PHP7 pthreads3 woes : Unable to increment public property of Threaded class from within an instantiated...
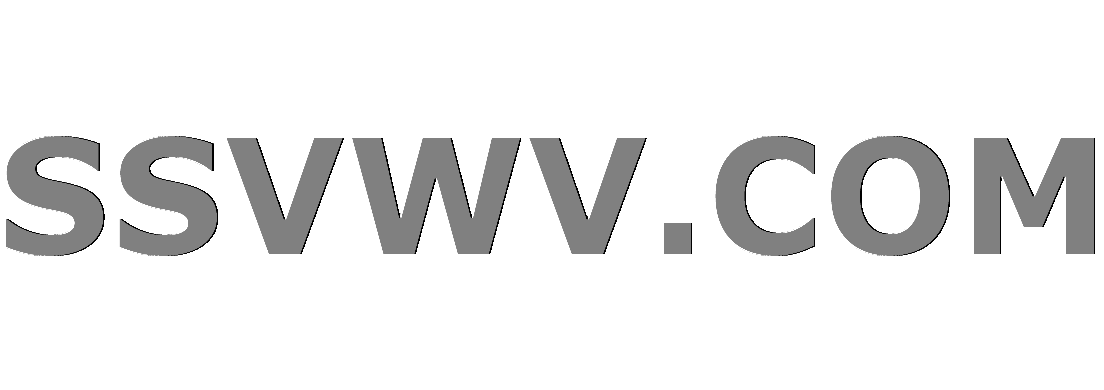
Multi tool use
up vote
1
down vote
favorite
I am confused by the results from the following simplified code.
A Threaded object MyPool is to instantiate a variable number of MyWorker objects. As each MyWorker object is correctly instantiated, it should increment the $counter inside the MyPool class, to reflect how many correctly instantiated workers there are.
When called from inside a MyWorker object, the increaseCounter() function appears to execute (as per echo statement), but has no affect on the $counter property. However calling the same from the main thread works as expected.
How do I implement this simple concept?
<?php
error_reporting(E_ALL & ~E_NOTICE);
class MyPool extends Threaded
{
public static $counter;
public $workers;
public function __construct()
{
self::$counter = (int) 0;
$workers = new MyWorker();
$workers = new MyWorker();
$workers = new MyWorker();
$workers[0]->start();
$workers[1]->start();
$workers[2]->start();
}
public static function getCounter()
{
return self::$counter;
}
public static function increaseCounter()
{
self::$counter++;
echo "counter has been increased inside MyPooln";
}
}
class MyWorker extends Worker
{
public function __construct()
{
}
public function run()
{
// do something then
$this->WorkerActive();
}
public function WorkerActive()
{
MyPool::$counter++;
MyPool::increaseCounter();
}
}
$MyPool = new MyPool();
echo "counter = ".MyPool::$counter."n";
echo "counter = ".$MyPool->getCounter()."n";
echo "counter = ".MyPool::getCounter()."n";
MyPool::$counter++;
MyPool::increaseCounter();
echo "counter = ".MyPool::$counter."n";
echo "counter = ".$MyPool->getCounter()."n";
echo "counter = ".MyPool::getCounter()."n";
The output is:
counter has been increased inside MyPool
counter has been increased inside MyPool
counter has been increased inside MyPool
counter = 0
counter = 0
counter = 0
counter has been increased inside MyPool
counter = 2
counter = 2
counter = 2
php scope static pthreads visibility
add a comment |
up vote
1
down vote
favorite
I am confused by the results from the following simplified code.
A Threaded object MyPool is to instantiate a variable number of MyWorker objects. As each MyWorker object is correctly instantiated, it should increment the $counter inside the MyPool class, to reflect how many correctly instantiated workers there are.
When called from inside a MyWorker object, the increaseCounter() function appears to execute (as per echo statement), but has no affect on the $counter property. However calling the same from the main thread works as expected.
How do I implement this simple concept?
<?php
error_reporting(E_ALL & ~E_NOTICE);
class MyPool extends Threaded
{
public static $counter;
public $workers;
public function __construct()
{
self::$counter = (int) 0;
$workers = new MyWorker();
$workers = new MyWorker();
$workers = new MyWorker();
$workers[0]->start();
$workers[1]->start();
$workers[2]->start();
}
public static function getCounter()
{
return self::$counter;
}
public static function increaseCounter()
{
self::$counter++;
echo "counter has been increased inside MyPooln";
}
}
class MyWorker extends Worker
{
public function __construct()
{
}
public function run()
{
// do something then
$this->WorkerActive();
}
public function WorkerActive()
{
MyPool::$counter++;
MyPool::increaseCounter();
}
}
$MyPool = new MyPool();
echo "counter = ".MyPool::$counter."n";
echo "counter = ".$MyPool->getCounter()."n";
echo "counter = ".MyPool::getCounter()."n";
MyPool::$counter++;
MyPool::increaseCounter();
echo "counter = ".MyPool::$counter."n";
echo "counter = ".$MyPool->getCounter()."n";
echo "counter = ".MyPool::getCounter()."n";
The output is:
counter has been increased inside MyPool
counter has been increased inside MyPool
counter has been increased inside MyPool
counter = 0
counter = 0
counter = 0
counter has been increased inside MyPool
counter = 2
counter = 2
counter = 2
php scope static pthreads visibility
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I am confused by the results from the following simplified code.
A Threaded object MyPool is to instantiate a variable number of MyWorker objects. As each MyWorker object is correctly instantiated, it should increment the $counter inside the MyPool class, to reflect how many correctly instantiated workers there are.
When called from inside a MyWorker object, the increaseCounter() function appears to execute (as per echo statement), but has no affect on the $counter property. However calling the same from the main thread works as expected.
How do I implement this simple concept?
<?php
error_reporting(E_ALL & ~E_NOTICE);
class MyPool extends Threaded
{
public static $counter;
public $workers;
public function __construct()
{
self::$counter = (int) 0;
$workers = new MyWorker();
$workers = new MyWorker();
$workers = new MyWorker();
$workers[0]->start();
$workers[1]->start();
$workers[2]->start();
}
public static function getCounter()
{
return self::$counter;
}
public static function increaseCounter()
{
self::$counter++;
echo "counter has been increased inside MyPooln";
}
}
class MyWorker extends Worker
{
public function __construct()
{
}
public function run()
{
// do something then
$this->WorkerActive();
}
public function WorkerActive()
{
MyPool::$counter++;
MyPool::increaseCounter();
}
}
$MyPool = new MyPool();
echo "counter = ".MyPool::$counter."n";
echo "counter = ".$MyPool->getCounter()."n";
echo "counter = ".MyPool::getCounter()."n";
MyPool::$counter++;
MyPool::increaseCounter();
echo "counter = ".MyPool::$counter."n";
echo "counter = ".$MyPool->getCounter()."n";
echo "counter = ".MyPool::getCounter()."n";
The output is:
counter has been increased inside MyPool
counter has been increased inside MyPool
counter has been increased inside MyPool
counter = 0
counter = 0
counter = 0
counter has been increased inside MyPool
counter = 2
counter = 2
counter = 2
php scope static pthreads visibility
I am confused by the results from the following simplified code.
A Threaded object MyPool is to instantiate a variable number of MyWorker objects. As each MyWorker object is correctly instantiated, it should increment the $counter inside the MyPool class, to reflect how many correctly instantiated workers there are.
When called from inside a MyWorker object, the increaseCounter() function appears to execute (as per echo statement), but has no affect on the $counter property. However calling the same from the main thread works as expected.
How do I implement this simple concept?
<?php
error_reporting(E_ALL & ~E_NOTICE);
class MyPool extends Threaded
{
public static $counter;
public $workers;
public function __construct()
{
self::$counter = (int) 0;
$workers = new MyWorker();
$workers = new MyWorker();
$workers = new MyWorker();
$workers[0]->start();
$workers[1]->start();
$workers[2]->start();
}
public static function getCounter()
{
return self::$counter;
}
public static function increaseCounter()
{
self::$counter++;
echo "counter has been increased inside MyPooln";
}
}
class MyWorker extends Worker
{
public function __construct()
{
}
public function run()
{
// do something then
$this->WorkerActive();
}
public function WorkerActive()
{
MyPool::$counter++;
MyPool::increaseCounter();
}
}
$MyPool = new MyPool();
echo "counter = ".MyPool::$counter."n";
echo "counter = ".$MyPool->getCounter()."n";
echo "counter = ".MyPool::getCounter()."n";
MyPool::$counter++;
MyPool::increaseCounter();
echo "counter = ".MyPool::$counter."n";
echo "counter = ".$MyPool->getCounter()."n";
echo "counter = ".MyPool::getCounter()."n";
The output is:
counter has been increased inside MyPool
counter has been increased inside MyPool
counter has been increased inside MyPool
counter = 0
counter = 0
counter = 0
counter has been increased inside MyPool
counter = 2
counter = 2
counter = 2
php scope static pthreads visibility
php scope static pthreads visibility
asked Nov 7 at 11:03
Viktorius
93
93
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
In pthreads static properties are thread-local and not shared between threads. Switch $counter property to object scope will help.
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
In pthreads static properties are thread-local and not shared between threads. Switch $counter property to object scope will help.
add a comment |
up vote
0
down vote
In pthreads static properties are thread-local and not shared between threads. Switch $counter property to object scope will help.
add a comment |
up vote
0
down vote
up vote
0
down vote
In pthreads static properties are thread-local and not shared between threads. Switch $counter property to object scope will help.
In pthreads static properties are thread-local and not shared between threads. Switch $counter property to object scope will help.
answered Nov 10 at 22:45
SirSnyder
462
462
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53188220%2fphp7-pthreads3-woes-unable-to-increment-public-property-of-threaded-class-from%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
q8tovW5HzDVoUFie2E0A9eJr7UyTfu2qecYVn0LAbxvlV D8EyaJj37BBwmt,Ncxh g94XTJis