Armadillo does not perform swap optimization
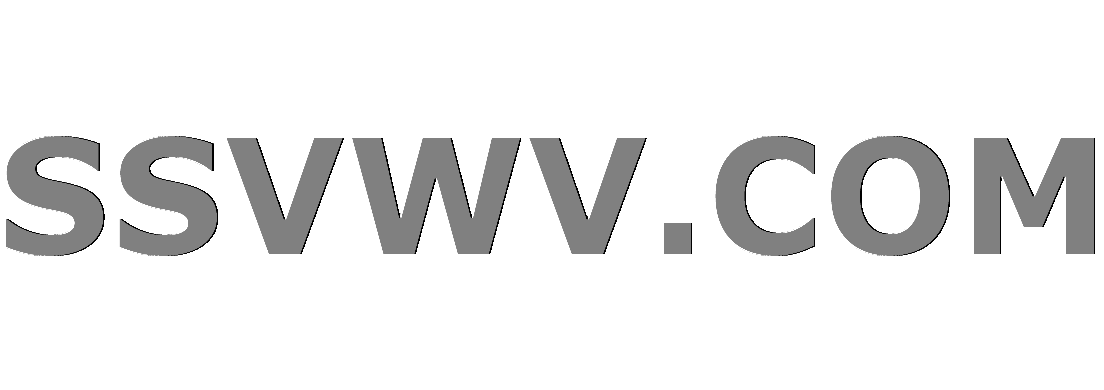
Multi tool use
up vote
1
down vote
favorite
How to swap Armadillo objects, e.g. arma::vec
s, without swapping contents?
void f5()
{
arma::vec x(10);
arma::vec y(10);
std::cout << &x[2] << ", " << &y[2] << "n";
x.swap(y);
std::cout << &x[2] << ", " << &y[2];
}
The above code outputs
0x24fbe50, 0x24fbef0
0x24fbe50, 0x24fbef0
Thank you!
c++ armadillo
add a comment |
up vote
1
down vote
favorite
How to swap Armadillo objects, e.g. arma::vec
s, without swapping contents?
void f5()
{
arma::vec x(10);
arma::vec y(10);
std::cout << &x[2] << ", " << &y[2] << "n";
x.swap(y);
std::cout << &x[2] << ", " << &y[2];
}
The above code outputs
0x24fbe50, 0x24fbef0
0x24fbe50, 0x24fbef0
Thank you!
c++ armadillo
Can you increase the vector size and try it again? Maybe they do silly memcpy if the array size is below some threshold.
– OZ17
Nov 10 at 22:56
@OZ17 Great! Seems swap optimization happens for >= 17 doubles on my machine. I'll accept it if you want to post your comment as an answer.
– user2961927
Nov 11 at 0:27
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
How to swap Armadillo objects, e.g. arma::vec
s, without swapping contents?
void f5()
{
arma::vec x(10);
arma::vec y(10);
std::cout << &x[2] << ", " << &y[2] << "n";
x.swap(y);
std::cout << &x[2] << ", " << &y[2];
}
The above code outputs
0x24fbe50, 0x24fbef0
0x24fbe50, 0x24fbef0
Thank you!
c++ armadillo
How to swap Armadillo objects, e.g. arma::vec
s, without swapping contents?
void f5()
{
arma::vec x(10);
arma::vec y(10);
std::cout << &x[2] << ", " << &y[2] << "n";
x.swap(y);
std::cout << &x[2] << ", " << &y[2];
}
The above code outputs
0x24fbe50, 0x24fbef0
0x24fbe50, 0x24fbef0
Thank you!
c++ armadillo
c++ armadillo
asked Nov 10 at 22:00
user2961927
430516
430516
Can you increase the vector size and try it again? Maybe they do silly memcpy if the array size is below some threshold.
– OZ17
Nov 10 at 22:56
@OZ17 Great! Seems swap optimization happens for >= 17 doubles on my machine. I'll accept it if you want to post your comment as an answer.
– user2961927
Nov 11 at 0:27
add a comment |
Can you increase the vector size and try it again? Maybe they do silly memcpy if the array size is below some threshold.
– OZ17
Nov 10 at 22:56
@OZ17 Great! Seems swap optimization happens for >= 17 doubles on my machine. I'll accept it if you want to post your comment as an answer.
– user2961927
Nov 11 at 0:27
Can you increase the vector size and try it again? Maybe they do silly memcpy if the array size is below some threshold.
– OZ17
Nov 10 at 22:56
Can you increase the vector size and try it again? Maybe they do silly memcpy if the array size is below some threshold.
– OZ17
Nov 10 at 22:56
@OZ17 Great! Seems swap optimization happens for >= 17 doubles on my machine. I'll accept it if you want to post your comment as an answer.
– user2961927
Nov 11 at 0:27
@OZ17 Great! Seems swap optimization happens for >= 17 doubles on my machine. I'll accept it if you want to post your comment as an answer.
– user2961927
Nov 11 at 0:27
add a comment |
2 Answers
2
active
oldest
votes
up vote
0
down vote
accepted
Looks like armadillo's swap is internally a memcpy below a certain array size (according to op <=16).
add a comment |
up vote
2
down vote
A small expansion of @OZ17's answer.
Armadillo seems to store data with sizes< 16 locally mem_local
and larger ones in an area pointed out by mem
From GDB:
> p x
{
<arma::Mat<double>> = {
<arma::Base<double, arma::Mat<double> >> = {
<arma::Base_inv_yes<arma::Mat<double> >> = {<No data fields>},
<arma::Base_eval_Mat<double, arma::Mat<double> >> = {<No data fields>},
<arma::Base_trans_default<arma::Mat<double> >> = {<No data fields>}, <No data fields>},
members of arma::Mat<double>:
n_rows = 1,
n_cols = 10,
n_elem = 10,
vec_state = 2,
mem_state = 0,
mem = 0x7fffffffb830,
mem_local = {0 <repeats 16 times>},
static is_col = false,
static is_row = false
},
members of arma::Row<double>:
static is_col = false,
static is_row = false
}
and a small example to visualize it:
arma::rowvec x(10,arma::fill::ones);
arma::rowvec y(10,arma::fill::zeros);
std::cout << "Size=10" << std::endl;
std::cout << "&x=" << x.memptr() << ", x[0..4]=" << x.subvec(1,5);
std::cout << "&y=" << y.memptr() << ", y[0..4]=" << y.subvec(1,5);
x.swap(y);
std::cout << "x.swap(y)" << std::endl;
std::cout << "&x=" << x.memptr() << ", x[0..4]=" << x.subvec(1,5);
std::cout << "&y=" << y.memptr() << ", y[0..4]=" << y.subvec(1,5);
arma::rowvec x2(17,arma::fill::ones);
arma::rowvec y2(17,arma::fill::zeros);
std::cout << "nSize=17" << std::endl;
std::cout << "&x=" << x2.memptr() << ", x[0..4]=" << x2.subvec(1,5);
std::cout << "&y=" << y2.memptr() << ", y[0..4]=" << y2.subvec(1,5);
x2.swap(y2);
std::cout << "x.swap(y)" << std::endl;
std::cout << "&x=" << x2.memptr() << ", x[0..4]=" << x2.subvec(1,5);
std::cout << "&y=" << y2.memptr() << ", y[0..4]=" << y2.subvec(1,5);
The output from the example shows that the content is swapped in both cases but for small arrays it has swapped the local mem area and for the larger case it has swapped the mem pointer.
Size=10
&x=0x7fffffffb830, x[0..4]= 1.0000 1.0000 1.0000 1.0000 1.0000
&y=0x7fffffffb8e0, y[0..4]= 0 0 0 0 0
x.swap(y)
&x=0x7fffffffb830, x[0..4]= 0 0 0 0 0
&y=0x7fffffffb8e0, y[0..4]= 1.0000 1.0000 1.0000 1.0000 1.0000
Size=17
&x=0x5555557d7fd0, x[0..4]= 1.0000 1.0000 1.0000 1.0000 1.0000
&y=0x5555557d8060, y[0..4]= 0 0 0 0 0
x.swap(y)
&x=0x5555557d8060, x[0..4]= 0 0 0 0 0
&y=0x5555557d7fd0, y[0..4]= 1.0000 1.0000 1.0000 1.0000 1.0000
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
Looks like armadillo's swap is internally a memcpy below a certain array size (according to op <=16).
add a comment |
up vote
0
down vote
accepted
Looks like armadillo's swap is internally a memcpy below a certain array size (according to op <=16).
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
Looks like armadillo's swap is internally a memcpy below a certain array size (according to op <=16).
Looks like armadillo's swap is internally a memcpy below a certain array size (according to op <=16).
answered Nov 11 at 1:36
OZ17
17819
17819
add a comment |
add a comment |
up vote
2
down vote
A small expansion of @OZ17's answer.
Armadillo seems to store data with sizes< 16 locally mem_local
and larger ones in an area pointed out by mem
From GDB:
> p x
{
<arma::Mat<double>> = {
<arma::Base<double, arma::Mat<double> >> = {
<arma::Base_inv_yes<arma::Mat<double> >> = {<No data fields>},
<arma::Base_eval_Mat<double, arma::Mat<double> >> = {<No data fields>},
<arma::Base_trans_default<arma::Mat<double> >> = {<No data fields>}, <No data fields>},
members of arma::Mat<double>:
n_rows = 1,
n_cols = 10,
n_elem = 10,
vec_state = 2,
mem_state = 0,
mem = 0x7fffffffb830,
mem_local = {0 <repeats 16 times>},
static is_col = false,
static is_row = false
},
members of arma::Row<double>:
static is_col = false,
static is_row = false
}
and a small example to visualize it:
arma::rowvec x(10,arma::fill::ones);
arma::rowvec y(10,arma::fill::zeros);
std::cout << "Size=10" << std::endl;
std::cout << "&x=" << x.memptr() << ", x[0..4]=" << x.subvec(1,5);
std::cout << "&y=" << y.memptr() << ", y[0..4]=" << y.subvec(1,5);
x.swap(y);
std::cout << "x.swap(y)" << std::endl;
std::cout << "&x=" << x.memptr() << ", x[0..4]=" << x.subvec(1,5);
std::cout << "&y=" << y.memptr() << ", y[0..4]=" << y.subvec(1,5);
arma::rowvec x2(17,arma::fill::ones);
arma::rowvec y2(17,arma::fill::zeros);
std::cout << "nSize=17" << std::endl;
std::cout << "&x=" << x2.memptr() << ", x[0..4]=" << x2.subvec(1,5);
std::cout << "&y=" << y2.memptr() << ", y[0..4]=" << y2.subvec(1,5);
x2.swap(y2);
std::cout << "x.swap(y)" << std::endl;
std::cout << "&x=" << x2.memptr() << ", x[0..4]=" << x2.subvec(1,5);
std::cout << "&y=" << y2.memptr() << ", y[0..4]=" << y2.subvec(1,5);
The output from the example shows that the content is swapped in both cases but for small arrays it has swapped the local mem area and for the larger case it has swapped the mem pointer.
Size=10
&x=0x7fffffffb830, x[0..4]= 1.0000 1.0000 1.0000 1.0000 1.0000
&y=0x7fffffffb8e0, y[0..4]= 0 0 0 0 0
x.swap(y)
&x=0x7fffffffb830, x[0..4]= 0 0 0 0 0
&y=0x7fffffffb8e0, y[0..4]= 1.0000 1.0000 1.0000 1.0000 1.0000
Size=17
&x=0x5555557d7fd0, x[0..4]= 1.0000 1.0000 1.0000 1.0000 1.0000
&y=0x5555557d8060, y[0..4]= 0 0 0 0 0
x.swap(y)
&x=0x5555557d8060, x[0..4]= 0 0 0 0 0
&y=0x5555557d7fd0, y[0..4]= 1.0000 1.0000 1.0000 1.0000 1.0000
add a comment |
up vote
2
down vote
A small expansion of @OZ17's answer.
Armadillo seems to store data with sizes< 16 locally mem_local
and larger ones in an area pointed out by mem
From GDB:
> p x
{
<arma::Mat<double>> = {
<arma::Base<double, arma::Mat<double> >> = {
<arma::Base_inv_yes<arma::Mat<double> >> = {<No data fields>},
<arma::Base_eval_Mat<double, arma::Mat<double> >> = {<No data fields>},
<arma::Base_trans_default<arma::Mat<double> >> = {<No data fields>}, <No data fields>},
members of arma::Mat<double>:
n_rows = 1,
n_cols = 10,
n_elem = 10,
vec_state = 2,
mem_state = 0,
mem = 0x7fffffffb830,
mem_local = {0 <repeats 16 times>},
static is_col = false,
static is_row = false
},
members of arma::Row<double>:
static is_col = false,
static is_row = false
}
and a small example to visualize it:
arma::rowvec x(10,arma::fill::ones);
arma::rowvec y(10,arma::fill::zeros);
std::cout << "Size=10" << std::endl;
std::cout << "&x=" << x.memptr() << ", x[0..4]=" << x.subvec(1,5);
std::cout << "&y=" << y.memptr() << ", y[0..4]=" << y.subvec(1,5);
x.swap(y);
std::cout << "x.swap(y)" << std::endl;
std::cout << "&x=" << x.memptr() << ", x[0..4]=" << x.subvec(1,5);
std::cout << "&y=" << y.memptr() << ", y[0..4]=" << y.subvec(1,5);
arma::rowvec x2(17,arma::fill::ones);
arma::rowvec y2(17,arma::fill::zeros);
std::cout << "nSize=17" << std::endl;
std::cout << "&x=" << x2.memptr() << ", x[0..4]=" << x2.subvec(1,5);
std::cout << "&y=" << y2.memptr() << ", y[0..4]=" << y2.subvec(1,5);
x2.swap(y2);
std::cout << "x.swap(y)" << std::endl;
std::cout << "&x=" << x2.memptr() << ", x[0..4]=" << x2.subvec(1,5);
std::cout << "&y=" << y2.memptr() << ", y[0..4]=" << y2.subvec(1,5);
The output from the example shows that the content is swapped in both cases but for small arrays it has swapped the local mem area and for the larger case it has swapped the mem pointer.
Size=10
&x=0x7fffffffb830, x[0..4]= 1.0000 1.0000 1.0000 1.0000 1.0000
&y=0x7fffffffb8e0, y[0..4]= 0 0 0 0 0
x.swap(y)
&x=0x7fffffffb830, x[0..4]= 0 0 0 0 0
&y=0x7fffffffb8e0, y[0..4]= 1.0000 1.0000 1.0000 1.0000 1.0000
Size=17
&x=0x5555557d7fd0, x[0..4]= 1.0000 1.0000 1.0000 1.0000 1.0000
&y=0x5555557d8060, y[0..4]= 0 0 0 0 0
x.swap(y)
&x=0x5555557d8060, x[0..4]= 0 0 0 0 0
&y=0x5555557d7fd0, y[0..4]= 1.0000 1.0000 1.0000 1.0000 1.0000
add a comment |
up vote
2
down vote
up vote
2
down vote
A small expansion of @OZ17's answer.
Armadillo seems to store data with sizes< 16 locally mem_local
and larger ones in an area pointed out by mem
From GDB:
> p x
{
<arma::Mat<double>> = {
<arma::Base<double, arma::Mat<double> >> = {
<arma::Base_inv_yes<arma::Mat<double> >> = {<No data fields>},
<arma::Base_eval_Mat<double, arma::Mat<double> >> = {<No data fields>},
<arma::Base_trans_default<arma::Mat<double> >> = {<No data fields>}, <No data fields>},
members of arma::Mat<double>:
n_rows = 1,
n_cols = 10,
n_elem = 10,
vec_state = 2,
mem_state = 0,
mem = 0x7fffffffb830,
mem_local = {0 <repeats 16 times>},
static is_col = false,
static is_row = false
},
members of arma::Row<double>:
static is_col = false,
static is_row = false
}
and a small example to visualize it:
arma::rowvec x(10,arma::fill::ones);
arma::rowvec y(10,arma::fill::zeros);
std::cout << "Size=10" << std::endl;
std::cout << "&x=" << x.memptr() << ", x[0..4]=" << x.subvec(1,5);
std::cout << "&y=" << y.memptr() << ", y[0..4]=" << y.subvec(1,5);
x.swap(y);
std::cout << "x.swap(y)" << std::endl;
std::cout << "&x=" << x.memptr() << ", x[0..4]=" << x.subvec(1,5);
std::cout << "&y=" << y.memptr() << ", y[0..4]=" << y.subvec(1,5);
arma::rowvec x2(17,arma::fill::ones);
arma::rowvec y2(17,arma::fill::zeros);
std::cout << "nSize=17" << std::endl;
std::cout << "&x=" << x2.memptr() << ", x[0..4]=" << x2.subvec(1,5);
std::cout << "&y=" << y2.memptr() << ", y[0..4]=" << y2.subvec(1,5);
x2.swap(y2);
std::cout << "x.swap(y)" << std::endl;
std::cout << "&x=" << x2.memptr() << ", x[0..4]=" << x2.subvec(1,5);
std::cout << "&y=" << y2.memptr() << ", y[0..4]=" << y2.subvec(1,5);
The output from the example shows that the content is swapped in both cases but for small arrays it has swapped the local mem area and for the larger case it has swapped the mem pointer.
Size=10
&x=0x7fffffffb830, x[0..4]= 1.0000 1.0000 1.0000 1.0000 1.0000
&y=0x7fffffffb8e0, y[0..4]= 0 0 0 0 0
x.swap(y)
&x=0x7fffffffb830, x[0..4]= 0 0 0 0 0
&y=0x7fffffffb8e0, y[0..4]= 1.0000 1.0000 1.0000 1.0000 1.0000
Size=17
&x=0x5555557d7fd0, x[0..4]= 1.0000 1.0000 1.0000 1.0000 1.0000
&y=0x5555557d8060, y[0..4]= 0 0 0 0 0
x.swap(y)
&x=0x5555557d8060, x[0..4]= 0 0 0 0 0
&y=0x5555557d7fd0, y[0..4]= 1.0000 1.0000 1.0000 1.0000 1.0000
A small expansion of @OZ17's answer.
Armadillo seems to store data with sizes< 16 locally mem_local
and larger ones in an area pointed out by mem
From GDB:
> p x
{
<arma::Mat<double>> = {
<arma::Base<double, arma::Mat<double> >> = {
<arma::Base_inv_yes<arma::Mat<double> >> = {<No data fields>},
<arma::Base_eval_Mat<double, arma::Mat<double> >> = {<No data fields>},
<arma::Base_trans_default<arma::Mat<double> >> = {<No data fields>}, <No data fields>},
members of arma::Mat<double>:
n_rows = 1,
n_cols = 10,
n_elem = 10,
vec_state = 2,
mem_state = 0,
mem = 0x7fffffffb830,
mem_local = {0 <repeats 16 times>},
static is_col = false,
static is_row = false
},
members of arma::Row<double>:
static is_col = false,
static is_row = false
}
and a small example to visualize it:
arma::rowvec x(10,arma::fill::ones);
arma::rowvec y(10,arma::fill::zeros);
std::cout << "Size=10" << std::endl;
std::cout << "&x=" << x.memptr() << ", x[0..4]=" << x.subvec(1,5);
std::cout << "&y=" << y.memptr() << ", y[0..4]=" << y.subvec(1,5);
x.swap(y);
std::cout << "x.swap(y)" << std::endl;
std::cout << "&x=" << x.memptr() << ", x[0..4]=" << x.subvec(1,5);
std::cout << "&y=" << y.memptr() << ", y[0..4]=" << y.subvec(1,5);
arma::rowvec x2(17,arma::fill::ones);
arma::rowvec y2(17,arma::fill::zeros);
std::cout << "nSize=17" << std::endl;
std::cout << "&x=" << x2.memptr() << ", x[0..4]=" << x2.subvec(1,5);
std::cout << "&y=" << y2.memptr() << ", y[0..4]=" << y2.subvec(1,5);
x2.swap(y2);
std::cout << "x.swap(y)" << std::endl;
std::cout << "&x=" << x2.memptr() << ", x[0..4]=" << x2.subvec(1,5);
std::cout << "&y=" << y2.memptr() << ", y[0..4]=" << y2.subvec(1,5);
The output from the example shows that the content is swapped in both cases but for small arrays it has swapped the local mem area and for the larger case it has swapped the mem pointer.
Size=10
&x=0x7fffffffb830, x[0..4]= 1.0000 1.0000 1.0000 1.0000 1.0000
&y=0x7fffffffb8e0, y[0..4]= 0 0 0 0 0
x.swap(y)
&x=0x7fffffffb830, x[0..4]= 0 0 0 0 0
&y=0x7fffffffb8e0, y[0..4]= 1.0000 1.0000 1.0000 1.0000 1.0000
Size=17
&x=0x5555557d7fd0, x[0..4]= 1.0000 1.0000 1.0000 1.0000 1.0000
&y=0x5555557d8060, y[0..4]= 0 0 0 0 0
x.swap(y)
&x=0x5555557d8060, x[0..4]= 0 0 0 0 0
&y=0x5555557d7fd0, y[0..4]= 1.0000 1.0000 1.0000 1.0000 1.0000
answered Nov 11 at 9:59


Claes Rolen
8661416
8661416
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53243845%2farmadillo-does-not-perform-swap-optimization%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
p4l cN7mjcg6Tk Dcn X2eweQkDOtGgn,BH sN8qnDfmu9HTLc6rb6nluOD,Kb aOm6IM UOZuWhCoH3f,cFhj,2HmQx5
Can you increase the vector size and try it again? Maybe they do silly memcpy if the array size is below some threshold.
– OZ17
Nov 10 at 22:56
@OZ17 Great! Seems swap optimization happens for >= 17 doubles on my machine. I'll accept it if you want to post your comment as an answer.
– user2961927
Nov 11 at 0:27