JavaFX 11 Replacement for column.impl_setWidth()?
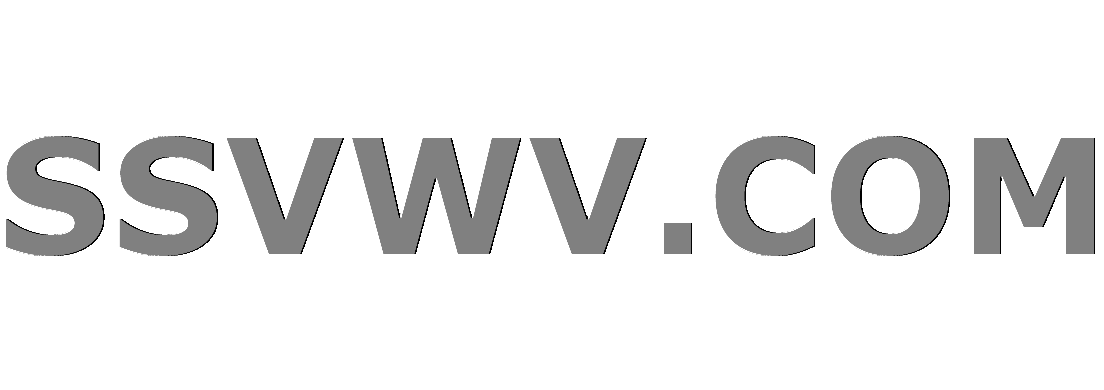
Multi tool use
I wrote a custom TableColumn width resize policy. You can see its code under Java
8 / JavaFX 8 here on github. As you can see, in the method distributeSpaceRatio()
and other places, it depends on TableColumn.impl_setWidth()
to set the width of the columns to the desired value after doing our calculations.
I am migrating my project to Java 11 / JavaFX 11 and the method impl_setWidth()
has been removed from public view.
Is there another method to tell a table column to set its width? I'm open to any solution as long as it is reliable and sets the with to (or close to) the requested value.
Here are two ideas I've tried so far that didn't work:
Attempt 1:
This one gives NoMethodFoundException:
private void setColumnWidth ( TableColumn column, double targetWidth ) {
try {
Method method = column.getClass().getDeclaredMethod( "setWidth" );
method.setAccessible( true );
Object r = method.invoke( targetWidth );
} catch ( Exception e ) {
e.printStackTrace();
}
}
Attempt 2:
This one has no effect:
private void setColumnWidth ( TableColumn column, double targetWidth ) {
double oldMax = column.getMaxWidth();
double oldMin = column.getMinWidth();
double oldPref = column.getPrefWidth();
column.setMaxWidth( targetWidth );
column.setMinWidth( targetWidth );
column.setPrefWidth( targetWidth );
column.getTableView().refresh();
column.setPrefWidth( oldPref );
column.setMinWidth( oldMin );
column.setMaxWidth( oldMax );
}
Attempt 3:
Looking at the source for JavaFX's TableView.UNCONSTRAINED_RESIZE_POLICY
, it seems the method invoked is ultimately TableColumnBase.doSetWidth()
, however that also doesn't work for me here:
private void setColumnWidth ( TableColumn column, double targetWidth ) {
try {
Method method = column.getClass().getDeclaredMethod( "doSetWidth" );
method.setAccessible( true );
Object r = method.invoke( targetWidth );
} catch ( Exception e ) {
e.printStackTrace();
}
}
Attempt 4:
I also tried using the solution provided in this answer to execute a private method, and got the same exception -- "There are no methods found with name doSetWidth and params [class java.lang.Double]".
Attempt 5:
I thought this one was going to work, but no such luck. It threw a "java.lang.NoSuchFieldException: width". However, when I go to the TableColumnBase source code, I clearly see on line 412 a private field called width. Not sure why this fails.
private void setColumnWidth ( TableColumnBase column, double targetWidth ) {
try {
Field widthField = column.getClass().getDeclaredField( "width" );
widthField.setAccessible( true );
ReadOnlyDoubleWrapper width = (ReadOnlyDoubleWrapper)widthField.get( column );
width.set( targetWidth );
} catch ( Exception e ) {
e.printStackTrace();
}
}
Attempt 6:
This got us closer, but still failed:
private void setColumnWidth ( TableColumnBase column, double targetWidth ) {
try {
Field widthField = column.getClass().getSuperclass().getDeclaredField( "width" );
widthField.setAccessible( true );
ReadOnlyDoubleWrapper width = (ReadOnlyDoubleWrapper)widthField.get( column );
width.set( targetWidth );
} catch ( Exception e ) {
e.printStackTrace();
}
}
java.lang.reflect.InaccessibleObjectException: Unable to make field private javafx.beans.property.ReadOnlyDoubleWrapper javafx.scene.control.TableColumnBase.width accessible: module javafx.controls does not "opens javafx.scene.control" to unnamed module @649dcffc
Attempt 7:
private void setColumnWidth ( TableColumn column, double targetWidth ) {
try {
Method method = column.getClass().getSuperclass().getDeclaredMethod( "setWidth", double.class );
method.setAccessible( true );
method.invoke ( targetWidth );
//ReadOnlyDoubleWrapper width = (ReadOnlyDoubleWrapper)widthField.get( column );
//width.set( targetWidth );
} catch ( Exception e ) {
e.printStackTrace();
}
}
java.lang.reflect.InaccessibleObjectException: Unable to make void javafx.scene.control.TableColumnBase.setWidth(double) accessible: module javafx.controls does not "opens javafx.scene.control" to unnamed module @5063f647
java javafx java-11 javafx-11
|
show 3 more comments
I wrote a custom TableColumn width resize policy. You can see its code under Java
8 / JavaFX 8 here on github. As you can see, in the method distributeSpaceRatio()
and other places, it depends on TableColumn.impl_setWidth()
to set the width of the columns to the desired value after doing our calculations.
I am migrating my project to Java 11 / JavaFX 11 and the method impl_setWidth()
has been removed from public view.
Is there another method to tell a table column to set its width? I'm open to any solution as long as it is reliable and sets the with to (or close to) the requested value.
Here are two ideas I've tried so far that didn't work:
Attempt 1:
This one gives NoMethodFoundException:
private void setColumnWidth ( TableColumn column, double targetWidth ) {
try {
Method method = column.getClass().getDeclaredMethod( "setWidth" );
method.setAccessible( true );
Object r = method.invoke( targetWidth );
} catch ( Exception e ) {
e.printStackTrace();
}
}
Attempt 2:
This one has no effect:
private void setColumnWidth ( TableColumn column, double targetWidth ) {
double oldMax = column.getMaxWidth();
double oldMin = column.getMinWidth();
double oldPref = column.getPrefWidth();
column.setMaxWidth( targetWidth );
column.setMinWidth( targetWidth );
column.setPrefWidth( targetWidth );
column.getTableView().refresh();
column.setPrefWidth( oldPref );
column.setMinWidth( oldMin );
column.setMaxWidth( oldMax );
}
Attempt 3:
Looking at the source for JavaFX's TableView.UNCONSTRAINED_RESIZE_POLICY
, it seems the method invoked is ultimately TableColumnBase.doSetWidth()
, however that also doesn't work for me here:
private void setColumnWidth ( TableColumn column, double targetWidth ) {
try {
Method method = column.getClass().getDeclaredMethod( "doSetWidth" );
method.setAccessible( true );
Object r = method.invoke( targetWidth );
} catch ( Exception e ) {
e.printStackTrace();
}
}
Attempt 4:
I also tried using the solution provided in this answer to execute a private method, and got the same exception -- "There are no methods found with name doSetWidth and params [class java.lang.Double]".
Attempt 5:
I thought this one was going to work, but no such luck. It threw a "java.lang.NoSuchFieldException: width". However, when I go to the TableColumnBase source code, I clearly see on line 412 a private field called width. Not sure why this fails.
private void setColumnWidth ( TableColumnBase column, double targetWidth ) {
try {
Field widthField = column.getClass().getDeclaredField( "width" );
widthField.setAccessible( true );
ReadOnlyDoubleWrapper width = (ReadOnlyDoubleWrapper)widthField.get( column );
width.set( targetWidth );
} catch ( Exception e ) {
e.printStackTrace();
}
}
Attempt 6:
This got us closer, but still failed:
private void setColumnWidth ( TableColumnBase column, double targetWidth ) {
try {
Field widthField = column.getClass().getSuperclass().getDeclaredField( "width" );
widthField.setAccessible( true );
ReadOnlyDoubleWrapper width = (ReadOnlyDoubleWrapper)widthField.get( column );
width.set( targetWidth );
} catch ( Exception e ) {
e.printStackTrace();
}
}
java.lang.reflect.InaccessibleObjectException: Unable to make field private javafx.beans.property.ReadOnlyDoubleWrapper javafx.scene.control.TableColumnBase.width accessible: module javafx.controls does not "opens javafx.scene.control" to unnamed module @649dcffc
Attempt 7:
private void setColumnWidth ( TableColumn column, double targetWidth ) {
try {
Method method = column.getClass().getSuperclass().getDeclaredMethod( "setWidth", double.class );
method.setAccessible( true );
method.invoke ( targetWidth );
//ReadOnlyDoubleWrapper width = (ReadOnlyDoubleWrapper)widthField.get( column );
//width.set( targetWidth );
} catch ( Exception e ) {
e.printStackTrace();
}
}
java.lang.reflect.InaccessibleObjectException: Unable to make void javafx.scene.control.TableColumnBase.setWidth(double) accessible: module javafx.controls does not "opens javafx.scene.control" to unnamed module @5063f647
java javafx java-11 javafx-11
1
I don't have the OpenJFX 11 source codes, but I think attempt 5 should be usingTableColumnBase.class.getDeclaredField("width")
. Runtime class of thecolumn
instance is probablyTableColumn
, which isn't going to match.
– Jai
Nov 12 at 4:31
Yea, Attempt 6 and 7 figure that out. But I run into that nasty "InaccessibleObjectException".
– JoshuaD
Nov 12 at 4:33
1
Attempts at reflection aren't going to work out of the box because of the module system. Since your code is, according to the error, in "unnamed module" you'll need to use--add-opens javafx.controls/javafx.scene.control=ALL-UNNAMED
in the command line.
– Slaw
Nov 12 at 4:34
That stopped the exceptions from firing, but the columns do not change size like they did when I called impl_setWidth().
– JoshuaD
Nov 12 at 4:40
CallTableColumnBase.doSetWidth(double)
reflectively instead. That is supposed to be the direct replacement ofimpl_setWidth(double)
, except that it's now package-private.
– Jai
Nov 12 at 4:57
|
show 3 more comments
I wrote a custom TableColumn width resize policy. You can see its code under Java
8 / JavaFX 8 here on github. As you can see, in the method distributeSpaceRatio()
and other places, it depends on TableColumn.impl_setWidth()
to set the width of the columns to the desired value after doing our calculations.
I am migrating my project to Java 11 / JavaFX 11 and the method impl_setWidth()
has been removed from public view.
Is there another method to tell a table column to set its width? I'm open to any solution as long as it is reliable and sets the with to (or close to) the requested value.
Here are two ideas I've tried so far that didn't work:
Attempt 1:
This one gives NoMethodFoundException:
private void setColumnWidth ( TableColumn column, double targetWidth ) {
try {
Method method = column.getClass().getDeclaredMethod( "setWidth" );
method.setAccessible( true );
Object r = method.invoke( targetWidth );
} catch ( Exception e ) {
e.printStackTrace();
}
}
Attempt 2:
This one has no effect:
private void setColumnWidth ( TableColumn column, double targetWidth ) {
double oldMax = column.getMaxWidth();
double oldMin = column.getMinWidth();
double oldPref = column.getPrefWidth();
column.setMaxWidth( targetWidth );
column.setMinWidth( targetWidth );
column.setPrefWidth( targetWidth );
column.getTableView().refresh();
column.setPrefWidth( oldPref );
column.setMinWidth( oldMin );
column.setMaxWidth( oldMax );
}
Attempt 3:
Looking at the source for JavaFX's TableView.UNCONSTRAINED_RESIZE_POLICY
, it seems the method invoked is ultimately TableColumnBase.doSetWidth()
, however that also doesn't work for me here:
private void setColumnWidth ( TableColumn column, double targetWidth ) {
try {
Method method = column.getClass().getDeclaredMethod( "doSetWidth" );
method.setAccessible( true );
Object r = method.invoke( targetWidth );
} catch ( Exception e ) {
e.printStackTrace();
}
}
Attempt 4:
I also tried using the solution provided in this answer to execute a private method, and got the same exception -- "There are no methods found with name doSetWidth and params [class java.lang.Double]".
Attempt 5:
I thought this one was going to work, but no such luck. It threw a "java.lang.NoSuchFieldException: width". However, when I go to the TableColumnBase source code, I clearly see on line 412 a private field called width. Not sure why this fails.
private void setColumnWidth ( TableColumnBase column, double targetWidth ) {
try {
Field widthField = column.getClass().getDeclaredField( "width" );
widthField.setAccessible( true );
ReadOnlyDoubleWrapper width = (ReadOnlyDoubleWrapper)widthField.get( column );
width.set( targetWidth );
} catch ( Exception e ) {
e.printStackTrace();
}
}
Attempt 6:
This got us closer, but still failed:
private void setColumnWidth ( TableColumnBase column, double targetWidth ) {
try {
Field widthField = column.getClass().getSuperclass().getDeclaredField( "width" );
widthField.setAccessible( true );
ReadOnlyDoubleWrapper width = (ReadOnlyDoubleWrapper)widthField.get( column );
width.set( targetWidth );
} catch ( Exception e ) {
e.printStackTrace();
}
}
java.lang.reflect.InaccessibleObjectException: Unable to make field private javafx.beans.property.ReadOnlyDoubleWrapper javafx.scene.control.TableColumnBase.width accessible: module javafx.controls does not "opens javafx.scene.control" to unnamed module @649dcffc
Attempt 7:
private void setColumnWidth ( TableColumn column, double targetWidth ) {
try {
Method method = column.getClass().getSuperclass().getDeclaredMethod( "setWidth", double.class );
method.setAccessible( true );
method.invoke ( targetWidth );
//ReadOnlyDoubleWrapper width = (ReadOnlyDoubleWrapper)widthField.get( column );
//width.set( targetWidth );
} catch ( Exception e ) {
e.printStackTrace();
}
}
java.lang.reflect.InaccessibleObjectException: Unable to make void javafx.scene.control.TableColumnBase.setWidth(double) accessible: module javafx.controls does not "opens javafx.scene.control" to unnamed module @5063f647
java javafx java-11 javafx-11
I wrote a custom TableColumn width resize policy. You can see its code under Java
8 / JavaFX 8 here on github. As you can see, in the method distributeSpaceRatio()
and other places, it depends on TableColumn.impl_setWidth()
to set the width of the columns to the desired value after doing our calculations.
I am migrating my project to Java 11 / JavaFX 11 and the method impl_setWidth()
has been removed from public view.
Is there another method to tell a table column to set its width? I'm open to any solution as long as it is reliable and sets the with to (or close to) the requested value.
Here are two ideas I've tried so far that didn't work:
Attempt 1:
This one gives NoMethodFoundException:
private void setColumnWidth ( TableColumn column, double targetWidth ) {
try {
Method method = column.getClass().getDeclaredMethod( "setWidth" );
method.setAccessible( true );
Object r = method.invoke( targetWidth );
} catch ( Exception e ) {
e.printStackTrace();
}
}
Attempt 2:
This one has no effect:
private void setColumnWidth ( TableColumn column, double targetWidth ) {
double oldMax = column.getMaxWidth();
double oldMin = column.getMinWidth();
double oldPref = column.getPrefWidth();
column.setMaxWidth( targetWidth );
column.setMinWidth( targetWidth );
column.setPrefWidth( targetWidth );
column.getTableView().refresh();
column.setPrefWidth( oldPref );
column.setMinWidth( oldMin );
column.setMaxWidth( oldMax );
}
Attempt 3:
Looking at the source for JavaFX's TableView.UNCONSTRAINED_RESIZE_POLICY
, it seems the method invoked is ultimately TableColumnBase.doSetWidth()
, however that also doesn't work for me here:
private void setColumnWidth ( TableColumn column, double targetWidth ) {
try {
Method method = column.getClass().getDeclaredMethod( "doSetWidth" );
method.setAccessible( true );
Object r = method.invoke( targetWidth );
} catch ( Exception e ) {
e.printStackTrace();
}
}
Attempt 4:
I also tried using the solution provided in this answer to execute a private method, and got the same exception -- "There are no methods found with name doSetWidth and params [class java.lang.Double]".
Attempt 5:
I thought this one was going to work, but no such luck. It threw a "java.lang.NoSuchFieldException: width". However, when I go to the TableColumnBase source code, I clearly see on line 412 a private field called width. Not sure why this fails.
private void setColumnWidth ( TableColumnBase column, double targetWidth ) {
try {
Field widthField = column.getClass().getDeclaredField( "width" );
widthField.setAccessible( true );
ReadOnlyDoubleWrapper width = (ReadOnlyDoubleWrapper)widthField.get( column );
width.set( targetWidth );
} catch ( Exception e ) {
e.printStackTrace();
}
}
Attempt 6:
This got us closer, but still failed:
private void setColumnWidth ( TableColumnBase column, double targetWidth ) {
try {
Field widthField = column.getClass().getSuperclass().getDeclaredField( "width" );
widthField.setAccessible( true );
ReadOnlyDoubleWrapper width = (ReadOnlyDoubleWrapper)widthField.get( column );
width.set( targetWidth );
} catch ( Exception e ) {
e.printStackTrace();
}
}
java.lang.reflect.InaccessibleObjectException: Unable to make field private javafx.beans.property.ReadOnlyDoubleWrapper javafx.scene.control.TableColumnBase.width accessible: module javafx.controls does not "opens javafx.scene.control" to unnamed module @649dcffc
Attempt 7:
private void setColumnWidth ( TableColumn column, double targetWidth ) {
try {
Method method = column.getClass().getSuperclass().getDeclaredMethod( "setWidth", double.class );
method.setAccessible( true );
method.invoke ( targetWidth );
//ReadOnlyDoubleWrapper width = (ReadOnlyDoubleWrapper)widthField.get( column );
//width.set( targetWidth );
} catch ( Exception e ) {
e.printStackTrace();
}
}
java.lang.reflect.InaccessibleObjectException: Unable to make void javafx.scene.control.TableColumnBase.setWidth(double) accessible: module javafx.controls does not "opens javafx.scene.control" to unnamed module @5063f647
java javafx java-11 javafx-11
java javafx java-11 javafx-11
edited Nov 12 at 4:33
asked Nov 12 at 3:04


JoshuaD
1,19031735
1,19031735
1
I don't have the OpenJFX 11 source codes, but I think attempt 5 should be usingTableColumnBase.class.getDeclaredField("width")
. Runtime class of thecolumn
instance is probablyTableColumn
, which isn't going to match.
– Jai
Nov 12 at 4:31
Yea, Attempt 6 and 7 figure that out. But I run into that nasty "InaccessibleObjectException".
– JoshuaD
Nov 12 at 4:33
1
Attempts at reflection aren't going to work out of the box because of the module system. Since your code is, according to the error, in "unnamed module" you'll need to use--add-opens javafx.controls/javafx.scene.control=ALL-UNNAMED
in the command line.
– Slaw
Nov 12 at 4:34
That stopped the exceptions from firing, but the columns do not change size like they did when I called impl_setWidth().
– JoshuaD
Nov 12 at 4:40
CallTableColumnBase.doSetWidth(double)
reflectively instead. That is supposed to be the direct replacement ofimpl_setWidth(double)
, except that it's now package-private.
– Jai
Nov 12 at 4:57
|
show 3 more comments
1
I don't have the OpenJFX 11 source codes, but I think attempt 5 should be usingTableColumnBase.class.getDeclaredField("width")
. Runtime class of thecolumn
instance is probablyTableColumn
, which isn't going to match.
– Jai
Nov 12 at 4:31
Yea, Attempt 6 and 7 figure that out. But I run into that nasty "InaccessibleObjectException".
– JoshuaD
Nov 12 at 4:33
1
Attempts at reflection aren't going to work out of the box because of the module system. Since your code is, according to the error, in "unnamed module" you'll need to use--add-opens javafx.controls/javafx.scene.control=ALL-UNNAMED
in the command line.
– Slaw
Nov 12 at 4:34
That stopped the exceptions from firing, but the columns do not change size like they did when I called impl_setWidth().
– JoshuaD
Nov 12 at 4:40
CallTableColumnBase.doSetWidth(double)
reflectively instead. That is supposed to be the direct replacement ofimpl_setWidth(double)
, except that it's now package-private.
– Jai
Nov 12 at 4:57
1
1
I don't have the OpenJFX 11 source codes, but I think attempt 5 should be using
TableColumnBase.class.getDeclaredField("width")
. Runtime class of the column
instance is probably TableColumn
, which isn't going to match.– Jai
Nov 12 at 4:31
I don't have the OpenJFX 11 source codes, but I think attempt 5 should be using
TableColumnBase.class.getDeclaredField("width")
. Runtime class of the column
instance is probably TableColumn
, which isn't going to match.– Jai
Nov 12 at 4:31
Yea, Attempt 6 and 7 figure that out. But I run into that nasty "InaccessibleObjectException".
– JoshuaD
Nov 12 at 4:33
Yea, Attempt 6 and 7 figure that out. But I run into that nasty "InaccessibleObjectException".
– JoshuaD
Nov 12 at 4:33
1
1
Attempts at reflection aren't going to work out of the box because of the module system. Since your code is, according to the error, in "unnamed module" you'll need to use
--add-opens javafx.controls/javafx.scene.control=ALL-UNNAMED
in the command line.– Slaw
Nov 12 at 4:34
Attempts at reflection aren't going to work out of the box because of the module system. Since your code is, according to the error, in "unnamed module" you'll need to use
--add-opens javafx.controls/javafx.scene.control=ALL-UNNAMED
in the command line.– Slaw
Nov 12 at 4:34
That stopped the exceptions from firing, but the columns do not change size like they did when I called impl_setWidth().
– JoshuaD
Nov 12 at 4:40
That stopped the exceptions from firing, but the columns do not change size like they did when I called impl_setWidth().
– JoshuaD
Nov 12 at 4:40
Call
TableColumnBase.doSetWidth(double)
reflectively instead. That is supposed to be the direct replacement of impl_setWidth(double)
, except that it's now package-private.– Jai
Nov 12 at 4:57
Call
TableColumnBase.doSetWidth(double)
reflectively instead. That is supposed to be the direct replacement of impl_setWidth(double)
, except that it's now package-private.– Jai
Nov 12 at 4:57
|
show 3 more comments
1 Answer
1
active
oldest
votes
Based on testing and the help of others, I found this solution:
private void setColumnWidth ( TableColumn column, double targetWidth ) {
try {
Method method = TableColumnBase.class.getDeclaredMethod( "doSetWidth", double.class );
method.setAccessible( true );
method.invoke( column, targetWidth );
} catch ( NoSuchMethodException | InvocationTargetException | IllegalAccessException e ) {
e.printStackTrace();
}
}
In addition, the JVM needs to be invoked with the following arguments:
--add-opens javafx.controls/javafx.scene.control=ALL-UNNAMED
1
By the way, if you ever put your code in a module replaceALL-UNNAMED
with your module name.
– Slaw
Nov 12 at 5:23
1
Just want to point out that it is safer to useTableColumnBase.class
, since you'd never know when someone extends fromTableColumn
.
– Jai
Nov 12 at 5:23
1
Also note thatcom.sun.javafx.scene.control.TableColumnBaseHelper.setWidth(TableColumnBase, double)
might work without using reflections, though you still have to use private API. Update: It looks like it was designed for this purpose. Found this by accident though.
– Jai
Nov 12 at 5:27
How would I use that private API without reflection?
– JoshuaD
Nov 12 at 5:32
1
Well, stuff incom.sun....
are considered private API. It should generate a warning which you would need to suppress at compile-time. I'm still using Java8/JavaFX8 so I'm not sure if this is still the case for OpenJFX11.
– Jai
Nov 12 at 5:33
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53255467%2fjavafx-11-replacement-for-column-impl-setwidth%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Based on testing and the help of others, I found this solution:
private void setColumnWidth ( TableColumn column, double targetWidth ) {
try {
Method method = TableColumnBase.class.getDeclaredMethod( "doSetWidth", double.class );
method.setAccessible( true );
method.invoke( column, targetWidth );
} catch ( NoSuchMethodException | InvocationTargetException | IllegalAccessException e ) {
e.printStackTrace();
}
}
In addition, the JVM needs to be invoked with the following arguments:
--add-opens javafx.controls/javafx.scene.control=ALL-UNNAMED
1
By the way, if you ever put your code in a module replaceALL-UNNAMED
with your module name.
– Slaw
Nov 12 at 5:23
1
Just want to point out that it is safer to useTableColumnBase.class
, since you'd never know when someone extends fromTableColumn
.
– Jai
Nov 12 at 5:23
1
Also note thatcom.sun.javafx.scene.control.TableColumnBaseHelper.setWidth(TableColumnBase, double)
might work without using reflections, though you still have to use private API. Update: It looks like it was designed for this purpose. Found this by accident though.
– Jai
Nov 12 at 5:27
How would I use that private API without reflection?
– JoshuaD
Nov 12 at 5:32
1
Well, stuff incom.sun....
are considered private API. It should generate a warning which you would need to suppress at compile-time. I'm still using Java8/JavaFX8 so I'm not sure if this is still the case for OpenJFX11.
– Jai
Nov 12 at 5:33
add a comment |
Based on testing and the help of others, I found this solution:
private void setColumnWidth ( TableColumn column, double targetWidth ) {
try {
Method method = TableColumnBase.class.getDeclaredMethod( "doSetWidth", double.class );
method.setAccessible( true );
method.invoke( column, targetWidth );
} catch ( NoSuchMethodException | InvocationTargetException | IllegalAccessException e ) {
e.printStackTrace();
}
}
In addition, the JVM needs to be invoked with the following arguments:
--add-opens javafx.controls/javafx.scene.control=ALL-UNNAMED
1
By the way, if you ever put your code in a module replaceALL-UNNAMED
with your module name.
– Slaw
Nov 12 at 5:23
1
Just want to point out that it is safer to useTableColumnBase.class
, since you'd never know when someone extends fromTableColumn
.
– Jai
Nov 12 at 5:23
1
Also note thatcom.sun.javafx.scene.control.TableColumnBaseHelper.setWidth(TableColumnBase, double)
might work without using reflections, though you still have to use private API. Update: It looks like it was designed for this purpose. Found this by accident though.
– Jai
Nov 12 at 5:27
How would I use that private API without reflection?
– JoshuaD
Nov 12 at 5:32
1
Well, stuff incom.sun....
are considered private API. It should generate a warning which you would need to suppress at compile-time. I'm still using Java8/JavaFX8 so I'm not sure if this is still the case for OpenJFX11.
– Jai
Nov 12 at 5:33
add a comment |
Based on testing and the help of others, I found this solution:
private void setColumnWidth ( TableColumn column, double targetWidth ) {
try {
Method method = TableColumnBase.class.getDeclaredMethod( "doSetWidth", double.class );
method.setAccessible( true );
method.invoke( column, targetWidth );
} catch ( NoSuchMethodException | InvocationTargetException | IllegalAccessException e ) {
e.printStackTrace();
}
}
In addition, the JVM needs to be invoked with the following arguments:
--add-opens javafx.controls/javafx.scene.control=ALL-UNNAMED
Based on testing and the help of others, I found this solution:
private void setColumnWidth ( TableColumn column, double targetWidth ) {
try {
Method method = TableColumnBase.class.getDeclaredMethod( "doSetWidth", double.class );
method.setAccessible( true );
method.invoke( column, targetWidth );
} catch ( NoSuchMethodException | InvocationTargetException | IllegalAccessException e ) {
e.printStackTrace();
}
}
In addition, the JVM needs to be invoked with the following arguments:
--add-opens javafx.controls/javafx.scene.control=ALL-UNNAMED
edited Nov 12 at 5:40
answered Nov 12 at 5:10


JoshuaD
1,19031735
1,19031735
1
By the way, if you ever put your code in a module replaceALL-UNNAMED
with your module name.
– Slaw
Nov 12 at 5:23
1
Just want to point out that it is safer to useTableColumnBase.class
, since you'd never know when someone extends fromTableColumn
.
– Jai
Nov 12 at 5:23
1
Also note thatcom.sun.javafx.scene.control.TableColumnBaseHelper.setWidth(TableColumnBase, double)
might work without using reflections, though you still have to use private API. Update: It looks like it was designed for this purpose. Found this by accident though.
– Jai
Nov 12 at 5:27
How would I use that private API without reflection?
– JoshuaD
Nov 12 at 5:32
1
Well, stuff incom.sun....
are considered private API. It should generate a warning which you would need to suppress at compile-time. I'm still using Java8/JavaFX8 so I'm not sure if this is still the case for OpenJFX11.
– Jai
Nov 12 at 5:33
add a comment |
1
By the way, if you ever put your code in a module replaceALL-UNNAMED
with your module name.
– Slaw
Nov 12 at 5:23
1
Just want to point out that it is safer to useTableColumnBase.class
, since you'd never know when someone extends fromTableColumn
.
– Jai
Nov 12 at 5:23
1
Also note thatcom.sun.javafx.scene.control.TableColumnBaseHelper.setWidth(TableColumnBase, double)
might work without using reflections, though you still have to use private API. Update: It looks like it was designed for this purpose. Found this by accident though.
– Jai
Nov 12 at 5:27
How would I use that private API without reflection?
– JoshuaD
Nov 12 at 5:32
1
Well, stuff incom.sun....
are considered private API. It should generate a warning which you would need to suppress at compile-time. I'm still using Java8/JavaFX8 so I'm not sure if this is still the case for OpenJFX11.
– Jai
Nov 12 at 5:33
1
1
By the way, if you ever put your code in a module replace
ALL-UNNAMED
with your module name.– Slaw
Nov 12 at 5:23
By the way, if you ever put your code in a module replace
ALL-UNNAMED
with your module name.– Slaw
Nov 12 at 5:23
1
1
Just want to point out that it is safer to use
TableColumnBase.class
, since you'd never know when someone extends from TableColumn
.– Jai
Nov 12 at 5:23
Just want to point out that it is safer to use
TableColumnBase.class
, since you'd never know when someone extends from TableColumn
.– Jai
Nov 12 at 5:23
1
1
Also note that
com.sun.javafx.scene.control.TableColumnBaseHelper.setWidth(TableColumnBase, double)
might work without using reflections, though you still have to use private API. Update: It looks like it was designed for this purpose. Found this by accident though.– Jai
Nov 12 at 5:27
Also note that
com.sun.javafx.scene.control.TableColumnBaseHelper.setWidth(TableColumnBase, double)
might work without using reflections, though you still have to use private API. Update: It looks like it was designed for this purpose. Found this by accident though.– Jai
Nov 12 at 5:27
How would I use that private API without reflection?
– JoshuaD
Nov 12 at 5:32
How would I use that private API without reflection?
– JoshuaD
Nov 12 at 5:32
1
1
Well, stuff in
com.sun....
are considered private API. It should generate a warning which you would need to suppress at compile-time. I'm still using Java8/JavaFX8 so I'm not sure if this is still the case for OpenJFX11.– Jai
Nov 12 at 5:33
Well, stuff in
com.sun....
are considered private API. It should generate a warning which you would need to suppress at compile-time. I'm still using Java8/JavaFX8 so I'm not sure if this is still the case for OpenJFX11.– Jai
Nov 12 at 5:33
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53255467%2fjavafx-11-replacement-for-column-impl-setwidth%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
QXPbsmXFICdKSh0EpLS OgLC1K4DVWTs0sIl
1
I don't have the OpenJFX 11 source codes, but I think attempt 5 should be using
TableColumnBase.class.getDeclaredField("width")
. Runtime class of thecolumn
instance is probablyTableColumn
, which isn't going to match.– Jai
Nov 12 at 4:31
Yea, Attempt 6 and 7 figure that out. But I run into that nasty "InaccessibleObjectException".
– JoshuaD
Nov 12 at 4:33
1
Attempts at reflection aren't going to work out of the box because of the module system. Since your code is, according to the error, in "unnamed module" you'll need to use
--add-opens javafx.controls/javafx.scene.control=ALL-UNNAMED
in the command line.– Slaw
Nov 12 at 4:34
That stopped the exceptions from firing, but the columns do not change size like they did when I called impl_setWidth().
– JoshuaD
Nov 12 at 4:40
Call
TableColumnBase.doSetWidth(double)
reflectively instead. That is supposed to be the direct replacement ofimpl_setWidth(double)
, except that it's now package-private.– Jai
Nov 12 at 4:57