Array is not a constructor in javascript unit test angular
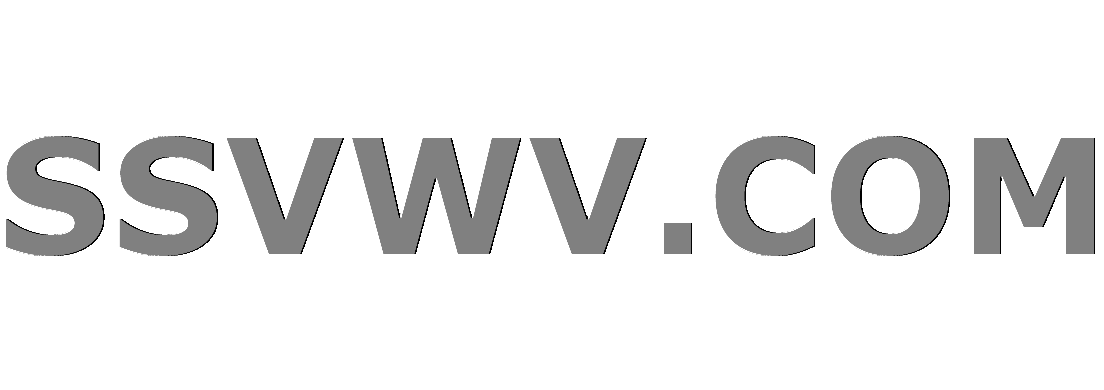
Multi tool use
I have a factory for create dialog:
module myModule {
export class myDialog {
constructor(private $uibModal: ng.ui.bootstrap.IModalService) {}
showDialog() {
var options: ng.ui.bootstrap.IModalSettings = {
templateUrl: '/dialog.html',
size: "lg",
controller: ['$scope', '$uibModalInstance', function($scope: any, $uibModalInstance: ng.ui.bootstrap.IModalServiceInstance) {
$scope.cancel = () => {
$uibModalInstance.close({
doAction: 'close'
});
}
}],
controllerAs: '$ctrl'
};
return this.$uibModal.open(options).result;
}
static factory(): any {
const dialog = ($uibModal: ng.ui.bootstrap.IModalService) => new myDialog($uibModal);
dialog.$inject = ['$uibModal'];
return dialog;
}
};
angular.module('myModule').factory('myDialog', myDialog.factory());
}
As you see, for controller
injection I used array (inline array annotation) in order to work when javascript file is minified.
I created a test using bardjs:
describe('My dialog service', function() {
beforeEach(function() {
module('myModule', function($provide) {
$provide.factory('$uibModalInstance', function() {
return {
close: function(result) {
return result;
}
};
});
});
module('myModule');
bard.inject('$uibModal', '$uibModalInstance', '$http', '$httpBackend', '$q', '$rootScope', 'myDialog');
bard.mockService($uibModal, {
open: function(options) {
return {
result: options
};
}
});
spyOn($uibModal, 'open').and.callThrough();
spyOn($uibModalInstance, 'close').and.callThrough();
});
it('expect it to be defined', function() {
expect(myDialog).toBeDefined();
});
});
But I got error:
TypeError: Array is not a constructor (evaluating
'options.controller(scope,$uibModalInstance)')
Why ? How to solve it ?
javascript angularjs
|
show 1 more comment
I have a factory for create dialog:
module myModule {
export class myDialog {
constructor(private $uibModal: ng.ui.bootstrap.IModalService) {}
showDialog() {
var options: ng.ui.bootstrap.IModalSettings = {
templateUrl: '/dialog.html',
size: "lg",
controller: ['$scope', '$uibModalInstance', function($scope: any, $uibModalInstance: ng.ui.bootstrap.IModalServiceInstance) {
$scope.cancel = () => {
$uibModalInstance.close({
doAction: 'close'
});
}
}],
controllerAs: '$ctrl'
};
return this.$uibModal.open(options).result;
}
static factory(): any {
const dialog = ($uibModal: ng.ui.bootstrap.IModalService) => new myDialog($uibModal);
dialog.$inject = ['$uibModal'];
return dialog;
}
};
angular.module('myModule').factory('myDialog', myDialog.factory());
}
As you see, for controller
injection I used array (inline array annotation) in order to work when javascript file is minified.
I created a test using bardjs:
describe('My dialog service', function() {
beforeEach(function() {
module('myModule', function($provide) {
$provide.factory('$uibModalInstance', function() {
return {
close: function(result) {
return result;
}
};
});
});
module('myModule');
bard.inject('$uibModal', '$uibModalInstance', '$http', '$httpBackend', '$q', '$rootScope', 'myDialog');
bard.mockService($uibModal, {
open: function(options) {
return {
result: options
};
}
});
spyOn($uibModal, 'open').and.callThrough();
spyOn($uibModalInstance, 'close').and.callThrough();
});
it('expect it to be defined', function() {
expect(myDialog).toBeDefined();
});
});
But I got error:
TypeError: Array is not a constructor (evaluating
'options.controller(scope,$uibModalInstance)')
Why ? How to solve it ?
javascript angularjs
Have you tried using$inject
like indialog
?
– barbsan
Nov 13 '18 at 10:36
Where and how ?
– Snake Eyes
Nov 13 '18 at 10:58
I'd tryoptions.controller.$inject = [/*your dependencies*/]
before passingoptions
toopen
method
– barbsan
Nov 13 '18 at 11:01
Typescript does not like it and won't generatejs
file ... :(
– Snake Eyes
Nov 13 '18 at 11:04
1
How about declaring controller before options?const ctrl = function ...; ctrl.$inject = [/*deps*/]; var options = {... controller: ctrl, ...}
– barbsan
Nov 13 '18 at 11:23
|
show 1 more comment
I have a factory for create dialog:
module myModule {
export class myDialog {
constructor(private $uibModal: ng.ui.bootstrap.IModalService) {}
showDialog() {
var options: ng.ui.bootstrap.IModalSettings = {
templateUrl: '/dialog.html',
size: "lg",
controller: ['$scope', '$uibModalInstance', function($scope: any, $uibModalInstance: ng.ui.bootstrap.IModalServiceInstance) {
$scope.cancel = () => {
$uibModalInstance.close({
doAction: 'close'
});
}
}],
controllerAs: '$ctrl'
};
return this.$uibModal.open(options).result;
}
static factory(): any {
const dialog = ($uibModal: ng.ui.bootstrap.IModalService) => new myDialog($uibModal);
dialog.$inject = ['$uibModal'];
return dialog;
}
};
angular.module('myModule').factory('myDialog', myDialog.factory());
}
As you see, for controller
injection I used array (inline array annotation) in order to work when javascript file is minified.
I created a test using bardjs:
describe('My dialog service', function() {
beforeEach(function() {
module('myModule', function($provide) {
$provide.factory('$uibModalInstance', function() {
return {
close: function(result) {
return result;
}
};
});
});
module('myModule');
bard.inject('$uibModal', '$uibModalInstance', '$http', '$httpBackend', '$q', '$rootScope', 'myDialog');
bard.mockService($uibModal, {
open: function(options) {
return {
result: options
};
}
});
spyOn($uibModal, 'open').and.callThrough();
spyOn($uibModalInstance, 'close').and.callThrough();
});
it('expect it to be defined', function() {
expect(myDialog).toBeDefined();
});
});
But I got error:
TypeError: Array is not a constructor (evaluating
'options.controller(scope,$uibModalInstance)')
Why ? How to solve it ?
javascript angularjs
I have a factory for create dialog:
module myModule {
export class myDialog {
constructor(private $uibModal: ng.ui.bootstrap.IModalService) {}
showDialog() {
var options: ng.ui.bootstrap.IModalSettings = {
templateUrl: '/dialog.html',
size: "lg",
controller: ['$scope', '$uibModalInstance', function($scope: any, $uibModalInstance: ng.ui.bootstrap.IModalServiceInstance) {
$scope.cancel = () => {
$uibModalInstance.close({
doAction: 'close'
});
}
}],
controllerAs: '$ctrl'
};
return this.$uibModal.open(options).result;
}
static factory(): any {
const dialog = ($uibModal: ng.ui.bootstrap.IModalService) => new myDialog($uibModal);
dialog.$inject = ['$uibModal'];
return dialog;
}
};
angular.module('myModule').factory('myDialog', myDialog.factory());
}
As you see, for controller
injection I used array (inline array annotation) in order to work when javascript file is minified.
I created a test using bardjs:
describe('My dialog service', function() {
beforeEach(function() {
module('myModule', function($provide) {
$provide.factory('$uibModalInstance', function() {
return {
close: function(result) {
return result;
}
};
});
});
module('myModule');
bard.inject('$uibModal', '$uibModalInstance', '$http', '$httpBackend', '$q', '$rootScope', 'myDialog');
bard.mockService($uibModal, {
open: function(options) {
return {
result: options
};
}
});
spyOn($uibModal, 'open').and.callThrough();
spyOn($uibModalInstance, 'close').and.callThrough();
});
it('expect it to be defined', function() {
expect(myDialog).toBeDefined();
});
});
But I got error:
TypeError: Array is not a constructor (evaluating
'options.controller(scope,$uibModalInstance)')
Why ? How to solve it ?
javascript angularjs
javascript angularjs
edited Nov 13 '18 at 10:59
Snake Eyes
asked Nov 13 '18 at 10:07


Snake EyesSnake Eyes
8,1982682157
8,1982682157
Have you tried using$inject
like indialog
?
– barbsan
Nov 13 '18 at 10:36
Where and how ?
– Snake Eyes
Nov 13 '18 at 10:58
I'd tryoptions.controller.$inject = [/*your dependencies*/]
before passingoptions
toopen
method
– barbsan
Nov 13 '18 at 11:01
Typescript does not like it and won't generatejs
file ... :(
– Snake Eyes
Nov 13 '18 at 11:04
1
How about declaring controller before options?const ctrl = function ...; ctrl.$inject = [/*deps*/]; var options = {... controller: ctrl, ...}
– barbsan
Nov 13 '18 at 11:23
|
show 1 more comment
Have you tried using$inject
like indialog
?
– barbsan
Nov 13 '18 at 10:36
Where and how ?
– Snake Eyes
Nov 13 '18 at 10:58
I'd tryoptions.controller.$inject = [/*your dependencies*/]
before passingoptions
toopen
method
– barbsan
Nov 13 '18 at 11:01
Typescript does not like it and won't generatejs
file ... :(
– Snake Eyes
Nov 13 '18 at 11:04
1
How about declaring controller before options?const ctrl = function ...; ctrl.$inject = [/*deps*/]; var options = {... controller: ctrl, ...}
– barbsan
Nov 13 '18 at 11:23
Have you tried using
$inject
like in dialog
?– barbsan
Nov 13 '18 at 10:36
Have you tried using
$inject
like in dialog
?– barbsan
Nov 13 '18 at 10:36
Where and how ?
– Snake Eyes
Nov 13 '18 at 10:58
Where and how ?
– Snake Eyes
Nov 13 '18 at 10:58
I'd try
options.controller.$inject = [/*your dependencies*/]
before passing options
to open
method– barbsan
Nov 13 '18 at 11:01
I'd try
options.controller.$inject = [/*your dependencies*/]
before passing options
to open
method– barbsan
Nov 13 '18 at 11:01
Typescript does not like it and won't generate
js
file ... :(– Snake Eyes
Nov 13 '18 at 11:04
Typescript does not like it and won't generate
js
file ... :(– Snake Eyes
Nov 13 '18 at 11:04
1
1
How about declaring controller before options?
const ctrl = function ...; ctrl.$inject = [/*deps*/]; var options = {... controller: ctrl, ...}
– barbsan
Nov 13 '18 at 11:23
How about declaring controller before options?
const ctrl = function ...; ctrl.$inject = [/*deps*/]; var options = {... controller: ctrl, ...}
– barbsan
Nov 13 '18 at 11:23
|
show 1 more comment
1 Answer
1
active
oldest
votes
Declare controller outside options
object and use $inject to inject dependencies.
showDialog() {
const ctrl = function($scope: any, $uibModalInstance: ng.ui.bootstrap.IModalServiceInstance) {
$scope.cancel = () => {
$uibModalInstance.close({
doAction: 'close'
});
}
};
ctrl.$inject = ['$scope', '$uibModalInstance'];
var options: ng.ui.bootstrap.IModalSettings = {
templateUrl: '/dialog.html',
size: "lg",
controller: ctrl,
controllerAs: '$ctrl'
};
return this.$uibModal.open(options).result;
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53278523%2farray-is-not-a-constructor-in-javascript-unit-test-angular%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Declare controller outside options
object and use $inject to inject dependencies.
showDialog() {
const ctrl = function($scope: any, $uibModalInstance: ng.ui.bootstrap.IModalServiceInstance) {
$scope.cancel = () => {
$uibModalInstance.close({
doAction: 'close'
});
}
};
ctrl.$inject = ['$scope', '$uibModalInstance'];
var options: ng.ui.bootstrap.IModalSettings = {
templateUrl: '/dialog.html',
size: "lg",
controller: ctrl,
controllerAs: '$ctrl'
};
return this.$uibModal.open(options).result;
}
add a comment |
Declare controller outside options
object and use $inject to inject dependencies.
showDialog() {
const ctrl = function($scope: any, $uibModalInstance: ng.ui.bootstrap.IModalServiceInstance) {
$scope.cancel = () => {
$uibModalInstance.close({
doAction: 'close'
});
}
};
ctrl.$inject = ['$scope', '$uibModalInstance'];
var options: ng.ui.bootstrap.IModalSettings = {
templateUrl: '/dialog.html',
size: "lg",
controller: ctrl,
controllerAs: '$ctrl'
};
return this.$uibModal.open(options).result;
}
add a comment |
Declare controller outside options
object and use $inject to inject dependencies.
showDialog() {
const ctrl = function($scope: any, $uibModalInstance: ng.ui.bootstrap.IModalServiceInstance) {
$scope.cancel = () => {
$uibModalInstance.close({
doAction: 'close'
});
}
};
ctrl.$inject = ['$scope', '$uibModalInstance'];
var options: ng.ui.bootstrap.IModalSettings = {
templateUrl: '/dialog.html',
size: "lg",
controller: ctrl,
controllerAs: '$ctrl'
};
return this.$uibModal.open(options).result;
}
Declare controller outside options
object and use $inject to inject dependencies.
showDialog() {
const ctrl = function($scope: any, $uibModalInstance: ng.ui.bootstrap.IModalServiceInstance) {
$scope.cancel = () => {
$uibModalInstance.close({
doAction: 'close'
});
}
};
ctrl.$inject = ['$scope', '$uibModalInstance'];
var options: ng.ui.bootstrap.IModalSettings = {
templateUrl: '/dialog.html',
size: "lg",
controller: ctrl,
controllerAs: '$ctrl'
};
return this.$uibModal.open(options).result;
}
answered Nov 13 '18 at 12:26
barbsanbarbsan
2,34321222
2,34321222
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53278523%2farray-is-not-a-constructor-in-javascript-unit-test-angular%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
neeS,YuLwoEejNdXdmc0SK V2qkSFLKJJ0Dtv4XQd1,ffBU2IB1xeu6VBtfI0pCV,Su5Ti 7Cp1pDsmrTayA5AGZg
Have you tried using
$inject
like indialog
?– barbsan
Nov 13 '18 at 10:36
Where and how ?
– Snake Eyes
Nov 13 '18 at 10:58
I'd try
options.controller.$inject = [/*your dependencies*/]
before passingoptions
toopen
method– barbsan
Nov 13 '18 at 11:01
Typescript does not like it and won't generate
js
file ... :(– Snake Eyes
Nov 13 '18 at 11:04
1
How about declaring controller before options?
const ctrl = function ...; ctrl.$inject = [/*deps*/]; var options = {... controller: ctrl, ...}
– barbsan
Nov 13 '18 at 11:23