How to get specific properties from a JSON object from HTTP request using URLSession
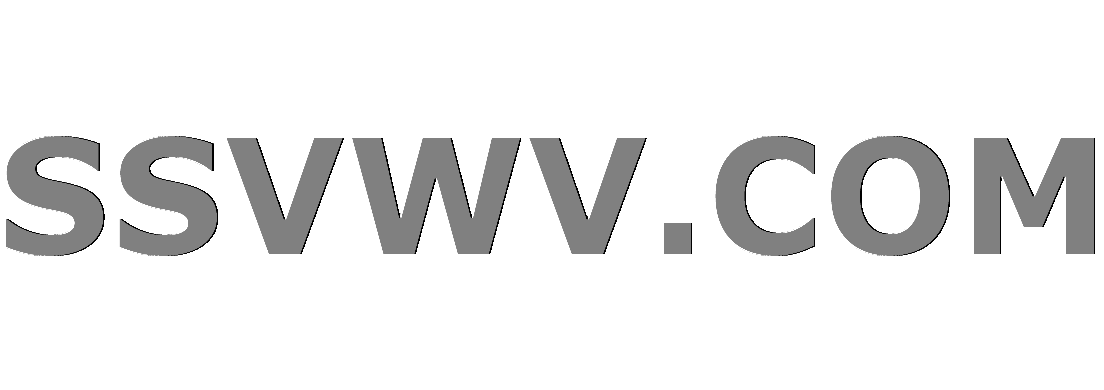
Multi tool use
With the following code I'm able to effectively get a JSON object, what I'm not sure how to do is retrieve the specific properties from the object.
Swift Code
@IBAction func testing(_ sender: Any) {
let url = URL(string: "http://example.com/cars/mustang")
let task = URLSession.shared.dataTask(with: url!) { data, response, error in
guard error == nil else {
print(error!)
return
}
guard let data = data else {
print("Data is empty")
return
}
let json = try! JSONSerialization.jsonObject(with: data, options: )
print(json)
}
task.resume()
}
Here is what I see when I run the above code...
Output - JSON Object
(
{
color = "red";
engine = "5.0";
}
)
How can I get just the property color?
Thanks
swift nsurlsession
|
show 1 more comment
With the following code I'm able to effectively get a JSON object, what I'm not sure how to do is retrieve the specific properties from the object.
Swift Code
@IBAction func testing(_ sender: Any) {
let url = URL(string: "http://example.com/cars/mustang")
let task = URLSession.shared.dataTask(with: url!) { data, response, error in
guard error == nil else {
print(error!)
return
}
guard let data = data else {
print("Data is empty")
return
}
let json = try! JSONSerialization.jsonObject(with: data, options: )
print(json)
}
task.resume()
}
Here is what I see when I run the above code...
Output - JSON Object
(
{
color = "red";
engine = "5.0";
}
)
How can I get just the property color?
Thanks
swift nsurlsession
3
json
is an array of dictionary. Access your data accordingly.
– rmaddy
Nov 12 '18 at 23:46
Hmm, I cannot figure it out. Can you please direct me to where I can find information on how to get items from an array of dictionaries in Swift?
– fs_tigre
Nov 13 '18 at 2:23
2
The Collection Types chapter of the Swift book is a good start.
– rmaddy
Nov 13 '18 at 2:24
1
Try this stackoverflow.com/questions/38743156/…
– Alex Bailey
Nov 13 '18 at 5:01
Please do not put the answer in your question. Either accept an answer below if it best helped or post your own showing your chosen solution.
– rmaddy
Nov 14 '18 at 0:27
|
show 1 more comment
With the following code I'm able to effectively get a JSON object, what I'm not sure how to do is retrieve the specific properties from the object.
Swift Code
@IBAction func testing(_ sender: Any) {
let url = URL(string: "http://example.com/cars/mustang")
let task = URLSession.shared.dataTask(with: url!) { data, response, error in
guard error == nil else {
print(error!)
return
}
guard let data = data else {
print("Data is empty")
return
}
let json = try! JSONSerialization.jsonObject(with: data, options: )
print(json)
}
task.resume()
}
Here is what I see when I run the above code...
Output - JSON Object
(
{
color = "red";
engine = "5.0";
}
)
How can I get just the property color?
Thanks
swift nsurlsession
With the following code I'm able to effectively get a JSON object, what I'm not sure how to do is retrieve the specific properties from the object.
Swift Code
@IBAction func testing(_ sender: Any) {
let url = URL(string: "http://example.com/cars/mustang")
let task = URLSession.shared.dataTask(with: url!) { data, response, error in
guard error == nil else {
print(error!)
return
}
guard let data = data else {
print("Data is empty")
return
}
let json = try! JSONSerialization.jsonObject(with: data, options: )
print(json)
}
task.resume()
}
Here is what I see when I run the above code...
Output - JSON Object
(
{
color = "red";
engine = "5.0";
}
)
How can I get just the property color?
Thanks
swift nsurlsession
swift nsurlsession
edited Nov 14 '18 at 0:26


rmaddy
239k27311376
239k27311376
asked Nov 12 '18 at 23:45
fs_tigrefs_tigre
4,57273972
4,57273972
3
json
is an array of dictionary. Access your data accordingly.
– rmaddy
Nov 12 '18 at 23:46
Hmm, I cannot figure it out. Can you please direct me to where I can find information on how to get items from an array of dictionaries in Swift?
– fs_tigre
Nov 13 '18 at 2:23
2
The Collection Types chapter of the Swift book is a good start.
– rmaddy
Nov 13 '18 at 2:24
1
Try this stackoverflow.com/questions/38743156/…
– Alex Bailey
Nov 13 '18 at 5:01
Please do not put the answer in your question. Either accept an answer below if it best helped or post your own showing your chosen solution.
– rmaddy
Nov 14 '18 at 0:27
|
show 1 more comment
3
json
is an array of dictionary. Access your data accordingly.
– rmaddy
Nov 12 '18 at 23:46
Hmm, I cannot figure it out. Can you please direct me to where I can find information on how to get items from an array of dictionaries in Swift?
– fs_tigre
Nov 13 '18 at 2:23
2
The Collection Types chapter of the Swift book is a good start.
– rmaddy
Nov 13 '18 at 2:24
1
Try this stackoverflow.com/questions/38743156/…
– Alex Bailey
Nov 13 '18 at 5:01
Please do not put the answer in your question. Either accept an answer below if it best helped or post your own showing your chosen solution.
– rmaddy
Nov 14 '18 at 0:27
3
3
json
is an array of dictionary. Access your data accordingly.– rmaddy
Nov 12 '18 at 23:46
json
is an array of dictionary. Access your data accordingly.– rmaddy
Nov 12 '18 at 23:46
Hmm, I cannot figure it out. Can you please direct me to where I can find information on how to get items from an array of dictionaries in Swift?
– fs_tigre
Nov 13 '18 at 2:23
Hmm, I cannot figure it out. Can you please direct me to where I can find information on how to get items from an array of dictionaries in Swift?
– fs_tigre
Nov 13 '18 at 2:23
2
2
The Collection Types chapter of the Swift book is a good start.
– rmaddy
Nov 13 '18 at 2:24
The Collection Types chapter of the Swift book is a good start.
– rmaddy
Nov 13 '18 at 2:24
1
1
Try this stackoverflow.com/questions/38743156/…
– Alex Bailey
Nov 13 '18 at 5:01
Try this stackoverflow.com/questions/38743156/…
– Alex Bailey
Nov 13 '18 at 5:01
Please do not put the answer in your question. Either accept an answer below if it best helped or post your own showing your chosen solution.
– rmaddy
Nov 14 '18 at 0:27
Please do not put the answer in your question. Either accept an answer below if it best helped or post your own showing your chosen solution.
– rmaddy
Nov 14 '18 at 0:27
|
show 1 more comment
2 Answers
2
active
oldest
votes
Create a class which confirm the decodable protocol; CarInfo for example in your case
class CarInfo: Decodable
Create attributes of the class
var color: String
var engine: String
Create JSON key enum which inherits from CodingKey
enum CarInfoCodingKey: String, CodingKey {
case color
case engine
}
implement init
required init(from decoder: Decoder) throws
the class will be
class CarInfo: Decodable {
var color: String
var engine: String
enum CarInfoCodingKey: String, CodingKey {
case color
case engine
}
public init(from decoder: Decodabler) throws {
let container = try decoder.container(keyedBy: CarInfoCodingKey.self)
self.color = try container.decode(String.self, forKey: .color)
self.engine = try contaire.decode(String.self, forKey: .engine)
}
}
call decoder
let carinfo = try JsonDecoder().decode(CarInfo.self, from: jsonData)
Is this the simplest way to do it?
– fs_tigre
Nov 13 '18 at 2:18
1
@fs_tigre You only need one or two more lines in the code you posted in your question. Using this answer's solution is just a different solution over usingJSONSerialization
.
– rmaddy
Nov 13 '18 at 3:09
@rmaddy - Thank you for the clarification, I edited my original question with my solution. @-Mohamed Mahdi Allani - Thanks a lot for demonstrating other methods, I when with the simples approach.
– fs_tigre
Nov 13 '18 at 23:13
add a comment |
Here is how I did it...
@IBAction func testing(_ sender: Any) {
let url = URL(string: "http://example.com/cars/mustang")
let task = URLSession.shared.dataTask(with: url!) { data, response, error in
guard error == nil else {
print(error!)
return
}
guard let data = data else {
print("Data is empty")
return
}
let json = try! JSONSerialization.jsonObject(with: data, options: )
guard let jsonArray = json as? [[String: String]] else {
return
}
let mustangColor = jsonArray[0]["color"]
print(mustangColor!)
}
task.resume()
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53271730%2fhow-to-get-specific-properties-from-a-json-object-from-http-request-using-urlses%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Create a class which confirm the decodable protocol; CarInfo for example in your case
class CarInfo: Decodable
Create attributes of the class
var color: String
var engine: String
Create JSON key enum which inherits from CodingKey
enum CarInfoCodingKey: String, CodingKey {
case color
case engine
}
implement init
required init(from decoder: Decoder) throws
the class will be
class CarInfo: Decodable {
var color: String
var engine: String
enum CarInfoCodingKey: String, CodingKey {
case color
case engine
}
public init(from decoder: Decodabler) throws {
let container = try decoder.container(keyedBy: CarInfoCodingKey.self)
self.color = try container.decode(String.self, forKey: .color)
self.engine = try contaire.decode(String.self, forKey: .engine)
}
}
call decoder
let carinfo = try JsonDecoder().decode(CarInfo.self, from: jsonData)
Is this the simplest way to do it?
– fs_tigre
Nov 13 '18 at 2:18
1
@fs_tigre You only need one or two more lines in the code you posted in your question. Using this answer's solution is just a different solution over usingJSONSerialization
.
– rmaddy
Nov 13 '18 at 3:09
@rmaddy - Thank you for the clarification, I edited my original question with my solution. @-Mohamed Mahdi Allani - Thanks a lot for demonstrating other methods, I when with the simples approach.
– fs_tigre
Nov 13 '18 at 23:13
add a comment |
Create a class which confirm the decodable protocol; CarInfo for example in your case
class CarInfo: Decodable
Create attributes of the class
var color: String
var engine: String
Create JSON key enum which inherits from CodingKey
enum CarInfoCodingKey: String, CodingKey {
case color
case engine
}
implement init
required init(from decoder: Decoder) throws
the class will be
class CarInfo: Decodable {
var color: String
var engine: String
enum CarInfoCodingKey: String, CodingKey {
case color
case engine
}
public init(from decoder: Decodabler) throws {
let container = try decoder.container(keyedBy: CarInfoCodingKey.self)
self.color = try container.decode(String.self, forKey: .color)
self.engine = try contaire.decode(String.self, forKey: .engine)
}
}
call decoder
let carinfo = try JsonDecoder().decode(CarInfo.self, from: jsonData)
Is this the simplest way to do it?
– fs_tigre
Nov 13 '18 at 2:18
1
@fs_tigre You only need one or two more lines in the code you posted in your question. Using this answer's solution is just a different solution over usingJSONSerialization
.
– rmaddy
Nov 13 '18 at 3:09
@rmaddy - Thank you for the clarification, I edited my original question with my solution. @-Mohamed Mahdi Allani - Thanks a lot for demonstrating other methods, I when with the simples approach.
– fs_tigre
Nov 13 '18 at 23:13
add a comment |
Create a class which confirm the decodable protocol; CarInfo for example in your case
class CarInfo: Decodable
Create attributes of the class
var color: String
var engine: String
Create JSON key enum which inherits from CodingKey
enum CarInfoCodingKey: String, CodingKey {
case color
case engine
}
implement init
required init(from decoder: Decoder) throws
the class will be
class CarInfo: Decodable {
var color: String
var engine: String
enum CarInfoCodingKey: String, CodingKey {
case color
case engine
}
public init(from decoder: Decodabler) throws {
let container = try decoder.container(keyedBy: CarInfoCodingKey.self)
self.color = try container.decode(String.self, forKey: .color)
self.engine = try contaire.decode(String.self, forKey: .engine)
}
}
call decoder
let carinfo = try JsonDecoder().decode(CarInfo.self, from: jsonData)
Create a class which confirm the decodable protocol; CarInfo for example in your case
class CarInfo: Decodable
Create attributes of the class
var color: String
var engine: String
Create JSON key enum which inherits from CodingKey
enum CarInfoCodingKey: String, CodingKey {
case color
case engine
}
implement init
required init(from decoder: Decoder) throws
the class will be
class CarInfo: Decodable {
var color: String
var engine: String
enum CarInfoCodingKey: String, CodingKey {
case color
case engine
}
public init(from decoder: Decodabler) throws {
let container = try decoder.container(keyedBy: CarInfoCodingKey.self)
self.color = try container.decode(String.self, forKey: .color)
self.engine = try contaire.decode(String.self, forKey: .engine)
}
}
call decoder
let carinfo = try JsonDecoder().decode(CarInfo.self, from: jsonData)
answered Nov 12 '18 at 23:59


Mohamed Mahdi AllaniMohamed Mahdi Allani
213
213
Is this the simplest way to do it?
– fs_tigre
Nov 13 '18 at 2:18
1
@fs_tigre You only need one or two more lines in the code you posted in your question. Using this answer's solution is just a different solution over usingJSONSerialization
.
– rmaddy
Nov 13 '18 at 3:09
@rmaddy - Thank you for the clarification, I edited my original question with my solution. @-Mohamed Mahdi Allani - Thanks a lot for demonstrating other methods, I when with the simples approach.
– fs_tigre
Nov 13 '18 at 23:13
add a comment |
Is this the simplest way to do it?
– fs_tigre
Nov 13 '18 at 2:18
1
@fs_tigre You only need one or two more lines in the code you posted in your question. Using this answer's solution is just a different solution over usingJSONSerialization
.
– rmaddy
Nov 13 '18 at 3:09
@rmaddy - Thank you for the clarification, I edited my original question with my solution. @-Mohamed Mahdi Allani - Thanks a lot for demonstrating other methods, I when with the simples approach.
– fs_tigre
Nov 13 '18 at 23:13
Is this the simplest way to do it?
– fs_tigre
Nov 13 '18 at 2:18
Is this the simplest way to do it?
– fs_tigre
Nov 13 '18 at 2:18
1
1
@fs_tigre You only need one or two more lines in the code you posted in your question. Using this answer's solution is just a different solution over using
JSONSerialization
.– rmaddy
Nov 13 '18 at 3:09
@fs_tigre You only need one or two more lines in the code you posted in your question. Using this answer's solution is just a different solution over using
JSONSerialization
.– rmaddy
Nov 13 '18 at 3:09
@rmaddy - Thank you for the clarification, I edited my original question with my solution. @-Mohamed Mahdi Allani - Thanks a lot for demonstrating other methods, I when with the simples approach.
– fs_tigre
Nov 13 '18 at 23:13
@rmaddy - Thank you for the clarification, I edited my original question with my solution. @-Mohamed Mahdi Allani - Thanks a lot for demonstrating other methods, I when with the simples approach.
– fs_tigre
Nov 13 '18 at 23:13
add a comment |
Here is how I did it...
@IBAction func testing(_ sender: Any) {
let url = URL(string: "http://example.com/cars/mustang")
let task = URLSession.shared.dataTask(with: url!) { data, response, error in
guard error == nil else {
print(error!)
return
}
guard let data = data else {
print("Data is empty")
return
}
let json = try! JSONSerialization.jsonObject(with: data, options: )
guard let jsonArray = json as? [[String: String]] else {
return
}
let mustangColor = jsonArray[0]["color"]
print(mustangColor!)
}
task.resume()
}
add a comment |
Here is how I did it...
@IBAction func testing(_ sender: Any) {
let url = URL(string: "http://example.com/cars/mustang")
let task = URLSession.shared.dataTask(with: url!) { data, response, error in
guard error == nil else {
print(error!)
return
}
guard let data = data else {
print("Data is empty")
return
}
let json = try! JSONSerialization.jsonObject(with: data, options: )
guard let jsonArray = json as? [[String: String]] else {
return
}
let mustangColor = jsonArray[0]["color"]
print(mustangColor!)
}
task.resume()
}
add a comment |
Here is how I did it...
@IBAction func testing(_ sender: Any) {
let url = URL(string: "http://example.com/cars/mustang")
let task = URLSession.shared.dataTask(with: url!) { data, response, error in
guard error == nil else {
print(error!)
return
}
guard let data = data else {
print("Data is empty")
return
}
let json = try! JSONSerialization.jsonObject(with: data, options: )
guard let jsonArray = json as? [[String: String]] else {
return
}
let mustangColor = jsonArray[0]["color"]
print(mustangColor!)
}
task.resume()
}
Here is how I did it...
@IBAction func testing(_ sender: Any) {
let url = URL(string: "http://example.com/cars/mustang")
let task = URLSession.shared.dataTask(with: url!) { data, response, error in
guard error == nil else {
print(error!)
return
}
guard let data = data else {
print("Data is empty")
return
}
let json = try! JSONSerialization.jsonObject(with: data, options: )
guard let jsonArray = json as? [[String: String]] else {
return
}
let mustangColor = jsonArray[0]["color"]
print(mustangColor!)
}
task.resume()
}
answered Nov 14 '18 at 12:45
fs_tigrefs_tigre
4,57273972
4,57273972
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53271730%2fhow-to-get-specific-properties-from-a-json-object-from-http-request-using-urlses%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Yni,JSERizro PxKdd1,KZkCF3IR6UbsSHA T1RYzujyni1H,FpLMUBIcR5DP70BBw
3
json
is an array of dictionary. Access your data accordingly.– rmaddy
Nov 12 '18 at 23:46
Hmm, I cannot figure it out. Can you please direct me to where I can find information on how to get items from an array of dictionaries in Swift?
– fs_tigre
Nov 13 '18 at 2:23
2
The Collection Types chapter of the Swift book is a good start.
– rmaddy
Nov 13 '18 at 2:24
1
Try this stackoverflow.com/questions/38743156/…
– Alex Bailey
Nov 13 '18 at 5:01
Please do not put the answer in your question. Either accept an answer below if it best helped or post your own showing your chosen solution.
– rmaddy
Nov 14 '18 at 0:27