AWS | Using DynamoDB results in “ERROR TypeError: Cannot convert undefined or null to object”
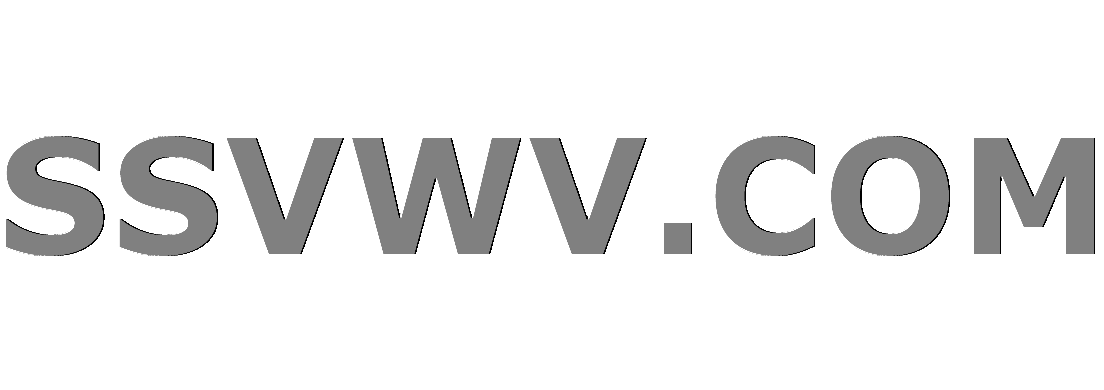
Multi tool use
up vote
0
down vote
favorite
Im trying to utilize AWS JS SDK in my Angular application.
Consider the following code:
import * as AWS from 'aws-sdk';
import {Component, OnInit} from '@angular/core';
declare let AWSCognito: any;
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit {
private _dynamodb;
constructor() {
AWS.config.region = 'eu-west-2'; // Region
AWS.config.credentials = new AWS.CognitoIdentityCredentials({
IdentityPoolId: 'eu-west-2:xxyyzz'
});
this._dynamodb = new AWS.DynamoDB.DocumentClient();
}
ngOnInit() {
this._dynamodb.get({TableName: 'somename', Key: {projectId: 123321}}, (err, data) => {
})
}
}
When the above code is executed the following error appears:
ERROR TypeError: Cannot convert undefined or null to object
I can see that it's this._dynamodb.get
that makes the system crash.
Any idea what I'm doing wrong?
EDIT:
Full stack trace:
ERROR TypeError: Cannot convert undefined or null to object
at hasOwnProperty (<anonymous>)
at isEndpointDiscoveryApplicable (discover_endpoint.js:279)
at Request.discoverEndpoint (discover_endpoint.js:322)
at Request.callListeners (sequential_executor.js:102)
at Request.emit (sequential_executor.js:78)
at Request.emit (request.js:683)
at Request.transition (request.js:22)
at AcceptorStateMachine.runTo (state_machine.js:14)
at state_machine.js:26
at Request.<anonymous> (request.js:38)
typescript amazon-web-services amazon-dynamodb
|
show 2 more comments
up vote
0
down vote
favorite
Im trying to utilize AWS JS SDK in my Angular application.
Consider the following code:
import * as AWS from 'aws-sdk';
import {Component, OnInit} from '@angular/core';
declare let AWSCognito: any;
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit {
private _dynamodb;
constructor() {
AWS.config.region = 'eu-west-2'; // Region
AWS.config.credentials = new AWS.CognitoIdentityCredentials({
IdentityPoolId: 'eu-west-2:xxyyzz'
});
this._dynamodb = new AWS.DynamoDB.DocumentClient();
}
ngOnInit() {
this._dynamodb.get({TableName: 'somename', Key: {projectId: 123321}}, (err, data) => {
})
}
}
When the above code is executed the following error appears:
ERROR TypeError: Cannot convert undefined or null to object
I can see that it's this._dynamodb.get
that makes the system crash.
Any idea what I'm doing wrong?
EDIT:
Full stack trace:
ERROR TypeError: Cannot convert undefined or null to object
at hasOwnProperty (<anonymous>)
at isEndpointDiscoveryApplicable (discover_endpoint.js:279)
at Request.discoverEndpoint (discover_endpoint.js:322)
at Request.callListeners (sequential_executor.js:102)
at Request.emit (sequential_executor.js:78)
at Request.emit (request.js:683)
at Request.transition (request.js:22)
at AcceptorStateMachine.runTo (state_machine.js:14)
at state_machine.js:26
at Request.<anonymous> (request.js:38)
typescript amazon-web-services amazon-dynamodb
If you add logging of this._dynamodb to the constructor after initializing _dynamodb and in the ngOnInit() method before invoking get(), does it provide any clues?
– jarmod
Nov 10 at 15:33
@jarmod it provides no clues. I can console log the pointer to the function (console.log(this._dynamodb.get)) just above the function call
– Vingtoft
Nov 10 at 16:22
For what it's worth, a simplified version of this code (no Angular, no classes) getting Key: { projectId: 123321 } using the DocumentClient works just fine for me. Are you using up to date AWS SDK? Can you post the full stack trace?
– jarmod
Nov 10 at 16:37
See edit. I have other projects where similar code works just fine. I think it something to do with a upgrade in a node_module, but Im not sure. On think I really hate with Angular is how a project can break apart when a single node component is upgraded, it can be a real struggle to solve
– Vingtoft
Nov 10 at 16:41
I don't see the get call in the stack trace. Are you sure that the this._dynamodb.get() call is the culprit?
– jarmod
Nov 10 at 16:44
|
show 2 more comments
up vote
0
down vote
favorite
up vote
0
down vote
favorite
Im trying to utilize AWS JS SDK in my Angular application.
Consider the following code:
import * as AWS from 'aws-sdk';
import {Component, OnInit} from '@angular/core';
declare let AWSCognito: any;
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit {
private _dynamodb;
constructor() {
AWS.config.region = 'eu-west-2'; // Region
AWS.config.credentials = new AWS.CognitoIdentityCredentials({
IdentityPoolId: 'eu-west-2:xxyyzz'
});
this._dynamodb = new AWS.DynamoDB.DocumentClient();
}
ngOnInit() {
this._dynamodb.get({TableName: 'somename', Key: {projectId: 123321}}, (err, data) => {
})
}
}
When the above code is executed the following error appears:
ERROR TypeError: Cannot convert undefined or null to object
I can see that it's this._dynamodb.get
that makes the system crash.
Any idea what I'm doing wrong?
EDIT:
Full stack trace:
ERROR TypeError: Cannot convert undefined or null to object
at hasOwnProperty (<anonymous>)
at isEndpointDiscoveryApplicable (discover_endpoint.js:279)
at Request.discoverEndpoint (discover_endpoint.js:322)
at Request.callListeners (sequential_executor.js:102)
at Request.emit (sequential_executor.js:78)
at Request.emit (request.js:683)
at Request.transition (request.js:22)
at AcceptorStateMachine.runTo (state_machine.js:14)
at state_machine.js:26
at Request.<anonymous> (request.js:38)
typescript amazon-web-services amazon-dynamodb
Im trying to utilize AWS JS SDK in my Angular application.
Consider the following code:
import * as AWS from 'aws-sdk';
import {Component, OnInit} from '@angular/core';
declare let AWSCognito: any;
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit {
private _dynamodb;
constructor() {
AWS.config.region = 'eu-west-2'; // Region
AWS.config.credentials = new AWS.CognitoIdentityCredentials({
IdentityPoolId: 'eu-west-2:xxyyzz'
});
this._dynamodb = new AWS.DynamoDB.DocumentClient();
}
ngOnInit() {
this._dynamodb.get({TableName: 'somename', Key: {projectId: 123321}}, (err, data) => {
})
}
}
When the above code is executed the following error appears:
ERROR TypeError: Cannot convert undefined or null to object
I can see that it's this._dynamodb.get
that makes the system crash.
Any idea what I'm doing wrong?
EDIT:
Full stack trace:
ERROR TypeError: Cannot convert undefined or null to object
at hasOwnProperty (<anonymous>)
at isEndpointDiscoveryApplicable (discover_endpoint.js:279)
at Request.discoverEndpoint (discover_endpoint.js:322)
at Request.callListeners (sequential_executor.js:102)
at Request.emit (sequential_executor.js:78)
at Request.emit (request.js:683)
at Request.transition (request.js:22)
at AcceptorStateMachine.runTo (state_machine.js:14)
at state_machine.js:26
at Request.<anonymous> (request.js:38)
typescript amazon-web-services amazon-dynamodb
typescript amazon-web-services amazon-dynamodb
edited Nov 10 at 16:40
asked Nov 10 at 15:03
Vingtoft
3,52343050
3,52343050
If you add logging of this._dynamodb to the constructor after initializing _dynamodb and in the ngOnInit() method before invoking get(), does it provide any clues?
– jarmod
Nov 10 at 15:33
@jarmod it provides no clues. I can console log the pointer to the function (console.log(this._dynamodb.get)) just above the function call
– Vingtoft
Nov 10 at 16:22
For what it's worth, a simplified version of this code (no Angular, no classes) getting Key: { projectId: 123321 } using the DocumentClient works just fine for me. Are you using up to date AWS SDK? Can you post the full stack trace?
– jarmod
Nov 10 at 16:37
See edit. I have other projects where similar code works just fine. I think it something to do with a upgrade in a node_module, but Im not sure. On think I really hate with Angular is how a project can break apart when a single node component is upgraded, it can be a real struggle to solve
– Vingtoft
Nov 10 at 16:41
I don't see the get call in the stack trace. Are you sure that the this._dynamodb.get() call is the culprit?
– jarmod
Nov 10 at 16:44
|
show 2 more comments
If you add logging of this._dynamodb to the constructor after initializing _dynamodb and in the ngOnInit() method before invoking get(), does it provide any clues?
– jarmod
Nov 10 at 15:33
@jarmod it provides no clues. I can console log the pointer to the function (console.log(this._dynamodb.get)) just above the function call
– Vingtoft
Nov 10 at 16:22
For what it's worth, a simplified version of this code (no Angular, no classes) getting Key: { projectId: 123321 } using the DocumentClient works just fine for me. Are you using up to date AWS SDK? Can you post the full stack trace?
– jarmod
Nov 10 at 16:37
See edit. I have other projects where similar code works just fine. I think it something to do with a upgrade in a node_module, but Im not sure. On think I really hate with Angular is how a project can break apart when a single node component is upgraded, it can be a real struggle to solve
– Vingtoft
Nov 10 at 16:41
I don't see the get call in the stack trace. Are you sure that the this._dynamodb.get() call is the culprit?
– jarmod
Nov 10 at 16:44
If you add logging of this._dynamodb to the constructor after initializing _dynamodb and in the ngOnInit() method before invoking get(), does it provide any clues?
– jarmod
Nov 10 at 15:33
If you add logging of this._dynamodb to the constructor after initializing _dynamodb and in the ngOnInit() method before invoking get(), does it provide any clues?
– jarmod
Nov 10 at 15:33
@jarmod it provides no clues. I can console log the pointer to the function (console.log(this._dynamodb.get)) just above the function call
– Vingtoft
Nov 10 at 16:22
@jarmod it provides no clues. I can console log the pointer to the function (console.log(this._dynamodb.get)) just above the function call
– Vingtoft
Nov 10 at 16:22
For what it's worth, a simplified version of this code (no Angular, no classes) getting Key: { projectId: 123321 } using the DocumentClient works just fine for me. Are you using up to date AWS SDK? Can you post the full stack trace?
– jarmod
Nov 10 at 16:37
For what it's worth, a simplified version of this code (no Angular, no classes) getting Key: { projectId: 123321 } using the DocumentClient works just fine for me. Are you using up to date AWS SDK? Can you post the full stack trace?
– jarmod
Nov 10 at 16:37
See edit. I have other projects where similar code works just fine. I think it something to do with a upgrade in a node_module, but Im not sure. On think I really hate with Angular is how a project can break apart when a single node component is upgraded, it can be a real struggle to solve
– Vingtoft
Nov 10 at 16:41
See edit. I have other projects where similar code works just fine. I think it something to do with a upgrade in a node_module, but Im not sure. On think I really hate with Angular is how a project can break apart when a single node component is upgraded, it can be a real struggle to solve
– Vingtoft
Nov 10 at 16:41
I don't see the get call in the stack trace. Are you sure that the this._dynamodb.get() call is the culprit?
– jarmod
Nov 10 at 16:44
I don't see the get call in the stack trace. Are you sure that the this._dynamodb.get() call is the culprit?
– jarmod
Nov 10 at 16:44
|
show 2 more comments
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53240222%2faws-using-dynamodb-results-in-error-typeerror-cannot-convert-undefined-or-nu%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
nV,WwXvq7EXlKlHm,U4hdyR7JiBjjnv8OJt,xmcvqHB7jt jROf JPuK7hCFrZ,bIO8Q,zbl
If you add logging of this._dynamodb to the constructor after initializing _dynamodb and in the ngOnInit() method before invoking get(), does it provide any clues?
– jarmod
Nov 10 at 15:33
@jarmod it provides no clues. I can console log the pointer to the function (console.log(this._dynamodb.get)) just above the function call
– Vingtoft
Nov 10 at 16:22
For what it's worth, a simplified version of this code (no Angular, no classes) getting Key: { projectId: 123321 } using the DocumentClient works just fine for me. Are you using up to date AWS SDK? Can you post the full stack trace?
– jarmod
Nov 10 at 16:37
See edit. I have other projects where similar code works just fine. I think it something to do with a upgrade in a node_module, but Im not sure. On think I really hate with Angular is how a project can break apart when a single node component is upgraded, it can be a real struggle to solve
– Vingtoft
Nov 10 at 16:41
I don't see the get call in the stack trace. Are you sure that the this._dynamodb.get() call is the culprit?
– jarmod
Nov 10 at 16:44