How can I find the average of a list with multiple cells?
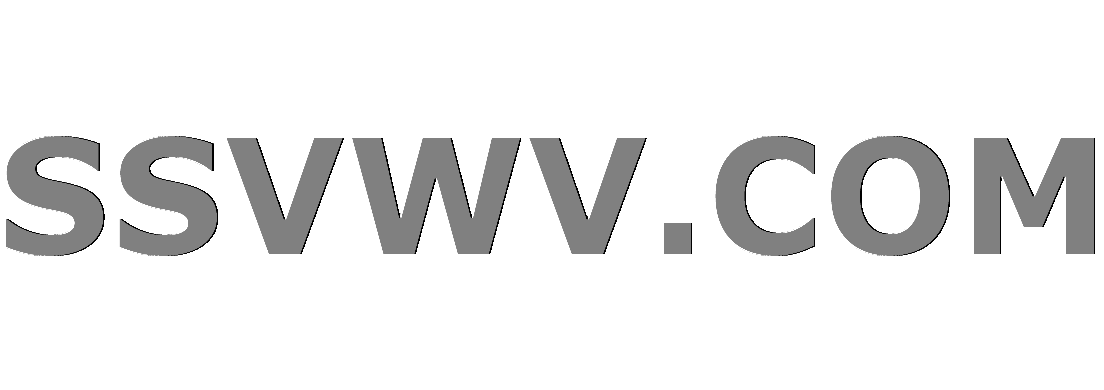
Multi tool use
up vote
-2
down vote
favorite
Example: x = [[1,3,2],[4,5,6],[7,8,9]]
I attempted to use:
sum(x) / len(x)
but it seems to give me this error
(TypeError: unsupported operand type(s) for +: 'int' and 'list')
The list is inputted by the user with command: average_list() and could contain any amount of numbers per square brackets.
python
add a comment |
up vote
-2
down vote
favorite
Example: x = [[1,3,2],[4,5,6],[7,8,9]]
I attempted to use:
sum(x) / len(x)
but it seems to give me this error
(TypeError: unsupported operand type(s) for +: 'int' and 'list')
The list is inputted by the user with command: average_list() and could contain any amount of numbers per square brackets.
python
2
Do you want the average of complete list or average of lists inside list?
– Sanchit Kumar
Nov 11 at 0:50
I want the average of the complete list! Sorry for not being clear I'm a beginner to this website and Python.
– Nima Yeganeh
Nov 11 at 0:51
Also,sum
does not work on list of lists, it only adds level 1 sub elements.
– Rocky Li
Nov 11 at 0:51
add a comment |
up vote
-2
down vote
favorite
up vote
-2
down vote
favorite
Example: x = [[1,3,2],[4,5,6],[7,8,9]]
I attempted to use:
sum(x) / len(x)
but it seems to give me this error
(TypeError: unsupported operand type(s) for +: 'int' and 'list')
The list is inputted by the user with command: average_list() and could contain any amount of numbers per square brackets.
python
Example: x = [[1,3,2],[4,5,6],[7,8,9]]
I attempted to use:
sum(x) / len(x)
but it seems to give me this error
(TypeError: unsupported operand type(s) for +: 'int' and 'list')
The list is inputted by the user with command: average_list() and could contain any amount of numbers per square brackets.
python
python
edited Nov 11 at 0:49
Rocky Li
2,4781315
2,4781315
asked Nov 11 at 0:48
Nima Yeganeh
31
31
2
Do you want the average of complete list or average of lists inside list?
– Sanchit Kumar
Nov 11 at 0:50
I want the average of the complete list! Sorry for not being clear I'm a beginner to this website and Python.
– Nima Yeganeh
Nov 11 at 0:51
Also,sum
does not work on list of lists, it only adds level 1 sub elements.
– Rocky Li
Nov 11 at 0:51
add a comment |
2
Do you want the average of complete list or average of lists inside list?
– Sanchit Kumar
Nov 11 at 0:50
I want the average of the complete list! Sorry for not being clear I'm a beginner to this website and Python.
– Nima Yeganeh
Nov 11 at 0:51
Also,sum
does not work on list of lists, it only adds level 1 sub elements.
– Rocky Li
Nov 11 at 0:51
2
2
Do you want the average of complete list or average of lists inside list?
– Sanchit Kumar
Nov 11 at 0:50
Do you want the average of complete list or average of lists inside list?
– Sanchit Kumar
Nov 11 at 0:50
I want the average of the complete list! Sorry for not being clear I'm a beginner to this website and Python.
– Nima Yeganeh
Nov 11 at 0:51
I want the average of the complete list! Sorry for not being clear I'm a beginner to this website and Python.
– Nima Yeganeh
Nov 11 at 0:51
Also,
sum
does not work on list of lists, it only adds level 1 sub elements.– Rocky Li
Nov 11 at 0:51
Also,
sum
does not work on list of lists, it only adds level 1 sub elements.– Rocky Li
Nov 11 at 0:51
add a comment |
6 Answers
6
active
oldest
votes
up vote
0
down vote
accepted
You can sum
the sums of the inner lists:
x = [[1,3,2],[4,5,6],[7,8,9]]
s = sum(sum(a) for a in x)
l = sum(len(a) for a in x)
print(s / l) # 5.0
This is working, but how would you return 0 if the user left the input empty perhaps? For example x =
– Nima Yeganeh
Nov 11 at 1:06
@NimaYeganeh you can check iflen(x) == 0
. If it is, return 0, otherwise, return the average.
– slider
Nov 11 at 1:09
add a comment |
up vote
0
down vote
Use numpy
import numpy as np
x = np.array(x)
avg = np.sum(x)/np.size(x) # avg = 5
Why not use numpy's mean method?np.array(x).mean()
– Bill
Nov 11 at 1:23
add a comment |
up vote
0
down vote
x = [i for sublist in x for i in sublist]
avg = sum(x)/len(x)
Here is an answer after flattening the list
add a comment |
up vote
0
down vote
This provides you both, the average of the lists of list as well as the average of complete list.
x = [[1,3,2],[4,5,6],[7,8,9]]
new_list = [sum(l)/len(l) for l in x]
print(sum(new_list)/len(new_list))
Output:
5.0
add a comment |
up vote
0
down vote
A more scholastic way to do this is the following:
x = [[1,3,2],[4,5,6],[7,8,9]]
#stripping square brackets
elementsString = ''.join( c for c in str(x) if c not in '' )
total = 0
numberOfElements = 0
#converting the string numbers into int
for i in elementsString.split(','):
#using int but can be also float for example
i = int(i)
numberOfElements += 1
total += i
average = total/numberOfElements
print(average)
#5.0 is the answer in your case
add a comment |
up vote
-2
down vote
are you trying to find the average of the sum of the entire list of (x) or the sum of each cell?
Trying to find the average of the sum of the entire list.
– Nima Yeganeh
Nov 11 at 0:53
@isaac Please ask the clarifications in the comment section.
– Sanchit Kumar
Nov 11 at 0:57
add a comment |
6 Answers
6
active
oldest
votes
6 Answers
6
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
You can sum
the sums of the inner lists:
x = [[1,3,2],[4,5,6],[7,8,9]]
s = sum(sum(a) for a in x)
l = sum(len(a) for a in x)
print(s / l) # 5.0
This is working, but how would you return 0 if the user left the input empty perhaps? For example x =
– Nima Yeganeh
Nov 11 at 1:06
@NimaYeganeh you can check iflen(x) == 0
. If it is, return 0, otherwise, return the average.
– slider
Nov 11 at 1:09
add a comment |
up vote
0
down vote
accepted
You can sum
the sums of the inner lists:
x = [[1,3,2],[4,5,6],[7,8,9]]
s = sum(sum(a) for a in x)
l = sum(len(a) for a in x)
print(s / l) # 5.0
This is working, but how would you return 0 if the user left the input empty perhaps? For example x =
– Nima Yeganeh
Nov 11 at 1:06
@NimaYeganeh you can check iflen(x) == 0
. If it is, return 0, otherwise, return the average.
– slider
Nov 11 at 1:09
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
You can sum
the sums of the inner lists:
x = [[1,3,2],[4,5,6],[7,8,9]]
s = sum(sum(a) for a in x)
l = sum(len(a) for a in x)
print(s / l) # 5.0
You can sum
the sums of the inner lists:
x = [[1,3,2],[4,5,6],[7,8,9]]
s = sum(sum(a) for a in x)
l = sum(len(a) for a in x)
print(s / l) # 5.0
answered Nov 11 at 0:53
slider
7,1051129
7,1051129
This is working, but how would you return 0 if the user left the input empty perhaps? For example x =
– Nima Yeganeh
Nov 11 at 1:06
@NimaYeganeh you can check iflen(x) == 0
. If it is, return 0, otherwise, return the average.
– slider
Nov 11 at 1:09
add a comment |
This is working, but how would you return 0 if the user left the input empty perhaps? For example x =
– Nima Yeganeh
Nov 11 at 1:06
@NimaYeganeh you can check iflen(x) == 0
. If it is, return 0, otherwise, return the average.
– slider
Nov 11 at 1:09
This is working, but how would you return 0 if the user left the input empty perhaps? For example x =
– Nima Yeganeh
Nov 11 at 1:06
This is working, but how would you return 0 if the user left the input empty perhaps? For example x =
– Nima Yeganeh
Nov 11 at 1:06
@NimaYeganeh you can check if
len(x) == 0
. If it is, return 0, otherwise, return the average.– slider
Nov 11 at 1:09
@NimaYeganeh you can check if
len(x) == 0
. If it is, return 0, otherwise, return the average.– slider
Nov 11 at 1:09
add a comment |
up vote
0
down vote
Use numpy
import numpy as np
x = np.array(x)
avg = np.sum(x)/np.size(x) # avg = 5
Why not use numpy's mean method?np.array(x).mean()
– Bill
Nov 11 at 1:23
add a comment |
up vote
0
down vote
Use numpy
import numpy as np
x = np.array(x)
avg = np.sum(x)/np.size(x) # avg = 5
Why not use numpy's mean method?np.array(x).mean()
– Bill
Nov 11 at 1:23
add a comment |
up vote
0
down vote
up vote
0
down vote
Use numpy
import numpy as np
x = np.array(x)
avg = np.sum(x)/np.size(x) # avg = 5
Use numpy
import numpy as np
x = np.array(x)
avg = np.sum(x)/np.size(x) # avg = 5
answered Nov 11 at 0:53
Rocky Li
2,4781315
2,4781315
Why not use numpy's mean method?np.array(x).mean()
– Bill
Nov 11 at 1:23
add a comment |
Why not use numpy's mean method?np.array(x).mean()
– Bill
Nov 11 at 1:23
Why not use numpy's mean method?
np.array(x).mean()
– Bill
Nov 11 at 1:23
Why not use numpy's mean method?
np.array(x).mean()
– Bill
Nov 11 at 1:23
add a comment |
up vote
0
down vote
x = [i for sublist in x for i in sublist]
avg = sum(x)/len(x)
Here is an answer after flattening the list
add a comment |
up vote
0
down vote
x = [i for sublist in x for i in sublist]
avg = sum(x)/len(x)
Here is an answer after flattening the list
add a comment |
up vote
0
down vote
up vote
0
down vote
x = [i for sublist in x for i in sublist]
avg = sum(x)/len(x)
Here is an answer after flattening the list
x = [i for sublist in x for i in sublist]
avg = sum(x)/len(x)
Here is an answer after flattening the list
answered Nov 11 at 0:53
leeym
312213
312213
add a comment |
add a comment |
up vote
0
down vote
This provides you both, the average of the lists of list as well as the average of complete list.
x = [[1,3,2],[4,5,6],[7,8,9]]
new_list = [sum(l)/len(l) for l in x]
print(sum(new_list)/len(new_list))
Output:
5.0
add a comment |
up vote
0
down vote
This provides you both, the average of the lists of list as well as the average of complete list.
x = [[1,3,2],[4,5,6],[7,8,9]]
new_list = [sum(l)/len(l) for l in x]
print(sum(new_list)/len(new_list))
Output:
5.0
add a comment |
up vote
0
down vote
up vote
0
down vote
This provides you both, the average of the lists of list as well as the average of complete list.
x = [[1,3,2],[4,5,6],[7,8,9]]
new_list = [sum(l)/len(l) for l in x]
print(sum(new_list)/len(new_list))
Output:
5.0
This provides you both, the average of the lists of list as well as the average of complete list.
x = [[1,3,2],[4,5,6],[7,8,9]]
new_list = [sum(l)/len(l) for l in x]
print(sum(new_list)/len(new_list))
Output:
5.0
answered Nov 11 at 0:56


Sanchit Kumar
31117
31117
add a comment |
add a comment |
up vote
0
down vote
A more scholastic way to do this is the following:
x = [[1,3,2],[4,5,6],[7,8,9]]
#stripping square brackets
elementsString = ''.join( c for c in str(x) if c not in '' )
total = 0
numberOfElements = 0
#converting the string numbers into int
for i in elementsString.split(','):
#using int but can be also float for example
i = int(i)
numberOfElements += 1
total += i
average = total/numberOfElements
print(average)
#5.0 is the answer in your case
add a comment |
up vote
0
down vote
A more scholastic way to do this is the following:
x = [[1,3,2],[4,5,6],[7,8,9]]
#stripping square brackets
elementsString = ''.join( c for c in str(x) if c not in '' )
total = 0
numberOfElements = 0
#converting the string numbers into int
for i in elementsString.split(','):
#using int but can be also float for example
i = int(i)
numberOfElements += 1
total += i
average = total/numberOfElements
print(average)
#5.0 is the answer in your case
add a comment |
up vote
0
down vote
up vote
0
down vote
A more scholastic way to do this is the following:
x = [[1,3,2],[4,5,6],[7,8,9]]
#stripping square brackets
elementsString = ''.join( c for c in str(x) if c not in '' )
total = 0
numberOfElements = 0
#converting the string numbers into int
for i in elementsString.split(','):
#using int but can be also float for example
i = int(i)
numberOfElements += 1
total += i
average = total/numberOfElements
print(average)
#5.0 is the answer in your case
A more scholastic way to do this is the following:
x = [[1,3,2],[4,5,6],[7,8,9]]
#stripping square brackets
elementsString = ''.join( c for c in str(x) if c not in '' )
total = 0
numberOfElements = 0
#converting the string numbers into int
for i in elementsString.split(','):
#using int but can be also float for example
i = int(i)
numberOfElements += 1
total += i
average = total/numberOfElements
print(average)
#5.0 is the answer in your case
answered Nov 11 at 1:39


Antonino
1,25211224
1,25211224
add a comment |
add a comment |
up vote
-2
down vote
are you trying to find the average of the sum of the entire list of (x) or the sum of each cell?
Trying to find the average of the sum of the entire list.
– Nima Yeganeh
Nov 11 at 0:53
@isaac Please ask the clarifications in the comment section.
– Sanchit Kumar
Nov 11 at 0:57
add a comment |
up vote
-2
down vote
are you trying to find the average of the sum of the entire list of (x) or the sum of each cell?
Trying to find the average of the sum of the entire list.
– Nima Yeganeh
Nov 11 at 0:53
@isaac Please ask the clarifications in the comment section.
– Sanchit Kumar
Nov 11 at 0:57
add a comment |
up vote
-2
down vote
up vote
-2
down vote
are you trying to find the average of the sum of the entire list of (x) or the sum of each cell?
are you trying to find the average of the sum of the entire list of (x) or the sum of each cell?
answered Nov 11 at 0:52
isaac fuller
263
263
Trying to find the average of the sum of the entire list.
– Nima Yeganeh
Nov 11 at 0:53
@isaac Please ask the clarifications in the comment section.
– Sanchit Kumar
Nov 11 at 0:57
add a comment |
Trying to find the average of the sum of the entire list.
– Nima Yeganeh
Nov 11 at 0:53
@isaac Please ask the clarifications in the comment section.
– Sanchit Kumar
Nov 11 at 0:57
Trying to find the average of the sum of the entire list.
– Nima Yeganeh
Nov 11 at 0:53
Trying to find the average of the sum of the entire list.
– Nima Yeganeh
Nov 11 at 0:53
@isaac Please ask the clarifications in the comment section.
– Sanchit Kumar
Nov 11 at 0:57
@isaac Please ask the clarifications in the comment section.
– Sanchit Kumar
Nov 11 at 0:57
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53244856%2fhow-can-i-find-the-average-of-a-list-with-multiple-cells%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
D9WlorcGqTd6ozTyrMYjyDK6OD7xh
2
Do you want the average of complete list or average of lists inside list?
– Sanchit Kumar
Nov 11 at 0:50
I want the average of the complete list! Sorry for not being clear I'm a beginner to this website and Python.
– Nima Yeganeh
Nov 11 at 0:51
Also,
sum
does not work on list of lists, it only adds level 1 sub elements.– Rocky Li
Nov 11 at 0:51