Get SharedPreferences before widget build
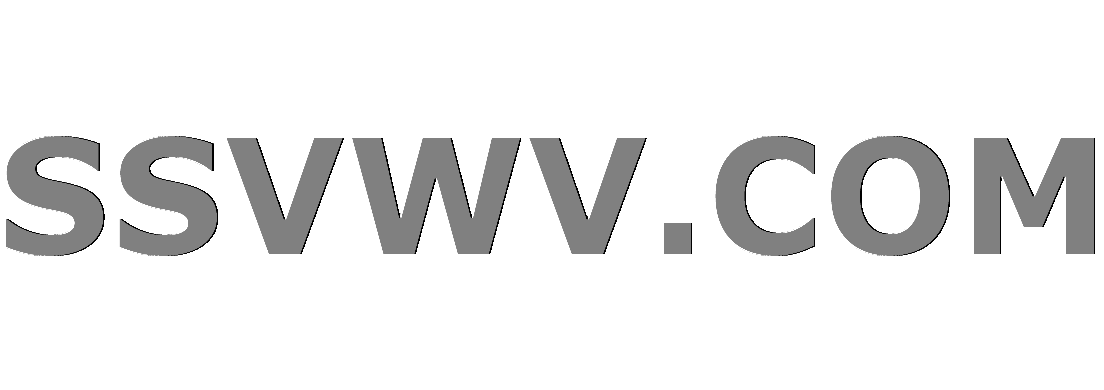
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I want to get my userid before the build fires in my statefulwidget.
If I do this, then build will render before I get my id. If I put it in setstate, my build will use the empty string first and then rerenders it again with my id, but this will cause unnecessary behaviours.
So how do I solve this?
String _userid = '';
Future<Null> setUserid() async {
SharedPreferences pref = await SharedPreferences.getInstance();
_userid = pref.getString('FB_USER');
}
initState() {
super.initState();
setUserid();
}
Build
// Widget build
new Flexible(
child: new StreamBuilder<QuerySnapshot>(
stream: Firestore.instance
.collection('users')
.document(_userid)
.collection('rooms')
.snapshots(),
builder:
(BuildContext context, AsyncSnapshot<QuerySnapshot> snapshot) {
if (!snapshot.hasData) return new Text('Loading...');
return new ListView(
children: snapshot.data.documents
.map(
(DocumentSnapshot document) => new Text('lol'),
// )
//new OverviewPresentation(presentation: document),
)
.toList(),
);
},
),
),

add a comment |
I want to get my userid before the build fires in my statefulwidget.
If I do this, then build will render before I get my id. If I put it in setstate, my build will use the empty string first and then rerenders it again with my id, but this will cause unnecessary behaviours.
So how do I solve this?
String _userid = '';
Future<Null> setUserid() async {
SharedPreferences pref = await SharedPreferences.getInstance();
_userid = pref.getString('FB_USER');
}
initState() {
super.initState();
setUserid();
}
Build
// Widget build
new Flexible(
child: new StreamBuilder<QuerySnapshot>(
stream: Firestore.instance
.collection('users')
.document(_userid)
.collection('rooms')
.snapshots(),
builder:
(BuildContext context, AsyncSnapshot<QuerySnapshot> snapshot) {
if (!snapshot.hasData) return new Text('Loading...');
return new ListView(
children: snapshot.data.documents
.map(
(DocumentSnapshot document) => new Text('lol'),
// )
//new OverviewPresentation(presentation: document),
)
.toList(),
);
},
),
),

add a comment |
I want to get my userid before the build fires in my statefulwidget.
If I do this, then build will render before I get my id. If I put it in setstate, my build will use the empty string first and then rerenders it again with my id, but this will cause unnecessary behaviours.
So how do I solve this?
String _userid = '';
Future<Null> setUserid() async {
SharedPreferences pref = await SharedPreferences.getInstance();
_userid = pref.getString('FB_USER');
}
initState() {
super.initState();
setUserid();
}
Build
// Widget build
new Flexible(
child: new StreamBuilder<QuerySnapshot>(
stream: Firestore.instance
.collection('users')
.document(_userid)
.collection('rooms')
.snapshots(),
builder:
(BuildContext context, AsyncSnapshot<QuerySnapshot> snapshot) {
if (!snapshot.hasData) return new Text('Loading...');
return new ListView(
children: snapshot.data.documents
.map(
(DocumentSnapshot document) => new Text('lol'),
// )
//new OverviewPresentation(presentation: document),
)
.toList(),
);
},
),
),

I want to get my userid before the build fires in my statefulwidget.
If I do this, then build will render before I get my id. If I put it in setstate, my build will use the empty string first and then rerenders it again with my id, but this will cause unnecessary behaviours.
So how do I solve this?
String _userid = '';
Future<Null> setUserid() async {
SharedPreferences pref = await SharedPreferences.getInstance();
_userid = pref.getString('FB_USER');
}
initState() {
super.initState();
setUserid();
}
Build
// Widget build
new Flexible(
child: new StreamBuilder<QuerySnapshot>(
stream: Firestore.instance
.collection('users')
.document(_userid)
.collection('rooms')
.snapshots(),
builder:
(BuildContext context, AsyncSnapshot<QuerySnapshot> snapshot) {
if (!snapshot.hasData) return new Text('Loading...');
return new ListView(
children: snapshot.data.documents
.map(
(DocumentSnapshot document) => new Text('lol'),
// )
//new OverviewPresentation(presentation: document),
)
.toList(),
);
},
),
),


asked Nov 16 '18 at 10:20
SyphSyph
1717
1717
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
You can't, but you can guard against it being null
.
Also it's easier to move the StreamBuilder
to initState
String _userid = '';
dynamic _data;
Future<Null> setUserid() async {
SharedPreferences pref = await SharedPreferences.getInstance();
_userid = pref.getString('FB_USER');
_data = await Firestore.instance
.collection('users')
.document(_userid)
.collection('rooms')
.snapshots().first;
setState(() {});
}
initState() {
super.initState();
setUserid();
}
return new Flexible(
child:
if(_data == null) return new Text('Loading...');
return new ListView(
children: _data.documents
.map(
(DocumentSnapshot document) => new Text('lol'),
// )
//new OverviewPresentation(presentation: document),
)
.toList(),
);
},
),
),
Doing it this way means, my widget doesn't listen for data being added or modified in firestore right and if my _userid is empty when I use it, firestore will throw an error since my call will be /'users'//'rooms'/
– Syph
Nov 16 '18 at 11:01
1
You can uselisten()
instead offirst
and movesetState(...)
intolisten(...)
– Günter Zöchbauer
Nov 16 '18 at 11:07
add a comment |
You can use FutureBuilder
Future<String> setUserid() async {
SharedPreferences pref = await SharedPreferences.getInstance();
_userid = pref.getString('FB_USER');
return _userid;
}
@override
Widget build(BuildContext context) {
return FutureBuilder(
future: setUserid(),
builder: (BuildContext context, AsyncSnapshot<String> snapshot) {
if (snapshot.hasData) {
return ... // your widget
} else return CircularProgressIndicator();
});
Something like this
don't call the async function within build: stackoverflow.com/questions/52249578/…
– Rémi Rousselet
Nov 16 '18 at 10:33
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53335797%2fget-sharedpreferences-before-widget-build%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can't, but you can guard against it being null
.
Also it's easier to move the StreamBuilder
to initState
String _userid = '';
dynamic _data;
Future<Null> setUserid() async {
SharedPreferences pref = await SharedPreferences.getInstance();
_userid = pref.getString('FB_USER');
_data = await Firestore.instance
.collection('users')
.document(_userid)
.collection('rooms')
.snapshots().first;
setState(() {});
}
initState() {
super.initState();
setUserid();
}
return new Flexible(
child:
if(_data == null) return new Text('Loading...');
return new ListView(
children: _data.documents
.map(
(DocumentSnapshot document) => new Text('lol'),
// )
//new OverviewPresentation(presentation: document),
)
.toList(),
);
},
),
),
Doing it this way means, my widget doesn't listen for data being added or modified in firestore right and if my _userid is empty when I use it, firestore will throw an error since my call will be /'users'//'rooms'/
– Syph
Nov 16 '18 at 11:01
1
You can uselisten()
instead offirst
and movesetState(...)
intolisten(...)
– Günter Zöchbauer
Nov 16 '18 at 11:07
add a comment |
You can't, but you can guard against it being null
.
Also it's easier to move the StreamBuilder
to initState
String _userid = '';
dynamic _data;
Future<Null> setUserid() async {
SharedPreferences pref = await SharedPreferences.getInstance();
_userid = pref.getString('FB_USER');
_data = await Firestore.instance
.collection('users')
.document(_userid)
.collection('rooms')
.snapshots().first;
setState(() {});
}
initState() {
super.initState();
setUserid();
}
return new Flexible(
child:
if(_data == null) return new Text('Loading...');
return new ListView(
children: _data.documents
.map(
(DocumentSnapshot document) => new Text('lol'),
// )
//new OverviewPresentation(presentation: document),
)
.toList(),
);
},
),
),
Doing it this way means, my widget doesn't listen for data being added or modified in firestore right and if my _userid is empty when I use it, firestore will throw an error since my call will be /'users'//'rooms'/
– Syph
Nov 16 '18 at 11:01
1
You can uselisten()
instead offirst
and movesetState(...)
intolisten(...)
– Günter Zöchbauer
Nov 16 '18 at 11:07
add a comment |
You can't, but you can guard against it being null
.
Also it's easier to move the StreamBuilder
to initState
String _userid = '';
dynamic _data;
Future<Null> setUserid() async {
SharedPreferences pref = await SharedPreferences.getInstance();
_userid = pref.getString('FB_USER');
_data = await Firestore.instance
.collection('users')
.document(_userid)
.collection('rooms')
.snapshots().first;
setState(() {});
}
initState() {
super.initState();
setUserid();
}
return new Flexible(
child:
if(_data == null) return new Text('Loading...');
return new ListView(
children: _data.documents
.map(
(DocumentSnapshot document) => new Text('lol'),
// )
//new OverviewPresentation(presentation: document),
)
.toList(),
);
},
),
),
You can't, but you can guard against it being null
.
Also it's easier to move the StreamBuilder
to initState
String _userid = '';
dynamic _data;
Future<Null> setUserid() async {
SharedPreferences pref = await SharedPreferences.getInstance();
_userid = pref.getString('FB_USER');
_data = await Firestore.instance
.collection('users')
.document(_userid)
.collection('rooms')
.snapshots().first;
setState(() {});
}
initState() {
super.initState();
setUserid();
}
return new Flexible(
child:
if(_data == null) return new Text('Loading...');
return new ListView(
children: _data.documents
.map(
(DocumentSnapshot document) => new Text('lol'),
// )
//new OverviewPresentation(presentation: document),
)
.toList(),
);
},
),
),
answered Nov 16 '18 at 10:28


Günter ZöchbauerGünter Zöchbauer
335k721018947
335k721018947
Doing it this way means, my widget doesn't listen for data being added or modified in firestore right and if my _userid is empty when I use it, firestore will throw an error since my call will be /'users'//'rooms'/
– Syph
Nov 16 '18 at 11:01
1
You can uselisten()
instead offirst
and movesetState(...)
intolisten(...)
– Günter Zöchbauer
Nov 16 '18 at 11:07
add a comment |
Doing it this way means, my widget doesn't listen for data being added or modified in firestore right and if my _userid is empty when I use it, firestore will throw an error since my call will be /'users'//'rooms'/
– Syph
Nov 16 '18 at 11:01
1
You can uselisten()
instead offirst
and movesetState(...)
intolisten(...)
– Günter Zöchbauer
Nov 16 '18 at 11:07
Doing it this way means, my widget doesn't listen for data being added or modified in firestore right and if my _userid is empty when I use it, firestore will throw an error since my call will be /'users'//'rooms'/
– Syph
Nov 16 '18 at 11:01
Doing it this way means, my widget doesn't listen for data being added or modified in firestore right and if my _userid is empty when I use it, firestore will throw an error since my call will be /'users'//'rooms'/
– Syph
Nov 16 '18 at 11:01
1
1
You can use
listen()
instead of first
and move setState(...)
into listen(...)
– Günter Zöchbauer
Nov 16 '18 at 11:07
You can use
listen()
instead of first
and move setState(...)
into listen(...)
– Günter Zöchbauer
Nov 16 '18 at 11:07
add a comment |
You can use FutureBuilder
Future<String> setUserid() async {
SharedPreferences pref = await SharedPreferences.getInstance();
_userid = pref.getString('FB_USER');
return _userid;
}
@override
Widget build(BuildContext context) {
return FutureBuilder(
future: setUserid(),
builder: (BuildContext context, AsyncSnapshot<String> snapshot) {
if (snapshot.hasData) {
return ... // your widget
} else return CircularProgressIndicator();
});
Something like this
don't call the async function within build: stackoverflow.com/questions/52249578/…
– Rémi Rousselet
Nov 16 '18 at 10:33
add a comment |
You can use FutureBuilder
Future<String> setUserid() async {
SharedPreferences pref = await SharedPreferences.getInstance();
_userid = pref.getString('FB_USER');
return _userid;
}
@override
Widget build(BuildContext context) {
return FutureBuilder(
future: setUserid(),
builder: (BuildContext context, AsyncSnapshot<String> snapshot) {
if (snapshot.hasData) {
return ... // your widget
} else return CircularProgressIndicator();
});
Something like this
don't call the async function within build: stackoverflow.com/questions/52249578/…
– Rémi Rousselet
Nov 16 '18 at 10:33
add a comment |
You can use FutureBuilder
Future<String> setUserid() async {
SharedPreferences pref = await SharedPreferences.getInstance();
_userid = pref.getString('FB_USER');
return _userid;
}
@override
Widget build(BuildContext context) {
return FutureBuilder(
future: setUserid(),
builder: (BuildContext context, AsyncSnapshot<String> snapshot) {
if (snapshot.hasData) {
return ... // your widget
} else return CircularProgressIndicator();
});
Something like this
You can use FutureBuilder
Future<String> setUserid() async {
SharedPreferences pref = await SharedPreferences.getInstance();
_userid = pref.getString('FB_USER');
return _userid;
}
@override
Widget build(BuildContext context) {
return FutureBuilder(
future: setUserid(),
builder: (BuildContext context, AsyncSnapshot<String> snapshot) {
if (snapshot.hasData) {
return ... // your widget
} else return CircularProgressIndicator();
});
Something like this
answered Nov 16 '18 at 10:26


Andrey TurkovskyAndrey Turkovsky
1
1
don't call the async function within build: stackoverflow.com/questions/52249578/…
– Rémi Rousselet
Nov 16 '18 at 10:33
add a comment |
don't call the async function within build: stackoverflow.com/questions/52249578/…
– Rémi Rousselet
Nov 16 '18 at 10:33
don't call the async function within build: stackoverflow.com/questions/52249578/…
– Rémi Rousselet
Nov 16 '18 at 10:33
don't call the async function within build: stackoverflow.com/questions/52249578/…
– Rémi Rousselet
Nov 16 '18 at 10:33
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53335797%2fget-sharedpreferences-before-widget-build%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
fL8aoNaRT5Yx8DTYP3vs4cI3