Unable to access the values a user inputs into text boxes
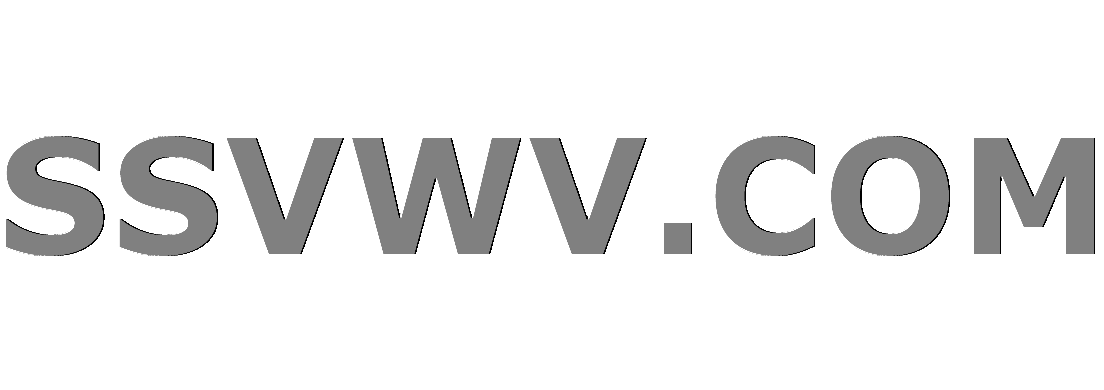
Multi tool use
up vote
1
down vote
favorite
I am trying to collect several values inputted into NumericEditField
controls by a user and store them in order to use them later in a computation, whose result gets presented back.
I seem to be able to store the variables, but am unable to access them later. Each of the variables is considered private because that is where it is having me add the callbacks. I have tried adding all of the variables in a public portion near the beginning, but that did not work.
Here is the code:
classdef Finalscalc < matlab.apps.AppBase
% Properties that correspond to app components
properties (Access = public)
UIFigure matlab.ui.Figure
PleaseenteryourcurrentgradeEditFieldLabel matlab.ui.control.Label
PleaseenteryourcurrentgradeEditField matlab.ui.control.NumericEditField
PleaseenterthegradeyouwantEditFieldLabel matlab.ui.control.Label
PleaseenterthegradeyouwantEditField matlab.ui.control.NumericEditField
PleaseentertheweightofthefinalexamEditFieldLabel matlab.ui.control.Label
PleaseentertheweightofthefinalexamEditField matlab.ui.control.NumericEditField
Label matlab.ui.control.Label
Label_2 matlab.ui.control.Label
Label_3 matlab.ui.control.Label
YouneedatleastaEditFieldLabel matlab.ui.control.Label
YouneedatleastaEditField matlab.ui.control.NumericEditField
Label_4 matlab.ui.control.Label
togetaEditFieldLabel matlab.ui.control.Label
togetaEditField matlab.ui.control.NumericEditField
Label_5 matlab.ui.control.Label
CalcualteButton matlab.ui.control.Button
GoodLuckLabel matlab.ui.control.Label
end
methods (Access = private)
% Code that executes after component creation
function startupFcn(app)
end
% Value changed function: PleaseenteryourcurrentgradeEditField
function PleaseenteryourcurrentgradeEditFieldValueChanged(app, event)
app.current = app.PleaseenteryourcurrentgradeEditField.Value;
current = app.current;
app.current = current;
end
% Value changed function: PleaseenterthegradeyouwantEditField
function PleaseenterthegradeyouwantEditFieldValueChanged(app, event)
app.want = app.PleaseenterthegradeyouwantEditField.Value;
want = app.want;
app.want = want;
end
% Value changed function:
% PleaseentertheweightofthefinalexamEditField
function PleaseentertheweightofthefinalexamEditFieldValueChanged(app, event)
app.weight = app.PleaseentertheweightofthefinalexamEditField.Value;
weight = app.weight;
app.weight = weight;
end
% Button pushed function: CalcualteButton
function CalcualteButtonPushed(app, event)
grade = ((100 * app.want)-(100 - app.weight) * app.current) / app.weight;
grade = app.grade;
app.grade = grade;
end
% Value changed function: YouneedatleastaEditField
function YouneedatleastaEditFieldValueChanged(app, event)
grade = app.YouneedatleastaEditField.Value;
fprintf('%,2f', grade);
end
% Value changed function: togetaEditField
function togetaEditFieldValueChanged(app, event)
app.PleaseenterthegradeyouwantEditField.Value = app.togetaEditField.Value;
end
end
% App initialization and construction
methods (Access = private)
% Create UIFigure and components
function createComponents(app)
% Create UIFigure
app.UIFigure = uifigure;
app.UIFigure.Position = [100 100 640 480];
app.UIFigure.Name = 'UI Figure';
% Create PleaseenteryourcurrentgradeEditFieldLabel
app.PleaseenteryourcurrentgradeEditFieldLabel = uilabel(app.UIFigure);
app.PleaseenteryourcurrentgradeEditFieldLabel.HorizontalAlignment = 'right';
app.PleaseenteryourcurrentgradeEditFieldLabel.Position = [117 337 174 22];
app.PleaseenteryourcurrentgradeEditFieldLabel.Text = 'Please enter your current grade';
% Create PleaseenteryourcurrentgradeEditField
app.PleaseenteryourcurrentgradeEditField = uieditfield(app.UIFigure, 'numeric');
app.PleaseenteryourcurrentgradeEditField.ValueChangedFcn = createCallbackFcn(app, @PleaseenteryourcurrentgradeEditFieldValueChanged, true);
app.PleaseenteryourcurrentgradeEditField.Position = [306 337 100 22];
% Create PleaseenterthegradeyouwantEditFieldLabel
app.PleaseenterthegradeyouwantEditFieldLabel = uilabel(app.UIFigure);
app.PleaseenterthegradeyouwantEditFieldLabel.HorizontalAlignment = 'right';
app.PleaseenterthegradeyouwantEditFieldLabel.Position = [116 289 178 22];
app.PleaseenterthegradeyouwantEditFieldLabel.Text = 'Please enter the grade you want';
% Create PleaseenterthegradeyouwantEditField
app.PleaseenterthegradeyouwantEditField = uieditfield(app.UIFigure, 'numeric');
app.PleaseenterthegradeyouwantEditField.ValueChangedFcn = createCallbackFcn(app, @PleaseenterthegradeyouwantEditFieldValueChanged, true);
app.PleaseenterthegradeyouwantEditField.Position = [309 289 100 22];
% Create PleaseentertheweightofthefinalexamEditFieldLabel
app.PleaseentertheweightofthefinalexamEditFieldLabel = uilabel(app.UIFigure);
app.PleaseentertheweightofthefinalexamEditFieldLabel.HorizontalAlignment = 'right';
app.PleaseentertheweightofthefinalexamEditFieldLabel.Position = [70 248 224 22];
app.PleaseentertheweightofthefinalexamEditFieldLabel.Text = 'Please enter the weight of the final exam';
% Create PleaseentertheweightofthefinalexamEditField
app.PleaseentertheweightofthefinalexamEditField = uieditfield(app.UIFigure, 'numeric');
app.PleaseentertheweightofthefinalexamEditField.ValueChangedFcn = createCallbackFcn(app, @PleaseentertheweightofthefinalexamEditFieldValueChanged, true);
app.PleaseentertheweightofthefinalexamEditField.Position = [309 248 100 22];
% Create Label
app.Label = uilabel(app.UIFigure);
app.Label.Position = [417 337 25 22];
app.Label.Text = '%';
% Create Label_2
app.Label_2 = uilabel(app.UIFigure);
app.Label_2.Position = [417 289 25 22];
app.Label_2.Text = '%';
% Create Label_3
app.Label_3 = uilabel(app.UIFigure);
app.Label_3.Position = [417 248 25 22];
app.Label_3.Text = '%';
% Create YouneedatleastaEditFieldLabel
app.YouneedatleastaEditFieldLabel = uilabel(app.UIFigure);
app.YouneedatleastaEditFieldLabel.HorizontalAlignment = 'right';
app.YouneedatleastaEditFieldLabel.Position = [177 105 107 22];
app.YouneedatleastaEditFieldLabel.Text = 'You need at least a';
% Create YouneedatleastaEditField
app.YouneedatleastaEditField = uieditfield(app.UIFigure, 'numeric');
app.YouneedatleastaEditField.ValueChangedFcn = createCallbackFcn(app, @YouneedatleastaEditFieldValueChanged, true);
app.YouneedatleastaEditField.Position = [291 105 19 22];
% Create Label_4
app.Label_4 = uilabel(app.UIFigure);
app.Label_4.Position = [323 105 25 22];
app.Label_4.Text = '%';
% Create togetaEditFieldLabel
app.togetaEditFieldLabel = uilabel(app.UIFigure);
app.togetaEditFieldLabel.HorizontalAlignment = 'right';
app.togetaEditFieldLabel.Position = [337 105 46 22];
app.togetaEditFieldLabel.Text = 'to get a';
% Create togetaEditField
app.togetaEditField = uieditfield(app.UIFigure, 'numeric');
app.togetaEditField.ValueChangedFcn = createCallbackFcn(app, @togetaEditFieldValueChanged, true);
app.togetaEditField.Position = [390 105 16 22];
% Create Label_5
app.Label_5 = uilabel(app.UIFigure);
app.Label_5.Position = [417 105 25 22];
app.Label_5.Text = '%';
% Create CalcualteButton
app.CalcualteButton = uibutton(app.UIFigure, 'push');
app.CalcualteButton.ButtonPushedFcn = createCallbackFcn(app, @CalcualteButtonPushed, true);
app.CalcualteButton.Position = [271 168 100 22];
app.CalcualteButton.Text = 'Calcualte';
% Create GoodLuckLabel
app.GoodLuckLabel = uilabel(app.UIFigure);
app.GoodLuckLabel.Position = [287 30 70 22];
app.GoodLuckLabel.Text = 'Good Luck !';
end
end
methods (Access = public)
% Construct app
function app = Finalscalc
% Create and configure components
createComponents(app)
% Register the app with App Designer
registerApp(app, app.UIFigure)
% Execute the startup function
runStartupFcn(app, @startupFcn)
if nargout == 0
clear app
end
end
% Code that executes before app deletion
function delete(app)
% Delete UIFigure when app is deleted
delete(app.UIFigure)
end
end
end
matlab user-interface private-members matlab-gui matlab-app-designer
add a comment |
up vote
1
down vote
favorite
I am trying to collect several values inputted into NumericEditField
controls by a user and store them in order to use them later in a computation, whose result gets presented back.
I seem to be able to store the variables, but am unable to access them later. Each of the variables is considered private because that is where it is having me add the callbacks. I have tried adding all of the variables in a public portion near the beginning, but that did not work.
Here is the code:
classdef Finalscalc < matlab.apps.AppBase
% Properties that correspond to app components
properties (Access = public)
UIFigure matlab.ui.Figure
PleaseenteryourcurrentgradeEditFieldLabel matlab.ui.control.Label
PleaseenteryourcurrentgradeEditField matlab.ui.control.NumericEditField
PleaseenterthegradeyouwantEditFieldLabel matlab.ui.control.Label
PleaseenterthegradeyouwantEditField matlab.ui.control.NumericEditField
PleaseentertheweightofthefinalexamEditFieldLabel matlab.ui.control.Label
PleaseentertheweightofthefinalexamEditField matlab.ui.control.NumericEditField
Label matlab.ui.control.Label
Label_2 matlab.ui.control.Label
Label_3 matlab.ui.control.Label
YouneedatleastaEditFieldLabel matlab.ui.control.Label
YouneedatleastaEditField matlab.ui.control.NumericEditField
Label_4 matlab.ui.control.Label
togetaEditFieldLabel matlab.ui.control.Label
togetaEditField matlab.ui.control.NumericEditField
Label_5 matlab.ui.control.Label
CalcualteButton matlab.ui.control.Button
GoodLuckLabel matlab.ui.control.Label
end
methods (Access = private)
% Code that executes after component creation
function startupFcn(app)
end
% Value changed function: PleaseenteryourcurrentgradeEditField
function PleaseenteryourcurrentgradeEditFieldValueChanged(app, event)
app.current = app.PleaseenteryourcurrentgradeEditField.Value;
current = app.current;
app.current = current;
end
% Value changed function: PleaseenterthegradeyouwantEditField
function PleaseenterthegradeyouwantEditFieldValueChanged(app, event)
app.want = app.PleaseenterthegradeyouwantEditField.Value;
want = app.want;
app.want = want;
end
% Value changed function:
% PleaseentertheweightofthefinalexamEditField
function PleaseentertheweightofthefinalexamEditFieldValueChanged(app, event)
app.weight = app.PleaseentertheweightofthefinalexamEditField.Value;
weight = app.weight;
app.weight = weight;
end
% Button pushed function: CalcualteButton
function CalcualteButtonPushed(app, event)
grade = ((100 * app.want)-(100 - app.weight) * app.current) / app.weight;
grade = app.grade;
app.grade = grade;
end
% Value changed function: YouneedatleastaEditField
function YouneedatleastaEditFieldValueChanged(app, event)
grade = app.YouneedatleastaEditField.Value;
fprintf('%,2f', grade);
end
% Value changed function: togetaEditField
function togetaEditFieldValueChanged(app, event)
app.PleaseenterthegradeyouwantEditField.Value = app.togetaEditField.Value;
end
end
% App initialization and construction
methods (Access = private)
% Create UIFigure and components
function createComponents(app)
% Create UIFigure
app.UIFigure = uifigure;
app.UIFigure.Position = [100 100 640 480];
app.UIFigure.Name = 'UI Figure';
% Create PleaseenteryourcurrentgradeEditFieldLabel
app.PleaseenteryourcurrentgradeEditFieldLabel = uilabel(app.UIFigure);
app.PleaseenteryourcurrentgradeEditFieldLabel.HorizontalAlignment = 'right';
app.PleaseenteryourcurrentgradeEditFieldLabel.Position = [117 337 174 22];
app.PleaseenteryourcurrentgradeEditFieldLabel.Text = 'Please enter your current grade';
% Create PleaseenteryourcurrentgradeEditField
app.PleaseenteryourcurrentgradeEditField = uieditfield(app.UIFigure, 'numeric');
app.PleaseenteryourcurrentgradeEditField.ValueChangedFcn = createCallbackFcn(app, @PleaseenteryourcurrentgradeEditFieldValueChanged, true);
app.PleaseenteryourcurrentgradeEditField.Position = [306 337 100 22];
% Create PleaseenterthegradeyouwantEditFieldLabel
app.PleaseenterthegradeyouwantEditFieldLabel = uilabel(app.UIFigure);
app.PleaseenterthegradeyouwantEditFieldLabel.HorizontalAlignment = 'right';
app.PleaseenterthegradeyouwantEditFieldLabel.Position = [116 289 178 22];
app.PleaseenterthegradeyouwantEditFieldLabel.Text = 'Please enter the grade you want';
% Create PleaseenterthegradeyouwantEditField
app.PleaseenterthegradeyouwantEditField = uieditfield(app.UIFigure, 'numeric');
app.PleaseenterthegradeyouwantEditField.ValueChangedFcn = createCallbackFcn(app, @PleaseenterthegradeyouwantEditFieldValueChanged, true);
app.PleaseenterthegradeyouwantEditField.Position = [309 289 100 22];
% Create PleaseentertheweightofthefinalexamEditFieldLabel
app.PleaseentertheweightofthefinalexamEditFieldLabel = uilabel(app.UIFigure);
app.PleaseentertheweightofthefinalexamEditFieldLabel.HorizontalAlignment = 'right';
app.PleaseentertheweightofthefinalexamEditFieldLabel.Position = [70 248 224 22];
app.PleaseentertheweightofthefinalexamEditFieldLabel.Text = 'Please enter the weight of the final exam';
% Create PleaseentertheweightofthefinalexamEditField
app.PleaseentertheweightofthefinalexamEditField = uieditfield(app.UIFigure, 'numeric');
app.PleaseentertheweightofthefinalexamEditField.ValueChangedFcn = createCallbackFcn(app, @PleaseentertheweightofthefinalexamEditFieldValueChanged, true);
app.PleaseentertheweightofthefinalexamEditField.Position = [309 248 100 22];
% Create Label
app.Label = uilabel(app.UIFigure);
app.Label.Position = [417 337 25 22];
app.Label.Text = '%';
% Create Label_2
app.Label_2 = uilabel(app.UIFigure);
app.Label_2.Position = [417 289 25 22];
app.Label_2.Text = '%';
% Create Label_3
app.Label_3 = uilabel(app.UIFigure);
app.Label_3.Position = [417 248 25 22];
app.Label_3.Text = '%';
% Create YouneedatleastaEditFieldLabel
app.YouneedatleastaEditFieldLabel = uilabel(app.UIFigure);
app.YouneedatleastaEditFieldLabel.HorizontalAlignment = 'right';
app.YouneedatleastaEditFieldLabel.Position = [177 105 107 22];
app.YouneedatleastaEditFieldLabel.Text = 'You need at least a';
% Create YouneedatleastaEditField
app.YouneedatleastaEditField = uieditfield(app.UIFigure, 'numeric');
app.YouneedatleastaEditField.ValueChangedFcn = createCallbackFcn(app, @YouneedatleastaEditFieldValueChanged, true);
app.YouneedatleastaEditField.Position = [291 105 19 22];
% Create Label_4
app.Label_4 = uilabel(app.UIFigure);
app.Label_4.Position = [323 105 25 22];
app.Label_4.Text = '%';
% Create togetaEditFieldLabel
app.togetaEditFieldLabel = uilabel(app.UIFigure);
app.togetaEditFieldLabel.HorizontalAlignment = 'right';
app.togetaEditFieldLabel.Position = [337 105 46 22];
app.togetaEditFieldLabel.Text = 'to get a';
% Create togetaEditField
app.togetaEditField = uieditfield(app.UIFigure, 'numeric');
app.togetaEditField.ValueChangedFcn = createCallbackFcn(app, @togetaEditFieldValueChanged, true);
app.togetaEditField.Position = [390 105 16 22];
% Create Label_5
app.Label_5 = uilabel(app.UIFigure);
app.Label_5.Position = [417 105 25 22];
app.Label_5.Text = '%';
% Create CalcualteButton
app.CalcualteButton = uibutton(app.UIFigure, 'push');
app.CalcualteButton.ButtonPushedFcn = createCallbackFcn(app, @CalcualteButtonPushed, true);
app.CalcualteButton.Position = [271 168 100 22];
app.CalcualteButton.Text = 'Calcualte';
% Create GoodLuckLabel
app.GoodLuckLabel = uilabel(app.UIFigure);
app.GoodLuckLabel.Position = [287 30 70 22];
app.GoodLuckLabel.Text = 'Good Luck !';
end
end
methods (Access = public)
% Construct app
function app = Finalscalc
% Create and configure components
createComponents(app)
% Register the app with App Designer
registerApp(app, app.UIFigure)
% Execute the startup function
runStartupFcn(app, @startupFcn)
if nargout == 0
clear app
end
end
% Code that executes before app deletion
function delete(app)
% Delete UIFigure when app is deleted
delete(app.UIFigure)
end
end
end
matlab user-interface private-members matlab-gui matlab-app-designer
Please explain the scenario better. Where do the external values come from? Are these workspace variables? Variables from an external file that should be loaded at the App start? User interactions in a different GUI? Command line arguments? || As a side note, I would suggest giving shorter names to your variable/controls (e.g.gradeEditField
instead ofPleaseenteryourcurrentgradeEditField
) as this makes the code readable and reduces the probability of typing errors.
– Dev-iL
Nov 11 at 9:37
Basically, it is an app in app designer. I am trying to set each of the variables equal to the values that are inputted into the fields within the app. And then use those variables later in the equation at the end to print the students needed final exam score.
– Austin Justman
Nov 11 at 20:16
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I am trying to collect several values inputted into NumericEditField
controls by a user and store them in order to use them later in a computation, whose result gets presented back.
I seem to be able to store the variables, but am unable to access them later. Each of the variables is considered private because that is where it is having me add the callbacks. I have tried adding all of the variables in a public portion near the beginning, but that did not work.
Here is the code:
classdef Finalscalc < matlab.apps.AppBase
% Properties that correspond to app components
properties (Access = public)
UIFigure matlab.ui.Figure
PleaseenteryourcurrentgradeEditFieldLabel matlab.ui.control.Label
PleaseenteryourcurrentgradeEditField matlab.ui.control.NumericEditField
PleaseenterthegradeyouwantEditFieldLabel matlab.ui.control.Label
PleaseenterthegradeyouwantEditField matlab.ui.control.NumericEditField
PleaseentertheweightofthefinalexamEditFieldLabel matlab.ui.control.Label
PleaseentertheweightofthefinalexamEditField matlab.ui.control.NumericEditField
Label matlab.ui.control.Label
Label_2 matlab.ui.control.Label
Label_3 matlab.ui.control.Label
YouneedatleastaEditFieldLabel matlab.ui.control.Label
YouneedatleastaEditField matlab.ui.control.NumericEditField
Label_4 matlab.ui.control.Label
togetaEditFieldLabel matlab.ui.control.Label
togetaEditField matlab.ui.control.NumericEditField
Label_5 matlab.ui.control.Label
CalcualteButton matlab.ui.control.Button
GoodLuckLabel matlab.ui.control.Label
end
methods (Access = private)
% Code that executes after component creation
function startupFcn(app)
end
% Value changed function: PleaseenteryourcurrentgradeEditField
function PleaseenteryourcurrentgradeEditFieldValueChanged(app, event)
app.current = app.PleaseenteryourcurrentgradeEditField.Value;
current = app.current;
app.current = current;
end
% Value changed function: PleaseenterthegradeyouwantEditField
function PleaseenterthegradeyouwantEditFieldValueChanged(app, event)
app.want = app.PleaseenterthegradeyouwantEditField.Value;
want = app.want;
app.want = want;
end
% Value changed function:
% PleaseentertheweightofthefinalexamEditField
function PleaseentertheweightofthefinalexamEditFieldValueChanged(app, event)
app.weight = app.PleaseentertheweightofthefinalexamEditField.Value;
weight = app.weight;
app.weight = weight;
end
% Button pushed function: CalcualteButton
function CalcualteButtonPushed(app, event)
grade = ((100 * app.want)-(100 - app.weight) * app.current) / app.weight;
grade = app.grade;
app.grade = grade;
end
% Value changed function: YouneedatleastaEditField
function YouneedatleastaEditFieldValueChanged(app, event)
grade = app.YouneedatleastaEditField.Value;
fprintf('%,2f', grade);
end
% Value changed function: togetaEditField
function togetaEditFieldValueChanged(app, event)
app.PleaseenterthegradeyouwantEditField.Value = app.togetaEditField.Value;
end
end
% App initialization and construction
methods (Access = private)
% Create UIFigure and components
function createComponents(app)
% Create UIFigure
app.UIFigure = uifigure;
app.UIFigure.Position = [100 100 640 480];
app.UIFigure.Name = 'UI Figure';
% Create PleaseenteryourcurrentgradeEditFieldLabel
app.PleaseenteryourcurrentgradeEditFieldLabel = uilabel(app.UIFigure);
app.PleaseenteryourcurrentgradeEditFieldLabel.HorizontalAlignment = 'right';
app.PleaseenteryourcurrentgradeEditFieldLabel.Position = [117 337 174 22];
app.PleaseenteryourcurrentgradeEditFieldLabel.Text = 'Please enter your current grade';
% Create PleaseenteryourcurrentgradeEditField
app.PleaseenteryourcurrentgradeEditField = uieditfield(app.UIFigure, 'numeric');
app.PleaseenteryourcurrentgradeEditField.ValueChangedFcn = createCallbackFcn(app, @PleaseenteryourcurrentgradeEditFieldValueChanged, true);
app.PleaseenteryourcurrentgradeEditField.Position = [306 337 100 22];
% Create PleaseenterthegradeyouwantEditFieldLabel
app.PleaseenterthegradeyouwantEditFieldLabel = uilabel(app.UIFigure);
app.PleaseenterthegradeyouwantEditFieldLabel.HorizontalAlignment = 'right';
app.PleaseenterthegradeyouwantEditFieldLabel.Position = [116 289 178 22];
app.PleaseenterthegradeyouwantEditFieldLabel.Text = 'Please enter the grade you want';
% Create PleaseenterthegradeyouwantEditField
app.PleaseenterthegradeyouwantEditField = uieditfield(app.UIFigure, 'numeric');
app.PleaseenterthegradeyouwantEditField.ValueChangedFcn = createCallbackFcn(app, @PleaseenterthegradeyouwantEditFieldValueChanged, true);
app.PleaseenterthegradeyouwantEditField.Position = [309 289 100 22];
% Create PleaseentertheweightofthefinalexamEditFieldLabel
app.PleaseentertheweightofthefinalexamEditFieldLabel = uilabel(app.UIFigure);
app.PleaseentertheweightofthefinalexamEditFieldLabel.HorizontalAlignment = 'right';
app.PleaseentertheweightofthefinalexamEditFieldLabel.Position = [70 248 224 22];
app.PleaseentertheweightofthefinalexamEditFieldLabel.Text = 'Please enter the weight of the final exam';
% Create PleaseentertheweightofthefinalexamEditField
app.PleaseentertheweightofthefinalexamEditField = uieditfield(app.UIFigure, 'numeric');
app.PleaseentertheweightofthefinalexamEditField.ValueChangedFcn = createCallbackFcn(app, @PleaseentertheweightofthefinalexamEditFieldValueChanged, true);
app.PleaseentertheweightofthefinalexamEditField.Position = [309 248 100 22];
% Create Label
app.Label = uilabel(app.UIFigure);
app.Label.Position = [417 337 25 22];
app.Label.Text = '%';
% Create Label_2
app.Label_2 = uilabel(app.UIFigure);
app.Label_2.Position = [417 289 25 22];
app.Label_2.Text = '%';
% Create Label_3
app.Label_3 = uilabel(app.UIFigure);
app.Label_3.Position = [417 248 25 22];
app.Label_3.Text = '%';
% Create YouneedatleastaEditFieldLabel
app.YouneedatleastaEditFieldLabel = uilabel(app.UIFigure);
app.YouneedatleastaEditFieldLabel.HorizontalAlignment = 'right';
app.YouneedatleastaEditFieldLabel.Position = [177 105 107 22];
app.YouneedatleastaEditFieldLabel.Text = 'You need at least a';
% Create YouneedatleastaEditField
app.YouneedatleastaEditField = uieditfield(app.UIFigure, 'numeric');
app.YouneedatleastaEditField.ValueChangedFcn = createCallbackFcn(app, @YouneedatleastaEditFieldValueChanged, true);
app.YouneedatleastaEditField.Position = [291 105 19 22];
% Create Label_4
app.Label_4 = uilabel(app.UIFigure);
app.Label_4.Position = [323 105 25 22];
app.Label_4.Text = '%';
% Create togetaEditFieldLabel
app.togetaEditFieldLabel = uilabel(app.UIFigure);
app.togetaEditFieldLabel.HorizontalAlignment = 'right';
app.togetaEditFieldLabel.Position = [337 105 46 22];
app.togetaEditFieldLabel.Text = 'to get a';
% Create togetaEditField
app.togetaEditField = uieditfield(app.UIFigure, 'numeric');
app.togetaEditField.ValueChangedFcn = createCallbackFcn(app, @togetaEditFieldValueChanged, true);
app.togetaEditField.Position = [390 105 16 22];
% Create Label_5
app.Label_5 = uilabel(app.UIFigure);
app.Label_5.Position = [417 105 25 22];
app.Label_5.Text = '%';
% Create CalcualteButton
app.CalcualteButton = uibutton(app.UIFigure, 'push');
app.CalcualteButton.ButtonPushedFcn = createCallbackFcn(app, @CalcualteButtonPushed, true);
app.CalcualteButton.Position = [271 168 100 22];
app.CalcualteButton.Text = 'Calcualte';
% Create GoodLuckLabel
app.GoodLuckLabel = uilabel(app.UIFigure);
app.GoodLuckLabel.Position = [287 30 70 22];
app.GoodLuckLabel.Text = 'Good Luck !';
end
end
methods (Access = public)
% Construct app
function app = Finalscalc
% Create and configure components
createComponents(app)
% Register the app with App Designer
registerApp(app, app.UIFigure)
% Execute the startup function
runStartupFcn(app, @startupFcn)
if nargout == 0
clear app
end
end
% Code that executes before app deletion
function delete(app)
% Delete UIFigure when app is deleted
delete(app.UIFigure)
end
end
end
matlab user-interface private-members matlab-gui matlab-app-designer
I am trying to collect several values inputted into NumericEditField
controls by a user and store them in order to use them later in a computation, whose result gets presented back.
I seem to be able to store the variables, but am unable to access them later. Each of the variables is considered private because that is where it is having me add the callbacks. I have tried adding all of the variables in a public portion near the beginning, but that did not work.
Here is the code:
classdef Finalscalc < matlab.apps.AppBase
% Properties that correspond to app components
properties (Access = public)
UIFigure matlab.ui.Figure
PleaseenteryourcurrentgradeEditFieldLabel matlab.ui.control.Label
PleaseenteryourcurrentgradeEditField matlab.ui.control.NumericEditField
PleaseenterthegradeyouwantEditFieldLabel matlab.ui.control.Label
PleaseenterthegradeyouwantEditField matlab.ui.control.NumericEditField
PleaseentertheweightofthefinalexamEditFieldLabel matlab.ui.control.Label
PleaseentertheweightofthefinalexamEditField matlab.ui.control.NumericEditField
Label matlab.ui.control.Label
Label_2 matlab.ui.control.Label
Label_3 matlab.ui.control.Label
YouneedatleastaEditFieldLabel matlab.ui.control.Label
YouneedatleastaEditField matlab.ui.control.NumericEditField
Label_4 matlab.ui.control.Label
togetaEditFieldLabel matlab.ui.control.Label
togetaEditField matlab.ui.control.NumericEditField
Label_5 matlab.ui.control.Label
CalcualteButton matlab.ui.control.Button
GoodLuckLabel matlab.ui.control.Label
end
methods (Access = private)
% Code that executes after component creation
function startupFcn(app)
end
% Value changed function: PleaseenteryourcurrentgradeEditField
function PleaseenteryourcurrentgradeEditFieldValueChanged(app, event)
app.current = app.PleaseenteryourcurrentgradeEditField.Value;
current = app.current;
app.current = current;
end
% Value changed function: PleaseenterthegradeyouwantEditField
function PleaseenterthegradeyouwantEditFieldValueChanged(app, event)
app.want = app.PleaseenterthegradeyouwantEditField.Value;
want = app.want;
app.want = want;
end
% Value changed function:
% PleaseentertheweightofthefinalexamEditField
function PleaseentertheweightofthefinalexamEditFieldValueChanged(app, event)
app.weight = app.PleaseentertheweightofthefinalexamEditField.Value;
weight = app.weight;
app.weight = weight;
end
% Button pushed function: CalcualteButton
function CalcualteButtonPushed(app, event)
grade = ((100 * app.want)-(100 - app.weight) * app.current) / app.weight;
grade = app.grade;
app.grade = grade;
end
% Value changed function: YouneedatleastaEditField
function YouneedatleastaEditFieldValueChanged(app, event)
grade = app.YouneedatleastaEditField.Value;
fprintf('%,2f', grade);
end
% Value changed function: togetaEditField
function togetaEditFieldValueChanged(app, event)
app.PleaseenterthegradeyouwantEditField.Value = app.togetaEditField.Value;
end
end
% App initialization and construction
methods (Access = private)
% Create UIFigure and components
function createComponents(app)
% Create UIFigure
app.UIFigure = uifigure;
app.UIFigure.Position = [100 100 640 480];
app.UIFigure.Name = 'UI Figure';
% Create PleaseenteryourcurrentgradeEditFieldLabel
app.PleaseenteryourcurrentgradeEditFieldLabel = uilabel(app.UIFigure);
app.PleaseenteryourcurrentgradeEditFieldLabel.HorizontalAlignment = 'right';
app.PleaseenteryourcurrentgradeEditFieldLabel.Position = [117 337 174 22];
app.PleaseenteryourcurrentgradeEditFieldLabel.Text = 'Please enter your current grade';
% Create PleaseenteryourcurrentgradeEditField
app.PleaseenteryourcurrentgradeEditField = uieditfield(app.UIFigure, 'numeric');
app.PleaseenteryourcurrentgradeEditField.ValueChangedFcn = createCallbackFcn(app, @PleaseenteryourcurrentgradeEditFieldValueChanged, true);
app.PleaseenteryourcurrentgradeEditField.Position = [306 337 100 22];
% Create PleaseenterthegradeyouwantEditFieldLabel
app.PleaseenterthegradeyouwantEditFieldLabel = uilabel(app.UIFigure);
app.PleaseenterthegradeyouwantEditFieldLabel.HorizontalAlignment = 'right';
app.PleaseenterthegradeyouwantEditFieldLabel.Position = [116 289 178 22];
app.PleaseenterthegradeyouwantEditFieldLabel.Text = 'Please enter the grade you want';
% Create PleaseenterthegradeyouwantEditField
app.PleaseenterthegradeyouwantEditField = uieditfield(app.UIFigure, 'numeric');
app.PleaseenterthegradeyouwantEditField.ValueChangedFcn = createCallbackFcn(app, @PleaseenterthegradeyouwantEditFieldValueChanged, true);
app.PleaseenterthegradeyouwantEditField.Position = [309 289 100 22];
% Create PleaseentertheweightofthefinalexamEditFieldLabel
app.PleaseentertheweightofthefinalexamEditFieldLabel = uilabel(app.UIFigure);
app.PleaseentertheweightofthefinalexamEditFieldLabel.HorizontalAlignment = 'right';
app.PleaseentertheweightofthefinalexamEditFieldLabel.Position = [70 248 224 22];
app.PleaseentertheweightofthefinalexamEditFieldLabel.Text = 'Please enter the weight of the final exam';
% Create PleaseentertheweightofthefinalexamEditField
app.PleaseentertheweightofthefinalexamEditField = uieditfield(app.UIFigure, 'numeric');
app.PleaseentertheweightofthefinalexamEditField.ValueChangedFcn = createCallbackFcn(app, @PleaseentertheweightofthefinalexamEditFieldValueChanged, true);
app.PleaseentertheweightofthefinalexamEditField.Position = [309 248 100 22];
% Create Label
app.Label = uilabel(app.UIFigure);
app.Label.Position = [417 337 25 22];
app.Label.Text = '%';
% Create Label_2
app.Label_2 = uilabel(app.UIFigure);
app.Label_2.Position = [417 289 25 22];
app.Label_2.Text = '%';
% Create Label_3
app.Label_3 = uilabel(app.UIFigure);
app.Label_3.Position = [417 248 25 22];
app.Label_3.Text = '%';
% Create YouneedatleastaEditFieldLabel
app.YouneedatleastaEditFieldLabel = uilabel(app.UIFigure);
app.YouneedatleastaEditFieldLabel.HorizontalAlignment = 'right';
app.YouneedatleastaEditFieldLabel.Position = [177 105 107 22];
app.YouneedatleastaEditFieldLabel.Text = 'You need at least a';
% Create YouneedatleastaEditField
app.YouneedatleastaEditField = uieditfield(app.UIFigure, 'numeric');
app.YouneedatleastaEditField.ValueChangedFcn = createCallbackFcn(app, @YouneedatleastaEditFieldValueChanged, true);
app.YouneedatleastaEditField.Position = [291 105 19 22];
% Create Label_4
app.Label_4 = uilabel(app.UIFigure);
app.Label_4.Position = [323 105 25 22];
app.Label_4.Text = '%';
% Create togetaEditFieldLabel
app.togetaEditFieldLabel = uilabel(app.UIFigure);
app.togetaEditFieldLabel.HorizontalAlignment = 'right';
app.togetaEditFieldLabel.Position = [337 105 46 22];
app.togetaEditFieldLabel.Text = 'to get a';
% Create togetaEditField
app.togetaEditField = uieditfield(app.UIFigure, 'numeric');
app.togetaEditField.ValueChangedFcn = createCallbackFcn(app, @togetaEditFieldValueChanged, true);
app.togetaEditField.Position = [390 105 16 22];
% Create Label_5
app.Label_5 = uilabel(app.UIFigure);
app.Label_5.Position = [417 105 25 22];
app.Label_5.Text = '%';
% Create CalcualteButton
app.CalcualteButton = uibutton(app.UIFigure, 'push');
app.CalcualteButton.ButtonPushedFcn = createCallbackFcn(app, @CalcualteButtonPushed, true);
app.CalcualteButton.Position = [271 168 100 22];
app.CalcualteButton.Text = 'Calcualte';
% Create GoodLuckLabel
app.GoodLuckLabel = uilabel(app.UIFigure);
app.GoodLuckLabel.Position = [287 30 70 22];
app.GoodLuckLabel.Text = 'Good Luck !';
end
end
methods (Access = public)
% Construct app
function app = Finalscalc
% Create and configure components
createComponents(app)
% Register the app with App Designer
registerApp(app, app.UIFigure)
% Execute the startup function
runStartupFcn(app, @startupFcn)
if nargout == 0
clear app
end
end
% Code that executes before app deletion
function delete(app)
% Delete UIFigure when app is deleted
delete(app.UIFigure)
end
end
end
matlab user-interface private-members matlab-gui matlab-app-designer
matlab user-interface private-members matlab-gui matlab-app-designer
edited Nov 12 at 8:25


Dev-iL
16.3k64074
16.3k64074
asked Nov 11 at 0:58


Austin Justman
62
62
Please explain the scenario better. Where do the external values come from? Are these workspace variables? Variables from an external file that should be loaded at the App start? User interactions in a different GUI? Command line arguments? || As a side note, I would suggest giving shorter names to your variable/controls (e.g.gradeEditField
instead ofPleaseenteryourcurrentgradeEditField
) as this makes the code readable and reduces the probability of typing errors.
– Dev-iL
Nov 11 at 9:37
Basically, it is an app in app designer. I am trying to set each of the variables equal to the values that are inputted into the fields within the app. And then use those variables later in the equation at the end to print the students needed final exam score.
– Austin Justman
Nov 11 at 20:16
add a comment |
Please explain the scenario better. Where do the external values come from? Are these workspace variables? Variables from an external file that should be loaded at the App start? User interactions in a different GUI? Command line arguments? || As a side note, I would suggest giving shorter names to your variable/controls (e.g.gradeEditField
instead ofPleaseenteryourcurrentgradeEditField
) as this makes the code readable and reduces the probability of typing errors.
– Dev-iL
Nov 11 at 9:37
Basically, it is an app in app designer. I am trying to set each of the variables equal to the values that are inputted into the fields within the app. And then use those variables later in the equation at the end to print the students needed final exam score.
– Austin Justman
Nov 11 at 20:16
Please explain the scenario better. Where do the external values come from? Are these workspace variables? Variables from an external file that should be loaded at the App start? User interactions in a different GUI? Command line arguments? || As a side note, I would suggest giving shorter names to your variable/controls (e.g.
gradeEditField
instead of PleaseenteryourcurrentgradeEditField
) as this makes the code readable and reduces the probability of typing errors.– Dev-iL
Nov 11 at 9:37
Please explain the scenario better. Where do the external values come from? Are these workspace variables? Variables from an external file that should be loaded at the App start? User interactions in a different GUI? Command line arguments? || As a side note, I would suggest giving shorter names to your variable/controls (e.g.
gradeEditField
instead of PleaseenteryourcurrentgradeEditField
) as this makes the code readable and reduces the probability of typing errors.– Dev-iL
Nov 11 at 9:37
Basically, it is an app in app designer. I am trying to set each of the variables equal to the values that are inputted into the fields within the app. And then use those variables later in the equation at the end to print the students needed final exam score.
– Austin Justman
Nov 11 at 20:16
Basically, it is an app in app designer. I am trying to set each of the variables equal to the values that are inputted into the fields within the app. And then use those variables later in the equation at the end to print the students needed final exam score.
– Austin Justman
Nov 11 at 20:16
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
Instead of using the ...ValueChanged
functions all you need to do is access the Value
field of the various controls from which you want to get values. Replace the current private methods
block you have with this:
methods (Access = private)
% Button pushed function: CalcualteButton
function CalcualteButtonPushed(app, ~)
% Get values from edit fields:
want = app.PleaseenterthegradeyouwantEditField.Value;
weight = app.PleaseentertheweightofthefinalexamEditField.Value;
current = app.PleaseenteryourcurrentgradeEditField.Value;
% Perform computation:
needed = ( (100 * want) - (100 - weight) * current) / weight;
% Write values to new edit fields:
app.YouneedatleastaEditField.Value = needed;
app.togetaEditField.Value = want;
end
end
In my humble opinion, TMW greatly managed to simplify the access of values from text fields in the new UIFigure system - from here it's just a matter of understanding how it works.
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
Instead of using the ...ValueChanged
functions all you need to do is access the Value
field of the various controls from which you want to get values. Replace the current private methods
block you have with this:
methods (Access = private)
% Button pushed function: CalcualteButton
function CalcualteButtonPushed(app, ~)
% Get values from edit fields:
want = app.PleaseenterthegradeyouwantEditField.Value;
weight = app.PleaseentertheweightofthefinalexamEditField.Value;
current = app.PleaseenteryourcurrentgradeEditField.Value;
% Perform computation:
needed = ( (100 * want) - (100 - weight) * current) / weight;
% Write values to new edit fields:
app.YouneedatleastaEditField.Value = needed;
app.togetaEditField.Value = want;
end
end
In my humble opinion, TMW greatly managed to simplify the access of values from text fields in the new UIFigure system - from here it's just a matter of understanding how it works.
add a comment |
up vote
0
down vote
Instead of using the ...ValueChanged
functions all you need to do is access the Value
field of the various controls from which you want to get values. Replace the current private methods
block you have with this:
methods (Access = private)
% Button pushed function: CalcualteButton
function CalcualteButtonPushed(app, ~)
% Get values from edit fields:
want = app.PleaseenterthegradeyouwantEditField.Value;
weight = app.PleaseentertheweightofthefinalexamEditField.Value;
current = app.PleaseenteryourcurrentgradeEditField.Value;
% Perform computation:
needed = ( (100 * want) - (100 - weight) * current) / weight;
% Write values to new edit fields:
app.YouneedatleastaEditField.Value = needed;
app.togetaEditField.Value = want;
end
end
In my humble opinion, TMW greatly managed to simplify the access of values from text fields in the new UIFigure system - from here it's just a matter of understanding how it works.
add a comment |
up vote
0
down vote
up vote
0
down vote
Instead of using the ...ValueChanged
functions all you need to do is access the Value
field of the various controls from which you want to get values. Replace the current private methods
block you have with this:
methods (Access = private)
% Button pushed function: CalcualteButton
function CalcualteButtonPushed(app, ~)
% Get values from edit fields:
want = app.PleaseenterthegradeyouwantEditField.Value;
weight = app.PleaseentertheweightofthefinalexamEditField.Value;
current = app.PleaseenteryourcurrentgradeEditField.Value;
% Perform computation:
needed = ( (100 * want) - (100 - weight) * current) / weight;
% Write values to new edit fields:
app.YouneedatleastaEditField.Value = needed;
app.togetaEditField.Value = want;
end
end
In my humble opinion, TMW greatly managed to simplify the access of values from text fields in the new UIFigure system - from here it's just a matter of understanding how it works.
Instead of using the ...ValueChanged
functions all you need to do is access the Value
field of the various controls from which you want to get values. Replace the current private methods
block you have with this:
methods (Access = private)
% Button pushed function: CalcualteButton
function CalcualteButtonPushed(app, ~)
% Get values from edit fields:
want = app.PleaseenterthegradeyouwantEditField.Value;
weight = app.PleaseentertheweightofthefinalexamEditField.Value;
current = app.PleaseenteryourcurrentgradeEditField.Value;
% Perform computation:
needed = ( (100 * want) - (100 - weight) * current) / weight;
% Write values to new edit fields:
app.YouneedatleastaEditField.Value = needed;
app.togetaEditField.Value = want;
end
end
In my humble opinion, TMW greatly managed to simplify the access of values from text fields in the new UIFigure system - from here it's just a matter of understanding how it works.
answered Nov 12 at 8:19


Dev-iL
16.3k64074
16.3k64074
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53244910%2funable-to-access-the-values-a-user-inputs-into-text-boxes%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
c,rFe9xZTtBb,OaDp h0NBu75J72lmjULbolbCsbZVzz0
Please explain the scenario better. Where do the external values come from? Are these workspace variables? Variables from an external file that should be loaded at the App start? User interactions in a different GUI? Command line arguments? || As a side note, I would suggest giving shorter names to your variable/controls (e.g.
gradeEditField
instead ofPleaseenteryourcurrentgradeEditField
) as this makes the code readable and reduces the probability of typing errors.– Dev-iL
Nov 11 at 9:37
Basically, it is an app in app designer. I am trying to set each of the variables equal to the values that are inputted into the fields within the app. And then use those variables later in the equation at the end to print the students needed final exam score.
– Austin Justman
Nov 11 at 20:16