Django Serilizer: Getting an ordered dictionary in embeded serializer
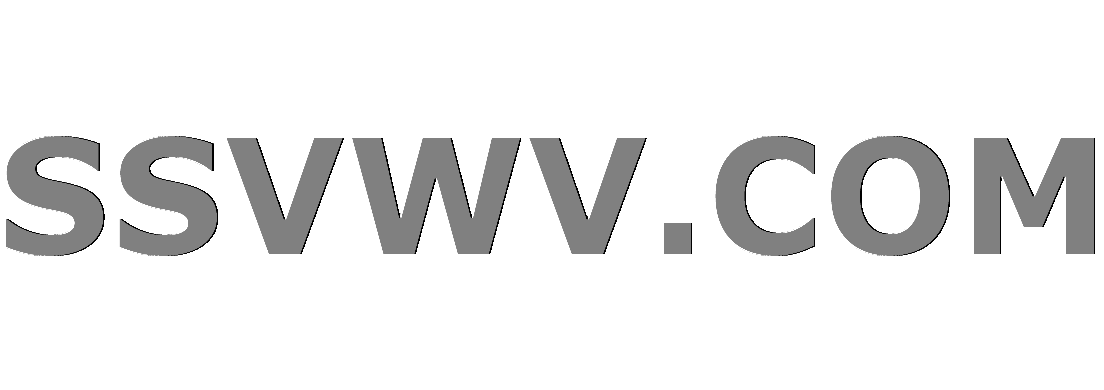
Multi tool use
up vote
1
down vote
favorite
I'm one serializer inside another to show information from one model, and selected fields from another in the same response.
This looks like this:
class SimpleRouteClientConstraintSerializer(serializers.ModelSerializer):
name = serializers.SerializerMethodField()
def get_name(self, obj):
default_constraint = RouteDefaultConstraint.objects.get(pk=obj.route_default_constraint_id)
return default_constraint.name
class Meta:
model = RouteClientConstraint
fields = ('name', 'value')
class RouteSerializer(serializers.ModelSerializer):
...
# Calling first serializer here
routeClientConstraints = SimpleRouteClientConstraintSerializer(many=True, required=False)
...
class Meta:
model = Route
fields = (..., 'routeClientConstraints', ...)
depth = 1
When running the RouteSerilalizer
, i want a response:
'routeClientConstraints': [{
'name': 'my name'
'value': 'confused'
},
...
]
If i use the serializer as in the code above, I get
'routeClientConstraints': [
OrderedDict([
('name', 'Minimum life boats'),
('value', '6')
])
]
I tried changing the serializer to: routeClientConstraints = SimpleRouteClientConstraintSerializer(many=True, required=False).data
, which i expected would give me json format, but i got this:
'routeClientConstraints': [
OrderedDict([
('id', 1),
('value', '6'),
('created_at', '2018-11-10T14:17:39.263848Z'),
('updated_at', '2018-11-10T14:17:39.263861Z'),
('route_default_constraint', 1),
('route', 1)])
]
How do i return a list of dictionaries?
python django django-serializer
add a comment |
up vote
1
down vote
favorite
I'm one serializer inside another to show information from one model, and selected fields from another in the same response.
This looks like this:
class SimpleRouteClientConstraintSerializer(serializers.ModelSerializer):
name = serializers.SerializerMethodField()
def get_name(self, obj):
default_constraint = RouteDefaultConstraint.objects.get(pk=obj.route_default_constraint_id)
return default_constraint.name
class Meta:
model = RouteClientConstraint
fields = ('name', 'value')
class RouteSerializer(serializers.ModelSerializer):
...
# Calling first serializer here
routeClientConstraints = SimpleRouteClientConstraintSerializer(many=True, required=False)
...
class Meta:
model = Route
fields = (..., 'routeClientConstraints', ...)
depth = 1
When running the RouteSerilalizer
, i want a response:
'routeClientConstraints': [{
'name': 'my name'
'value': 'confused'
},
...
]
If i use the serializer as in the code above, I get
'routeClientConstraints': [
OrderedDict([
('name', 'Minimum life boats'),
('value', '6')
])
]
I tried changing the serializer to: routeClientConstraints = SimpleRouteClientConstraintSerializer(many=True, required=False).data
, which i expected would give me json format, but i got this:
'routeClientConstraints': [
OrderedDict([
('id', 1),
('value', '6'),
('created_at', '2018-11-10T14:17:39.263848Z'),
('updated_at', '2018-11-10T14:17:39.263861Z'),
('route_default_constraint', 1),
('route', 1)])
]
How do i return a list of dictionaries?
python django django-serializer
AnOrderedDict
is a dictionary. When it is converted to JSON it will do the right thing.
– Will Keeling
Nov 10 at 21:40
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I'm one serializer inside another to show information from one model, and selected fields from another in the same response.
This looks like this:
class SimpleRouteClientConstraintSerializer(serializers.ModelSerializer):
name = serializers.SerializerMethodField()
def get_name(self, obj):
default_constraint = RouteDefaultConstraint.objects.get(pk=obj.route_default_constraint_id)
return default_constraint.name
class Meta:
model = RouteClientConstraint
fields = ('name', 'value')
class RouteSerializer(serializers.ModelSerializer):
...
# Calling first serializer here
routeClientConstraints = SimpleRouteClientConstraintSerializer(many=True, required=False)
...
class Meta:
model = Route
fields = (..., 'routeClientConstraints', ...)
depth = 1
When running the RouteSerilalizer
, i want a response:
'routeClientConstraints': [{
'name': 'my name'
'value': 'confused'
},
...
]
If i use the serializer as in the code above, I get
'routeClientConstraints': [
OrderedDict([
('name', 'Minimum life boats'),
('value', '6')
])
]
I tried changing the serializer to: routeClientConstraints = SimpleRouteClientConstraintSerializer(many=True, required=False).data
, which i expected would give me json format, but i got this:
'routeClientConstraints': [
OrderedDict([
('id', 1),
('value', '6'),
('created_at', '2018-11-10T14:17:39.263848Z'),
('updated_at', '2018-11-10T14:17:39.263861Z'),
('route_default_constraint', 1),
('route', 1)])
]
How do i return a list of dictionaries?
python django django-serializer
I'm one serializer inside another to show information from one model, and selected fields from another in the same response.
This looks like this:
class SimpleRouteClientConstraintSerializer(serializers.ModelSerializer):
name = serializers.SerializerMethodField()
def get_name(self, obj):
default_constraint = RouteDefaultConstraint.objects.get(pk=obj.route_default_constraint_id)
return default_constraint.name
class Meta:
model = RouteClientConstraint
fields = ('name', 'value')
class RouteSerializer(serializers.ModelSerializer):
...
# Calling first serializer here
routeClientConstraints = SimpleRouteClientConstraintSerializer(many=True, required=False)
...
class Meta:
model = Route
fields = (..., 'routeClientConstraints', ...)
depth = 1
When running the RouteSerilalizer
, i want a response:
'routeClientConstraints': [{
'name': 'my name'
'value': 'confused'
},
...
]
If i use the serializer as in the code above, I get
'routeClientConstraints': [
OrderedDict([
('name', 'Minimum life boats'),
('value', '6')
])
]
I tried changing the serializer to: routeClientConstraints = SimpleRouteClientConstraintSerializer(many=True, required=False).data
, which i expected would give me json format, but i got this:
'routeClientConstraints': [
OrderedDict([
('id', 1),
('value', '6'),
('created_at', '2018-11-10T14:17:39.263848Z'),
('updated_at', '2018-11-10T14:17:39.263861Z'),
('route_default_constraint', 1),
('route', 1)])
]
How do i return a list of dictionaries?
python django django-serializer
python django django-serializer
edited Nov 10 at 16:33
asked Nov 10 at 14:41
User632716
2,38911233
2,38911233
AnOrderedDict
is a dictionary. When it is converted to JSON it will do the right thing.
– Will Keeling
Nov 10 at 21:40
add a comment |
AnOrderedDict
is a dictionary. When it is converted to JSON it will do the right thing.
– Will Keeling
Nov 10 at 21:40
An
OrderedDict
is a dictionary. When it is converted to JSON it will do the right thing.– Will Keeling
Nov 10 at 21:40
An
OrderedDict
is a dictionary. When it is converted to JSON it will do the right thing.– Will Keeling
Nov 10 at 21:40
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53240027%2fdjango-serilizer-getting-an-ordered-dictionary-in-embeded-serializer%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
BttHH2
An
OrderedDict
is a dictionary. When it is converted to JSON it will do the right thing.– Will Keeling
Nov 10 at 21:40