'child class' object has no attribute 'attribute_name'
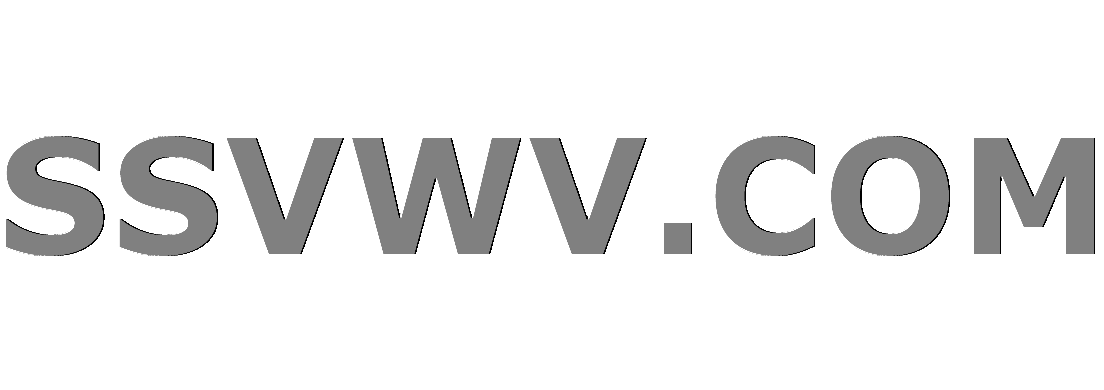
Multi tool use
up vote
0
down vote
favorite
I can not retrieve the value of a variable created only by the inherited child class.
Also, changing the value of a variable inherited from the parent class in the init of the child class does not apply.
Here's the parent class:
class Car():
def __init__(self, make, model, year):
self.make = make
self.model = model
self.year = year
self.odometer_reading = 0
def __str__(self):
return str(self.__class__) + ": " + str(self.__dict__)
def get_descriptive_name(self):
long_name = str(self.year) + ' ' + self.make + ' ' + self.model
return long_name.title()
def read_odometer(self):
print("This car has " + str(self.odometer_reading) + " miles on it.")
def update_odometer(self, mileage):
if mileage >= self.odometer_reading:
self.odometer_reading = mileage
else:
print("You can't roll back an odometer!")
def increment_odometer(self, miles):
self.odometer_reading += miles
And here's the child class:
class ElectricCar(Car):
def __init___(self, make, model, year):
super(ElectricCar, self).__init__(make, model, year)
self.odometer_reading = 100
self.battery_size = 70
def __str__(self):
return str(self.__class__) + ": " + str(self.__dict__)
def describe_battery(self):
print(self.battery_size)
Now, if I try to run this code:
my_tesla = ElectricCar('tesla', 'model s', 2016)
print(my_tesla)
print(my_tesla.describe_battery())
I get the following exception:
<class '__main__.ElectricCar'>:
{'make': 'tesla', 'model': 'model s', 'year': 2016, 'odometer_reading': 0}
AttributeError: 'ElectricCar' object has no attribute 'battery_size'
I have an oddmeter_reading variable whose value is 0 in the parent class.
I changed from child class to 100, but it did not apply.
Also, the variable battery_size
, which is set only in the child class, is not created in init.
What's the problem? What am I missing?
python python-3.x class attributes super
New contributor
Ssungnni is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
favorite
I can not retrieve the value of a variable created only by the inherited child class.
Also, changing the value of a variable inherited from the parent class in the init of the child class does not apply.
Here's the parent class:
class Car():
def __init__(self, make, model, year):
self.make = make
self.model = model
self.year = year
self.odometer_reading = 0
def __str__(self):
return str(self.__class__) + ": " + str(self.__dict__)
def get_descriptive_name(self):
long_name = str(self.year) + ' ' + self.make + ' ' + self.model
return long_name.title()
def read_odometer(self):
print("This car has " + str(self.odometer_reading) + " miles on it.")
def update_odometer(self, mileage):
if mileage >= self.odometer_reading:
self.odometer_reading = mileage
else:
print("You can't roll back an odometer!")
def increment_odometer(self, miles):
self.odometer_reading += miles
And here's the child class:
class ElectricCar(Car):
def __init___(self, make, model, year):
super(ElectricCar, self).__init__(make, model, year)
self.odometer_reading = 100
self.battery_size = 70
def __str__(self):
return str(self.__class__) + ": " + str(self.__dict__)
def describe_battery(self):
print(self.battery_size)
Now, if I try to run this code:
my_tesla = ElectricCar('tesla', 'model s', 2016)
print(my_tesla)
print(my_tesla.describe_battery())
I get the following exception:
<class '__main__.ElectricCar'>:
{'make': 'tesla', 'model': 'model s', 'year': 2016, 'odometer_reading': 0}
AttributeError: 'ElectricCar' object has no attribute 'battery_size'
I have an oddmeter_reading variable whose value is 0 in the parent class.
I changed from child class to 100, but it did not apply.
Also, the variable battery_size
, which is set only in the child class, is not created in init.
What's the problem? What am I missing?
python python-3.x class attributes super
New contributor
Ssungnni is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
2
You wrote__init__
with three underscores at the right, so you did not "patch" the constructor.
– Willem Van Onsem
Nov 10 at 12:15
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I can not retrieve the value of a variable created only by the inherited child class.
Also, changing the value of a variable inherited from the parent class in the init of the child class does not apply.
Here's the parent class:
class Car():
def __init__(self, make, model, year):
self.make = make
self.model = model
self.year = year
self.odometer_reading = 0
def __str__(self):
return str(self.__class__) + ": " + str(self.__dict__)
def get_descriptive_name(self):
long_name = str(self.year) + ' ' + self.make + ' ' + self.model
return long_name.title()
def read_odometer(self):
print("This car has " + str(self.odometer_reading) + " miles on it.")
def update_odometer(self, mileage):
if mileage >= self.odometer_reading:
self.odometer_reading = mileage
else:
print("You can't roll back an odometer!")
def increment_odometer(self, miles):
self.odometer_reading += miles
And here's the child class:
class ElectricCar(Car):
def __init___(self, make, model, year):
super(ElectricCar, self).__init__(make, model, year)
self.odometer_reading = 100
self.battery_size = 70
def __str__(self):
return str(self.__class__) + ": " + str(self.__dict__)
def describe_battery(self):
print(self.battery_size)
Now, if I try to run this code:
my_tesla = ElectricCar('tesla', 'model s', 2016)
print(my_tesla)
print(my_tesla.describe_battery())
I get the following exception:
<class '__main__.ElectricCar'>:
{'make': 'tesla', 'model': 'model s', 'year': 2016, 'odometer_reading': 0}
AttributeError: 'ElectricCar' object has no attribute 'battery_size'
I have an oddmeter_reading variable whose value is 0 in the parent class.
I changed from child class to 100, but it did not apply.
Also, the variable battery_size
, which is set only in the child class, is not created in init.
What's the problem? What am I missing?
python python-3.x class attributes super
New contributor
Ssungnni is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
I can not retrieve the value of a variable created only by the inherited child class.
Also, changing the value of a variable inherited from the parent class in the init of the child class does not apply.
Here's the parent class:
class Car():
def __init__(self, make, model, year):
self.make = make
self.model = model
self.year = year
self.odometer_reading = 0
def __str__(self):
return str(self.__class__) + ": " + str(self.__dict__)
def get_descriptive_name(self):
long_name = str(self.year) + ' ' + self.make + ' ' + self.model
return long_name.title()
def read_odometer(self):
print("This car has " + str(self.odometer_reading) + " miles on it.")
def update_odometer(self, mileage):
if mileage >= self.odometer_reading:
self.odometer_reading = mileage
else:
print("You can't roll back an odometer!")
def increment_odometer(self, miles):
self.odometer_reading += miles
And here's the child class:
class ElectricCar(Car):
def __init___(self, make, model, year):
super(ElectricCar, self).__init__(make, model, year)
self.odometer_reading = 100
self.battery_size = 70
def __str__(self):
return str(self.__class__) + ": " + str(self.__dict__)
def describe_battery(self):
print(self.battery_size)
Now, if I try to run this code:
my_tesla = ElectricCar('tesla', 'model s', 2016)
print(my_tesla)
print(my_tesla.describe_battery())
I get the following exception:
<class '__main__.ElectricCar'>:
{'make': 'tesla', 'model': 'model s', 'year': 2016, 'odometer_reading': 0}
AttributeError: 'ElectricCar' object has no attribute 'battery_size'
I have an oddmeter_reading variable whose value is 0 in the parent class.
I changed from child class to 100, but it did not apply.
Also, the variable battery_size
, which is set only in the child class, is not created in init.
What's the problem? What am I missing?
python python-3.x class attributes super
python python-3.x class attributes super
New contributor
Ssungnni is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Ssungnni is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
edited Nov 10 at 13:11


martineau
64.2k887170
64.2k887170
New contributor
Ssungnni is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked Nov 10 at 12:12
Ssungnni
1
1
New contributor
Ssungnni is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Ssungnni is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Ssungnni is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
2
You wrote__init__
with three underscores at the right, so you did not "patch" the constructor.
– Willem Van Onsem
Nov 10 at 12:15
add a comment |
2
You wrote__init__
with three underscores at the right, so you did not "patch" the constructor.
– Willem Van Onsem
Nov 10 at 12:15
2
2
You wrote
__init__
with three underscores at the right, so you did not "patch" the constructor.– Willem Van Onsem
Nov 10 at 12:15
You wrote
__init__
with three underscores at the right, so you did not "patch" the constructor.– Willem Van Onsem
Nov 10 at 12:15
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Ssungnni is a new contributor. Be nice, and check out our Code of Conduct.
Ssungnni is a new contributor. Be nice, and check out our Code of Conduct.
Ssungnni is a new contributor. Be nice, and check out our Code of Conduct.
Ssungnni is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53238824%2fchild-class-object-has-no-attribute-attribute-name%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
UE9s1S3FgQM1qbme7Al,yfX,V r mv4z,wdMqwEm,msA4h5H,c liTvyvQii9VF0Jf1P,vM1H4Ew,SEl7V,G21a
2
You wrote
__init__
with three underscores at the right, so you did not "patch" the constructor.– Willem Van Onsem
Nov 10 at 12:15