Python: min(None, x)
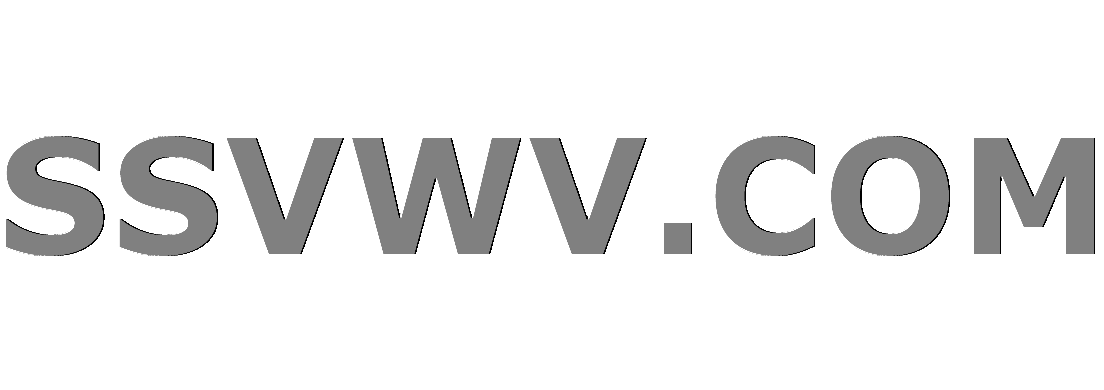
Multi tool use
I would like to perform the following:
a=max(a,3)
b=min(b,3)
However sometimes a
and b
may be None
.
I was happy to discover that in the case of max
it works out nicely, giving my required result 3
, however if b
is None
, b
remains None
...
Anyone can think of an elegant little trick to make min
return the number in case one of the arguments in None?
python python-2.x
add a comment |
I would like to perform the following:
a=max(a,3)
b=min(b,3)
However sometimes a
and b
may be None
.
I was happy to discover that in the case of max
it works out nicely, giving my required result 3
, however if b
is None
, b
remains None
...
Anyone can think of an elegant little trick to make min
return the number in case one of the arguments in None?
python python-2.x
6
It doesn't do the right thing. It happens to give the result you expect in one of two cases because the nonsensical comparision betweenNoneType
andint
returns a fixed value regardless of the integer value. In Python 3, you get aTypeError
when you do things like that (comparing types that have no meaningful ordering).
– user395760
Jun 6 '11 at 16:11
2
Seems like an inconsistency in Python, more than anything else.
– Rafe Kettler
Jun 6 '11 at 16:12
5
stackoverflow.com/questions/2214194/…
– Ben Jackson
Jun 6 '11 at 16:13
add a comment |
I would like to perform the following:
a=max(a,3)
b=min(b,3)
However sometimes a
and b
may be None
.
I was happy to discover that in the case of max
it works out nicely, giving my required result 3
, however if b
is None
, b
remains None
...
Anyone can think of an elegant little trick to make min
return the number in case one of the arguments in None?
python python-2.x
I would like to perform the following:
a=max(a,3)
b=min(b,3)
However sometimes a
and b
may be None
.
I was happy to discover that in the case of max
it works out nicely, giving my required result 3
, however if b
is None
, b
remains None
...
Anyone can think of an elegant little trick to make min
return the number in case one of the arguments in None?
python python-2.x
python python-2.x
edited Sep 14 '16 at 6:36
marshall.ward
3,14852450
3,14852450
asked Jun 6 '11 at 16:09
JonathanJonathan
35.1k73203308
35.1k73203308
6
It doesn't do the right thing. It happens to give the result you expect in one of two cases because the nonsensical comparision betweenNoneType
andint
returns a fixed value regardless of the integer value. In Python 3, you get aTypeError
when you do things like that (comparing types that have no meaningful ordering).
– user395760
Jun 6 '11 at 16:11
2
Seems like an inconsistency in Python, more than anything else.
– Rafe Kettler
Jun 6 '11 at 16:12
5
stackoverflow.com/questions/2214194/…
– Ben Jackson
Jun 6 '11 at 16:13
add a comment |
6
It doesn't do the right thing. It happens to give the result you expect in one of two cases because the nonsensical comparision betweenNoneType
andint
returns a fixed value regardless of the integer value. In Python 3, you get aTypeError
when you do things like that (comparing types that have no meaningful ordering).
– user395760
Jun 6 '11 at 16:11
2
Seems like an inconsistency in Python, more than anything else.
– Rafe Kettler
Jun 6 '11 at 16:12
5
stackoverflow.com/questions/2214194/…
– Ben Jackson
Jun 6 '11 at 16:13
6
6
It doesn't do the right thing. It happens to give the result you expect in one of two cases because the nonsensical comparision between
NoneType
and int
returns a fixed value regardless of the integer value. In Python 3, you get a TypeError
when you do things like that (comparing types that have no meaningful ordering).– user395760
Jun 6 '11 at 16:11
It doesn't do the right thing. It happens to give the result you expect in one of two cases because the nonsensical comparision between
NoneType
and int
returns a fixed value regardless of the integer value. In Python 3, you get a TypeError
when you do things like that (comparing types that have no meaningful ordering).– user395760
Jun 6 '11 at 16:11
2
2
Seems like an inconsistency in Python, more than anything else.
– Rafe Kettler
Jun 6 '11 at 16:12
Seems like an inconsistency in Python, more than anything else.
– Rafe Kettler
Jun 6 '11 at 16:12
5
5
stackoverflow.com/questions/2214194/…
– Ben Jackson
Jun 6 '11 at 16:13
stackoverflow.com/questions/2214194/…
– Ben Jackson
Jun 6 '11 at 16:13
add a comment |
8 Answers
8
active
oldest
votes
Why don't you just create a generator without None values? It's simplier and cleaner.
>>> l=[None ,3]
>>> min(i for i in l if i is not None)
3
3
No need for the list comprehension. Still +1 (in advance - seriously, please remove the brackets), it's a clean and simple solution.
– user395760
Jun 6 '11 at 16:18
Thanks, without the braces, does Python interprets it like a generator expression?
– utdemir
Jun 6 '11 at 16:22
2
Yes. The parens around generator expressions are optional if the genexpr is the sole argument to a function call.
– user395760
Jun 6 '11 at 16:27
7
One potential problem with a solution like this is that it works fine for the listed example but if you have a list with[None, None]
, themin()
function will fail because you're not giving it a valid argument.
– Kevin London
May 12 '16 at 17:29
add a comment |
A solution for the Python 3
Code:
# variable lst is your sequence
min(filter(lambda x: x is not None, lst)) if any(lst) else None
Examples:
In [3]: lst = [None, 1, None]
In [4]: min(filter(lambda x: x is not None, lst)) if any(lst) else None
Out[4]: 1
In [5]: lst = [-4, None, 11]
In [6]: min(filter(lambda x: x is not None, lst)) if any(lst) else None
Out[6]: -4
In [7]: lst = [0, 7, -79]
In [8]: min(filter(lambda x: x is not None, lst)) if any(lst) else None
Out[8]: -79
In [9]: lst = [None, None, None]
In [10]: min(filter(lambda x: x is not None, lst)) if any(lst) else None
In [11]: print(min(filter(lambda x: x is not None, lst)) if any(lst) else None)
None
Notes:
Worked in sequence presents as numbers as well as None. If all values is None min() raise exception
ValueError: min() arg is an empty sequence
This code resolve this problem at all
Pros:
- Worked if None presents in sequence
- Worked on Python 3
- max() will be work also
Cons
- Need more than one non-zero variable in the list. i.e. [0,None] fails.
- Need a variable (example lst) or need duplicate the sequence
This also works for Python 2.7. Since it also resolves the min(None) problem of the other solutions, it gets my +1
– UlrichWuenstel
Mar 23 '18 at 15:23
add a comment |
Here is an inline decorator that you can use to filter out None values that might be passed to a function:
noNones = lambda fn : lambda *args : fn(a for a in args if a is not None)
print noNones(min)(None, 3)
print noNones(max)(None, 3)
prints:
3
3
add a comment |
def max_none(a, b):
if a is None:
a = float('-inf')
if b is None:
b = float('-inf')
return max(a, b)
def min_none(a, b):
if a is None:
a = float('inf')
if b is None:
b = float('inf')
return min(a, b)
max_none(None, 3)
max_none(3, None)
min_none(None, 3)
min_none(3, None)
Note that this only works if the default is 0. Passing 0 instead ofNone
still triggers passing the default but doesn't change the value since the default is 0 (and0 == 0.0 == 0j
).
– user395760
Jun 6 '11 at 16:16
1
The OP said max was not a problem. Also what happens if b is negative ?
– Pavan Yalamanchili
Jun 6 '11 at 16:16
Modified answer.
– Steve Mayne
Jun 7 '11 at 8:52
add a comment |
You can use an inline if
and an infinity as the default, as that will work for any value:
a = max(a if a is not None else float('-inf'), 3)
b = min(b if b is not None else float('inf'), 3)
a = 0
breaks this.
– user395760
Jun 6 '11 at 16:14
Okay, then I'll make it a bit more explicit
– Blender
Jun 6 '11 at 16:15
Then this will passTrue
on occasion ;)
– user395760
Jun 6 '11 at 16:17
See my other edit...
– Blender
Jun 6 '11 at 16:19
add a comment |
My solution for Python 3 (3.4 and greater):
min((x for x in lst if x is not None), default=None)
max((x for x in lst if x is not None), default=None)
add a comment |
a=max(a,3) if a is not None else 3
b=min(b,3) if b is not None else 3
add a comment |
@utdemir's answer works great for the provided example but would raise an error in some scenarios.
One issue that comes up is if you have a list with only None
values. If you provide an empty sequence to min()
, it will raise an error:
>>> mylist = [None, None]
>>> min(value for value in mylist if value)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: min() arg is an empty sequence
As such, this snippet would prevent the error:
def find_minimum(minimums):
potential_mins = (value for value in minimums if value is not None)
if potential_mins:
return min(potential_mins)
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f6254871%2fpython-minnone-x%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
8 Answers
8
active
oldest
votes
8 Answers
8
active
oldest
votes
active
oldest
votes
active
oldest
votes
Why don't you just create a generator without None values? It's simplier and cleaner.
>>> l=[None ,3]
>>> min(i for i in l if i is not None)
3
3
No need for the list comprehension. Still +1 (in advance - seriously, please remove the brackets), it's a clean and simple solution.
– user395760
Jun 6 '11 at 16:18
Thanks, without the braces, does Python interprets it like a generator expression?
– utdemir
Jun 6 '11 at 16:22
2
Yes. The parens around generator expressions are optional if the genexpr is the sole argument to a function call.
– user395760
Jun 6 '11 at 16:27
7
One potential problem with a solution like this is that it works fine for the listed example but if you have a list with[None, None]
, themin()
function will fail because you're not giving it a valid argument.
– Kevin London
May 12 '16 at 17:29
add a comment |
Why don't you just create a generator without None values? It's simplier and cleaner.
>>> l=[None ,3]
>>> min(i for i in l if i is not None)
3
3
No need for the list comprehension. Still +1 (in advance - seriously, please remove the brackets), it's a clean and simple solution.
– user395760
Jun 6 '11 at 16:18
Thanks, without the braces, does Python interprets it like a generator expression?
– utdemir
Jun 6 '11 at 16:22
2
Yes. The parens around generator expressions are optional if the genexpr is the sole argument to a function call.
– user395760
Jun 6 '11 at 16:27
7
One potential problem with a solution like this is that it works fine for the listed example but if you have a list with[None, None]
, themin()
function will fail because you're not giving it a valid argument.
– Kevin London
May 12 '16 at 17:29
add a comment |
Why don't you just create a generator without None values? It's simplier and cleaner.
>>> l=[None ,3]
>>> min(i for i in l if i is not None)
3
Why don't you just create a generator without None values? It's simplier and cleaner.
>>> l=[None ,3]
>>> min(i for i in l if i is not None)
3
answered Jun 6 '11 at 16:16
utdemirutdemir
18.6k84475
18.6k84475
3
No need for the list comprehension. Still +1 (in advance - seriously, please remove the brackets), it's a clean and simple solution.
– user395760
Jun 6 '11 at 16:18
Thanks, without the braces, does Python interprets it like a generator expression?
– utdemir
Jun 6 '11 at 16:22
2
Yes. The parens around generator expressions are optional if the genexpr is the sole argument to a function call.
– user395760
Jun 6 '11 at 16:27
7
One potential problem with a solution like this is that it works fine for the listed example but if you have a list with[None, None]
, themin()
function will fail because you're not giving it a valid argument.
– Kevin London
May 12 '16 at 17:29
add a comment |
3
No need for the list comprehension. Still +1 (in advance - seriously, please remove the brackets), it's a clean and simple solution.
– user395760
Jun 6 '11 at 16:18
Thanks, without the braces, does Python interprets it like a generator expression?
– utdemir
Jun 6 '11 at 16:22
2
Yes. The parens around generator expressions are optional if the genexpr is the sole argument to a function call.
– user395760
Jun 6 '11 at 16:27
7
One potential problem with a solution like this is that it works fine for the listed example but if you have a list with[None, None]
, themin()
function will fail because you're not giving it a valid argument.
– Kevin London
May 12 '16 at 17:29
3
3
No need for the list comprehension. Still +1 (in advance - seriously, please remove the brackets), it's a clean and simple solution.
– user395760
Jun 6 '11 at 16:18
No need for the list comprehension. Still +1 (in advance - seriously, please remove the brackets), it's a clean and simple solution.
– user395760
Jun 6 '11 at 16:18
Thanks, without the braces, does Python interprets it like a generator expression?
– utdemir
Jun 6 '11 at 16:22
Thanks, without the braces, does Python interprets it like a generator expression?
– utdemir
Jun 6 '11 at 16:22
2
2
Yes. The parens around generator expressions are optional if the genexpr is the sole argument to a function call.
– user395760
Jun 6 '11 at 16:27
Yes. The parens around generator expressions are optional if the genexpr is the sole argument to a function call.
– user395760
Jun 6 '11 at 16:27
7
7
One potential problem with a solution like this is that it works fine for the listed example but if you have a list with
[None, None]
, the min()
function will fail because you're not giving it a valid argument.– Kevin London
May 12 '16 at 17:29
One potential problem with a solution like this is that it works fine for the listed example but if you have a list with
[None, None]
, the min()
function will fail because you're not giving it a valid argument.– Kevin London
May 12 '16 at 17:29
add a comment |
A solution for the Python 3
Code:
# variable lst is your sequence
min(filter(lambda x: x is not None, lst)) if any(lst) else None
Examples:
In [3]: lst = [None, 1, None]
In [4]: min(filter(lambda x: x is not None, lst)) if any(lst) else None
Out[4]: 1
In [5]: lst = [-4, None, 11]
In [6]: min(filter(lambda x: x is not None, lst)) if any(lst) else None
Out[6]: -4
In [7]: lst = [0, 7, -79]
In [8]: min(filter(lambda x: x is not None, lst)) if any(lst) else None
Out[8]: -79
In [9]: lst = [None, None, None]
In [10]: min(filter(lambda x: x is not None, lst)) if any(lst) else None
In [11]: print(min(filter(lambda x: x is not None, lst)) if any(lst) else None)
None
Notes:
Worked in sequence presents as numbers as well as None. If all values is None min() raise exception
ValueError: min() arg is an empty sequence
This code resolve this problem at all
Pros:
- Worked if None presents in sequence
- Worked on Python 3
- max() will be work also
Cons
- Need more than one non-zero variable in the list. i.e. [0,None] fails.
- Need a variable (example lst) or need duplicate the sequence
This also works for Python 2.7. Since it also resolves the min(None) problem of the other solutions, it gets my +1
– UlrichWuenstel
Mar 23 '18 at 15:23
add a comment |
A solution for the Python 3
Code:
# variable lst is your sequence
min(filter(lambda x: x is not None, lst)) if any(lst) else None
Examples:
In [3]: lst = [None, 1, None]
In [4]: min(filter(lambda x: x is not None, lst)) if any(lst) else None
Out[4]: 1
In [5]: lst = [-4, None, 11]
In [6]: min(filter(lambda x: x is not None, lst)) if any(lst) else None
Out[6]: -4
In [7]: lst = [0, 7, -79]
In [8]: min(filter(lambda x: x is not None, lst)) if any(lst) else None
Out[8]: -79
In [9]: lst = [None, None, None]
In [10]: min(filter(lambda x: x is not None, lst)) if any(lst) else None
In [11]: print(min(filter(lambda x: x is not None, lst)) if any(lst) else None)
None
Notes:
Worked in sequence presents as numbers as well as None. If all values is None min() raise exception
ValueError: min() arg is an empty sequence
This code resolve this problem at all
Pros:
- Worked if None presents in sequence
- Worked on Python 3
- max() will be work also
Cons
- Need more than one non-zero variable in the list. i.e. [0,None] fails.
- Need a variable (example lst) or need duplicate the sequence
This also works for Python 2.7. Since it also resolves the min(None) problem of the other solutions, it gets my +1
– UlrichWuenstel
Mar 23 '18 at 15:23
add a comment |
A solution for the Python 3
Code:
# variable lst is your sequence
min(filter(lambda x: x is not None, lst)) if any(lst) else None
Examples:
In [3]: lst = [None, 1, None]
In [4]: min(filter(lambda x: x is not None, lst)) if any(lst) else None
Out[4]: 1
In [5]: lst = [-4, None, 11]
In [6]: min(filter(lambda x: x is not None, lst)) if any(lst) else None
Out[6]: -4
In [7]: lst = [0, 7, -79]
In [8]: min(filter(lambda x: x is not None, lst)) if any(lst) else None
Out[8]: -79
In [9]: lst = [None, None, None]
In [10]: min(filter(lambda x: x is not None, lst)) if any(lst) else None
In [11]: print(min(filter(lambda x: x is not None, lst)) if any(lst) else None)
None
Notes:
Worked in sequence presents as numbers as well as None. If all values is None min() raise exception
ValueError: min() arg is an empty sequence
This code resolve this problem at all
Pros:
- Worked if None presents in sequence
- Worked on Python 3
- max() will be work also
Cons
- Need more than one non-zero variable in the list. i.e. [0,None] fails.
- Need a variable (example lst) or need duplicate the sequence
A solution for the Python 3
Code:
# variable lst is your sequence
min(filter(lambda x: x is not None, lst)) if any(lst) else None
Examples:
In [3]: lst = [None, 1, None]
In [4]: min(filter(lambda x: x is not None, lst)) if any(lst) else None
Out[4]: 1
In [5]: lst = [-4, None, 11]
In [6]: min(filter(lambda x: x is not None, lst)) if any(lst) else None
Out[6]: -4
In [7]: lst = [0, 7, -79]
In [8]: min(filter(lambda x: x is not None, lst)) if any(lst) else None
Out[8]: -79
In [9]: lst = [None, None, None]
In [10]: min(filter(lambda x: x is not None, lst)) if any(lst) else None
In [11]: print(min(filter(lambda x: x is not None, lst)) if any(lst) else None)
None
Notes:
Worked in sequence presents as numbers as well as None. If all values is None min() raise exception
ValueError: min() arg is an empty sequence
This code resolve this problem at all
Pros:
- Worked if None presents in sequence
- Worked on Python 3
- max() will be work also
Cons
- Need more than one non-zero variable in the list. i.e. [0,None] fails.
- Need a variable (example lst) or need duplicate the sequence
edited Nov 13 '18 at 5:09
André Guerra
27110
27110
answered Nov 6 '16 at 8:35
Seti VolkylanySeti Volkylany
1,76211625
1,76211625
This also works for Python 2.7. Since it also resolves the min(None) problem of the other solutions, it gets my +1
– UlrichWuenstel
Mar 23 '18 at 15:23
add a comment |
This also works for Python 2.7. Since it also resolves the min(None) problem of the other solutions, it gets my +1
– UlrichWuenstel
Mar 23 '18 at 15:23
This also works for Python 2.7. Since it also resolves the min(None) problem of the other solutions, it gets my +1
– UlrichWuenstel
Mar 23 '18 at 15:23
This also works for Python 2.7. Since it also resolves the min(None) problem of the other solutions, it gets my +1
– UlrichWuenstel
Mar 23 '18 at 15:23
add a comment |
Here is an inline decorator that you can use to filter out None values that might be passed to a function:
noNones = lambda fn : lambda *args : fn(a for a in args if a is not None)
print noNones(min)(None, 3)
print noNones(max)(None, 3)
prints:
3
3
add a comment |
Here is an inline decorator that you can use to filter out None values that might be passed to a function:
noNones = lambda fn : lambda *args : fn(a for a in args if a is not None)
print noNones(min)(None, 3)
print noNones(max)(None, 3)
prints:
3
3
add a comment |
Here is an inline decorator that you can use to filter out None values that might be passed to a function:
noNones = lambda fn : lambda *args : fn(a for a in args if a is not None)
print noNones(min)(None, 3)
print noNones(max)(None, 3)
prints:
3
3
Here is an inline decorator that you can use to filter out None values that might be passed to a function:
noNones = lambda fn : lambda *args : fn(a for a in args if a is not None)
print noNones(min)(None, 3)
print noNones(max)(None, 3)
prints:
3
3
answered Jun 6 '11 at 17:25
PaulMcGPaulMcG
45.9k967111
45.9k967111
add a comment |
add a comment |
def max_none(a, b):
if a is None:
a = float('-inf')
if b is None:
b = float('-inf')
return max(a, b)
def min_none(a, b):
if a is None:
a = float('inf')
if b is None:
b = float('inf')
return min(a, b)
max_none(None, 3)
max_none(3, None)
min_none(None, 3)
min_none(3, None)
Note that this only works if the default is 0. Passing 0 instead ofNone
still triggers passing the default but doesn't change the value since the default is 0 (and0 == 0.0 == 0j
).
– user395760
Jun 6 '11 at 16:16
1
The OP said max was not a problem. Also what happens if b is negative ?
– Pavan Yalamanchili
Jun 6 '11 at 16:16
Modified answer.
– Steve Mayne
Jun 7 '11 at 8:52
add a comment |
def max_none(a, b):
if a is None:
a = float('-inf')
if b is None:
b = float('-inf')
return max(a, b)
def min_none(a, b):
if a is None:
a = float('inf')
if b is None:
b = float('inf')
return min(a, b)
max_none(None, 3)
max_none(3, None)
min_none(None, 3)
min_none(3, None)
Note that this only works if the default is 0. Passing 0 instead ofNone
still triggers passing the default but doesn't change the value since the default is 0 (and0 == 0.0 == 0j
).
– user395760
Jun 6 '11 at 16:16
1
The OP said max was not a problem. Also what happens if b is negative ?
– Pavan Yalamanchili
Jun 6 '11 at 16:16
Modified answer.
– Steve Mayne
Jun 7 '11 at 8:52
add a comment |
def max_none(a, b):
if a is None:
a = float('-inf')
if b is None:
b = float('-inf')
return max(a, b)
def min_none(a, b):
if a is None:
a = float('inf')
if b is None:
b = float('inf')
return min(a, b)
max_none(None, 3)
max_none(3, None)
min_none(None, 3)
min_none(3, None)
def max_none(a, b):
if a is None:
a = float('-inf')
if b is None:
b = float('-inf')
return max(a, b)
def min_none(a, b):
if a is None:
a = float('inf')
if b is None:
b = float('inf')
return min(a, b)
max_none(None, 3)
max_none(3, None)
min_none(None, 3)
min_none(3, None)
edited Apr 12 '12 at 9:01
answered Jun 6 '11 at 16:11
Steve MayneSteve Mayne
16.2k43644
16.2k43644
Note that this only works if the default is 0. Passing 0 instead ofNone
still triggers passing the default but doesn't change the value since the default is 0 (and0 == 0.0 == 0j
).
– user395760
Jun 6 '11 at 16:16
1
The OP said max was not a problem. Also what happens if b is negative ?
– Pavan Yalamanchili
Jun 6 '11 at 16:16
Modified answer.
– Steve Mayne
Jun 7 '11 at 8:52
add a comment |
Note that this only works if the default is 0. Passing 0 instead ofNone
still triggers passing the default but doesn't change the value since the default is 0 (and0 == 0.0 == 0j
).
– user395760
Jun 6 '11 at 16:16
1
The OP said max was not a problem. Also what happens if b is negative ?
– Pavan Yalamanchili
Jun 6 '11 at 16:16
Modified answer.
– Steve Mayne
Jun 7 '11 at 8:52
Note that this only works if the default is 0. Passing 0 instead of
None
still triggers passing the default but doesn't change the value since the default is 0 (and 0 == 0.0 == 0j
).– user395760
Jun 6 '11 at 16:16
Note that this only works if the default is 0. Passing 0 instead of
None
still triggers passing the default but doesn't change the value since the default is 0 (and 0 == 0.0 == 0j
).– user395760
Jun 6 '11 at 16:16
1
1
The OP said max was not a problem. Also what happens if b is negative ?
– Pavan Yalamanchili
Jun 6 '11 at 16:16
The OP said max was not a problem. Also what happens if b is negative ?
– Pavan Yalamanchili
Jun 6 '11 at 16:16
Modified answer.
– Steve Mayne
Jun 7 '11 at 8:52
Modified answer.
– Steve Mayne
Jun 7 '11 at 8:52
add a comment |
You can use an inline if
and an infinity as the default, as that will work for any value:
a = max(a if a is not None else float('-inf'), 3)
b = min(b if b is not None else float('inf'), 3)
a = 0
breaks this.
– user395760
Jun 6 '11 at 16:14
Okay, then I'll make it a bit more explicit
– Blender
Jun 6 '11 at 16:15
Then this will passTrue
on occasion ;)
– user395760
Jun 6 '11 at 16:17
See my other edit...
– Blender
Jun 6 '11 at 16:19
add a comment |
You can use an inline if
and an infinity as the default, as that will work for any value:
a = max(a if a is not None else float('-inf'), 3)
b = min(b if b is not None else float('inf'), 3)
a = 0
breaks this.
– user395760
Jun 6 '11 at 16:14
Okay, then I'll make it a bit more explicit
– Blender
Jun 6 '11 at 16:15
Then this will passTrue
on occasion ;)
– user395760
Jun 6 '11 at 16:17
See my other edit...
– Blender
Jun 6 '11 at 16:19
add a comment |
You can use an inline if
and an infinity as the default, as that will work for any value:
a = max(a if a is not None else float('-inf'), 3)
b = min(b if b is not None else float('inf'), 3)
You can use an inline if
and an infinity as the default, as that will work for any value:
a = max(a if a is not None else float('-inf'), 3)
b = min(b if b is not None else float('inf'), 3)
edited Jun 6 '11 at 16:19
answered Jun 6 '11 at 16:14
BlenderBlender
206k36334400
206k36334400
a = 0
breaks this.
– user395760
Jun 6 '11 at 16:14
Okay, then I'll make it a bit more explicit
– Blender
Jun 6 '11 at 16:15
Then this will passTrue
on occasion ;)
– user395760
Jun 6 '11 at 16:17
See my other edit...
– Blender
Jun 6 '11 at 16:19
add a comment |
a = 0
breaks this.
– user395760
Jun 6 '11 at 16:14
Okay, then I'll make it a bit more explicit
– Blender
Jun 6 '11 at 16:15
Then this will passTrue
on occasion ;)
– user395760
Jun 6 '11 at 16:17
See my other edit...
– Blender
Jun 6 '11 at 16:19
a = 0
breaks this.– user395760
Jun 6 '11 at 16:14
a = 0
breaks this.– user395760
Jun 6 '11 at 16:14
Okay, then I'll make it a bit more explicit
– Blender
Jun 6 '11 at 16:15
Okay, then I'll make it a bit more explicit
– Blender
Jun 6 '11 at 16:15
Then this will pass
True
on occasion ;)– user395760
Jun 6 '11 at 16:17
Then this will pass
True
on occasion ;)– user395760
Jun 6 '11 at 16:17
See my other edit...
– Blender
Jun 6 '11 at 16:19
See my other edit...
– Blender
Jun 6 '11 at 16:19
add a comment |
My solution for Python 3 (3.4 and greater):
min((x for x in lst if x is not None), default=None)
max((x for x in lst if x is not None), default=None)
add a comment |
My solution for Python 3 (3.4 and greater):
min((x for x in lst if x is not None), default=None)
max((x for x in lst if x is not None), default=None)
add a comment |
My solution for Python 3 (3.4 and greater):
min((x for x in lst if x is not None), default=None)
max((x for x in lst if x is not None), default=None)
My solution for Python 3 (3.4 and greater):
min((x for x in lst if x is not None), default=None)
max((x for x in lst if x is not None), default=None)
answered Nov 8 '18 at 16:32
R1tschYR1tschY
1,5531424
1,5531424
add a comment |
add a comment |
a=max(a,3) if a is not None else 3
b=min(b,3) if b is not None else 3
add a comment |
a=max(a,3) if a is not None else 3
b=min(b,3) if b is not None else 3
add a comment |
a=max(a,3) if a is not None else 3
b=min(b,3) if b is not None else 3
a=max(a,3) if a is not None else 3
b=min(b,3) if b is not None else 3
edited Apr 28 '15 at 0:56
answered Apr 27 '15 at 20:27


Steve MitchellSteve Mitchell
48968
48968
add a comment |
add a comment |
@utdemir's answer works great for the provided example but would raise an error in some scenarios.
One issue that comes up is if you have a list with only None
values. If you provide an empty sequence to min()
, it will raise an error:
>>> mylist = [None, None]
>>> min(value for value in mylist if value)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: min() arg is an empty sequence
As such, this snippet would prevent the error:
def find_minimum(minimums):
potential_mins = (value for value in minimums if value is not None)
if potential_mins:
return min(potential_mins)
add a comment |
@utdemir's answer works great for the provided example but would raise an error in some scenarios.
One issue that comes up is if you have a list with only None
values. If you provide an empty sequence to min()
, it will raise an error:
>>> mylist = [None, None]
>>> min(value for value in mylist if value)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: min() arg is an empty sequence
As such, this snippet would prevent the error:
def find_minimum(minimums):
potential_mins = (value for value in minimums if value is not None)
if potential_mins:
return min(potential_mins)
add a comment |
@utdemir's answer works great for the provided example but would raise an error in some scenarios.
One issue that comes up is if you have a list with only None
values. If you provide an empty sequence to min()
, it will raise an error:
>>> mylist = [None, None]
>>> min(value for value in mylist if value)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: min() arg is an empty sequence
As such, this snippet would prevent the error:
def find_minimum(minimums):
potential_mins = (value for value in minimums if value is not None)
if potential_mins:
return min(potential_mins)
@utdemir's answer works great for the provided example but would raise an error in some scenarios.
One issue that comes up is if you have a list with only None
values. If you provide an empty sequence to min()
, it will raise an error:
>>> mylist = [None, None]
>>> min(value for value in mylist if value)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: min() arg is an empty sequence
As such, this snippet would prevent the error:
def find_minimum(minimums):
potential_mins = (value for value in minimums if value is not None)
if potential_mins:
return min(potential_mins)
edited May 12 '16 at 17:42
answered May 12 '16 at 17:35
Kevin LondonKevin London
3,19711225
3,19711225
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f6254871%2fpython-minnone-x%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
OGnJSi p0LBYH i8ac5wE5ySNjO rg 4X N2Imlx9NpjaprJt6i
6
It doesn't do the right thing. It happens to give the result you expect in one of two cases because the nonsensical comparision between
NoneType
andint
returns a fixed value regardless of the integer value. In Python 3, you get aTypeError
when you do things like that (comparing types that have no meaningful ordering).– user395760
Jun 6 '11 at 16:11
2
Seems like an inconsistency in Python, more than anything else.
– Rafe Kettler
Jun 6 '11 at 16:12
5
stackoverflow.com/questions/2214194/…
– Ben Jackson
Jun 6 '11 at 16:13