Filter array and add a property
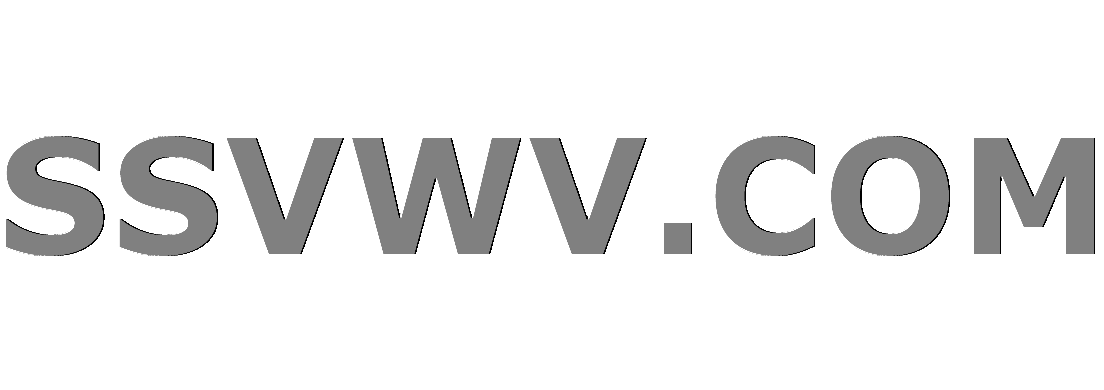
Multi tool use
up vote
1
down vote
favorite
I have an array where I have the weekly progress of some projects .
In the cuurent week, timestamp of 1529539200 or date 06/21/2018, I have the information of 3 projects. Project 20, 21 and 22.
In the other week, a week before the current 06/14/2018, there's only information of 2 projects. Because project 22 didn't exist.
progressList = [
// current 06/21/2018
{
project: 20,
timestamp: 1529539200, // seconds
current: true,
progress: 90
},
{
project: 21,
timestamp: 1529539200,
current: true,
progress: 70
},
{
project: 22,
timestamp: 1529539200,
current: true,
progress: 100
},
// 06/14/2018
{
project: 20,
timestamp: 1528934400, // a week before (1529539200 - 604800)
current: false,
progress: 80
},
{
project: 21,
timestamp: 1528934400,
current: false,
progress: 50
}
]
What I need is a list of current projects, but includes a new property "increase", that is equals to the difference between current project progress and the progress of the week before.
For example I should get something like this:
currentProgressList = [
{
project: 20,
timestamp: 1529539200,
current: true,
progress: 90,
increase: 10 // difference
},
{
project: 21,
timestamp: 1529539200,
current: true,
progress: 70,
increase: 20
},
{
project: 22,
timestamp: 1529539200,
current: true,
progress: 100,
increase: 0 //
}
]
I guess that filtering the projects by current
property may be simple:
listadoprogresss.filter(p => {
return p.current === true
})
But, I don't know how to add the increase
property according with my needs.
Thanks in advance
javascript
add a comment |
up vote
1
down vote
favorite
I have an array where I have the weekly progress of some projects .
In the cuurent week, timestamp of 1529539200 or date 06/21/2018, I have the information of 3 projects. Project 20, 21 and 22.
In the other week, a week before the current 06/14/2018, there's only information of 2 projects. Because project 22 didn't exist.
progressList = [
// current 06/21/2018
{
project: 20,
timestamp: 1529539200, // seconds
current: true,
progress: 90
},
{
project: 21,
timestamp: 1529539200,
current: true,
progress: 70
},
{
project: 22,
timestamp: 1529539200,
current: true,
progress: 100
},
// 06/14/2018
{
project: 20,
timestamp: 1528934400, // a week before (1529539200 - 604800)
current: false,
progress: 80
},
{
project: 21,
timestamp: 1528934400,
current: false,
progress: 50
}
]
What I need is a list of current projects, but includes a new property "increase", that is equals to the difference between current project progress and the progress of the week before.
For example I should get something like this:
currentProgressList = [
{
project: 20,
timestamp: 1529539200,
current: true,
progress: 90,
increase: 10 // difference
},
{
project: 21,
timestamp: 1529539200,
current: true,
progress: 70,
increase: 20
},
{
project: 22,
timestamp: 1529539200,
current: true,
progress: 100,
increase: 0 //
}
]
I guess that filtering the projects by current
property may be simple:
listadoprogresss.filter(p => {
return p.current === true
})
But, I don't know how to add the increase
property according with my needs.
Thanks in advance
javascript
You can simply use the Array map method to generate a new Array with the 'increase' property.
– Vishnu Vishwa
Nov 10 at 21:09
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I have an array where I have the weekly progress of some projects .
In the cuurent week, timestamp of 1529539200 or date 06/21/2018, I have the information of 3 projects. Project 20, 21 and 22.
In the other week, a week before the current 06/14/2018, there's only information of 2 projects. Because project 22 didn't exist.
progressList = [
// current 06/21/2018
{
project: 20,
timestamp: 1529539200, // seconds
current: true,
progress: 90
},
{
project: 21,
timestamp: 1529539200,
current: true,
progress: 70
},
{
project: 22,
timestamp: 1529539200,
current: true,
progress: 100
},
// 06/14/2018
{
project: 20,
timestamp: 1528934400, // a week before (1529539200 - 604800)
current: false,
progress: 80
},
{
project: 21,
timestamp: 1528934400,
current: false,
progress: 50
}
]
What I need is a list of current projects, but includes a new property "increase", that is equals to the difference between current project progress and the progress of the week before.
For example I should get something like this:
currentProgressList = [
{
project: 20,
timestamp: 1529539200,
current: true,
progress: 90,
increase: 10 // difference
},
{
project: 21,
timestamp: 1529539200,
current: true,
progress: 70,
increase: 20
},
{
project: 22,
timestamp: 1529539200,
current: true,
progress: 100,
increase: 0 //
}
]
I guess that filtering the projects by current
property may be simple:
listadoprogresss.filter(p => {
return p.current === true
})
But, I don't know how to add the increase
property according with my needs.
Thanks in advance
javascript
I have an array where I have the weekly progress of some projects .
In the cuurent week, timestamp of 1529539200 or date 06/21/2018, I have the information of 3 projects. Project 20, 21 and 22.
In the other week, a week before the current 06/14/2018, there's only information of 2 projects. Because project 22 didn't exist.
progressList = [
// current 06/21/2018
{
project: 20,
timestamp: 1529539200, // seconds
current: true,
progress: 90
},
{
project: 21,
timestamp: 1529539200,
current: true,
progress: 70
},
{
project: 22,
timestamp: 1529539200,
current: true,
progress: 100
},
// 06/14/2018
{
project: 20,
timestamp: 1528934400, // a week before (1529539200 - 604800)
current: false,
progress: 80
},
{
project: 21,
timestamp: 1528934400,
current: false,
progress: 50
}
]
What I need is a list of current projects, but includes a new property "increase", that is equals to the difference between current project progress and the progress of the week before.
For example I should get something like this:
currentProgressList = [
{
project: 20,
timestamp: 1529539200,
current: true,
progress: 90,
increase: 10 // difference
},
{
project: 21,
timestamp: 1529539200,
current: true,
progress: 70,
increase: 20
},
{
project: 22,
timestamp: 1529539200,
current: true,
progress: 100,
increase: 0 //
}
]
I guess that filtering the projects by current
property may be simple:
listadoprogresss.filter(p => {
return p.current === true
})
But, I don't know how to add the increase
property according with my needs.
Thanks in advance
javascript
javascript
asked Nov 10 at 20:52


Javier Cárdenas
6342920
6342920
You can simply use the Array map method to generate a new Array with the 'increase' property.
– Vishnu Vishwa
Nov 10 at 21:09
add a comment |
You can simply use the Array map method to generate a new Array with the 'increase' property.
– Vishnu Vishwa
Nov 10 at 21:09
You can simply use the Array map method to generate a new Array with the 'increase' property.
– Vishnu Vishwa
Nov 10 at 21:09
You can simply use the Array map method to generate a new Array with the 'increase' property.
– Vishnu Vishwa
Nov 10 at 21:09
add a comment |
2 Answers
2
active
oldest
votes
up vote
2
down vote
accepted
Here is a possible solution, you can adjust the checkIncrease to suit your solution. but run the code snippet
let checkIncrease = function(list, p) {
let prevProgress = list.find(np => np.project === p.project && !np.current)
var increase = 0;
// confirm that its defined
if (prevProgress) increase = p.progress - prevProgress.progress
// create a clone and return
return { project: p.project, timestamp: p.timestamp, current: p.current, progress: p.progress, increase: increase }
}
let getProgressIncrease = function(progressList) {
return progressList
.filter(p => p.current)
.map(p => checkIncrease(progressList, p))
}
var progressList = [
// current 06/21/2018
{
project: 20,
timestamp: 1529539200, // seconds
current: true,
progress: 90
},
{
project: 21,
timestamp: 1529539200,
current: true,
progress: 70
},
{
project: 22,
timestamp: 1529539200,
current: true,
progress: 100
},
// 06/14/2018
{
project: 20,
timestamp: 1528934400, // a week before (1529539200 - 604800)
current: false,
progress: 80
},
{
project: 21,
timestamp: 1528934400,
current: false,
progress: 50
}
]
// try it out
let result = getProgressIncrease(progressList)
console.log(result)
add a comment |
up vote
2
down vote
const progressList = [
{ project: 20, timestamp: 1529539200, current: true, progress: 90 },
{ project: 21, timestamp: 1529539200, current: true, progress: 70 },
{ project: 22, timestamp: 1529539200, current: true, progress: 100 },
{ project: 20, timestamp: 1528934400, current: false, progress: 80 },
{ project: 21, timestamp: 1528934400, current: false, progress: 50 }
]
function getCurrentProgressList(progressList) {
const projects = ;
const indexes = {};
for(const Project of progressList) {
const index = indexes[Project.project];
const CurrentProject = projects[index];
if (Project.current) {
indexes[Project.project] = projects.length;
projects.push({ ...Project, increase: 0 });
} else if (index !== undefined) {
const a = CurrentProject.progress;
const b = Project.progress;
CurrentProject['increase'] = a - b;
}
}
return projects;
}
console.log(getCurrentProgressList(progressList));
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
Here is a possible solution, you can adjust the checkIncrease to suit your solution. but run the code snippet
let checkIncrease = function(list, p) {
let prevProgress = list.find(np => np.project === p.project && !np.current)
var increase = 0;
// confirm that its defined
if (prevProgress) increase = p.progress - prevProgress.progress
// create a clone and return
return { project: p.project, timestamp: p.timestamp, current: p.current, progress: p.progress, increase: increase }
}
let getProgressIncrease = function(progressList) {
return progressList
.filter(p => p.current)
.map(p => checkIncrease(progressList, p))
}
var progressList = [
// current 06/21/2018
{
project: 20,
timestamp: 1529539200, // seconds
current: true,
progress: 90
},
{
project: 21,
timestamp: 1529539200,
current: true,
progress: 70
},
{
project: 22,
timestamp: 1529539200,
current: true,
progress: 100
},
// 06/14/2018
{
project: 20,
timestamp: 1528934400, // a week before (1529539200 - 604800)
current: false,
progress: 80
},
{
project: 21,
timestamp: 1528934400,
current: false,
progress: 50
}
]
// try it out
let result = getProgressIncrease(progressList)
console.log(result)
add a comment |
up vote
2
down vote
accepted
Here is a possible solution, you can adjust the checkIncrease to suit your solution. but run the code snippet
let checkIncrease = function(list, p) {
let prevProgress = list.find(np => np.project === p.project && !np.current)
var increase = 0;
// confirm that its defined
if (prevProgress) increase = p.progress - prevProgress.progress
// create a clone and return
return { project: p.project, timestamp: p.timestamp, current: p.current, progress: p.progress, increase: increase }
}
let getProgressIncrease = function(progressList) {
return progressList
.filter(p => p.current)
.map(p => checkIncrease(progressList, p))
}
var progressList = [
// current 06/21/2018
{
project: 20,
timestamp: 1529539200, // seconds
current: true,
progress: 90
},
{
project: 21,
timestamp: 1529539200,
current: true,
progress: 70
},
{
project: 22,
timestamp: 1529539200,
current: true,
progress: 100
},
// 06/14/2018
{
project: 20,
timestamp: 1528934400, // a week before (1529539200 - 604800)
current: false,
progress: 80
},
{
project: 21,
timestamp: 1528934400,
current: false,
progress: 50
}
]
// try it out
let result = getProgressIncrease(progressList)
console.log(result)
add a comment |
up vote
2
down vote
accepted
up vote
2
down vote
accepted
Here is a possible solution, you can adjust the checkIncrease to suit your solution. but run the code snippet
let checkIncrease = function(list, p) {
let prevProgress = list.find(np => np.project === p.project && !np.current)
var increase = 0;
// confirm that its defined
if (prevProgress) increase = p.progress - prevProgress.progress
// create a clone and return
return { project: p.project, timestamp: p.timestamp, current: p.current, progress: p.progress, increase: increase }
}
let getProgressIncrease = function(progressList) {
return progressList
.filter(p => p.current)
.map(p => checkIncrease(progressList, p))
}
var progressList = [
// current 06/21/2018
{
project: 20,
timestamp: 1529539200, // seconds
current: true,
progress: 90
},
{
project: 21,
timestamp: 1529539200,
current: true,
progress: 70
},
{
project: 22,
timestamp: 1529539200,
current: true,
progress: 100
},
// 06/14/2018
{
project: 20,
timestamp: 1528934400, // a week before (1529539200 - 604800)
current: false,
progress: 80
},
{
project: 21,
timestamp: 1528934400,
current: false,
progress: 50
}
]
// try it out
let result = getProgressIncrease(progressList)
console.log(result)
Here is a possible solution, you can adjust the checkIncrease to suit your solution. but run the code snippet
let checkIncrease = function(list, p) {
let prevProgress = list.find(np => np.project === p.project && !np.current)
var increase = 0;
// confirm that its defined
if (prevProgress) increase = p.progress - prevProgress.progress
// create a clone and return
return { project: p.project, timestamp: p.timestamp, current: p.current, progress: p.progress, increase: increase }
}
let getProgressIncrease = function(progressList) {
return progressList
.filter(p => p.current)
.map(p => checkIncrease(progressList, p))
}
var progressList = [
// current 06/21/2018
{
project: 20,
timestamp: 1529539200, // seconds
current: true,
progress: 90
},
{
project: 21,
timestamp: 1529539200,
current: true,
progress: 70
},
{
project: 22,
timestamp: 1529539200,
current: true,
progress: 100
},
// 06/14/2018
{
project: 20,
timestamp: 1528934400, // a week before (1529539200 - 604800)
current: false,
progress: 80
},
{
project: 21,
timestamp: 1528934400,
current: false,
progress: 50
}
]
// try it out
let result = getProgressIncrease(progressList)
console.log(result)
let checkIncrease = function(list, p) {
let prevProgress = list.find(np => np.project === p.project && !np.current)
var increase = 0;
// confirm that its defined
if (prevProgress) increase = p.progress - prevProgress.progress
// create a clone and return
return { project: p.project, timestamp: p.timestamp, current: p.current, progress: p.progress, increase: increase }
}
let getProgressIncrease = function(progressList) {
return progressList
.filter(p => p.current)
.map(p => checkIncrease(progressList, p))
}
var progressList = [
// current 06/21/2018
{
project: 20,
timestamp: 1529539200, // seconds
current: true,
progress: 90
},
{
project: 21,
timestamp: 1529539200,
current: true,
progress: 70
},
{
project: 22,
timestamp: 1529539200,
current: true,
progress: 100
},
// 06/14/2018
{
project: 20,
timestamp: 1528934400, // a week before (1529539200 - 604800)
current: false,
progress: 80
},
{
project: 21,
timestamp: 1528934400,
current: false,
progress: 50
}
]
// try it out
let result = getProgressIncrease(progressList)
console.log(result)
let checkIncrease = function(list, p) {
let prevProgress = list.find(np => np.project === p.project && !np.current)
var increase = 0;
// confirm that its defined
if (prevProgress) increase = p.progress - prevProgress.progress
// create a clone and return
return { project: p.project, timestamp: p.timestamp, current: p.current, progress: p.progress, increase: increase }
}
let getProgressIncrease = function(progressList) {
return progressList
.filter(p => p.current)
.map(p => checkIncrease(progressList, p))
}
var progressList = [
// current 06/21/2018
{
project: 20,
timestamp: 1529539200, // seconds
current: true,
progress: 90
},
{
project: 21,
timestamp: 1529539200,
current: true,
progress: 70
},
{
project: 22,
timestamp: 1529539200,
current: true,
progress: 100
},
// 06/14/2018
{
project: 20,
timestamp: 1528934400, // a week before (1529539200 - 604800)
current: false,
progress: 80
},
{
project: 21,
timestamp: 1528934400,
current: false,
progress: 50
}
]
// try it out
let result = getProgressIncrease(progressList)
console.log(result)
edited Nov 10 at 22:28
answered Nov 10 at 21:27


Ebi Igweze
22927
22927
add a comment |
add a comment |
up vote
2
down vote
const progressList = [
{ project: 20, timestamp: 1529539200, current: true, progress: 90 },
{ project: 21, timestamp: 1529539200, current: true, progress: 70 },
{ project: 22, timestamp: 1529539200, current: true, progress: 100 },
{ project: 20, timestamp: 1528934400, current: false, progress: 80 },
{ project: 21, timestamp: 1528934400, current: false, progress: 50 }
]
function getCurrentProgressList(progressList) {
const projects = ;
const indexes = {};
for(const Project of progressList) {
const index = indexes[Project.project];
const CurrentProject = projects[index];
if (Project.current) {
indexes[Project.project] = projects.length;
projects.push({ ...Project, increase: 0 });
} else if (index !== undefined) {
const a = CurrentProject.progress;
const b = Project.progress;
CurrentProject['increase'] = a - b;
}
}
return projects;
}
console.log(getCurrentProgressList(progressList));
add a comment |
up vote
2
down vote
const progressList = [
{ project: 20, timestamp: 1529539200, current: true, progress: 90 },
{ project: 21, timestamp: 1529539200, current: true, progress: 70 },
{ project: 22, timestamp: 1529539200, current: true, progress: 100 },
{ project: 20, timestamp: 1528934400, current: false, progress: 80 },
{ project: 21, timestamp: 1528934400, current: false, progress: 50 }
]
function getCurrentProgressList(progressList) {
const projects = ;
const indexes = {};
for(const Project of progressList) {
const index = indexes[Project.project];
const CurrentProject = projects[index];
if (Project.current) {
indexes[Project.project] = projects.length;
projects.push({ ...Project, increase: 0 });
} else if (index !== undefined) {
const a = CurrentProject.progress;
const b = Project.progress;
CurrentProject['increase'] = a - b;
}
}
return projects;
}
console.log(getCurrentProgressList(progressList));
add a comment |
up vote
2
down vote
up vote
2
down vote
const progressList = [
{ project: 20, timestamp: 1529539200, current: true, progress: 90 },
{ project: 21, timestamp: 1529539200, current: true, progress: 70 },
{ project: 22, timestamp: 1529539200, current: true, progress: 100 },
{ project: 20, timestamp: 1528934400, current: false, progress: 80 },
{ project: 21, timestamp: 1528934400, current: false, progress: 50 }
]
function getCurrentProgressList(progressList) {
const projects = ;
const indexes = {};
for(const Project of progressList) {
const index = indexes[Project.project];
const CurrentProject = projects[index];
if (Project.current) {
indexes[Project.project] = projects.length;
projects.push({ ...Project, increase: 0 });
} else if (index !== undefined) {
const a = CurrentProject.progress;
const b = Project.progress;
CurrentProject['increase'] = a - b;
}
}
return projects;
}
console.log(getCurrentProgressList(progressList));
const progressList = [
{ project: 20, timestamp: 1529539200, current: true, progress: 90 },
{ project: 21, timestamp: 1529539200, current: true, progress: 70 },
{ project: 22, timestamp: 1529539200, current: true, progress: 100 },
{ project: 20, timestamp: 1528934400, current: false, progress: 80 },
{ project: 21, timestamp: 1528934400, current: false, progress: 50 }
]
function getCurrentProgressList(progressList) {
const projects = ;
const indexes = {};
for(const Project of progressList) {
const index = indexes[Project.project];
const CurrentProject = projects[index];
if (Project.current) {
indexes[Project.project] = projects.length;
projects.push({ ...Project, increase: 0 });
} else if (index !== undefined) {
const a = CurrentProject.progress;
const b = Project.progress;
CurrentProject['increase'] = a - b;
}
}
return projects;
}
console.log(getCurrentProgressList(progressList));
const progressList = [
{ project: 20, timestamp: 1529539200, current: true, progress: 90 },
{ project: 21, timestamp: 1529539200, current: true, progress: 70 },
{ project: 22, timestamp: 1529539200, current: true, progress: 100 },
{ project: 20, timestamp: 1528934400, current: false, progress: 80 },
{ project: 21, timestamp: 1528934400, current: false, progress: 50 }
]
function getCurrentProgressList(progressList) {
const projects = ;
const indexes = {};
for(const Project of progressList) {
const index = indexes[Project.project];
const CurrentProject = projects[index];
if (Project.current) {
indexes[Project.project] = projects.length;
projects.push({ ...Project, increase: 0 });
} else if (index !== undefined) {
const a = CurrentProject.progress;
const b = Project.progress;
CurrentProject['increase'] = a - b;
}
}
return projects;
}
console.log(getCurrentProgressList(progressList));
const progressList = [
{ project: 20, timestamp: 1529539200, current: true, progress: 90 },
{ project: 21, timestamp: 1529539200, current: true, progress: 70 },
{ project: 22, timestamp: 1529539200, current: true, progress: 100 },
{ project: 20, timestamp: 1528934400, current: false, progress: 80 },
{ project: 21, timestamp: 1528934400, current: false, progress: 50 }
]
function getCurrentProgressList(progressList) {
const projects = ;
const indexes = {};
for(const Project of progressList) {
const index = indexes[Project.project];
const CurrentProject = projects[index];
if (Project.current) {
indexes[Project.project] = projects.length;
projects.push({ ...Project, increase: 0 });
} else if (index !== undefined) {
const a = CurrentProject.progress;
const b = Project.progress;
CurrentProject['increase'] = a - b;
}
}
return projects;
}
console.log(getCurrentProgressList(progressList));
edited Nov 10 at 21:51
answered Nov 10 at 21:24


Callam
7,70721522
7,70721522
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53243296%2ffilter-array-and-add-a-property%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
GioZuYIZFYR3CIHnSj5PxlST5CE,k8sg4Bp,63Jnt5jC89wH7UfWYSl,Pljva,5zTEqPewSzqBJXQHbckpMPCI,gq
You can simply use the Array map method to generate a new Array with the 'increase' property.
– Vishnu Vishwa
Nov 10 at 21:09