Detect if a graph is bipartite using union find (aka disjoint sets)
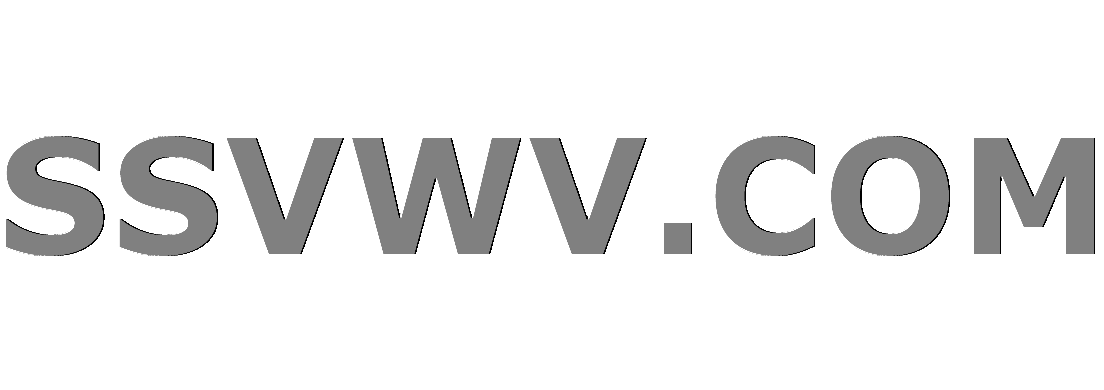
Multi tool use
up vote
-2
down vote
favorite
I'm doing a problem on Spoj that basically reduces to detecting if a graph is bipartite. I'm trying to just color the graph using dfs, but it is too slow. Some guy comments this
No bfs, no dfs, no bipartie graph. Simple Union-Find Set would make it (with speed, indeed).
Hint #1:
Cycle of even length does not affect the divisibility by 2 ( wow, so many i in a word ) of length of paths between two node.
Hint #2:
(SPOILER)
let dist[i] be the distance of a path from i to parent[i]. update it with the find and union function. It can be improved to make dist a bool array.
Could someone explain what he means with this? I think what he is trying to say is that for each node, you store the distance between the node and the representative element. Then if you try to merge two nodes in the same set, and if they have the same parity, then you create an odd cycle, so the graph cannot be bipartite. However, I don't understand how this would be implemented. How can you merge two sets while accounting for the distance? Wouldn't you have to look through the entire set to find all elements to update?
Link to the problem: https://www.spoj.com/problems/BUGLIFE/
algorithm bipartite disjoint-sets
add a comment |
up vote
-2
down vote
favorite
I'm doing a problem on Spoj that basically reduces to detecting if a graph is bipartite. I'm trying to just color the graph using dfs, but it is too slow. Some guy comments this
No bfs, no dfs, no bipartie graph. Simple Union-Find Set would make it (with speed, indeed).
Hint #1:
Cycle of even length does not affect the divisibility by 2 ( wow, so many i in a word ) of length of paths between two node.
Hint #2:
(SPOILER)
let dist[i] be the distance of a path from i to parent[i]. update it with the find and union function. It can be improved to make dist a bool array.
Could someone explain what he means with this? I think what he is trying to say is that for each node, you store the distance between the node and the representative element. Then if you try to merge two nodes in the same set, and if they have the same parity, then you create an odd cycle, so the graph cannot be bipartite. However, I don't understand how this would be implemented. How can you merge two sets while accounting for the distance? Wouldn't you have to look through the entire set to find all elements to update?
Link to the problem: https://www.spoj.com/problems/BUGLIFE/
algorithm bipartite disjoint-sets
@user3386109 Yes, but how would I do this using the disjoint set data structure (I'm not trying to to use disjoint sets to find the two sets that only connect with each other).
– Blubber
Nov 11 at 20:03
add a comment |
up vote
-2
down vote
favorite
up vote
-2
down vote
favorite
I'm doing a problem on Spoj that basically reduces to detecting if a graph is bipartite. I'm trying to just color the graph using dfs, but it is too slow. Some guy comments this
No bfs, no dfs, no bipartie graph. Simple Union-Find Set would make it (with speed, indeed).
Hint #1:
Cycle of even length does not affect the divisibility by 2 ( wow, so many i in a word ) of length of paths between two node.
Hint #2:
(SPOILER)
let dist[i] be the distance of a path from i to parent[i]. update it with the find and union function. It can be improved to make dist a bool array.
Could someone explain what he means with this? I think what he is trying to say is that for each node, you store the distance between the node and the representative element. Then if you try to merge two nodes in the same set, and if they have the same parity, then you create an odd cycle, so the graph cannot be bipartite. However, I don't understand how this would be implemented. How can you merge two sets while accounting for the distance? Wouldn't you have to look through the entire set to find all elements to update?
Link to the problem: https://www.spoj.com/problems/BUGLIFE/
algorithm bipartite disjoint-sets
I'm doing a problem on Spoj that basically reduces to detecting if a graph is bipartite. I'm trying to just color the graph using dfs, but it is too slow. Some guy comments this
No bfs, no dfs, no bipartie graph. Simple Union-Find Set would make it (with speed, indeed).
Hint #1:
Cycle of even length does not affect the divisibility by 2 ( wow, so many i in a word ) of length of paths between two node.
Hint #2:
(SPOILER)
let dist[i] be the distance of a path from i to parent[i]. update it with the find and union function. It can be improved to make dist a bool array.
Could someone explain what he means with this? I think what he is trying to say is that for each node, you store the distance between the node and the representative element. Then if you try to merge two nodes in the same set, and if they have the same parity, then you create an odd cycle, so the graph cannot be bipartite. However, I don't understand how this would be implemented. How can you merge two sets while accounting for the distance? Wouldn't you have to look through the entire set to find all elements to update?
Link to the problem: https://www.spoj.com/problems/BUGLIFE/
algorithm bipartite disjoint-sets
algorithm bipartite disjoint-sets
edited Nov 11 at 19:45
asked Nov 11 at 6:40
Blubber
366317
366317
@user3386109 Yes, but how would I do this using the disjoint set data structure (I'm not trying to to use disjoint sets to find the two sets that only connect with each other).
– Blubber
Nov 11 at 20:03
add a comment |
@user3386109 Yes, but how would I do this using the disjoint set data structure (I'm not trying to to use disjoint sets to find the two sets that only connect with each other).
– Blubber
Nov 11 at 20:03
@user3386109 Yes, but how would I do this using the disjoint set data structure (I'm not trying to to use disjoint sets to find the two sets that only connect with each other).
– Blubber
Nov 11 at 20:03
@user3386109 Yes, but how would I do this using the disjoint set data structure (I'm not trying to to use disjoint sets to find the two sets that only connect with each other).
– Blubber
Nov 11 at 20:03
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
Given a graph represented as an adjacency-list (i.e. a list of edges), you can determine if it's bipartite as follows:
- Initialize a disjoint-set data structure SETS, with a singleton set for each vertex. (If there is an even-length path between two vertices, then we will ultimately unify those two vertices into the same set, unless we return ꜰᴀʟꜱᴇ first.)
- Initialize a mapping MAP from each vertex to ɴɪʟ. (As we examine edges, we will populate MAP with a mapping from each vertex to one of its neighbors.)
- For each edge {u, v}:
- If u and v belong to the same set in SETS, then return ꜰᴀʟꜱᴇ.
- If MAP[u] = ɴɪʟ, set MAP[u] := v.
Otherwise, update SETS to unify v with MAP[u]. - If MAP[v] = ɴɪʟ, set MAP[v] := u.
Otherwise, update SETS to unify u with MAP[v].
- Return ᴛʀᴜᴇ.
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
Given a graph represented as an adjacency-list (i.e. a list of edges), you can determine if it's bipartite as follows:
- Initialize a disjoint-set data structure SETS, with a singleton set for each vertex. (If there is an even-length path between two vertices, then we will ultimately unify those two vertices into the same set, unless we return ꜰᴀʟꜱᴇ first.)
- Initialize a mapping MAP from each vertex to ɴɪʟ. (As we examine edges, we will populate MAP with a mapping from each vertex to one of its neighbors.)
- For each edge {u, v}:
- If u and v belong to the same set in SETS, then return ꜰᴀʟꜱᴇ.
- If MAP[u] = ɴɪʟ, set MAP[u] := v.
Otherwise, update SETS to unify v with MAP[u]. - If MAP[v] = ɴɪʟ, set MAP[v] := u.
Otherwise, update SETS to unify u with MAP[v].
- Return ᴛʀᴜᴇ.
add a comment |
up vote
1
down vote
Given a graph represented as an adjacency-list (i.e. a list of edges), you can determine if it's bipartite as follows:
- Initialize a disjoint-set data structure SETS, with a singleton set for each vertex. (If there is an even-length path between two vertices, then we will ultimately unify those two vertices into the same set, unless we return ꜰᴀʟꜱᴇ first.)
- Initialize a mapping MAP from each vertex to ɴɪʟ. (As we examine edges, we will populate MAP with a mapping from each vertex to one of its neighbors.)
- For each edge {u, v}:
- If u and v belong to the same set in SETS, then return ꜰᴀʟꜱᴇ.
- If MAP[u] = ɴɪʟ, set MAP[u] := v.
Otherwise, update SETS to unify v with MAP[u]. - If MAP[v] = ɴɪʟ, set MAP[v] := u.
Otherwise, update SETS to unify u with MAP[v].
- Return ᴛʀᴜᴇ.
add a comment |
up vote
1
down vote
up vote
1
down vote
Given a graph represented as an adjacency-list (i.e. a list of edges), you can determine if it's bipartite as follows:
- Initialize a disjoint-set data structure SETS, with a singleton set for each vertex. (If there is an even-length path between two vertices, then we will ultimately unify those two vertices into the same set, unless we return ꜰᴀʟꜱᴇ first.)
- Initialize a mapping MAP from each vertex to ɴɪʟ. (As we examine edges, we will populate MAP with a mapping from each vertex to one of its neighbors.)
- For each edge {u, v}:
- If u and v belong to the same set in SETS, then return ꜰᴀʟꜱᴇ.
- If MAP[u] = ɴɪʟ, set MAP[u] := v.
Otherwise, update SETS to unify v with MAP[u]. - If MAP[v] = ɴɪʟ, set MAP[v] := u.
Otherwise, update SETS to unify u with MAP[v].
- Return ᴛʀᴜᴇ.
Given a graph represented as an adjacency-list (i.e. a list of edges), you can determine if it's bipartite as follows:
- Initialize a disjoint-set data structure SETS, with a singleton set for each vertex. (If there is an even-length path between two vertices, then we will ultimately unify those two vertices into the same set, unless we return ꜰᴀʟꜱᴇ first.)
- Initialize a mapping MAP from each vertex to ɴɪʟ. (As we examine edges, we will populate MAP with a mapping from each vertex to one of its neighbors.)
- For each edge {u, v}:
- If u and v belong to the same set in SETS, then return ꜰᴀʟꜱᴇ.
- If MAP[u] = ɴɪʟ, set MAP[u] := v.
Otherwise, update SETS to unify v with MAP[u]. - If MAP[v] = ɴɪʟ, set MAP[v] := u.
Otherwise, update SETS to unify u with MAP[v].
- Return ᴛʀᴜᴇ.
answered Nov 12 at 6:30
ruakh
122k12196250
122k12196250
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53246453%2fdetect-if-a-graph-is-bipartite-using-union-find-aka-disjoint-sets%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
mbgaKor
@user3386109 Yes, but how would I do this using the disjoint set data structure (I'm not trying to to use disjoint sets to find the two sets that only connect with each other).
– Blubber
Nov 11 at 20:03