Testing MVC controller using reactor with WebClient
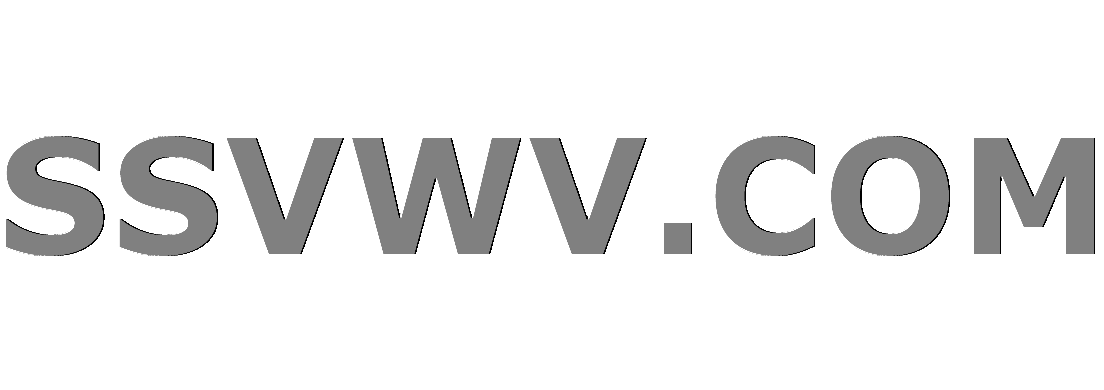
Multi tool use
@RestController
@RequestMapping("/api/v1/platform")
public class PlatformController {
@Autowired
private PlatformRepository platformRepository;
@GetMapping
public Flux<Platform> findAll() {
log.info("Calling findAll platforms");
return platformRepository.findAll();
}
}
@RunWith(SpringRunner.class)
@WebFluxTest
public class PlatformControllerTest {
@MockBean
private PlatformRepository platformRepository;
@Test
public void findAll() throws Exception {
WebTestClient client = WebTestClient.bindToController(new PlatformController()).build();
client.get()
.uri("/api/v1/platform")
.exchange()
.expectStatus().isOk();
}
}
Above I have attached a simple POC of what i want to achieve. I am unable to inject the mock into the controller for my tests and the tests fails. Is there any other way to do this or am i missing some fundamental concept?
java spring spring-boot spring-webflux project-reactor
add a comment |
@RestController
@RequestMapping("/api/v1/platform")
public class PlatformController {
@Autowired
private PlatformRepository platformRepository;
@GetMapping
public Flux<Platform> findAll() {
log.info("Calling findAll platforms");
return platformRepository.findAll();
}
}
@RunWith(SpringRunner.class)
@WebFluxTest
public class PlatformControllerTest {
@MockBean
private PlatformRepository platformRepository;
@Test
public void findAll() throws Exception {
WebTestClient client = WebTestClient.bindToController(new PlatformController()).build();
client.get()
.uri("/api/v1/platform")
.exchange()
.expectStatus().isOk();
}
}
Above I have attached a simple POC of what i want to achieve. I am unable to inject the mock into the controller for my tests and the tests fails. Is there any other way to do this or am i missing some fundamental concept?
java spring spring-boot spring-webflux project-reactor
add a comment |
@RestController
@RequestMapping("/api/v1/platform")
public class PlatformController {
@Autowired
private PlatformRepository platformRepository;
@GetMapping
public Flux<Platform> findAll() {
log.info("Calling findAll platforms");
return platformRepository.findAll();
}
}
@RunWith(SpringRunner.class)
@WebFluxTest
public class PlatformControllerTest {
@MockBean
private PlatformRepository platformRepository;
@Test
public void findAll() throws Exception {
WebTestClient client = WebTestClient.bindToController(new PlatformController()).build();
client.get()
.uri("/api/v1/platform")
.exchange()
.expectStatus().isOk();
}
}
Above I have attached a simple POC of what i want to achieve. I am unable to inject the mock into the controller for my tests and the tests fails. Is there any other way to do this or am i missing some fundamental concept?
java spring spring-boot spring-webflux project-reactor
@RestController
@RequestMapping("/api/v1/platform")
public class PlatformController {
@Autowired
private PlatformRepository platformRepository;
@GetMapping
public Flux<Platform> findAll() {
log.info("Calling findAll platforms");
return platformRepository.findAll();
}
}
@RunWith(SpringRunner.class)
@WebFluxTest
public class PlatformControllerTest {
@MockBean
private PlatformRepository platformRepository;
@Test
public void findAll() throws Exception {
WebTestClient client = WebTestClient.bindToController(new PlatformController()).build();
client.get()
.uri("/api/v1/platform")
.exchange()
.expectStatus().isOk();
}
}
Above I have attached a simple POC of what i want to achieve. I am unable to inject the mock into the controller for my tests and the tests fails. Is there any other way to do this or am i missing some fundamental concept?
java spring spring-boot spring-webflux project-reactor
java spring spring-boot spring-webflux project-reactor
edited Nov 15 '18 at 13:25
Nandagopal Sekar
asked Nov 15 '18 at 13:12
Nandagopal SekarNandagopal Sekar
668
668
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
Things started to work after i added the controller class to the context configuration.
@RunWith(SpringRunner.class)
@ContextConfiguration(classes = {
PlatformController.class,
MongoTestConfig.class
})
@WebFluxTest(PlatformController.class)
I think it could be a bug, as the controller should be in the context after being included in the WebFluxTest annotation.
add a comment |
From the docs:
@WebFluxTest auto-configures the Spring WebFlux infrastructure and
limits scanned beans to @Controller, @ControllerAdvice,
@JsonComponent, Converter, GenericConverter, and WebFluxConfigurer.
Regular @Component beans are not scanned when the @WebFluxTest
annotation is used.
Could you try to add your controller class inside WebFluxTest
annotation?
@WebFluxTest(PlatformController.class)
Unfortunately that does not help. I have tried another approach as well. I tried adding the @WebFluxTest(PlatformController.class) and tried to Autowire WebTestClient. In that case it does not even call the controller. It replies back with a 404. – Nandagopal Sekar 23 secs ago edit
– Nandagopal Sekar
Nov 15 '18 at 14:27
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53320296%2ftesting-mvc-controller-using-reactor-with-webclient%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Things started to work after i added the controller class to the context configuration.
@RunWith(SpringRunner.class)
@ContextConfiguration(classes = {
PlatformController.class,
MongoTestConfig.class
})
@WebFluxTest(PlatformController.class)
I think it could be a bug, as the controller should be in the context after being included in the WebFluxTest annotation.
add a comment |
Things started to work after i added the controller class to the context configuration.
@RunWith(SpringRunner.class)
@ContextConfiguration(classes = {
PlatformController.class,
MongoTestConfig.class
})
@WebFluxTest(PlatformController.class)
I think it could be a bug, as the controller should be in the context after being included in the WebFluxTest annotation.
add a comment |
Things started to work after i added the controller class to the context configuration.
@RunWith(SpringRunner.class)
@ContextConfiguration(classes = {
PlatformController.class,
MongoTestConfig.class
})
@WebFluxTest(PlatformController.class)
I think it could be a bug, as the controller should be in the context after being included in the WebFluxTest annotation.
Things started to work after i added the controller class to the context configuration.
@RunWith(SpringRunner.class)
@ContextConfiguration(classes = {
PlatformController.class,
MongoTestConfig.class
})
@WebFluxTest(PlatformController.class)
I think it could be a bug, as the controller should be in the context after being included in the WebFluxTest annotation.
answered Nov 16 '18 at 9:45
Nandagopal SekarNandagopal Sekar
668
668
add a comment |
add a comment |
From the docs:
@WebFluxTest auto-configures the Spring WebFlux infrastructure and
limits scanned beans to @Controller, @ControllerAdvice,
@JsonComponent, Converter, GenericConverter, and WebFluxConfigurer.
Regular @Component beans are not scanned when the @WebFluxTest
annotation is used.
Could you try to add your controller class inside WebFluxTest
annotation?
@WebFluxTest(PlatformController.class)
Unfortunately that does not help. I have tried another approach as well. I tried adding the @WebFluxTest(PlatformController.class) and tried to Autowire WebTestClient. In that case it does not even call the controller. It replies back with a 404. – Nandagopal Sekar 23 secs ago edit
– Nandagopal Sekar
Nov 15 '18 at 14:27
add a comment |
From the docs:
@WebFluxTest auto-configures the Spring WebFlux infrastructure and
limits scanned beans to @Controller, @ControllerAdvice,
@JsonComponent, Converter, GenericConverter, and WebFluxConfigurer.
Regular @Component beans are not scanned when the @WebFluxTest
annotation is used.
Could you try to add your controller class inside WebFluxTest
annotation?
@WebFluxTest(PlatformController.class)
Unfortunately that does not help. I have tried another approach as well. I tried adding the @WebFluxTest(PlatformController.class) and tried to Autowire WebTestClient. In that case it does not even call the controller. It replies back with a 404. – Nandagopal Sekar 23 secs ago edit
– Nandagopal Sekar
Nov 15 '18 at 14:27
add a comment |
From the docs:
@WebFluxTest auto-configures the Spring WebFlux infrastructure and
limits scanned beans to @Controller, @ControllerAdvice,
@JsonComponent, Converter, GenericConverter, and WebFluxConfigurer.
Regular @Component beans are not scanned when the @WebFluxTest
annotation is used.
Could you try to add your controller class inside WebFluxTest
annotation?
@WebFluxTest(PlatformController.class)
From the docs:
@WebFluxTest auto-configures the Spring WebFlux infrastructure and
limits scanned beans to @Controller, @ControllerAdvice,
@JsonComponent, Converter, GenericConverter, and WebFluxConfigurer.
Regular @Component beans are not scanned when the @WebFluxTest
annotation is used.
Could you try to add your controller class inside WebFluxTest
annotation?
@WebFluxTest(PlatformController.class)
answered Nov 15 '18 at 14:25
alasteralaster
1,91921527
1,91921527
Unfortunately that does not help. I have tried another approach as well. I tried adding the @WebFluxTest(PlatformController.class) and tried to Autowire WebTestClient. In that case it does not even call the controller. It replies back with a 404. – Nandagopal Sekar 23 secs ago edit
– Nandagopal Sekar
Nov 15 '18 at 14:27
add a comment |
Unfortunately that does not help. I have tried another approach as well. I tried adding the @WebFluxTest(PlatformController.class) and tried to Autowire WebTestClient. In that case it does not even call the controller. It replies back with a 404. – Nandagopal Sekar 23 secs ago edit
– Nandagopal Sekar
Nov 15 '18 at 14:27
Unfortunately that does not help. I have tried another approach as well. I tried adding the @WebFluxTest(PlatformController.class) and tried to Autowire WebTestClient. In that case it does not even call the controller. It replies back with a 404. – Nandagopal Sekar 23 secs ago edit
– Nandagopal Sekar
Nov 15 '18 at 14:27
Unfortunately that does not help. I have tried another approach as well. I tried adding the @WebFluxTest(PlatformController.class) and tried to Autowire WebTestClient. In that case it does not even call the controller. It replies back with a 404. – Nandagopal Sekar 23 secs ago edit
– Nandagopal Sekar
Nov 15 '18 at 14:27
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53320296%2ftesting-mvc-controller-using-reactor-with-webclient%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ByFnPjAFUVPnIZwbh8qGAGk1BaPYO5xhyRmiRdwDdDIqDOYtuQX9Du6aQImXiS9WU82 jD wYilV8Ud,Q7snZwJj RAG3 W9D,upPvTd