How to obtain products when a relationship meets the Laravel Eloquent condition
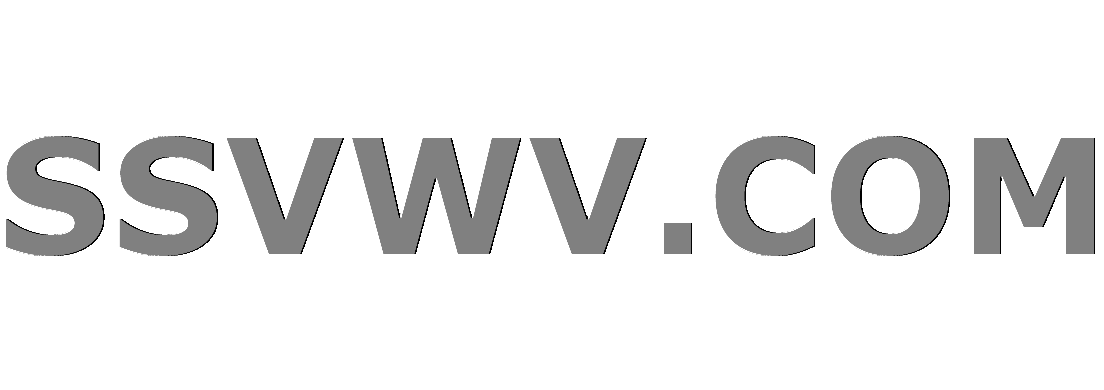
Multi tool use
up vote
0
down vote
favorite
I have the following relationship in the database, each table with its respective and related model.
I have products where each product has its recipe, which is composed of zero or more elements and the amount needed.
After this I have a warehouse table, where I have the current stock of the element, what I want is to obtain the products in which the amount needed in the recipe is less than the stock.
How could I obtain this data? it occurred to me to create a scope in the model products but I do not know how to perform the validation since they can be 0 or n elements in the recipe ?
Controller
Product::with('recipe')
->enableElements()->get();
Model Product
public function scopeEnableElements($query)
{
}
php laravel laravel-5 eloquent
add a comment |
up vote
0
down vote
favorite
I have the following relationship in the database, each table with its respective and related model.
I have products where each product has its recipe, which is composed of zero or more elements and the amount needed.
After this I have a warehouse table, where I have the current stock of the element, what I want is to obtain the products in which the amount needed in the recipe is less than the stock.
How could I obtain this data? it occurred to me to create a scope in the model products but I do not know how to perform the validation since they can be 0 or n elements in the recipe ?
Controller
Product::with('recipe')
->enableElements()->get();
Model Product
public function scopeEnableElements($query)
{
}
php laravel laravel-5 eloquent
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have the following relationship in the database, each table with its respective and related model.
I have products where each product has its recipe, which is composed of zero or more elements and the amount needed.
After this I have a warehouse table, where I have the current stock of the element, what I want is to obtain the products in which the amount needed in the recipe is less than the stock.
How could I obtain this data? it occurred to me to create a scope in the model products but I do not know how to perform the validation since they can be 0 or n elements in the recipe ?
Controller
Product::with('recipe')
->enableElements()->get();
Model Product
public function scopeEnableElements($query)
{
}
php laravel laravel-5 eloquent
I have the following relationship in the database, each table with its respective and related model.
I have products where each product has its recipe, which is composed of zero or more elements and the amount needed.
After this I have a warehouse table, where I have the current stock of the element, what I want is to obtain the products in which the amount needed in the recipe is less than the stock.
How could I obtain this data? it occurred to me to create a scope in the model products but I do not know how to perform the validation since they can be 0 or n elements in the recipe ?
Controller
Product::with('recipe')
->enableElements()->get();
Model Product
public function scopeEnableElements($query)
{
}
php laravel laravel-5 eloquent
php laravel laravel-5 eloquent
asked Nov 10 at 22:26
DarkFenix
305315
305315
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
accepted
You can query from Recipe
to WareHouse
by relating the element_id
.
Recipe model:
public function warehouses()
{
return $this->hasMany(AppModelsWareHouse::class, 'element_id', 'element_id');
}
WareHouse model:
public function recipes()
{
return $this->hasMany(AppModelsRecipe::class, 'element_id', 'element_id');
}
Controller code:
Product::whereHas('recipes', function($q) {
$q->whereHas('warehouses', function($query) {
$query->whereRaw('WareHouse.stock > Recipe.count'); // use table names here, not model names
});
})->get();
If you wanted to do it with a scope in the Recipe model:
public function scopeEnableElements($query)
{
$query->whereHas('warehouses', function($query2) {
$query2->whereRaw('WareHouse.stock > Recipe.count'); // use table names here, not model names
});
}
Then in your controller:
Product::whereHas('recipes', function($query) {
$query->enableElements();
})->get();
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
You can query from Recipe
to WareHouse
by relating the element_id
.
Recipe model:
public function warehouses()
{
return $this->hasMany(AppModelsWareHouse::class, 'element_id', 'element_id');
}
WareHouse model:
public function recipes()
{
return $this->hasMany(AppModelsRecipe::class, 'element_id', 'element_id');
}
Controller code:
Product::whereHas('recipes', function($q) {
$q->whereHas('warehouses', function($query) {
$query->whereRaw('WareHouse.stock > Recipe.count'); // use table names here, not model names
});
})->get();
If you wanted to do it with a scope in the Recipe model:
public function scopeEnableElements($query)
{
$query->whereHas('warehouses', function($query2) {
$query2->whereRaw('WareHouse.stock > Recipe.count'); // use table names here, not model names
});
}
Then in your controller:
Product::whereHas('recipes', function($query) {
$query->enableElements();
})->get();
add a comment |
up vote
1
down vote
accepted
You can query from Recipe
to WareHouse
by relating the element_id
.
Recipe model:
public function warehouses()
{
return $this->hasMany(AppModelsWareHouse::class, 'element_id', 'element_id');
}
WareHouse model:
public function recipes()
{
return $this->hasMany(AppModelsRecipe::class, 'element_id', 'element_id');
}
Controller code:
Product::whereHas('recipes', function($q) {
$q->whereHas('warehouses', function($query) {
$query->whereRaw('WareHouse.stock > Recipe.count'); // use table names here, not model names
});
})->get();
If you wanted to do it with a scope in the Recipe model:
public function scopeEnableElements($query)
{
$query->whereHas('warehouses', function($query2) {
$query2->whereRaw('WareHouse.stock > Recipe.count'); // use table names here, not model names
});
}
Then in your controller:
Product::whereHas('recipes', function($query) {
$query->enableElements();
})->get();
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
You can query from Recipe
to WareHouse
by relating the element_id
.
Recipe model:
public function warehouses()
{
return $this->hasMany(AppModelsWareHouse::class, 'element_id', 'element_id');
}
WareHouse model:
public function recipes()
{
return $this->hasMany(AppModelsRecipe::class, 'element_id', 'element_id');
}
Controller code:
Product::whereHas('recipes', function($q) {
$q->whereHas('warehouses', function($query) {
$query->whereRaw('WareHouse.stock > Recipe.count'); // use table names here, not model names
});
})->get();
If you wanted to do it with a scope in the Recipe model:
public function scopeEnableElements($query)
{
$query->whereHas('warehouses', function($query2) {
$query2->whereRaw('WareHouse.stock > Recipe.count'); // use table names here, not model names
});
}
Then in your controller:
Product::whereHas('recipes', function($query) {
$query->enableElements();
})->get();
You can query from Recipe
to WareHouse
by relating the element_id
.
Recipe model:
public function warehouses()
{
return $this->hasMany(AppModelsWareHouse::class, 'element_id', 'element_id');
}
WareHouse model:
public function recipes()
{
return $this->hasMany(AppModelsRecipe::class, 'element_id', 'element_id');
}
Controller code:
Product::whereHas('recipes', function($q) {
$q->whereHas('warehouses', function($query) {
$query->whereRaw('WareHouse.stock > Recipe.count'); // use table names here, not model names
});
})->get();
If you wanted to do it with a scope in the Recipe model:
public function scopeEnableElements($query)
{
$query->whereHas('warehouses', function($query2) {
$query2->whereRaw('WareHouse.stock > Recipe.count'); // use table names here, not model names
});
}
Then in your controller:
Product::whereHas('recipes', function($query) {
$query->enableElements();
})->get();
edited Nov 11 at 0:12
answered Nov 10 at 23:39
newUserName02
46838
46838
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53244037%2fhow-to-obtain-products-when-a-relationship-meets-the-laravel-eloquent-condition%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ReVDm Hxe8C sK A