAttribute('class', 'eachGridBlock');
document.getElementById(nextPosition).setAttribute('class', 'focusedBlock');
positionX = nextPosition;
};
console.log(positionX);
return positionX;
};
function processRight(positionX) {
let nowPosition = positionX;
if (nowPosition === 10 || nowPosition === 20 || nowPosition === 30 || nowPosition === 40 || nowPosition === 50 || nowPosition === 60 || nowPosition === 70 || nowPosition === 80 || nowPosition === 90 || nowPosition === 100) {
alert('you cant step here');
} else {
let nextPosition = nowPosition + 1;
document.getElementById(nowPosition).setAttribute('class', 'eachGridBlock');
document.getElementById(nextPosition).setAttribute('class', 'focusedBlock');
positionX = nextPosition;
};
console.log(positionX);
return positionX;
};
function processReset(positionX) {
let nowPosition = positionX
let nextPosition = 1;
document.getElementById(nowPosition).setAttribute('class', 'eachGridBlock');
document.getElementById(nextPosition).setAttribute('class', 'focusedBlock');
return positionX;
};
};
* {
margin: 0;
padding: 0;
}
#plarform {
width: 500px;
height: 500px;
background-color: #222222;
}
.eachGridBlock {
margin: 0;
padding: 0;
width: 50px;
height: 50px;
background-color: #000044;
border: 0;
}
.focusedBlock {
margin: 0;
padding: 0;
width: 50px;
height: 50px;
background-color: #9900ff;
border: 0;
}
.actionButton {
border: 0;
width: 100px;
height: 35px;
background-color: #999999;
color: #222222;
outline: none;
}
.actionButton:hover {
background-color: #dddddd;
}
<html>
<head>
</head>
<body>
</body>
</html>
window.onload = function core() {
// creating elements
let platform = document.createElement('div');
platform.setAttribute('id', 'platform');
document.body.appendChild(platform);
let up = document.createElement('button');
up.setAttribute('id', 'up');
up.setAttribute('class', 'actionButton');
document.body.appendChild(up);
document.getElementById('up').innerHTML = 'up';
let down = document.createElement('button');
down.setAttribute('id', 'down');
down.setAttribute('class', 'actionButton');
document.body.appendChild(down);
document.getElementById('down').innerHTML = 'down';
let left = document.createElement('button');
left.setAttribute('id', 'left');
left.setAttribute('class', 'actionButton');
document.body.appendChild(left);
document.getElementById('left').innerHTML = 'left';
let right = document.createElement('button');
right.setAttribute('id', 'right');
right.setAttribute('class', 'actionButton');
document.body.appendChild(right);
document.getElementById('right').innerHTML = 'right';
let reset = document.createElement('button');
reset.setAttribute('id', 'reset');
reset.setAttribute('class', 'actionButton');
document.body.appendChild(reset);
document.getElementById('reset').innerHTML = 'reset';
// binding platform
let count = 0;
let defaultPosition = 1;
for (let i = 1; i < 101; i++) {
let grid = document.createElement('button');
grid.setAttribute('id', i);
grid.setAttribute('class', 'eachGridBlock');
document.getElementById('platform').appendChild(grid);
count++;
if (count === 10) {
let newLine = document.createElement('br');
document.getElementById('platform').appendChild(newLine);
count = 0;
};
};
// waiting for action
document.getElementById(defaultPosition).setAttribute('class', 'focusedBlock');
console.log(defaultPosition);
document.getElementById('up').onclick = function() {
processUp(defaultPosition)
};
document.getElementById('down').onclick = function() {
processDown(defaultPosition)
};
document.getElementById('left').onclick = function() {
processLeft(defaultPosition)
};
document.getElementById('right').onclick = function() {
processRight(defaultPosition)
};
document.getElementById('reset').onclick = function() {
processReset(defaultPosition)
};
// action functions
function processUp(positionX) {
let nowPosition = positionX;
if (nowPosition <= 10) {
alert('you cant step here');
} else {
let nextPosition = nowPosition - 10;
document.getElementById(nowPosition).setAttribute('class', 'eachGridBlock');
document.getElementById(nextPosition).setAttribute('class', 'focusedBlock');
positionX = nextPosition;
};
console.log(positionX);
return positionX;
};
function processDown(positionX) {
let nowPosition = positionX;
if (nowPosition >= 91) {
alert('you cant step here');
} else {
let nextPosition = nowPosition + 10;
document.getElementById(nowPosition).setAttribute('class', 'eachGridBlock');
document.getElementById(nextPosition).setAttribute('class', 'focusedBlock');
positionX = nextPosition;
};
console.log(positionX);
return positionX;
};
function processLeft(positionX) {
let nowPosition = positionX;
if (nowPosition === 1 || nowPosition === 11 || nowPosition === 21 || nowPosition === 31 || nowPosition === 41 || nowPosition === 51 || nowPosition === 61 || nowPosition === 71 || nowPosition === 81 || nowPosition === 91) {
alert('you cant step here');
} else {
let nextPosition = nowPosition - 1;
document.getElementById(nowPosition).setAttribute('class', 'eachGridBlock');
document.getElementById(nextPosition).setAttribute('class', 'focusedBlock');
positionX = nextPosition;
};
console.log(positionX);
return positionX;
};
function processRight(positionX) {
let nowPosition = positionX;
if (nowPosition === 10 || nowPosition === 20 || nowPosition === 30 || nowPosition === 40 || nowPosition === 50 || nowPosition === 60 || nowPosition === 70 || nowPosition === 80 || nowPosition === 90 || nowPosition === 100) {
alert('you cant step here');
} else {
let nextPosition = nowPosition + 1;
document.getElementById(nowPosition).setAttribute('class', 'eachGridBlock');
document.getElementById(nextPosition).setAttribute('class', 'focusedBlock');
positionX = nextPosition;
};
console.log(positionX);
return positionX;
};
function processReset(positionX) {
let nowPosition = positionX
let nextPosition = 1;
document.getElementById(nowPosition).setAttribute('class', 'eachGridBlock');
document.getElementById(nextPosition).setAttribute('class', 'focusedBlock');
return positionX;
};
};
* {
margin: 0;
padding: 0;
}
#plarform {
width: 500px;
height: 500px;
background-color: #222222;
}
.eachGridBlock {
margin: 0;
padding: 0;
width: 50px;
height: 50px;
background-color: #000044;
border: 0;
}
.focusedBlock {
margin: 0;
padding: 0;
width: 50px;
height: 50px;
background-color: #9900ff;
border: 0;
}
.actionButton {
border: 0;
width: 100px;
height: 35px;
background-color: #999999;
color: #222222;
outline: none;
}
.actionButton:hover {
background-color: #dddddd;
}
<html>
<head>
</head>
<body>
</body>
</html>
javascript html
javascript html
edited Nov 15 '18 at 1:02
Barmar
429k36250352
429k36250352
asked Nov 15 '18 at 0:31
Eugene ZolotuhinEugene Zolotuhin
32
32
1
I cannot think of more unreadable core. Perhaps the odd line break and comment?
– Ed Heal
Nov 15 '18 at 0:36
Please only add the bare essentials.
– Ed Heal
Nov 15 '18 at 0:37
Look at the code's comments. I think it's enough commenting. Just need to return position value and use it when some button clicked again.
– Eugene Zolotuhin
Nov 15 '18 at 0:38
Look at the //action functions
– Eugene Zolotuhin
Nov 15 '18 at 0:39
1. 4 comments stating the obvious. 2.A lot of code for us to decipher. 3. Where is the HTML. 4 Perhaps reproduce in jsfiddle et al.
– Ed Heal
Nov 15 '18 at 0:41
|
show 7 more comments
1
I cannot think of more unreadable core. Perhaps the odd line break and comment?
– Ed Heal
Nov 15 '18 at 0:36
Please only add the bare essentials.
– Ed Heal
Nov 15 '18 at 0:37
Look at the code's comments. I think it's enough commenting. Just need to return position value and use it when some button clicked again.
– Eugene Zolotuhin
Nov 15 '18 at 0:38
Look at the //action functions
– Eugene Zolotuhin
Nov 15 '18 at 0:39
1. 4 comments stating the obvious. 2.A lot of code for us to decipher. 3. Where is the HTML. 4 Perhaps reproduce in jsfiddle et al.
– Ed Heal
Nov 15 '18 at 0:41
1
1
I cannot think of more unreadable core. Perhaps the odd line break and comment?
– Ed Heal
Nov 15 '18 at 0:36
I cannot think of more unreadable core. Perhaps the odd line break and comment?
– Ed Heal
Nov 15 '18 at 0:36
Please only add the bare essentials.
– Ed Heal
Nov 15 '18 at 0:37
Please only add the bare essentials.
– Ed Heal
Nov 15 '18 at 0:37
Look at the code's comments. I think it's enough commenting. Just need to return position value and use it when some button clicked again.
– Eugene Zolotuhin
Nov 15 '18 at 0:38
Look at the code's comments. I think it's enough commenting. Just need to return position value and use it when some button clicked again.
– Eugene Zolotuhin
Nov 15 '18 at 0:38
Look at the //action functions
– Eugene Zolotuhin
Nov 15 '18 at 0:39
Look at the //action functions
– Eugene Zolotuhin
Nov 15 '18 at 0:39
1. 4 comments stating the obvious. 2.A lot of code for us to decipher. 3. Where is the HTML. 4 Perhaps reproduce in jsfiddle et al.
– Ed Heal
Nov 15 '18 at 0:41
1. 4 comments stating the obvious. 2.A lot of code for us to decipher. 3. Where is the HTML. 4 Perhaps reproduce in jsfiddle et al.
– Ed Heal
Nov 15 '18 at 0:41
|
show 7 more comments
1 Answer
1
active
oldest
votes
You need to update defaultPosition
when you call the functions, e.g.
document.getElementById('right').onclick = function() {
defaultPosition = processRight(defaultPosition)
};
Oh, I see. Just a second please, I'll try
– Eugene Zolotuhin
Nov 15 '18 at 1:08
Oh my, It works!! Thank you so much) I'll learn this problem and understand it!)
– Eugene Zolotuhin
Nov 15 '18 at 1:10
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53310802%2five-got-some-troubles-with-return%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You need to update defaultPosition
when you call the functions, e.g.
document.getElementById('right').onclick = function() {
defaultPosition = processRight(defaultPosition)
};
Oh, I see. Just a second please, I'll try
– Eugene Zolotuhin
Nov 15 '18 at 1:08
Oh my, It works!! Thank you so much) I'll learn this problem and understand it!)
– Eugene Zolotuhin
Nov 15 '18 at 1:10
add a comment |
You need to update defaultPosition
when you call the functions, e.g.
document.getElementById('right').onclick = function() {
defaultPosition = processRight(defaultPosition)
};
Oh, I see. Just a second please, I'll try
– Eugene Zolotuhin
Nov 15 '18 at 1:08
Oh my, It works!! Thank you so much) I'll learn this problem and understand it!)
– Eugene Zolotuhin
Nov 15 '18 at 1:10
add a comment |
You need to update defaultPosition
when you call the functions, e.g.
document.getElementById('right').onclick = function() {
defaultPosition = processRight(defaultPosition)
};
You need to update defaultPosition
when you call the functions, e.g.
document.getElementById('right').onclick = function() {
defaultPosition = processRight(defaultPosition)
};
answered Nov 15 '18 at 1:07
BarmarBarmar
429k36250352
429k36250352
Oh, I see. Just a second please, I'll try
– Eugene Zolotuhin
Nov 15 '18 at 1:08
Oh my, It works!! Thank you so much) I'll learn this problem and understand it!)
– Eugene Zolotuhin
Nov 15 '18 at 1:10
add a comment |
Oh, I see. Just a second please, I'll try
– Eugene Zolotuhin
Nov 15 '18 at 1:08
Oh my, It works!! Thank you so much) I'll learn this problem and understand it!)
– Eugene Zolotuhin
Nov 15 '18 at 1:10
Oh, I see. Just a second please, I'll try
– Eugene Zolotuhin
Nov 15 '18 at 1:08
Oh, I see. Just a second please, I'll try
– Eugene Zolotuhin
Nov 15 '18 at 1:08
Oh my, It works!! Thank you so much) I'll learn this problem and understand it!)
– Eugene Zolotuhin
Nov 15 '18 at 1:10
Oh my, It works!! Thank you so much) I'll learn this problem and understand it!)
– Eugene Zolotuhin
Nov 15 '18 at 1:10
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53310802%2five-got-some-troubles-with-return%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Mu09ffT
1
I cannot think of more unreadable core. Perhaps the odd line break and comment?
– Ed Heal
Nov 15 '18 at 0:36
Please only add the bare essentials.
– Ed Heal
Nov 15 '18 at 0:37
Look at the code's comments. I think it's enough commenting. Just need to return position value and use it when some button clicked again.
– Eugene Zolotuhin
Nov 15 '18 at 0:38
Look at the //action functions
– Eugene Zolotuhin
Nov 15 '18 at 0:39
1. 4 comments stating the obvious. 2.A lot of code for us to decipher. 3. Where is the HTML. 4 Perhaps reproduce in jsfiddle et al.
– Ed Heal
Nov 15 '18 at 0:41