How can I setup test data for functional testing in Grails?
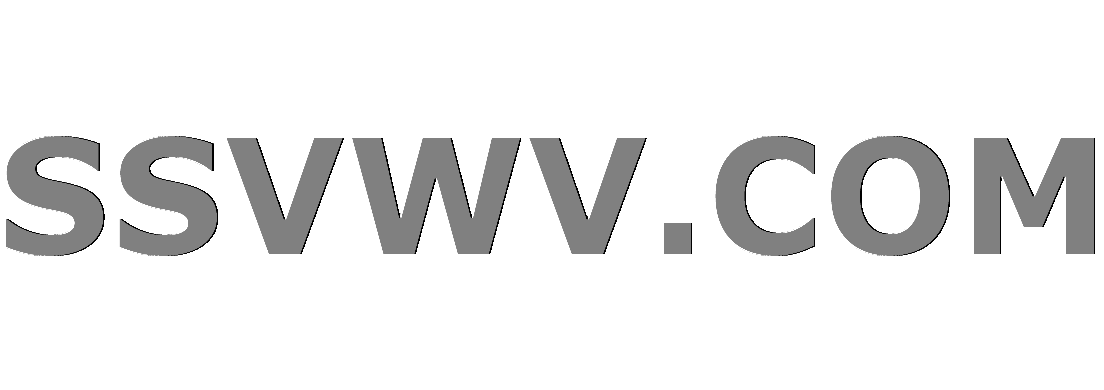
Multi tool use
So we have a restful service that we want to test using a restclient in grails.
The test code should go something like this...
class MyControllerSpec extends Specification {
def setup() {
this.dbEntity = new DbEntity("someid123").save();
}
void "Test entity GET"{
given:
RestBuilder rest = new RestBuilder()
when: "The DB entity service is hit"
RestResponse restResponse = rest.post("http://localhost:8080/api/someentity/$id");
then: "A 200 error is sent"
restResponse.status == 200
}
The problem I am having is the setup method blows up on .save() because there is not hibernate session. How can I manipulate my database before running a test?
unit-testing grails integration-testing functional-testing spock
add a comment |
So we have a restful service that we want to test using a restclient in grails.
The test code should go something like this...
class MyControllerSpec extends Specification {
def setup() {
this.dbEntity = new DbEntity("someid123").save();
}
void "Test entity GET"{
given:
RestBuilder rest = new RestBuilder()
when: "The DB entity service is hit"
RestResponse restResponse = rest.post("http://localhost:8080/api/someentity/$id");
then: "A 200 error is sent"
restResponse.status == 200
}
The problem I am having is the setup method blows up on .save() because there is not hibernate session. How can I manipulate my database before running a test?
unit-testing grails integration-testing functional-testing spock
Are you tried to add in test "setup:" block?
– Koloritnij
Dec 3 '15 at 9:33
add a comment |
So we have a restful service that we want to test using a restclient in grails.
The test code should go something like this...
class MyControllerSpec extends Specification {
def setup() {
this.dbEntity = new DbEntity("someid123").save();
}
void "Test entity GET"{
given:
RestBuilder rest = new RestBuilder()
when: "The DB entity service is hit"
RestResponse restResponse = rest.post("http://localhost:8080/api/someentity/$id");
then: "A 200 error is sent"
restResponse.status == 200
}
The problem I am having is the setup method blows up on .save() because there is not hibernate session. How can I manipulate my database before running a test?
unit-testing grails integration-testing functional-testing spock
So we have a restful service that we want to test using a restclient in grails.
The test code should go something like this...
class MyControllerSpec extends Specification {
def setup() {
this.dbEntity = new DbEntity("someid123").save();
}
void "Test entity GET"{
given:
RestBuilder rest = new RestBuilder()
when: "The DB entity service is hit"
RestResponse restResponse = rest.post("http://localhost:8080/api/someentity/$id");
then: "A 200 error is sent"
restResponse.status == 200
}
The problem I am having is the setup method blows up on .save() because there is not hibernate session. How can I manipulate my database before running a test?
unit-testing grails integration-testing functional-testing spock
unit-testing grails integration-testing functional-testing spock
asked Dec 3 '15 at 1:06


benstpierrebenstpierre
14.4k38146256
14.4k38146256
Are you tried to add in test "setup:" block?
– Koloritnij
Dec 3 '15 at 9:33
add a comment |
Are you tried to add in test "setup:" block?
– Koloritnij
Dec 3 '15 at 9:33
Are you tried to add in test "setup:" block?
– Koloritnij
Dec 3 '15 at 9:33
Are you tried to add in test "setup:" block?
– Koloritnij
Dec 3 '15 at 9:33
add a comment |
4 Answers
4
active
oldest
votes
You can define a method named like "setupData", and call it in the "given" block of "Test entity GET" testcase.
def setupData() { this.dbEntity = new DbEntity("someid123").save(); }
add a comment |
If you need to load some data before each funcional test, you can create a helper class, with @Shared variables or methods or both. Even you could override the setup, setupSpec methods in that class.
Your first class does not extends Specification now, DataLoader class (helper class) instead.
class MyControllerSpec extends DataLoader {
void setup(){
createEntity()
}
void "Test entity GET"{
given:
RestBuilder rest = new RestBuilder()
when: "The DB entity service is hit"
RestResponse restResponse = rest.post("http://localhost:8080/api/someentity/$dbEntity.id");
then: "A 200 error is sent"
restResponse.status == 200
}
}
And your helper class is the one which extends Specification, with its methods and @Shared variables.
import spock.lang.Shared
class DataLoader extends Specification {
@Shared DbEntity dbEntity
void createEntity(){
dbEntity = new DbEntity("someid123").save();
}
}
add a comment |
When extending GebSpec
in Grails 2.5.6, none of the other answers helped: I would still get
Method on class [...] was used outside of a Grails application
on the save()
call.
Adding @TestFor(DbEntity)
to the test class helped.
NB: While that annotation breaks integration tests, it seems to be necessary here. Not sure why that is.
This only kind-of works if you have one type you need to do this with, and cleanup isn't really possible.
– Raphael
Nov 13 '18 at 8:27
add a comment |
You probably want to use the remote-control
plugin. In Grails 2.x, add this to your BuildConfig.groovy
:
repositories {
...
mavenRepo "http://dl.bintray.com/alkemist/maven/"
}
plugins {
...
test ":remote-control:2.0"
}
After refreshing dependencies and potentially adjusting some settings (see e.g. here and here), you can use it like so in tests:
// <project>/test/functional/<package>/MyControllerSpec.groovy
class MyControllerSpec extends GebSpec {
RemoteControl remote
DbEntity dbEntity
def setup() {
this.remote = new RemoteControl()
this.dbEntity = remote {
new DbEntity("someid123").save()
}
}
def cleanup() {
remote {
DbEntity.findAll().each { dbe ->
println("Deleting $dbe")
dbe.delete()
}
}
}
Note:
- You can invoke
remote
ingiven
/setup
andwhen
blocks as well. - Sometimes, it seems to be necessary to wrap core in
remote { ... }
in aDbEntity.withTransaction { ... }
. Maybe that's obvious for the more intitiated; I stumbled over that. - If you want to return a
DbEntity
fromremote
it must be serializable.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f34056108%2fhow-can-i-setup-test-data-for-functional-testing-in-grails%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can define a method named like "setupData", and call it in the "given" block of "Test entity GET" testcase.
def setupData() { this.dbEntity = new DbEntity("someid123").save(); }
add a comment |
You can define a method named like "setupData", and call it in the "given" block of "Test entity GET" testcase.
def setupData() { this.dbEntity = new DbEntity("someid123").save(); }
add a comment |
You can define a method named like "setupData", and call it in the "given" block of "Test entity GET" testcase.
def setupData() { this.dbEntity = new DbEntity("someid123").save(); }
You can define a method named like "setupData", and call it in the "given" block of "Test entity GET" testcase.
def setupData() { this.dbEntity = new DbEntity("someid123").save(); }
answered Dec 30 '15 at 10:58
Andy.DAndy.D
14
14
add a comment |
add a comment |
If you need to load some data before each funcional test, you can create a helper class, with @Shared variables or methods or both. Even you could override the setup, setupSpec methods in that class.
Your first class does not extends Specification now, DataLoader class (helper class) instead.
class MyControllerSpec extends DataLoader {
void setup(){
createEntity()
}
void "Test entity GET"{
given:
RestBuilder rest = new RestBuilder()
when: "The DB entity service is hit"
RestResponse restResponse = rest.post("http://localhost:8080/api/someentity/$dbEntity.id");
then: "A 200 error is sent"
restResponse.status == 200
}
}
And your helper class is the one which extends Specification, with its methods and @Shared variables.
import spock.lang.Shared
class DataLoader extends Specification {
@Shared DbEntity dbEntity
void createEntity(){
dbEntity = new DbEntity("someid123").save();
}
}
add a comment |
If you need to load some data before each funcional test, you can create a helper class, with @Shared variables or methods or both. Even you could override the setup, setupSpec methods in that class.
Your first class does not extends Specification now, DataLoader class (helper class) instead.
class MyControllerSpec extends DataLoader {
void setup(){
createEntity()
}
void "Test entity GET"{
given:
RestBuilder rest = new RestBuilder()
when: "The DB entity service is hit"
RestResponse restResponse = rest.post("http://localhost:8080/api/someentity/$dbEntity.id");
then: "A 200 error is sent"
restResponse.status == 200
}
}
And your helper class is the one which extends Specification, with its methods and @Shared variables.
import spock.lang.Shared
class DataLoader extends Specification {
@Shared DbEntity dbEntity
void createEntity(){
dbEntity = new DbEntity("someid123").save();
}
}
add a comment |
If you need to load some data before each funcional test, you can create a helper class, with @Shared variables or methods or both. Even you could override the setup, setupSpec methods in that class.
Your first class does not extends Specification now, DataLoader class (helper class) instead.
class MyControllerSpec extends DataLoader {
void setup(){
createEntity()
}
void "Test entity GET"{
given:
RestBuilder rest = new RestBuilder()
when: "The DB entity service is hit"
RestResponse restResponse = rest.post("http://localhost:8080/api/someentity/$dbEntity.id");
then: "A 200 error is sent"
restResponse.status == 200
}
}
And your helper class is the one which extends Specification, with its methods and @Shared variables.
import spock.lang.Shared
class DataLoader extends Specification {
@Shared DbEntity dbEntity
void createEntity(){
dbEntity = new DbEntity("someid123").save();
}
}
If you need to load some data before each funcional test, you can create a helper class, with @Shared variables or methods or both. Even you could override the setup, setupSpec methods in that class.
Your first class does not extends Specification now, DataLoader class (helper class) instead.
class MyControllerSpec extends DataLoader {
void setup(){
createEntity()
}
void "Test entity GET"{
given:
RestBuilder rest = new RestBuilder()
when: "The DB entity service is hit"
RestResponse restResponse = rest.post("http://localhost:8080/api/someentity/$dbEntity.id");
then: "A 200 error is sent"
restResponse.status == 200
}
}
And your helper class is the one which extends Specification, with its methods and @Shared variables.
import spock.lang.Shared
class DataLoader extends Specification {
@Shared DbEntity dbEntity
void createEntity(){
dbEntity = new DbEntity("someid123").save();
}
}
answered Dec 30 '15 at 18:55


quindimildevquindimildev
990518
990518
add a comment |
add a comment |
When extending GebSpec
in Grails 2.5.6, none of the other answers helped: I would still get
Method on class [...] was used outside of a Grails application
on the save()
call.
Adding @TestFor(DbEntity)
to the test class helped.
NB: While that annotation breaks integration tests, it seems to be necessary here. Not sure why that is.
This only kind-of works if you have one type you need to do this with, and cleanup isn't really possible.
– Raphael
Nov 13 '18 at 8:27
add a comment |
When extending GebSpec
in Grails 2.5.6, none of the other answers helped: I would still get
Method on class [...] was used outside of a Grails application
on the save()
call.
Adding @TestFor(DbEntity)
to the test class helped.
NB: While that annotation breaks integration tests, it seems to be necessary here. Not sure why that is.
This only kind-of works if you have one type you need to do this with, and cleanup isn't really possible.
– Raphael
Nov 13 '18 at 8:27
add a comment |
When extending GebSpec
in Grails 2.5.6, none of the other answers helped: I would still get
Method on class [...] was used outside of a Grails application
on the save()
call.
Adding @TestFor(DbEntity)
to the test class helped.
NB: While that annotation breaks integration tests, it seems to be necessary here. Not sure why that is.
When extending GebSpec
in Grails 2.5.6, none of the other answers helped: I would still get
Method on class [...] was used outside of a Grails application
on the save()
call.
Adding @TestFor(DbEntity)
to the test class helped.
NB: While that annotation breaks integration tests, it seems to be necessary here. Not sure why that is.
answered Nov 8 '18 at 11:31


RaphaelRaphael
3,4162952
3,4162952
This only kind-of works if you have one type you need to do this with, and cleanup isn't really possible.
– Raphael
Nov 13 '18 at 8:27
add a comment |
This only kind-of works if you have one type you need to do this with, and cleanup isn't really possible.
– Raphael
Nov 13 '18 at 8:27
This only kind-of works if you have one type you need to do this with, and cleanup isn't really possible.
– Raphael
Nov 13 '18 at 8:27
This only kind-of works if you have one type you need to do this with, and cleanup isn't really possible.
– Raphael
Nov 13 '18 at 8:27
add a comment |
You probably want to use the remote-control
plugin. In Grails 2.x, add this to your BuildConfig.groovy
:
repositories {
...
mavenRepo "http://dl.bintray.com/alkemist/maven/"
}
plugins {
...
test ":remote-control:2.0"
}
After refreshing dependencies and potentially adjusting some settings (see e.g. here and here), you can use it like so in tests:
// <project>/test/functional/<package>/MyControllerSpec.groovy
class MyControllerSpec extends GebSpec {
RemoteControl remote
DbEntity dbEntity
def setup() {
this.remote = new RemoteControl()
this.dbEntity = remote {
new DbEntity("someid123").save()
}
}
def cleanup() {
remote {
DbEntity.findAll().each { dbe ->
println("Deleting $dbe")
dbe.delete()
}
}
}
Note:
- You can invoke
remote
ingiven
/setup
andwhen
blocks as well. - Sometimes, it seems to be necessary to wrap core in
remote { ... }
in aDbEntity.withTransaction { ... }
. Maybe that's obvious for the more intitiated; I stumbled over that. - If you want to return a
DbEntity
fromremote
it must be serializable.
add a comment |
You probably want to use the remote-control
plugin. In Grails 2.x, add this to your BuildConfig.groovy
:
repositories {
...
mavenRepo "http://dl.bintray.com/alkemist/maven/"
}
plugins {
...
test ":remote-control:2.0"
}
After refreshing dependencies and potentially adjusting some settings (see e.g. here and here), you can use it like so in tests:
// <project>/test/functional/<package>/MyControllerSpec.groovy
class MyControllerSpec extends GebSpec {
RemoteControl remote
DbEntity dbEntity
def setup() {
this.remote = new RemoteControl()
this.dbEntity = remote {
new DbEntity("someid123").save()
}
}
def cleanup() {
remote {
DbEntity.findAll().each { dbe ->
println("Deleting $dbe")
dbe.delete()
}
}
}
Note:
- You can invoke
remote
ingiven
/setup
andwhen
blocks as well. - Sometimes, it seems to be necessary to wrap core in
remote { ... }
in aDbEntity.withTransaction { ... }
. Maybe that's obvious for the more intitiated; I stumbled over that. - If you want to return a
DbEntity
fromremote
it must be serializable.
add a comment |
You probably want to use the remote-control
plugin. In Grails 2.x, add this to your BuildConfig.groovy
:
repositories {
...
mavenRepo "http://dl.bintray.com/alkemist/maven/"
}
plugins {
...
test ":remote-control:2.0"
}
After refreshing dependencies and potentially adjusting some settings (see e.g. here and here), you can use it like so in tests:
// <project>/test/functional/<package>/MyControllerSpec.groovy
class MyControllerSpec extends GebSpec {
RemoteControl remote
DbEntity dbEntity
def setup() {
this.remote = new RemoteControl()
this.dbEntity = remote {
new DbEntity("someid123").save()
}
}
def cleanup() {
remote {
DbEntity.findAll().each { dbe ->
println("Deleting $dbe")
dbe.delete()
}
}
}
Note:
- You can invoke
remote
ingiven
/setup
andwhen
blocks as well. - Sometimes, it seems to be necessary to wrap core in
remote { ... }
in aDbEntity.withTransaction { ... }
. Maybe that's obvious for the more intitiated; I stumbled over that. - If you want to return a
DbEntity
fromremote
it must be serializable.
You probably want to use the remote-control
plugin. In Grails 2.x, add this to your BuildConfig.groovy
:
repositories {
...
mavenRepo "http://dl.bintray.com/alkemist/maven/"
}
plugins {
...
test ":remote-control:2.0"
}
After refreshing dependencies and potentially adjusting some settings (see e.g. here and here), you can use it like so in tests:
// <project>/test/functional/<package>/MyControllerSpec.groovy
class MyControllerSpec extends GebSpec {
RemoteControl remote
DbEntity dbEntity
def setup() {
this.remote = new RemoteControl()
this.dbEntity = remote {
new DbEntity("someid123").save()
}
}
def cleanup() {
remote {
DbEntity.findAll().each { dbe ->
println("Deleting $dbe")
dbe.delete()
}
}
}
Note:
- You can invoke
remote
ingiven
/setup
andwhen
blocks as well. - Sometimes, it seems to be necessary to wrap core in
remote { ... }
in aDbEntity.withTransaction { ... }
. Maybe that's obvious for the more intitiated; I stumbled over that. - If you want to return a
DbEntity
fromremote
it must be serializable.
answered Nov 13 '18 at 10:00


RaphaelRaphael
3,4162952
3,4162952
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f34056108%2fhow-can-i-setup-test-data-for-functional-testing-in-grails%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
B9JrkRWECk9X0u8Rnn,5EHnGt8UB ZChOGgpDYk,QVhzUav4q
Are you tried to add in test "setup:" block?
– Koloritnij
Dec 3 '15 at 9:33