How can I unit test Android View animation endAction?
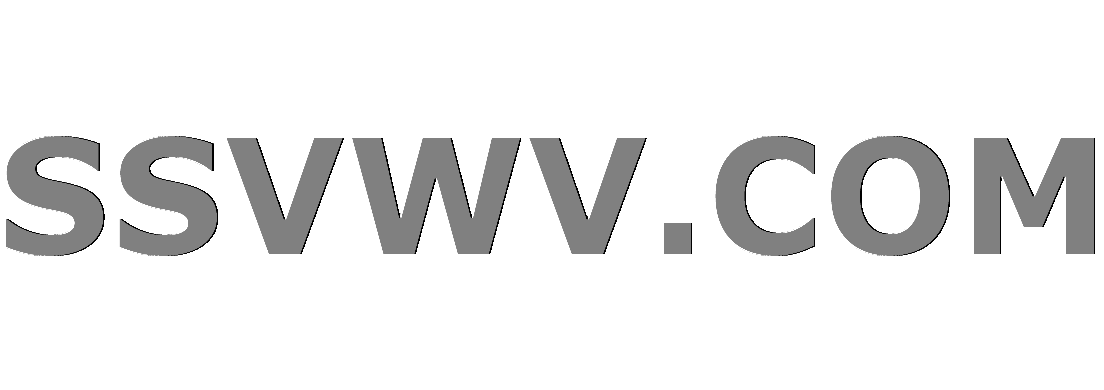
Multi tool use
up vote
1
down vote
favorite
fun TextView.setTextWithFade(text: String, duration: Long = 300) {
this.animate().alpha(0f).setDuration(duration).withEndAction {
this.text = text
this.animate().alpha(1f).setDuration(duration).start()
}.start()
}
Hello,
I have a simple Kotlin extension function for a TextView to change text with animation. There is three steps in this animation:
- Fades out old text
- Change the text in a TextView
- Fades the text back in
What is the best way to write Unit Test that checks, whether the text was changed after the fade out animation?
@Test
fun setTextWithFadeTest() {
val context = ApplicationProvider.getApplicationContext<Context>()
val textView = TextView(context)
textView.text = "test1"
textView.setTextWithFade("test2", duration = 10)
val handler = Handler()
handler.postDelayed({
println("Text: ${textView.text}, alpha: ${textView.alpha}")
assert(textView.text == "test2")
}, 300)
Robolectric.getForegroundThreadScheduler().advanceBy(300, TimeUnit.MILLISECONDS)
}
This is a code how I try to achieve it. That handler prints:
Text: test1, alpha: 1.0
So it seems that animation don't even started.

add a comment |
up vote
1
down vote
favorite
fun TextView.setTextWithFade(text: String, duration: Long = 300) {
this.animate().alpha(0f).setDuration(duration).withEndAction {
this.text = text
this.animate().alpha(1f).setDuration(duration).start()
}.start()
}
Hello,
I have a simple Kotlin extension function for a TextView to change text with animation. There is three steps in this animation:
- Fades out old text
- Change the text in a TextView
- Fades the text back in
What is the best way to write Unit Test that checks, whether the text was changed after the fade out animation?
@Test
fun setTextWithFadeTest() {
val context = ApplicationProvider.getApplicationContext<Context>()
val textView = TextView(context)
textView.text = "test1"
textView.setTextWithFade("test2", duration = 10)
val handler = Handler()
handler.postDelayed({
println("Text: ${textView.text}, alpha: ${textView.alpha}")
assert(textView.text == "test2")
}, 300)
Robolectric.getForegroundThreadScheduler().advanceBy(300, TimeUnit.MILLISECONDS)
}
This is a code how I try to achieve it. That handler prints:
Text: test1, alpha: 1.0
So it seems that animation don't even started.

There was (is) known bug that Robolectric doesn't run animations well: github.com/robolectric/robolectric/issues/2845
– Eugen Martynov
Nov 11 at 16:44
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
fun TextView.setTextWithFade(text: String, duration: Long = 300) {
this.animate().alpha(0f).setDuration(duration).withEndAction {
this.text = text
this.animate().alpha(1f).setDuration(duration).start()
}.start()
}
Hello,
I have a simple Kotlin extension function for a TextView to change text with animation. There is three steps in this animation:
- Fades out old text
- Change the text in a TextView
- Fades the text back in
What is the best way to write Unit Test that checks, whether the text was changed after the fade out animation?
@Test
fun setTextWithFadeTest() {
val context = ApplicationProvider.getApplicationContext<Context>()
val textView = TextView(context)
textView.text = "test1"
textView.setTextWithFade("test2", duration = 10)
val handler = Handler()
handler.postDelayed({
println("Text: ${textView.text}, alpha: ${textView.alpha}")
assert(textView.text == "test2")
}, 300)
Robolectric.getForegroundThreadScheduler().advanceBy(300, TimeUnit.MILLISECONDS)
}
This is a code how I try to achieve it. That handler prints:
Text: test1, alpha: 1.0
So it seems that animation don't even started.

fun TextView.setTextWithFade(text: String, duration: Long = 300) {
this.animate().alpha(0f).setDuration(duration).withEndAction {
this.text = text
this.animate().alpha(1f).setDuration(duration).start()
}.start()
}
Hello,
I have a simple Kotlin extension function for a TextView to change text with animation. There is three steps in this animation:
- Fades out old text
- Change the text in a TextView
- Fades the text back in
What is the best way to write Unit Test that checks, whether the text was changed after the fade out animation?
@Test
fun setTextWithFadeTest() {
val context = ApplicationProvider.getApplicationContext<Context>()
val textView = TextView(context)
textView.text = "test1"
textView.setTextWithFade("test2", duration = 10)
val handler = Handler()
handler.postDelayed({
println("Text: ${textView.text}, alpha: ${textView.alpha}")
assert(textView.text == "test2")
}, 300)
Robolectric.getForegroundThreadScheduler().advanceBy(300, TimeUnit.MILLISECONDS)
}
This is a code how I try to achieve it. That handler prints:
Text: test1, alpha: 1.0
So it seems that animation don't even started.


asked Nov 11 at 14:39
Arnas Smičius
61
61
There was (is) known bug that Robolectric doesn't run animations well: github.com/robolectric/robolectric/issues/2845
– Eugen Martynov
Nov 11 at 16:44
add a comment |
There was (is) known bug that Robolectric doesn't run animations well: github.com/robolectric/robolectric/issues/2845
– Eugen Martynov
Nov 11 at 16:44
There was (is) known bug that Robolectric doesn't run animations well: github.com/robolectric/robolectric/issues/2845
– Eugen Martynov
Nov 11 at 16:44
There was (is) known bug that Robolectric doesn't run animations well: github.com/robolectric/robolectric/issues/2845
– Eugen Martynov
Nov 11 at 16:44
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53249783%2fhow-can-i-unit-test-android-view-animation-endaction%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
CAKfEq 4JLQP,l12vGqan3PkS,EtB9r9rF9OkMerYMxtMlA,skRtMxl66He9Z5kpL
There was (is) known bug that Robolectric doesn't run animations well: github.com/robolectric/robolectric/issues/2845
– Eugen Martynov
Nov 11 at 16:44