Create method for foreign key relationships with Django Rest Framework serializers
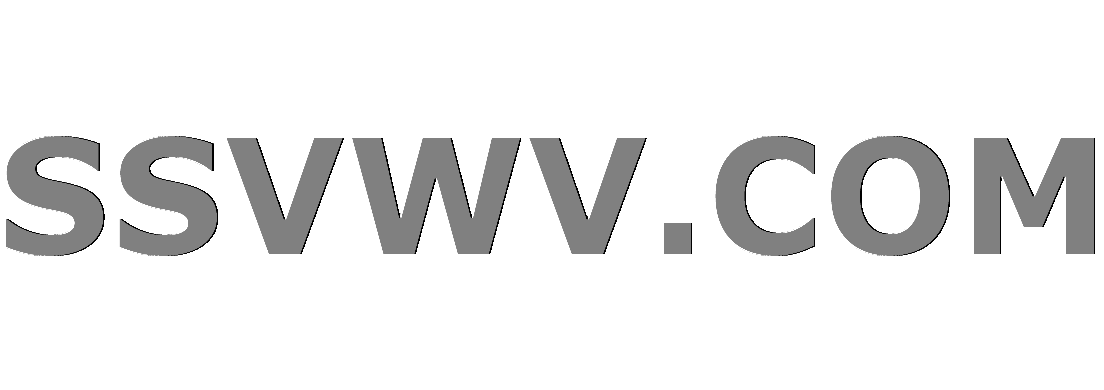
Multi tool use
up vote
0
down vote
favorite
My models are like this:
class FirewallPolicy(models.Model):
name = models.CharField(max_length=100, unique=True)
team = models.ForeignKey(Team)
source_ip = models.ForeignKey(IP)
destination_ip = models.ForeignKey(IP)
Now, in order to create a new Firewall Policy, there should already be an existing team, source_ip and destination_ip. My payload to create a new Firewall Policy is as follows:
{"name": "test-create-policy-911",
"team": "avengers",
"source_ip": "1.1.1.1",
"destination_ip": "2.2.2.2",
}
My serializer to create a new Firewall Policy is as follows:
class FirewallPolicyCreateSerializer(serializers.ModelSerializer):
name = serializers.CharField(max_length=100)
team = serializers.CharField(max_length=100)
source_ip = serializers.CharField(max_length=100)
destination_ip = serializers.CharField(max_length=100)
class Meta:
model = Policy
fields = ['id', 'name', 'team', 'source_ip', 'destination_ip']
def validate(self, data):
try:
Team.objects.get(name=data['team'])
IP.objects.get(name=data['source_ip'])
IP.objects.get(name=data['destination_ip'])
except ObjectDoesNotExist:
raise serializers.ValidationError("Entities must exist before you can associate it with a Firewall Policy")
def create(self, validated_data):
team = Team.objects.get(name=validated_data['team'])
source_ip = IP.objects.get(name=validated_data['source_ip'])
destination_ip = IP.objects.get(name=validated_data['destination_ip'])
policy = Policy.objects.create(name=validated_data['name'],
team_id=team.id,
source_ip_id = source_ip.id,
destination_ip_id = destination_ip.id )
return policy
I am not sure if this is the right way of adding foreign keys to a model as it seems too much work. Is there something I am missing where the serializer can automatically check all this and add the foreign keys ?
python django django-rest-framework serializer
add a comment |
up vote
0
down vote
favorite
My models are like this:
class FirewallPolicy(models.Model):
name = models.CharField(max_length=100, unique=True)
team = models.ForeignKey(Team)
source_ip = models.ForeignKey(IP)
destination_ip = models.ForeignKey(IP)
Now, in order to create a new Firewall Policy, there should already be an existing team, source_ip and destination_ip. My payload to create a new Firewall Policy is as follows:
{"name": "test-create-policy-911",
"team": "avengers",
"source_ip": "1.1.1.1",
"destination_ip": "2.2.2.2",
}
My serializer to create a new Firewall Policy is as follows:
class FirewallPolicyCreateSerializer(serializers.ModelSerializer):
name = serializers.CharField(max_length=100)
team = serializers.CharField(max_length=100)
source_ip = serializers.CharField(max_length=100)
destination_ip = serializers.CharField(max_length=100)
class Meta:
model = Policy
fields = ['id', 'name', 'team', 'source_ip', 'destination_ip']
def validate(self, data):
try:
Team.objects.get(name=data['team'])
IP.objects.get(name=data['source_ip'])
IP.objects.get(name=data['destination_ip'])
except ObjectDoesNotExist:
raise serializers.ValidationError("Entities must exist before you can associate it with a Firewall Policy")
def create(self, validated_data):
team = Team.objects.get(name=validated_data['team'])
source_ip = IP.objects.get(name=validated_data['source_ip'])
destination_ip = IP.objects.get(name=validated_data['destination_ip'])
policy = Policy.objects.create(name=validated_data['name'],
team_id=team.id,
source_ip_id = source_ip.id,
destination_ip_id = destination_ip.id )
return policy
I am not sure if this is the right way of adding foreign keys to a model as it seems too much work. Is there something I am missing where the serializer can automatically check all this and add the foreign keys ?
python django django-rest-framework serializer
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
My models are like this:
class FirewallPolicy(models.Model):
name = models.CharField(max_length=100, unique=True)
team = models.ForeignKey(Team)
source_ip = models.ForeignKey(IP)
destination_ip = models.ForeignKey(IP)
Now, in order to create a new Firewall Policy, there should already be an existing team, source_ip and destination_ip. My payload to create a new Firewall Policy is as follows:
{"name": "test-create-policy-911",
"team": "avengers",
"source_ip": "1.1.1.1",
"destination_ip": "2.2.2.2",
}
My serializer to create a new Firewall Policy is as follows:
class FirewallPolicyCreateSerializer(serializers.ModelSerializer):
name = serializers.CharField(max_length=100)
team = serializers.CharField(max_length=100)
source_ip = serializers.CharField(max_length=100)
destination_ip = serializers.CharField(max_length=100)
class Meta:
model = Policy
fields = ['id', 'name', 'team', 'source_ip', 'destination_ip']
def validate(self, data):
try:
Team.objects.get(name=data['team'])
IP.objects.get(name=data['source_ip'])
IP.objects.get(name=data['destination_ip'])
except ObjectDoesNotExist:
raise serializers.ValidationError("Entities must exist before you can associate it with a Firewall Policy")
def create(self, validated_data):
team = Team.objects.get(name=validated_data['team'])
source_ip = IP.objects.get(name=validated_data['source_ip'])
destination_ip = IP.objects.get(name=validated_data['destination_ip'])
policy = Policy.objects.create(name=validated_data['name'],
team_id=team.id,
source_ip_id = source_ip.id,
destination_ip_id = destination_ip.id )
return policy
I am not sure if this is the right way of adding foreign keys to a model as it seems too much work. Is there something I am missing where the serializer can automatically check all this and add the foreign keys ?
python django django-rest-framework serializer
My models are like this:
class FirewallPolicy(models.Model):
name = models.CharField(max_length=100, unique=True)
team = models.ForeignKey(Team)
source_ip = models.ForeignKey(IP)
destination_ip = models.ForeignKey(IP)
Now, in order to create a new Firewall Policy, there should already be an existing team, source_ip and destination_ip. My payload to create a new Firewall Policy is as follows:
{"name": "test-create-policy-911",
"team": "avengers",
"source_ip": "1.1.1.1",
"destination_ip": "2.2.2.2",
}
My serializer to create a new Firewall Policy is as follows:
class FirewallPolicyCreateSerializer(serializers.ModelSerializer):
name = serializers.CharField(max_length=100)
team = serializers.CharField(max_length=100)
source_ip = serializers.CharField(max_length=100)
destination_ip = serializers.CharField(max_length=100)
class Meta:
model = Policy
fields = ['id', 'name', 'team', 'source_ip', 'destination_ip']
def validate(self, data):
try:
Team.objects.get(name=data['team'])
IP.objects.get(name=data['source_ip'])
IP.objects.get(name=data['destination_ip'])
except ObjectDoesNotExist:
raise serializers.ValidationError("Entities must exist before you can associate it with a Firewall Policy")
def create(self, validated_data):
team = Team.objects.get(name=validated_data['team'])
source_ip = IP.objects.get(name=validated_data['source_ip'])
destination_ip = IP.objects.get(name=validated_data['destination_ip'])
policy = Policy.objects.create(name=validated_data['name'],
team_id=team.id,
source_ip_id = source_ip.id,
destination_ip_id = destination_ip.id )
return policy
I am not sure if this is the right way of adding foreign keys to a model as it seems too much work. Is there something I am missing where the serializer can automatically check all this and add the foreign keys ?
python django django-rest-framework serializer
python django django-rest-framework serializer
asked Nov 11 at 18:16
Amistad
2,51862438
2,51862438
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
2
down vote
accepted
Yes, you are doing much more work than you need to.
You should define your fields using SlugRelatedField to allow DRF to automatically populate them from a field on the related model. So:
class FirewallPolicyCreateSerializer(serializers.ModelSerializer):
team = serializers.SlugRelatedField(queryset=Team.objects.all(), slug_field='name')
source_ip = serializers.SlugRelatedField(queryset=IP.objects.all(), slug_field='source_ip')
destination_ip = serializers.SlugRelatedField(queryset=IP.objects.all(), slug_field='destination_ip')
class Meta:
model = Policy
fields = ['id', 'name', 'team', 'source_ip', 'destination_ip']
Now you shouldn't need to define validate
or create
at all, as DRF will do all the relevant validation and assignment.
(Note, you didn't need to redefine the name
field either, as you're not changing anything from the underlying model field.)
This is perfect !! so many lines of code less !!
– Amistad
Nov 11 at 18:43
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53251757%2fcreate-method-for-foreign-key-relationships-with-django-rest-framework-serialize%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
Yes, you are doing much more work than you need to.
You should define your fields using SlugRelatedField to allow DRF to automatically populate them from a field on the related model. So:
class FirewallPolicyCreateSerializer(serializers.ModelSerializer):
team = serializers.SlugRelatedField(queryset=Team.objects.all(), slug_field='name')
source_ip = serializers.SlugRelatedField(queryset=IP.objects.all(), slug_field='source_ip')
destination_ip = serializers.SlugRelatedField(queryset=IP.objects.all(), slug_field='destination_ip')
class Meta:
model = Policy
fields = ['id', 'name', 'team', 'source_ip', 'destination_ip']
Now you shouldn't need to define validate
or create
at all, as DRF will do all the relevant validation and assignment.
(Note, you didn't need to redefine the name
field either, as you're not changing anything from the underlying model field.)
This is perfect !! so many lines of code less !!
– Amistad
Nov 11 at 18:43
add a comment |
up vote
2
down vote
accepted
Yes, you are doing much more work than you need to.
You should define your fields using SlugRelatedField to allow DRF to automatically populate them from a field on the related model. So:
class FirewallPolicyCreateSerializer(serializers.ModelSerializer):
team = serializers.SlugRelatedField(queryset=Team.objects.all(), slug_field='name')
source_ip = serializers.SlugRelatedField(queryset=IP.objects.all(), slug_field='source_ip')
destination_ip = serializers.SlugRelatedField(queryset=IP.objects.all(), slug_field='destination_ip')
class Meta:
model = Policy
fields = ['id', 'name', 'team', 'source_ip', 'destination_ip']
Now you shouldn't need to define validate
or create
at all, as DRF will do all the relevant validation and assignment.
(Note, you didn't need to redefine the name
field either, as you're not changing anything from the underlying model field.)
This is perfect !! so many lines of code less !!
– Amistad
Nov 11 at 18:43
add a comment |
up vote
2
down vote
accepted
up vote
2
down vote
accepted
Yes, you are doing much more work than you need to.
You should define your fields using SlugRelatedField to allow DRF to automatically populate them from a field on the related model. So:
class FirewallPolicyCreateSerializer(serializers.ModelSerializer):
team = serializers.SlugRelatedField(queryset=Team.objects.all(), slug_field='name')
source_ip = serializers.SlugRelatedField(queryset=IP.objects.all(), slug_field='source_ip')
destination_ip = serializers.SlugRelatedField(queryset=IP.objects.all(), slug_field='destination_ip')
class Meta:
model = Policy
fields = ['id', 'name', 'team', 'source_ip', 'destination_ip']
Now you shouldn't need to define validate
or create
at all, as DRF will do all the relevant validation and assignment.
(Note, you didn't need to redefine the name
field either, as you're not changing anything from the underlying model field.)
Yes, you are doing much more work than you need to.
You should define your fields using SlugRelatedField to allow DRF to automatically populate them from a field on the related model. So:
class FirewallPolicyCreateSerializer(serializers.ModelSerializer):
team = serializers.SlugRelatedField(queryset=Team.objects.all(), slug_field='name')
source_ip = serializers.SlugRelatedField(queryset=IP.objects.all(), slug_field='source_ip')
destination_ip = serializers.SlugRelatedField(queryset=IP.objects.all(), slug_field='destination_ip')
class Meta:
model = Policy
fields = ['id', 'name', 'team', 'source_ip', 'destination_ip']
Now you shouldn't need to define validate
or create
at all, as DRF will do all the relevant validation and assignment.
(Note, you didn't need to redefine the name
field either, as you're not changing anything from the underlying model field.)
edited Nov 11 at 18:35
answered Nov 11 at 18:27
Daniel Roseman
442k41573628
442k41573628
This is perfect !! so many lines of code less !!
– Amistad
Nov 11 at 18:43
add a comment |
This is perfect !! so many lines of code less !!
– Amistad
Nov 11 at 18:43
This is perfect !! so many lines of code less !!
– Amistad
Nov 11 at 18:43
This is perfect !! so many lines of code less !!
– Amistad
Nov 11 at 18:43
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53251757%2fcreate-method-for-foreign-key-relationships-with-django-rest-framework-serialize%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
KObE8gO3YSi7Ob 5 32gJwN U NfqSaU u4Q4wwW3boWbgjk 89LebXQb HnZbeVaPmHIIZhcnUZkeqcMjYfMy23s6GWE909n25dlkI3X0