Is it possible to make a wear app that connects to my android app activity(maps)
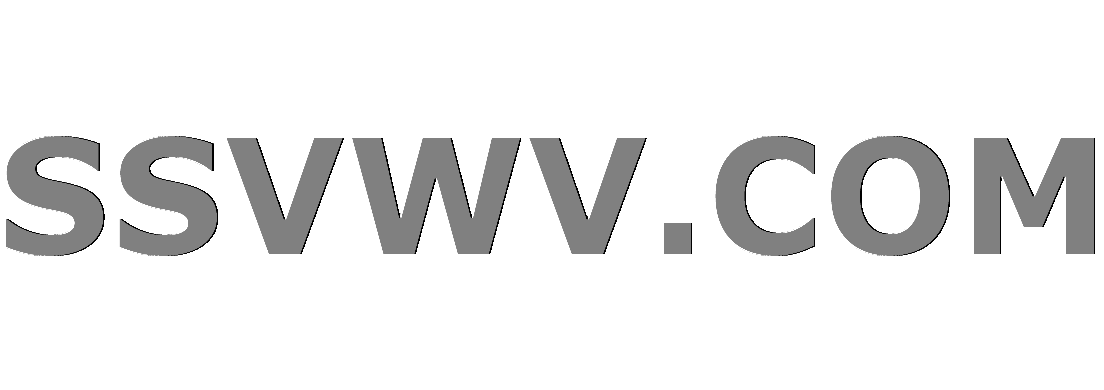
Multi tool use
I have a program that has one activity which the user can record his trip by pressing a button from one place to an other.I want somehow to make a wear app that connects with this activity so the user can press that button from the smartwatch and basically do whatever the android activity does.
Here is the code in case it's helpful
Map.java
public class Map extends AppCompatActivity implements LocationListener, OnMapReadyCallback, NavigationView.OnNavigationItemSelectedListener {
//VARIABLES
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.map);
//CALL SERVICE
Intent serviceIntent=new Intent(this, MyLocationService.class);
startService(serviceIntent);
getWindow().addFlags(WindowManager.LayoutParams.FLAG_KEEP_SCREEN_ON);
toolbar = (Toolbar) findViewById(R.id.toolbar);
setSupportActionBar(toolbar);
getSupportActionBar().setDisplayShowTitleEnabled(false);
toolbar.setTitle("");
toolbar.setSubtitle("");
//gpsStatus = (TextView) findViewById(R.id.gpsStatus);
dis = (TextView) findViewById(R.id.distancePreview);
newActL = (RelativeLayout) findViewById(R.id.newAct);
startActL = (RelativeLayout) findViewById(R.id.Act);
timer = (TextView) findViewById(R.id.timePreview);
newActB = (ImageButton) findViewById(R.id.newActB);
stopActB = (ImageButton) findViewById(R.id.stopActB);
gearMap = (ImageButton) findViewById(R.id.gearmap);
List<String> spinnerArray = new ArrayList<String>();
spinnerArray.add("Arrival");
spinnerArray.add("Departure");
ArrayAdapter<String> adapter = new ArrayAdapter<String>(
this, android.R.layout.simple_spinner_item, spinnerArray);
adapter.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item);
sItems = (Spinner) findViewById(R.id.ardep);
sItems.setAdapter(adapter);
List<String> spinnerArray2 = new ArrayList<String>();
spinnerArray2.add("Running");
spinnerArray2.add("Walking");
spinnerArray2.add("Cycling");
spinnerArray2.add("Roller skating");
spinnerArray2.add("Skateboarding");
spinnerArray2.add("Kickbiking");
spinnerArray2.add("Teleporting");
ArrayAdapter<String> adapter2 = new ArrayAdapter<String>(
this, android.R.layout.simple_spinner_item, spinnerArray2);
adapter2.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item);
actCombo = (Spinner) findViewById(R.id.actCombo);
actCombo.setAdapter(adapter2);
Calendar rightNow = Calendar.getInstance();
int currentHour = rightNow.get(Calendar.HOUR_OF_DAY);
if (currentHour < 12) {
actCombo.setSelection(GetInfo.arract - 1);
sItems.setSelection(0);
} else {
actCombo.setSelection(GetInfo.depact - 1);
sItems.setSelection(1);
}
locationManager = (LocationManager) getSystemService(Context.LOCATION_SERVICE);
if (ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION) != PackageManager.PERMISSION_GRANTED &&
ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_COARSE_LOCATION) != PackageManager.PERMISSION_GRANTED) {
return;
}
locationManager.requestLocationUpdates(LocationManager.GPS_PROVIDER,
3000,
1, this);
SupportMapFragment mapFragment = (SupportMapFragment) getSupportFragmentManager().findFragmentById(R.id.mapview);
mapFragment.getMapAsync(this);
line = new PolylineOptions().width(3).color(Color.BLUE);
DrawerLayout drawer = (DrawerLayout) findViewById(R.id.drawer_layout);
ActionBarDrawerToggle toggle = new ActionBarDrawerToggle(
this, drawer, toolbar, R.string.navigation_drawer_open, R.string.navigation_drawer_close);
drawer.addDrawerListener(toggle);
toggle.syncState();
navigationView = (NavigationView) findViewById(R.id.nav_view);
headerView = navigationView.getHeaderView(0);
fullnameside = (TextView) headerView.findViewById(R.id.fullnameside);
emailside = (TextView) headerView.findViewById(R.id.emailside);
fullnameside.setText("" + GetInfo.fullname);
emailside.setText("" + GetInfo.email);
navigationView.setNavigationItemSelectedListener(this);
navigationView.getMenu().getItem(1).setChecked(true);
//Toast.makeText(this.getBaseContext(), ""+ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION), Toast.LENGTH_SHORT).show();
}
private void getLocation() {
//Toast.makeText(getBaseContext(), "e paides", Toast.LENGTH_SHORT).show();
String locationProvider = LocationManager.NETWORK_PROVIDER;
if (ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION) != PackageManager.PERMISSION_GRANTED && ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_COARSE_LOCATION) != PackageManager.PERMISSION_GRANTED) {
// TODO: Consider calling
// ActivityCompat#requestPermissions
// here to request the missing permissions, and then overriding
// public void onRequestPermissionsResult(int requestCode, String permissions,
// int grantResults)
// to handle the case where the user grants the permission. See the documentation
// for ActivityCompat#requestPermissions for more details.
return;
}
Location location = locationManager.getLastKnownLocation(locationProvider);
try {
line.add(new LatLng(location.getLatitude(), location.getLongitude()));
GMap.addMarker(new MarkerOptions().position(new LatLng(location.getLatitude(), location.getLongitude())).title(""));
GMap.animateCamera(CameraUpdateFactory.newLatLngZoom(new LatLng(location.getLatitude(), location.getLongitude()), 16.0f));
steps++;
getloc = true;
} catch (NullPointerException e) {
//Toast.makeText(this.getBaseContext(), "gyhg" + e.toString(), Toast.LENGTH_LONG).show();
}
}
public void StopTrip(View v) {
AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setMessage("Stop and upload your trip?")
.setCancelable(true)
.setNegativeButton("NO", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
}
})
.setPositiveButton("YES", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
float finalDistance = (float) (distance / 1000.0);
if (finalDistance < 2) {
Intent myIntent = new Intent(Map.this, Profile.class);
startActivity(myIntent);
}
TimeBuff += MillisecondTime;
handler.removeCallbacks(runnable);
// newActL.setVisibility(View.GONE);
// startActL.setVisibility(View.VISIBLE);
enabledActivity = false;
//database post
try {
Date currentTime = Calendar.getInstance().getTime();
SimpleDateFormat df = new SimpleDateFormat("yyyy-MM-dd");
String formattedDate = df.format(currentTime);
Date currentTime2 = Calendar.getInstance().getTime();
SimpleDateFormat df2 = new SimpleDateFormat("yyyy-MM-dd%20HH:mm:ss");
dateActEnd = df2.format(currentTime2);
String act = actCombo.getSelectedItem().toString();
String act_id = "7";
switch (act) {
case "Running":
act_id = "1";
break;
case "Walking":
act_id = "2";
break;
case "Cycling":
act_id = "3";
break;
case "Roller skating":
act_id = "4";
break;
case "Skateboarding":
act_id = "5";
break;
case "Kickbiking":
act_id = "6";
break;
case "Teleporting":
act_id = "7";
break;
}
String direcor;
if (sItems.getSelectedItem().toString().equals("Arrival"))
direcor = "arrival";
else
direcor = "departure";
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build();
StrictMode.setThreadPolicy(policy);
// String.format("%.1f", finalDistance)
URL obj = new URL("https://gekon.technologypark.cz/api/v1/record/create?user=" + LoginInfo.UserID
+ "&date=" + formattedDate + "&distance=" + String.format("%.1f", finalDistance) + "&direction=" + direcor
+ "&activity=" + act_id + "&polyline=" + PolyUtil.encode(line.getPoints()) + "&start=" + dateActStart
+ "&end=" + dateActEnd + "&source=mobileapp");
HttpsURLConnection conn = (HttpsURLConnection) obj.openConnection();
conn.setRequestMethod("POST");
conn.setRequestProperty("ApiSecret", LoginInfo.ApiSecret);
conn.connect();
BufferedReader br = new BufferedReader(new InputStreamReader((conn.getInputStream())));
StringBuilder sb = new StringBuilder();
String output;
while ((output = br.readLine()) != null)
sb.append(output);
JSONObject jsonObj = new JSONObject(sb.toString());
JSONObject curRecord = new JSONObject(jsonObj.getString("data"));
Trips.datet.add(currentTime);
Trips.datestr.add(formattedDate);
Trips.act.add(act_id);
Trips.tripType.add(sItems.getSelectedItem().toString());
Trips.dist.add(String.format("%.1f", finalDistance));
Trips.trip_ids.add(curRecord.getString("trip_id"));
Trips.calc(++Trips.points);
TripsCalendarInfo.datet.add(currentTime);
TripsCalendarInfo.act.add(act_id);
TripsCalendarInfo.act_str.add(act);
TripsCalendarInfo.tripType.add(sItems.getSelectedItem().toString());
TripsCalendarInfo.dist.add(String.format("%.1f", finalDistance));
TripsCalendarInfo.datestr.add(formattedDate);
TripsCalendarInfo.trip_ids.add(curRecord.getString("trip_id"));
TripsCalendarInfo.trip_source.add("mobileapp");
TripsCalendarInfo.polyline.add(PolyUtil.encode(line.getPoints()));
TripsCalendarInfo.CanItrip();
float km_up = Float.parseFloat(TripsInfo.km.get(TripsInfo.userRank - 1)) + finalDistance;
int trip_up = Integer.parseInt(TripsInfo.trips.get(TripsInfo.userRank - 1)) + 1;
TripsInfo.trips.set(TripsInfo.userRank - 1, "" + trip_up);
TripsInfo.km.set(TripsInfo.userRank - 1, String.format("%.1f", km_up));
TripsInfo.rankSort();
getloc = false;
Intent myIntent = new Intent(Map.this, Profile.class);
startActivity(myIntent);
} catch (Exception e) {
Toast.makeText(getBaseContext(), "Error upload, please check your options at gear button", Toast.LENGTH_LONG).show();
}
}
});
float finalDistance = (float) (distance / 1000.0);
if (finalDistance < 2) {
builder.setMessage("Your trip is below 2km and it will not be counted.nAre you sure you want to stop?");
}
AlertDialog alert = builder.create();
alert.show();
}
public void StartAct(View v) {
timer.setVisibility(View.VISIBLE);
dis.setVisibility(View.VISIBLE);
newActB.setVisibility(View.GONE);
stopActB.setVisibility(View.VISIBLE);
//gearMap.setVisibility(View.GONE);
navigationView.setVisibility(View.GONE);
//headerView.setSystemUiVisibility(View.SYSTEM_UI_FLAG_HIDE_NAVIGATION);
toolbar.setNavigationIcon(null); // to hide Navigation icon
//toolbar.setDisplayHomeAsUpEnabled(false);
handler = new Handler();
Date currentTime = Calendar.getInstance().getTime();
SimpleDateFormat df = new SimpleDateFormat("yyyy-MM-dd%20HH:mm:ss");
dateActStart = df.format(currentTime);
StartTime = SystemClock.uptimeMillis();
handler.postDelayed(runnable, 0);
startService(new Intent(this, LocationService.class));
//enabledActivity = true;
}
public void ChangeAct(View v) {
if (newActL.getVisibility() == View.GONE) {
newActL.setVisibility(View.VISIBLE);
startActL.setVisibility(View.GONE);
} else {
newActL.setVisibility(View.GONE);
startActL.setVisibility(View.VISIBLE);
}
}
public Runnable runnable = new Runnable() {
public void run() {
MillisecondTime = SystemClock.uptimeMillis() - StartTime;
UpdateTime = TimeBuff + MillisecondTime;
Seconds = (int) (UpdateTime / 1000);
Minutes = Seconds / 60;
Hours = Minutes / 60;
Seconds = Seconds % 60;
Minutes = Minutes % 60;
MilliSeconds = (int) (UpdateTime % 100);
timer.setText("Time: " + String.format("%02d", Hours) + ":"
+ String.format("%02d", Minutes) + ":"
+ String.format("%02d", Seconds));
handler.postDelayed(this, 950);
}
};
@Override
public void onMapReady(GoogleMap map) {
GMap = map;
GMap.moveCamera(CameraUpdateFactory.newLatLngZoom(new LatLng(49.8117806, 15.6970293), 6.0f));
if (!getloc)
getLocation();
}

add a comment |
I have a program that has one activity which the user can record his trip by pressing a button from one place to an other.I want somehow to make a wear app that connects with this activity so the user can press that button from the smartwatch and basically do whatever the android activity does.
Here is the code in case it's helpful
Map.java
public class Map extends AppCompatActivity implements LocationListener, OnMapReadyCallback, NavigationView.OnNavigationItemSelectedListener {
//VARIABLES
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.map);
//CALL SERVICE
Intent serviceIntent=new Intent(this, MyLocationService.class);
startService(serviceIntent);
getWindow().addFlags(WindowManager.LayoutParams.FLAG_KEEP_SCREEN_ON);
toolbar = (Toolbar) findViewById(R.id.toolbar);
setSupportActionBar(toolbar);
getSupportActionBar().setDisplayShowTitleEnabled(false);
toolbar.setTitle("");
toolbar.setSubtitle("");
//gpsStatus = (TextView) findViewById(R.id.gpsStatus);
dis = (TextView) findViewById(R.id.distancePreview);
newActL = (RelativeLayout) findViewById(R.id.newAct);
startActL = (RelativeLayout) findViewById(R.id.Act);
timer = (TextView) findViewById(R.id.timePreview);
newActB = (ImageButton) findViewById(R.id.newActB);
stopActB = (ImageButton) findViewById(R.id.stopActB);
gearMap = (ImageButton) findViewById(R.id.gearmap);
List<String> spinnerArray = new ArrayList<String>();
spinnerArray.add("Arrival");
spinnerArray.add("Departure");
ArrayAdapter<String> adapter = new ArrayAdapter<String>(
this, android.R.layout.simple_spinner_item, spinnerArray);
adapter.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item);
sItems = (Spinner) findViewById(R.id.ardep);
sItems.setAdapter(adapter);
List<String> spinnerArray2 = new ArrayList<String>();
spinnerArray2.add("Running");
spinnerArray2.add("Walking");
spinnerArray2.add("Cycling");
spinnerArray2.add("Roller skating");
spinnerArray2.add("Skateboarding");
spinnerArray2.add("Kickbiking");
spinnerArray2.add("Teleporting");
ArrayAdapter<String> adapter2 = new ArrayAdapter<String>(
this, android.R.layout.simple_spinner_item, spinnerArray2);
adapter2.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item);
actCombo = (Spinner) findViewById(R.id.actCombo);
actCombo.setAdapter(adapter2);
Calendar rightNow = Calendar.getInstance();
int currentHour = rightNow.get(Calendar.HOUR_OF_DAY);
if (currentHour < 12) {
actCombo.setSelection(GetInfo.arract - 1);
sItems.setSelection(0);
} else {
actCombo.setSelection(GetInfo.depact - 1);
sItems.setSelection(1);
}
locationManager = (LocationManager) getSystemService(Context.LOCATION_SERVICE);
if (ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION) != PackageManager.PERMISSION_GRANTED &&
ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_COARSE_LOCATION) != PackageManager.PERMISSION_GRANTED) {
return;
}
locationManager.requestLocationUpdates(LocationManager.GPS_PROVIDER,
3000,
1, this);
SupportMapFragment mapFragment = (SupportMapFragment) getSupportFragmentManager().findFragmentById(R.id.mapview);
mapFragment.getMapAsync(this);
line = new PolylineOptions().width(3).color(Color.BLUE);
DrawerLayout drawer = (DrawerLayout) findViewById(R.id.drawer_layout);
ActionBarDrawerToggle toggle = new ActionBarDrawerToggle(
this, drawer, toolbar, R.string.navigation_drawer_open, R.string.navigation_drawer_close);
drawer.addDrawerListener(toggle);
toggle.syncState();
navigationView = (NavigationView) findViewById(R.id.nav_view);
headerView = navigationView.getHeaderView(0);
fullnameside = (TextView) headerView.findViewById(R.id.fullnameside);
emailside = (TextView) headerView.findViewById(R.id.emailside);
fullnameside.setText("" + GetInfo.fullname);
emailside.setText("" + GetInfo.email);
navigationView.setNavigationItemSelectedListener(this);
navigationView.getMenu().getItem(1).setChecked(true);
//Toast.makeText(this.getBaseContext(), ""+ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION), Toast.LENGTH_SHORT).show();
}
private void getLocation() {
//Toast.makeText(getBaseContext(), "e paides", Toast.LENGTH_SHORT).show();
String locationProvider = LocationManager.NETWORK_PROVIDER;
if (ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION) != PackageManager.PERMISSION_GRANTED && ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_COARSE_LOCATION) != PackageManager.PERMISSION_GRANTED) {
// TODO: Consider calling
// ActivityCompat#requestPermissions
// here to request the missing permissions, and then overriding
// public void onRequestPermissionsResult(int requestCode, String permissions,
// int grantResults)
// to handle the case where the user grants the permission. See the documentation
// for ActivityCompat#requestPermissions for more details.
return;
}
Location location = locationManager.getLastKnownLocation(locationProvider);
try {
line.add(new LatLng(location.getLatitude(), location.getLongitude()));
GMap.addMarker(new MarkerOptions().position(new LatLng(location.getLatitude(), location.getLongitude())).title(""));
GMap.animateCamera(CameraUpdateFactory.newLatLngZoom(new LatLng(location.getLatitude(), location.getLongitude()), 16.0f));
steps++;
getloc = true;
} catch (NullPointerException e) {
//Toast.makeText(this.getBaseContext(), "gyhg" + e.toString(), Toast.LENGTH_LONG).show();
}
}
public void StopTrip(View v) {
AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setMessage("Stop and upload your trip?")
.setCancelable(true)
.setNegativeButton("NO", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
}
})
.setPositiveButton("YES", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
float finalDistance = (float) (distance / 1000.0);
if (finalDistance < 2) {
Intent myIntent = new Intent(Map.this, Profile.class);
startActivity(myIntent);
}
TimeBuff += MillisecondTime;
handler.removeCallbacks(runnable);
// newActL.setVisibility(View.GONE);
// startActL.setVisibility(View.VISIBLE);
enabledActivity = false;
//database post
try {
Date currentTime = Calendar.getInstance().getTime();
SimpleDateFormat df = new SimpleDateFormat("yyyy-MM-dd");
String formattedDate = df.format(currentTime);
Date currentTime2 = Calendar.getInstance().getTime();
SimpleDateFormat df2 = new SimpleDateFormat("yyyy-MM-dd%20HH:mm:ss");
dateActEnd = df2.format(currentTime2);
String act = actCombo.getSelectedItem().toString();
String act_id = "7";
switch (act) {
case "Running":
act_id = "1";
break;
case "Walking":
act_id = "2";
break;
case "Cycling":
act_id = "3";
break;
case "Roller skating":
act_id = "4";
break;
case "Skateboarding":
act_id = "5";
break;
case "Kickbiking":
act_id = "6";
break;
case "Teleporting":
act_id = "7";
break;
}
String direcor;
if (sItems.getSelectedItem().toString().equals("Arrival"))
direcor = "arrival";
else
direcor = "departure";
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build();
StrictMode.setThreadPolicy(policy);
// String.format("%.1f", finalDistance)
URL obj = new URL("https://gekon.technologypark.cz/api/v1/record/create?user=" + LoginInfo.UserID
+ "&date=" + formattedDate + "&distance=" + String.format("%.1f", finalDistance) + "&direction=" + direcor
+ "&activity=" + act_id + "&polyline=" + PolyUtil.encode(line.getPoints()) + "&start=" + dateActStart
+ "&end=" + dateActEnd + "&source=mobileapp");
HttpsURLConnection conn = (HttpsURLConnection) obj.openConnection();
conn.setRequestMethod("POST");
conn.setRequestProperty("ApiSecret", LoginInfo.ApiSecret);
conn.connect();
BufferedReader br = new BufferedReader(new InputStreamReader((conn.getInputStream())));
StringBuilder sb = new StringBuilder();
String output;
while ((output = br.readLine()) != null)
sb.append(output);
JSONObject jsonObj = new JSONObject(sb.toString());
JSONObject curRecord = new JSONObject(jsonObj.getString("data"));
Trips.datet.add(currentTime);
Trips.datestr.add(formattedDate);
Trips.act.add(act_id);
Trips.tripType.add(sItems.getSelectedItem().toString());
Trips.dist.add(String.format("%.1f", finalDistance));
Trips.trip_ids.add(curRecord.getString("trip_id"));
Trips.calc(++Trips.points);
TripsCalendarInfo.datet.add(currentTime);
TripsCalendarInfo.act.add(act_id);
TripsCalendarInfo.act_str.add(act);
TripsCalendarInfo.tripType.add(sItems.getSelectedItem().toString());
TripsCalendarInfo.dist.add(String.format("%.1f", finalDistance));
TripsCalendarInfo.datestr.add(formattedDate);
TripsCalendarInfo.trip_ids.add(curRecord.getString("trip_id"));
TripsCalendarInfo.trip_source.add("mobileapp");
TripsCalendarInfo.polyline.add(PolyUtil.encode(line.getPoints()));
TripsCalendarInfo.CanItrip();
float km_up = Float.parseFloat(TripsInfo.km.get(TripsInfo.userRank - 1)) + finalDistance;
int trip_up = Integer.parseInt(TripsInfo.trips.get(TripsInfo.userRank - 1)) + 1;
TripsInfo.trips.set(TripsInfo.userRank - 1, "" + trip_up);
TripsInfo.km.set(TripsInfo.userRank - 1, String.format("%.1f", km_up));
TripsInfo.rankSort();
getloc = false;
Intent myIntent = new Intent(Map.this, Profile.class);
startActivity(myIntent);
} catch (Exception e) {
Toast.makeText(getBaseContext(), "Error upload, please check your options at gear button", Toast.LENGTH_LONG).show();
}
}
});
float finalDistance = (float) (distance / 1000.0);
if (finalDistance < 2) {
builder.setMessage("Your trip is below 2km and it will not be counted.nAre you sure you want to stop?");
}
AlertDialog alert = builder.create();
alert.show();
}
public void StartAct(View v) {
timer.setVisibility(View.VISIBLE);
dis.setVisibility(View.VISIBLE);
newActB.setVisibility(View.GONE);
stopActB.setVisibility(View.VISIBLE);
//gearMap.setVisibility(View.GONE);
navigationView.setVisibility(View.GONE);
//headerView.setSystemUiVisibility(View.SYSTEM_UI_FLAG_HIDE_NAVIGATION);
toolbar.setNavigationIcon(null); // to hide Navigation icon
//toolbar.setDisplayHomeAsUpEnabled(false);
handler = new Handler();
Date currentTime = Calendar.getInstance().getTime();
SimpleDateFormat df = new SimpleDateFormat("yyyy-MM-dd%20HH:mm:ss");
dateActStart = df.format(currentTime);
StartTime = SystemClock.uptimeMillis();
handler.postDelayed(runnable, 0);
startService(new Intent(this, LocationService.class));
//enabledActivity = true;
}
public void ChangeAct(View v) {
if (newActL.getVisibility() == View.GONE) {
newActL.setVisibility(View.VISIBLE);
startActL.setVisibility(View.GONE);
} else {
newActL.setVisibility(View.GONE);
startActL.setVisibility(View.VISIBLE);
}
}
public Runnable runnable = new Runnable() {
public void run() {
MillisecondTime = SystemClock.uptimeMillis() - StartTime;
UpdateTime = TimeBuff + MillisecondTime;
Seconds = (int) (UpdateTime / 1000);
Minutes = Seconds / 60;
Hours = Minutes / 60;
Seconds = Seconds % 60;
Minutes = Minutes % 60;
MilliSeconds = (int) (UpdateTime % 100);
timer.setText("Time: " + String.format("%02d", Hours) + ":"
+ String.format("%02d", Minutes) + ":"
+ String.format("%02d", Seconds));
handler.postDelayed(this, 950);
}
};
@Override
public void onMapReady(GoogleMap map) {
GMap = map;
GMap.moveCamera(CameraUpdateFactory.newLatLngZoom(new LatLng(49.8117806, 15.6970293), 6.0f));
if (!getloc)
getLocation();
}

add a comment |
I have a program that has one activity which the user can record his trip by pressing a button from one place to an other.I want somehow to make a wear app that connects with this activity so the user can press that button from the smartwatch and basically do whatever the android activity does.
Here is the code in case it's helpful
Map.java
public class Map extends AppCompatActivity implements LocationListener, OnMapReadyCallback, NavigationView.OnNavigationItemSelectedListener {
//VARIABLES
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.map);
//CALL SERVICE
Intent serviceIntent=new Intent(this, MyLocationService.class);
startService(serviceIntent);
getWindow().addFlags(WindowManager.LayoutParams.FLAG_KEEP_SCREEN_ON);
toolbar = (Toolbar) findViewById(R.id.toolbar);
setSupportActionBar(toolbar);
getSupportActionBar().setDisplayShowTitleEnabled(false);
toolbar.setTitle("");
toolbar.setSubtitle("");
//gpsStatus = (TextView) findViewById(R.id.gpsStatus);
dis = (TextView) findViewById(R.id.distancePreview);
newActL = (RelativeLayout) findViewById(R.id.newAct);
startActL = (RelativeLayout) findViewById(R.id.Act);
timer = (TextView) findViewById(R.id.timePreview);
newActB = (ImageButton) findViewById(R.id.newActB);
stopActB = (ImageButton) findViewById(R.id.stopActB);
gearMap = (ImageButton) findViewById(R.id.gearmap);
List<String> spinnerArray = new ArrayList<String>();
spinnerArray.add("Arrival");
spinnerArray.add("Departure");
ArrayAdapter<String> adapter = new ArrayAdapter<String>(
this, android.R.layout.simple_spinner_item, spinnerArray);
adapter.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item);
sItems = (Spinner) findViewById(R.id.ardep);
sItems.setAdapter(adapter);
List<String> spinnerArray2 = new ArrayList<String>();
spinnerArray2.add("Running");
spinnerArray2.add("Walking");
spinnerArray2.add("Cycling");
spinnerArray2.add("Roller skating");
spinnerArray2.add("Skateboarding");
spinnerArray2.add("Kickbiking");
spinnerArray2.add("Teleporting");
ArrayAdapter<String> adapter2 = new ArrayAdapter<String>(
this, android.R.layout.simple_spinner_item, spinnerArray2);
adapter2.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item);
actCombo = (Spinner) findViewById(R.id.actCombo);
actCombo.setAdapter(adapter2);
Calendar rightNow = Calendar.getInstance();
int currentHour = rightNow.get(Calendar.HOUR_OF_DAY);
if (currentHour < 12) {
actCombo.setSelection(GetInfo.arract - 1);
sItems.setSelection(0);
} else {
actCombo.setSelection(GetInfo.depact - 1);
sItems.setSelection(1);
}
locationManager = (LocationManager) getSystemService(Context.LOCATION_SERVICE);
if (ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION) != PackageManager.PERMISSION_GRANTED &&
ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_COARSE_LOCATION) != PackageManager.PERMISSION_GRANTED) {
return;
}
locationManager.requestLocationUpdates(LocationManager.GPS_PROVIDER,
3000,
1, this);
SupportMapFragment mapFragment = (SupportMapFragment) getSupportFragmentManager().findFragmentById(R.id.mapview);
mapFragment.getMapAsync(this);
line = new PolylineOptions().width(3).color(Color.BLUE);
DrawerLayout drawer = (DrawerLayout) findViewById(R.id.drawer_layout);
ActionBarDrawerToggle toggle = new ActionBarDrawerToggle(
this, drawer, toolbar, R.string.navigation_drawer_open, R.string.navigation_drawer_close);
drawer.addDrawerListener(toggle);
toggle.syncState();
navigationView = (NavigationView) findViewById(R.id.nav_view);
headerView = navigationView.getHeaderView(0);
fullnameside = (TextView) headerView.findViewById(R.id.fullnameside);
emailside = (TextView) headerView.findViewById(R.id.emailside);
fullnameside.setText("" + GetInfo.fullname);
emailside.setText("" + GetInfo.email);
navigationView.setNavigationItemSelectedListener(this);
navigationView.getMenu().getItem(1).setChecked(true);
//Toast.makeText(this.getBaseContext(), ""+ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION), Toast.LENGTH_SHORT).show();
}
private void getLocation() {
//Toast.makeText(getBaseContext(), "e paides", Toast.LENGTH_SHORT).show();
String locationProvider = LocationManager.NETWORK_PROVIDER;
if (ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION) != PackageManager.PERMISSION_GRANTED && ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_COARSE_LOCATION) != PackageManager.PERMISSION_GRANTED) {
// TODO: Consider calling
// ActivityCompat#requestPermissions
// here to request the missing permissions, and then overriding
// public void onRequestPermissionsResult(int requestCode, String permissions,
// int grantResults)
// to handle the case where the user grants the permission. See the documentation
// for ActivityCompat#requestPermissions for more details.
return;
}
Location location = locationManager.getLastKnownLocation(locationProvider);
try {
line.add(new LatLng(location.getLatitude(), location.getLongitude()));
GMap.addMarker(new MarkerOptions().position(new LatLng(location.getLatitude(), location.getLongitude())).title(""));
GMap.animateCamera(CameraUpdateFactory.newLatLngZoom(new LatLng(location.getLatitude(), location.getLongitude()), 16.0f));
steps++;
getloc = true;
} catch (NullPointerException e) {
//Toast.makeText(this.getBaseContext(), "gyhg" + e.toString(), Toast.LENGTH_LONG).show();
}
}
public void StopTrip(View v) {
AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setMessage("Stop and upload your trip?")
.setCancelable(true)
.setNegativeButton("NO", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
}
})
.setPositiveButton("YES", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
float finalDistance = (float) (distance / 1000.0);
if (finalDistance < 2) {
Intent myIntent = new Intent(Map.this, Profile.class);
startActivity(myIntent);
}
TimeBuff += MillisecondTime;
handler.removeCallbacks(runnable);
// newActL.setVisibility(View.GONE);
// startActL.setVisibility(View.VISIBLE);
enabledActivity = false;
//database post
try {
Date currentTime = Calendar.getInstance().getTime();
SimpleDateFormat df = new SimpleDateFormat("yyyy-MM-dd");
String formattedDate = df.format(currentTime);
Date currentTime2 = Calendar.getInstance().getTime();
SimpleDateFormat df2 = new SimpleDateFormat("yyyy-MM-dd%20HH:mm:ss");
dateActEnd = df2.format(currentTime2);
String act = actCombo.getSelectedItem().toString();
String act_id = "7";
switch (act) {
case "Running":
act_id = "1";
break;
case "Walking":
act_id = "2";
break;
case "Cycling":
act_id = "3";
break;
case "Roller skating":
act_id = "4";
break;
case "Skateboarding":
act_id = "5";
break;
case "Kickbiking":
act_id = "6";
break;
case "Teleporting":
act_id = "7";
break;
}
String direcor;
if (sItems.getSelectedItem().toString().equals("Arrival"))
direcor = "arrival";
else
direcor = "departure";
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build();
StrictMode.setThreadPolicy(policy);
// String.format("%.1f", finalDistance)
URL obj = new URL("https://gekon.technologypark.cz/api/v1/record/create?user=" + LoginInfo.UserID
+ "&date=" + formattedDate + "&distance=" + String.format("%.1f", finalDistance) + "&direction=" + direcor
+ "&activity=" + act_id + "&polyline=" + PolyUtil.encode(line.getPoints()) + "&start=" + dateActStart
+ "&end=" + dateActEnd + "&source=mobileapp");
HttpsURLConnection conn = (HttpsURLConnection) obj.openConnection();
conn.setRequestMethod("POST");
conn.setRequestProperty("ApiSecret", LoginInfo.ApiSecret);
conn.connect();
BufferedReader br = new BufferedReader(new InputStreamReader((conn.getInputStream())));
StringBuilder sb = new StringBuilder();
String output;
while ((output = br.readLine()) != null)
sb.append(output);
JSONObject jsonObj = new JSONObject(sb.toString());
JSONObject curRecord = new JSONObject(jsonObj.getString("data"));
Trips.datet.add(currentTime);
Trips.datestr.add(formattedDate);
Trips.act.add(act_id);
Trips.tripType.add(sItems.getSelectedItem().toString());
Trips.dist.add(String.format("%.1f", finalDistance));
Trips.trip_ids.add(curRecord.getString("trip_id"));
Trips.calc(++Trips.points);
TripsCalendarInfo.datet.add(currentTime);
TripsCalendarInfo.act.add(act_id);
TripsCalendarInfo.act_str.add(act);
TripsCalendarInfo.tripType.add(sItems.getSelectedItem().toString());
TripsCalendarInfo.dist.add(String.format("%.1f", finalDistance));
TripsCalendarInfo.datestr.add(formattedDate);
TripsCalendarInfo.trip_ids.add(curRecord.getString("trip_id"));
TripsCalendarInfo.trip_source.add("mobileapp");
TripsCalendarInfo.polyline.add(PolyUtil.encode(line.getPoints()));
TripsCalendarInfo.CanItrip();
float km_up = Float.parseFloat(TripsInfo.km.get(TripsInfo.userRank - 1)) + finalDistance;
int trip_up = Integer.parseInt(TripsInfo.trips.get(TripsInfo.userRank - 1)) + 1;
TripsInfo.trips.set(TripsInfo.userRank - 1, "" + trip_up);
TripsInfo.km.set(TripsInfo.userRank - 1, String.format("%.1f", km_up));
TripsInfo.rankSort();
getloc = false;
Intent myIntent = new Intent(Map.this, Profile.class);
startActivity(myIntent);
} catch (Exception e) {
Toast.makeText(getBaseContext(), "Error upload, please check your options at gear button", Toast.LENGTH_LONG).show();
}
}
});
float finalDistance = (float) (distance / 1000.0);
if (finalDistance < 2) {
builder.setMessage("Your trip is below 2km and it will not be counted.nAre you sure you want to stop?");
}
AlertDialog alert = builder.create();
alert.show();
}
public void StartAct(View v) {
timer.setVisibility(View.VISIBLE);
dis.setVisibility(View.VISIBLE);
newActB.setVisibility(View.GONE);
stopActB.setVisibility(View.VISIBLE);
//gearMap.setVisibility(View.GONE);
navigationView.setVisibility(View.GONE);
//headerView.setSystemUiVisibility(View.SYSTEM_UI_FLAG_HIDE_NAVIGATION);
toolbar.setNavigationIcon(null); // to hide Navigation icon
//toolbar.setDisplayHomeAsUpEnabled(false);
handler = new Handler();
Date currentTime = Calendar.getInstance().getTime();
SimpleDateFormat df = new SimpleDateFormat("yyyy-MM-dd%20HH:mm:ss");
dateActStart = df.format(currentTime);
StartTime = SystemClock.uptimeMillis();
handler.postDelayed(runnable, 0);
startService(new Intent(this, LocationService.class));
//enabledActivity = true;
}
public void ChangeAct(View v) {
if (newActL.getVisibility() == View.GONE) {
newActL.setVisibility(View.VISIBLE);
startActL.setVisibility(View.GONE);
} else {
newActL.setVisibility(View.GONE);
startActL.setVisibility(View.VISIBLE);
}
}
public Runnable runnable = new Runnable() {
public void run() {
MillisecondTime = SystemClock.uptimeMillis() - StartTime;
UpdateTime = TimeBuff + MillisecondTime;
Seconds = (int) (UpdateTime / 1000);
Minutes = Seconds / 60;
Hours = Minutes / 60;
Seconds = Seconds % 60;
Minutes = Minutes % 60;
MilliSeconds = (int) (UpdateTime % 100);
timer.setText("Time: " + String.format("%02d", Hours) + ":"
+ String.format("%02d", Minutes) + ":"
+ String.format("%02d", Seconds));
handler.postDelayed(this, 950);
}
};
@Override
public void onMapReady(GoogleMap map) {
GMap = map;
GMap.moveCamera(CameraUpdateFactory.newLatLngZoom(new LatLng(49.8117806, 15.6970293), 6.0f));
if (!getloc)
getLocation();
}

I have a program that has one activity which the user can record his trip by pressing a button from one place to an other.I want somehow to make a wear app that connects with this activity so the user can press that button from the smartwatch and basically do whatever the android activity does.
Here is the code in case it's helpful
Map.java
public class Map extends AppCompatActivity implements LocationListener, OnMapReadyCallback, NavigationView.OnNavigationItemSelectedListener {
//VARIABLES
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.map);
//CALL SERVICE
Intent serviceIntent=new Intent(this, MyLocationService.class);
startService(serviceIntent);
getWindow().addFlags(WindowManager.LayoutParams.FLAG_KEEP_SCREEN_ON);
toolbar = (Toolbar) findViewById(R.id.toolbar);
setSupportActionBar(toolbar);
getSupportActionBar().setDisplayShowTitleEnabled(false);
toolbar.setTitle("");
toolbar.setSubtitle("");
//gpsStatus = (TextView) findViewById(R.id.gpsStatus);
dis = (TextView) findViewById(R.id.distancePreview);
newActL = (RelativeLayout) findViewById(R.id.newAct);
startActL = (RelativeLayout) findViewById(R.id.Act);
timer = (TextView) findViewById(R.id.timePreview);
newActB = (ImageButton) findViewById(R.id.newActB);
stopActB = (ImageButton) findViewById(R.id.stopActB);
gearMap = (ImageButton) findViewById(R.id.gearmap);
List<String> spinnerArray = new ArrayList<String>();
spinnerArray.add("Arrival");
spinnerArray.add("Departure");
ArrayAdapter<String> adapter = new ArrayAdapter<String>(
this, android.R.layout.simple_spinner_item, spinnerArray);
adapter.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item);
sItems = (Spinner) findViewById(R.id.ardep);
sItems.setAdapter(adapter);
List<String> spinnerArray2 = new ArrayList<String>();
spinnerArray2.add("Running");
spinnerArray2.add("Walking");
spinnerArray2.add("Cycling");
spinnerArray2.add("Roller skating");
spinnerArray2.add("Skateboarding");
spinnerArray2.add("Kickbiking");
spinnerArray2.add("Teleporting");
ArrayAdapter<String> adapter2 = new ArrayAdapter<String>(
this, android.R.layout.simple_spinner_item, spinnerArray2);
adapter2.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item);
actCombo = (Spinner) findViewById(R.id.actCombo);
actCombo.setAdapter(adapter2);
Calendar rightNow = Calendar.getInstance();
int currentHour = rightNow.get(Calendar.HOUR_OF_DAY);
if (currentHour < 12) {
actCombo.setSelection(GetInfo.arract - 1);
sItems.setSelection(0);
} else {
actCombo.setSelection(GetInfo.depact - 1);
sItems.setSelection(1);
}
locationManager = (LocationManager) getSystemService(Context.LOCATION_SERVICE);
if (ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION) != PackageManager.PERMISSION_GRANTED &&
ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_COARSE_LOCATION) != PackageManager.PERMISSION_GRANTED) {
return;
}
locationManager.requestLocationUpdates(LocationManager.GPS_PROVIDER,
3000,
1, this);
SupportMapFragment mapFragment = (SupportMapFragment) getSupportFragmentManager().findFragmentById(R.id.mapview);
mapFragment.getMapAsync(this);
line = new PolylineOptions().width(3).color(Color.BLUE);
DrawerLayout drawer = (DrawerLayout) findViewById(R.id.drawer_layout);
ActionBarDrawerToggle toggle = new ActionBarDrawerToggle(
this, drawer, toolbar, R.string.navigation_drawer_open, R.string.navigation_drawer_close);
drawer.addDrawerListener(toggle);
toggle.syncState();
navigationView = (NavigationView) findViewById(R.id.nav_view);
headerView = navigationView.getHeaderView(0);
fullnameside = (TextView) headerView.findViewById(R.id.fullnameside);
emailside = (TextView) headerView.findViewById(R.id.emailside);
fullnameside.setText("" + GetInfo.fullname);
emailside.setText("" + GetInfo.email);
navigationView.setNavigationItemSelectedListener(this);
navigationView.getMenu().getItem(1).setChecked(true);
//Toast.makeText(this.getBaseContext(), ""+ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION), Toast.LENGTH_SHORT).show();
}
private void getLocation() {
//Toast.makeText(getBaseContext(), "e paides", Toast.LENGTH_SHORT).show();
String locationProvider = LocationManager.NETWORK_PROVIDER;
if (ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION) != PackageManager.PERMISSION_GRANTED && ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_COARSE_LOCATION) != PackageManager.PERMISSION_GRANTED) {
// TODO: Consider calling
// ActivityCompat#requestPermissions
// here to request the missing permissions, and then overriding
// public void onRequestPermissionsResult(int requestCode, String permissions,
// int grantResults)
// to handle the case where the user grants the permission. See the documentation
// for ActivityCompat#requestPermissions for more details.
return;
}
Location location = locationManager.getLastKnownLocation(locationProvider);
try {
line.add(new LatLng(location.getLatitude(), location.getLongitude()));
GMap.addMarker(new MarkerOptions().position(new LatLng(location.getLatitude(), location.getLongitude())).title(""));
GMap.animateCamera(CameraUpdateFactory.newLatLngZoom(new LatLng(location.getLatitude(), location.getLongitude()), 16.0f));
steps++;
getloc = true;
} catch (NullPointerException e) {
//Toast.makeText(this.getBaseContext(), "gyhg" + e.toString(), Toast.LENGTH_LONG).show();
}
}
public void StopTrip(View v) {
AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setMessage("Stop and upload your trip?")
.setCancelable(true)
.setNegativeButton("NO", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
}
})
.setPositiveButton("YES", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
float finalDistance = (float) (distance / 1000.0);
if (finalDistance < 2) {
Intent myIntent = new Intent(Map.this, Profile.class);
startActivity(myIntent);
}
TimeBuff += MillisecondTime;
handler.removeCallbacks(runnable);
// newActL.setVisibility(View.GONE);
// startActL.setVisibility(View.VISIBLE);
enabledActivity = false;
//database post
try {
Date currentTime = Calendar.getInstance().getTime();
SimpleDateFormat df = new SimpleDateFormat("yyyy-MM-dd");
String formattedDate = df.format(currentTime);
Date currentTime2 = Calendar.getInstance().getTime();
SimpleDateFormat df2 = new SimpleDateFormat("yyyy-MM-dd%20HH:mm:ss");
dateActEnd = df2.format(currentTime2);
String act = actCombo.getSelectedItem().toString();
String act_id = "7";
switch (act) {
case "Running":
act_id = "1";
break;
case "Walking":
act_id = "2";
break;
case "Cycling":
act_id = "3";
break;
case "Roller skating":
act_id = "4";
break;
case "Skateboarding":
act_id = "5";
break;
case "Kickbiking":
act_id = "6";
break;
case "Teleporting":
act_id = "7";
break;
}
String direcor;
if (sItems.getSelectedItem().toString().equals("Arrival"))
direcor = "arrival";
else
direcor = "departure";
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build();
StrictMode.setThreadPolicy(policy);
// String.format("%.1f", finalDistance)
URL obj = new URL("https://gekon.technologypark.cz/api/v1/record/create?user=" + LoginInfo.UserID
+ "&date=" + formattedDate + "&distance=" + String.format("%.1f", finalDistance) + "&direction=" + direcor
+ "&activity=" + act_id + "&polyline=" + PolyUtil.encode(line.getPoints()) + "&start=" + dateActStart
+ "&end=" + dateActEnd + "&source=mobileapp");
HttpsURLConnection conn = (HttpsURLConnection) obj.openConnection();
conn.setRequestMethod("POST");
conn.setRequestProperty("ApiSecret", LoginInfo.ApiSecret);
conn.connect();
BufferedReader br = new BufferedReader(new InputStreamReader((conn.getInputStream())));
StringBuilder sb = new StringBuilder();
String output;
while ((output = br.readLine()) != null)
sb.append(output);
JSONObject jsonObj = new JSONObject(sb.toString());
JSONObject curRecord = new JSONObject(jsonObj.getString("data"));
Trips.datet.add(currentTime);
Trips.datestr.add(formattedDate);
Trips.act.add(act_id);
Trips.tripType.add(sItems.getSelectedItem().toString());
Trips.dist.add(String.format("%.1f", finalDistance));
Trips.trip_ids.add(curRecord.getString("trip_id"));
Trips.calc(++Trips.points);
TripsCalendarInfo.datet.add(currentTime);
TripsCalendarInfo.act.add(act_id);
TripsCalendarInfo.act_str.add(act);
TripsCalendarInfo.tripType.add(sItems.getSelectedItem().toString());
TripsCalendarInfo.dist.add(String.format("%.1f", finalDistance));
TripsCalendarInfo.datestr.add(formattedDate);
TripsCalendarInfo.trip_ids.add(curRecord.getString("trip_id"));
TripsCalendarInfo.trip_source.add("mobileapp");
TripsCalendarInfo.polyline.add(PolyUtil.encode(line.getPoints()));
TripsCalendarInfo.CanItrip();
float km_up = Float.parseFloat(TripsInfo.km.get(TripsInfo.userRank - 1)) + finalDistance;
int trip_up = Integer.parseInt(TripsInfo.trips.get(TripsInfo.userRank - 1)) + 1;
TripsInfo.trips.set(TripsInfo.userRank - 1, "" + trip_up);
TripsInfo.km.set(TripsInfo.userRank - 1, String.format("%.1f", km_up));
TripsInfo.rankSort();
getloc = false;
Intent myIntent = new Intent(Map.this, Profile.class);
startActivity(myIntent);
} catch (Exception e) {
Toast.makeText(getBaseContext(), "Error upload, please check your options at gear button", Toast.LENGTH_LONG).show();
}
}
});
float finalDistance = (float) (distance / 1000.0);
if (finalDistance < 2) {
builder.setMessage("Your trip is below 2km and it will not be counted.nAre you sure you want to stop?");
}
AlertDialog alert = builder.create();
alert.show();
}
public void StartAct(View v) {
timer.setVisibility(View.VISIBLE);
dis.setVisibility(View.VISIBLE);
newActB.setVisibility(View.GONE);
stopActB.setVisibility(View.VISIBLE);
//gearMap.setVisibility(View.GONE);
navigationView.setVisibility(View.GONE);
//headerView.setSystemUiVisibility(View.SYSTEM_UI_FLAG_HIDE_NAVIGATION);
toolbar.setNavigationIcon(null); // to hide Navigation icon
//toolbar.setDisplayHomeAsUpEnabled(false);
handler = new Handler();
Date currentTime = Calendar.getInstance().getTime();
SimpleDateFormat df = new SimpleDateFormat("yyyy-MM-dd%20HH:mm:ss");
dateActStart = df.format(currentTime);
StartTime = SystemClock.uptimeMillis();
handler.postDelayed(runnable, 0);
startService(new Intent(this, LocationService.class));
//enabledActivity = true;
}
public void ChangeAct(View v) {
if (newActL.getVisibility() == View.GONE) {
newActL.setVisibility(View.VISIBLE);
startActL.setVisibility(View.GONE);
} else {
newActL.setVisibility(View.GONE);
startActL.setVisibility(View.VISIBLE);
}
}
public Runnable runnable = new Runnable() {
public void run() {
MillisecondTime = SystemClock.uptimeMillis() - StartTime;
UpdateTime = TimeBuff + MillisecondTime;
Seconds = (int) (UpdateTime / 1000);
Minutes = Seconds / 60;
Hours = Minutes / 60;
Seconds = Seconds % 60;
Minutes = Minutes % 60;
MilliSeconds = (int) (UpdateTime % 100);
timer.setText("Time: " + String.format("%02d", Hours) + ":"
+ String.format("%02d", Minutes) + ":"
+ String.format("%02d", Seconds));
handler.postDelayed(this, 950);
}
};
@Override
public void onMapReady(GoogleMap map) {
GMap = map;
GMap.moveCamera(CameraUpdateFactory.newLatLngZoom(new LatLng(49.8117806, 15.6970293), 6.0f));
if (!getloc)
getLocation();
}


edited Nov 2 at 8:42
asked Nov 2 at 8:04


Alex Kolydas
304114
304114
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Using the Maps SDK for Android, you can create a map-based wearable app
The following code sample enables ambient mode in the app and in the map:
public class MainActivity extends WearableActivity
implements OnMapReadyCallback, GoogleMap.OnMapLongClickListener {
private MapFragment mMapFragment;
public void onCreate(Bundle savedState) {
super.onCreate(savedState);
// Enable ambient support, so the map remains visible in a simplified,
// low-color display when the user is no longer actively using the app
// and the app is still visible on the watch face.
setAmbientEnabled();
// ... Perform other activity setup processes here too ...
}
/**
* Starts ambient mode on the map.
* The API swaps to a non-interactive and low-color rendering of the map
* when the user is no longer actively using the app.
*/
@Override
public void onEnterAmbient(Bundle ambientDetails) {
super.onEnterAmbient(ambientDetails);
mMapFragment.onEnterAmbient(ambientDetails);
}
/**
* Exits ambient mode on the map.
* The API swaps to the normal rendering of the map when the user starts
* actively using the app.
*/
@Override
public void onExitAmbient() {
super.onExitAmbient();
mMapFragment.onExitAmbient();
}
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53114763%2fis-it-possible-to-make-a-wear-app-that-connects-to-my-android-app-activitymaps%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Using the Maps SDK for Android, you can create a map-based wearable app
The following code sample enables ambient mode in the app and in the map:
public class MainActivity extends WearableActivity
implements OnMapReadyCallback, GoogleMap.OnMapLongClickListener {
private MapFragment mMapFragment;
public void onCreate(Bundle savedState) {
super.onCreate(savedState);
// Enable ambient support, so the map remains visible in a simplified,
// low-color display when the user is no longer actively using the app
// and the app is still visible on the watch face.
setAmbientEnabled();
// ... Perform other activity setup processes here too ...
}
/**
* Starts ambient mode on the map.
* The API swaps to a non-interactive and low-color rendering of the map
* when the user is no longer actively using the app.
*/
@Override
public void onEnterAmbient(Bundle ambientDetails) {
super.onEnterAmbient(ambientDetails);
mMapFragment.onEnterAmbient(ambientDetails);
}
/**
* Exits ambient mode on the map.
* The API swaps to the normal rendering of the map when the user starts
* actively using the app.
*/
@Override
public void onExitAmbient() {
super.onExitAmbient();
mMapFragment.onExitAmbient();
}
}
add a comment |
Using the Maps SDK for Android, you can create a map-based wearable app
The following code sample enables ambient mode in the app and in the map:
public class MainActivity extends WearableActivity
implements OnMapReadyCallback, GoogleMap.OnMapLongClickListener {
private MapFragment mMapFragment;
public void onCreate(Bundle savedState) {
super.onCreate(savedState);
// Enable ambient support, so the map remains visible in a simplified,
// low-color display when the user is no longer actively using the app
// and the app is still visible on the watch face.
setAmbientEnabled();
// ... Perform other activity setup processes here too ...
}
/**
* Starts ambient mode on the map.
* The API swaps to a non-interactive and low-color rendering of the map
* when the user is no longer actively using the app.
*/
@Override
public void onEnterAmbient(Bundle ambientDetails) {
super.onEnterAmbient(ambientDetails);
mMapFragment.onEnterAmbient(ambientDetails);
}
/**
* Exits ambient mode on the map.
* The API swaps to the normal rendering of the map when the user starts
* actively using the app.
*/
@Override
public void onExitAmbient() {
super.onExitAmbient();
mMapFragment.onExitAmbient();
}
}
add a comment |
Using the Maps SDK for Android, you can create a map-based wearable app
The following code sample enables ambient mode in the app and in the map:
public class MainActivity extends WearableActivity
implements OnMapReadyCallback, GoogleMap.OnMapLongClickListener {
private MapFragment mMapFragment;
public void onCreate(Bundle savedState) {
super.onCreate(savedState);
// Enable ambient support, so the map remains visible in a simplified,
// low-color display when the user is no longer actively using the app
// and the app is still visible on the watch face.
setAmbientEnabled();
// ... Perform other activity setup processes here too ...
}
/**
* Starts ambient mode on the map.
* The API swaps to a non-interactive and low-color rendering of the map
* when the user is no longer actively using the app.
*/
@Override
public void onEnterAmbient(Bundle ambientDetails) {
super.onEnterAmbient(ambientDetails);
mMapFragment.onEnterAmbient(ambientDetails);
}
/**
* Exits ambient mode on the map.
* The API swaps to the normal rendering of the map when the user starts
* actively using the app.
*/
@Override
public void onExitAmbient() {
super.onExitAmbient();
mMapFragment.onExitAmbient();
}
}
Using the Maps SDK for Android, you can create a map-based wearable app
The following code sample enables ambient mode in the app and in the map:
public class MainActivity extends WearableActivity
implements OnMapReadyCallback, GoogleMap.OnMapLongClickListener {
private MapFragment mMapFragment;
public void onCreate(Bundle savedState) {
super.onCreate(savedState);
// Enable ambient support, so the map remains visible in a simplified,
// low-color display when the user is no longer actively using the app
// and the app is still visible on the watch face.
setAmbientEnabled();
// ... Perform other activity setup processes here too ...
}
/**
* Starts ambient mode on the map.
* The API swaps to a non-interactive and low-color rendering of the map
* when the user is no longer actively using the app.
*/
@Override
public void onEnterAmbient(Bundle ambientDetails) {
super.onEnterAmbient(ambientDetails);
mMapFragment.onEnterAmbient(ambientDetails);
}
/**
* Exits ambient mode on the map.
* The API swaps to the normal rendering of the map when the user starts
* actively using the app.
*/
@Override
public void onExitAmbient() {
super.onExitAmbient();
mMapFragment.onExitAmbient();
}
}
answered Nov 12 at 9:18
Sachin Kasaraddi
28027
28027
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53114763%2fis-it-possible-to-make-a-wear-app-that-connects-to-my-android-app-activitymaps%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
D,b0Tm3wHWfcN,SSgR,Wu6r,j7usBZVT,7AOL,Zzps,zUueyuQ H6jIgI2mlEx