I am trying to calculate this continued fraction but i cant seem to work, the program however can be compiled...
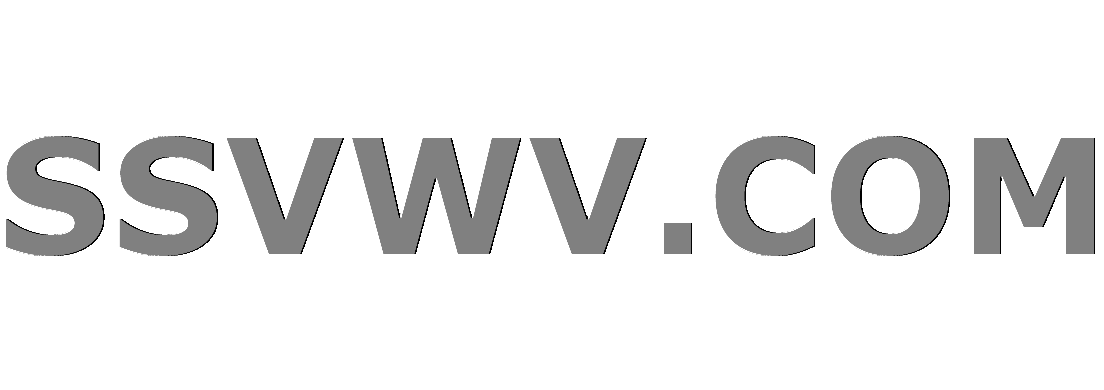
Multi tool use
Im trying to get this program working as it requires to calculate continued fraction inputed in a linked list. the programme doesn't show any error however it always crashes in the middle. Can someone help me?
The task is simply to store the programme on a linked list and then calculate it by taking the parameter from the link. It is a continued fraction.
#include <iostream>
#include <cmath>
using namespace std;
struct node
{
int data;
node *next;
} *h=nullptr, *t=nullptr;
float calculation (int co)
{
float a,c;
node *b,*f;
b->next = f;
while(co != 0)
{
f = t;
a = (float)f->data;
a = 1/a;
c = (float)b->data;
a = c + a;
t = b;
co--;
}
return a;
}
void storedata (int& c)
{
node *n = new node;
n->data = c;
n->next = nullptr;
cout<<n->data<<endl;
if(h==nullptr)
{
h=n;
t=n;
n=nullptr;
}
else
{
t->next=n;
t=n;
}
}
void formula (int a, int b, int co)
{
int c;
int z;
while (co!=0)
{
c = a/b;
storedata(c);
z = b*c;
z = a-z;
a = b;
b = z;
co--;
}
}
int main ()
{
int a,b,c,z,co,d;
float e;
a = 123;
b = 100;
co = 5;
formula (a,b,co);
e = calculation(co);
cout<<"cf1 = 123/100 ="<<e;
}
c++ linked-list floating-point singly-linked-list continued-fractions
add a comment |
Im trying to get this program working as it requires to calculate continued fraction inputed in a linked list. the programme doesn't show any error however it always crashes in the middle. Can someone help me?
The task is simply to store the programme on a linked list and then calculate it by taking the parameter from the link. It is a continued fraction.
#include <iostream>
#include <cmath>
using namespace std;
struct node
{
int data;
node *next;
} *h=nullptr, *t=nullptr;
float calculation (int co)
{
float a,c;
node *b,*f;
b->next = f;
while(co != 0)
{
f = t;
a = (float)f->data;
a = 1/a;
c = (float)b->data;
a = c + a;
t = b;
co--;
}
return a;
}
void storedata (int& c)
{
node *n = new node;
n->data = c;
n->next = nullptr;
cout<<n->data<<endl;
if(h==nullptr)
{
h=n;
t=n;
n=nullptr;
}
else
{
t->next=n;
t=n;
}
}
void formula (int a, int b, int co)
{
int c;
int z;
while (co!=0)
{
c = a/b;
storedata(c);
z = b*c;
z = a-z;
a = b;
b = z;
co--;
}
}
int main ()
{
int a,b,c,z,co,d;
float e;
a = 123;
b = 100;
co = 5;
formula (a,b,co);
e = calculation(co);
cout<<"cf1 = 123/100 ="<<e;
}
c++ linked-list floating-point singly-linked-list continued-fractions
Nobody wants to read code with one-letter variable names. Least of all your instructor.
– stark
Nov 15 '18 at 14:26
I don't know if it can help, but in a recent question I posted a code that calculates continued fraction: stackoverflow.com/questions/53267626/…. I don't feel at ease with ads. I will suppress this comment if people disagree.
– Damien
Nov 15 '18 at 16:13
add a comment |
Im trying to get this program working as it requires to calculate continued fraction inputed in a linked list. the programme doesn't show any error however it always crashes in the middle. Can someone help me?
The task is simply to store the programme on a linked list and then calculate it by taking the parameter from the link. It is a continued fraction.
#include <iostream>
#include <cmath>
using namespace std;
struct node
{
int data;
node *next;
} *h=nullptr, *t=nullptr;
float calculation (int co)
{
float a,c;
node *b,*f;
b->next = f;
while(co != 0)
{
f = t;
a = (float)f->data;
a = 1/a;
c = (float)b->data;
a = c + a;
t = b;
co--;
}
return a;
}
void storedata (int& c)
{
node *n = new node;
n->data = c;
n->next = nullptr;
cout<<n->data<<endl;
if(h==nullptr)
{
h=n;
t=n;
n=nullptr;
}
else
{
t->next=n;
t=n;
}
}
void formula (int a, int b, int co)
{
int c;
int z;
while (co!=0)
{
c = a/b;
storedata(c);
z = b*c;
z = a-z;
a = b;
b = z;
co--;
}
}
int main ()
{
int a,b,c,z,co,d;
float e;
a = 123;
b = 100;
co = 5;
formula (a,b,co);
e = calculation(co);
cout<<"cf1 = 123/100 ="<<e;
}
c++ linked-list floating-point singly-linked-list continued-fractions
Im trying to get this program working as it requires to calculate continued fraction inputed in a linked list. the programme doesn't show any error however it always crashes in the middle. Can someone help me?
The task is simply to store the programme on a linked list and then calculate it by taking the parameter from the link. It is a continued fraction.
#include <iostream>
#include <cmath>
using namespace std;
struct node
{
int data;
node *next;
} *h=nullptr, *t=nullptr;
float calculation (int co)
{
float a,c;
node *b,*f;
b->next = f;
while(co != 0)
{
f = t;
a = (float)f->data;
a = 1/a;
c = (float)b->data;
a = c + a;
t = b;
co--;
}
return a;
}
void storedata (int& c)
{
node *n = new node;
n->data = c;
n->next = nullptr;
cout<<n->data<<endl;
if(h==nullptr)
{
h=n;
t=n;
n=nullptr;
}
else
{
t->next=n;
t=n;
}
}
void formula (int a, int b, int co)
{
int c;
int z;
while (co!=0)
{
c = a/b;
storedata(c);
z = b*c;
z = a-z;
a = b;
b = z;
co--;
}
}
int main ()
{
int a,b,c,z,co,d;
float e;
a = 123;
b = 100;
co = 5;
formula (a,b,co);
e = calculation(co);
cout<<"cf1 = 123/100 ="<<e;
}
c++ linked-list floating-point singly-linked-list continued-fractions
c++ linked-list floating-point singly-linked-list continued-fractions
edited Nov 16 '18 at 9:23


Rudy Velthuis
24.9k43675
24.9k43675
asked Nov 15 '18 at 13:10
navesaurusnavesaurus
41
41
Nobody wants to read code with one-letter variable names. Least of all your instructor.
– stark
Nov 15 '18 at 14:26
I don't know if it can help, but in a recent question I posted a code that calculates continued fraction: stackoverflow.com/questions/53267626/…. I don't feel at ease with ads. I will suppress this comment if people disagree.
– Damien
Nov 15 '18 at 16:13
add a comment |
Nobody wants to read code with one-letter variable names. Least of all your instructor.
– stark
Nov 15 '18 at 14:26
I don't know if it can help, but in a recent question I posted a code that calculates continued fraction: stackoverflow.com/questions/53267626/…. I don't feel at ease with ads. I will suppress this comment if people disagree.
– Damien
Nov 15 '18 at 16:13
Nobody wants to read code with one-letter variable names. Least of all your instructor.
– stark
Nov 15 '18 at 14:26
Nobody wants to read code with one-letter variable names. Least of all your instructor.
– stark
Nov 15 '18 at 14:26
I don't know if it can help, but in a recent question I posted a code that calculates continued fraction: stackoverflow.com/questions/53267626/…. I don't feel at ease with ads. I will suppress this comment if people disagree.
– Damien
Nov 15 '18 at 16:13
I don't know if it can help, but in a recent question I posted a code that calculates continued fraction: stackoverflow.com/questions/53267626/…. I don't feel at ease with ads. I will suppress this comment if people disagree.
– Damien
Nov 15 '18 at 16:13
add a comment |
1 Answer
1
active
oldest
votes
At least the following problems (some of which would be caught with warnings enabled, e.g. -Wall
):
Using uninitialized pointers, e.g.
node *b,*f; b->next = f;
. This causes undefined behavior.new
but nodelete
anywhere, therefore memory leaks will be present.
What is the connection between formula and calculation. Dynamic memory variables not connected between each other. You can`t get access from one memory block to other if variables are temporary. If after working of function you exit from function all variables are destroyed and dynamic will be memory leaks. You need to connect them.
– Timur Kukharskiy
Nov 15 '18 at 13:30
@TimurKukharskiy They are actually connected. It is kind of difficult to spot, butformula
modifies the globalt
throughstoredata
andcalculation
accessest
as well. I agree though, that this is bad design.h
andt
should be local and passed around as function arguments. The naming is also bad, they should probably behead
andtail
.
– user10605163
Nov 15 '18 at 13:33
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53320257%2fi-am-trying-to-calculate-this-continued-fraction-but-i-cant-seem-to-work-the-pr%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
At least the following problems (some of which would be caught with warnings enabled, e.g. -Wall
):
Using uninitialized pointers, e.g.
node *b,*f; b->next = f;
. This causes undefined behavior.new
but nodelete
anywhere, therefore memory leaks will be present.
What is the connection between formula and calculation. Dynamic memory variables not connected between each other. You can`t get access from one memory block to other if variables are temporary. If after working of function you exit from function all variables are destroyed and dynamic will be memory leaks. You need to connect them.
– Timur Kukharskiy
Nov 15 '18 at 13:30
@TimurKukharskiy They are actually connected. It is kind of difficult to spot, butformula
modifies the globalt
throughstoredata
andcalculation
accessest
as well. I agree though, that this is bad design.h
andt
should be local and passed around as function arguments. The naming is also bad, they should probably behead
andtail
.
– user10605163
Nov 15 '18 at 13:33
add a comment |
At least the following problems (some of which would be caught with warnings enabled, e.g. -Wall
):
Using uninitialized pointers, e.g.
node *b,*f; b->next = f;
. This causes undefined behavior.new
but nodelete
anywhere, therefore memory leaks will be present.
What is the connection between formula and calculation. Dynamic memory variables not connected between each other. You can`t get access from one memory block to other if variables are temporary. If after working of function you exit from function all variables are destroyed and dynamic will be memory leaks. You need to connect them.
– Timur Kukharskiy
Nov 15 '18 at 13:30
@TimurKukharskiy They are actually connected. It is kind of difficult to spot, butformula
modifies the globalt
throughstoredata
andcalculation
accessest
as well. I agree though, that this is bad design.h
andt
should be local and passed around as function arguments. The naming is also bad, they should probably behead
andtail
.
– user10605163
Nov 15 '18 at 13:33
add a comment |
At least the following problems (some of which would be caught with warnings enabled, e.g. -Wall
):
Using uninitialized pointers, e.g.
node *b,*f; b->next = f;
. This causes undefined behavior.new
but nodelete
anywhere, therefore memory leaks will be present.
At least the following problems (some of which would be caught with warnings enabled, e.g. -Wall
):
Using uninitialized pointers, e.g.
node *b,*f; b->next = f;
. This causes undefined behavior.new
but nodelete
anywhere, therefore memory leaks will be present.
answered Nov 15 '18 at 13:19
user10605163user10605163
2,868624
2,868624
What is the connection between formula and calculation. Dynamic memory variables not connected between each other. You can`t get access from one memory block to other if variables are temporary. If after working of function you exit from function all variables are destroyed and dynamic will be memory leaks. You need to connect them.
– Timur Kukharskiy
Nov 15 '18 at 13:30
@TimurKukharskiy They are actually connected. It is kind of difficult to spot, butformula
modifies the globalt
throughstoredata
andcalculation
accessest
as well. I agree though, that this is bad design.h
andt
should be local and passed around as function arguments. The naming is also bad, they should probably behead
andtail
.
– user10605163
Nov 15 '18 at 13:33
add a comment |
What is the connection between formula and calculation. Dynamic memory variables not connected between each other. You can`t get access from one memory block to other if variables are temporary. If after working of function you exit from function all variables are destroyed and dynamic will be memory leaks. You need to connect them.
– Timur Kukharskiy
Nov 15 '18 at 13:30
@TimurKukharskiy They are actually connected. It is kind of difficult to spot, butformula
modifies the globalt
throughstoredata
andcalculation
accessest
as well. I agree though, that this is bad design.h
andt
should be local and passed around as function arguments. The naming is also bad, they should probably behead
andtail
.
– user10605163
Nov 15 '18 at 13:33
What is the connection between formula and calculation. Dynamic memory variables not connected between each other. You can`t get access from one memory block to other if variables are temporary. If after working of function you exit from function all variables are destroyed and dynamic will be memory leaks. You need to connect them.
– Timur Kukharskiy
Nov 15 '18 at 13:30
What is the connection between formula and calculation. Dynamic memory variables not connected between each other. You can`t get access from one memory block to other if variables are temporary. If after working of function you exit from function all variables are destroyed and dynamic will be memory leaks. You need to connect them.
– Timur Kukharskiy
Nov 15 '18 at 13:30
@TimurKukharskiy They are actually connected. It is kind of difficult to spot, but
formula
modifies the global t
through storedata
and calculation
accesses t
as well. I agree though, that this is bad design. h
and t
should be local and passed around as function arguments. The naming is also bad, they should probably be head
and tail
.– user10605163
Nov 15 '18 at 13:33
@TimurKukharskiy They are actually connected. It is kind of difficult to spot, but
formula
modifies the global t
through storedata
and calculation
accesses t
as well. I agree though, that this is bad design. h
and t
should be local and passed around as function arguments. The naming is also bad, they should probably be head
and tail
.– user10605163
Nov 15 '18 at 13:33
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53320257%2fi-am-trying-to-calculate-this-continued-fraction-but-i-cant-seem-to-work-the-pr%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
hHSrDj7GNsBY7R9Li n1 IcnjtfuFSL1Me81m7 y,PaAREPfz3a
Nobody wants to read code with one-letter variable names. Least of all your instructor.
– stark
Nov 15 '18 at 14:26
I don't know if it can help, but in a recent question I posted a code that calculates continued fraction: stackoverflow.com/questions/53267626/…. I don't feel at ease with ads. I will suppress this comment if people disagree.
– Damien
Nov 15 '18 at 16:13